The `using namespace` directive in C++ allows you to use all the identifiers from a specified namespace without needing to prefix them with the namespace name, simplifying code writing and readability.
Here's an example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Understanding C++ Namespaces
What is a Namespace in C++?
A namespace in C++ is a declarative region that provides a scope to the identifiers (the names of types, functions, variables, etc.) in your program. It serves as a container for organizing code, thus preventing naming conflicts. When different libraries define the same name (for instance, a function or a class), using namespaces allows you to avoid collisions.
Namespaces promote modularity and reusability in code. This is particularly important as programs grow larger and more complex. The need for a systematic way to manage identity—without unintended conflicts—is what makes namespaces crucial in C++ programming.
Importance of Namespaces in C++
Namespaces are vital in C++ for several reasons:
- Clarity: Namespaces group related functionality together, enhancing code readability.
- Conflict Avoidance: They prevent naming clashes when integrating multiple libraries or modules, especially when names are generic, like `print` or `function`.
- Organization: Keeping related declarations together helps in maintaining the code. For teams working on larger projects, namespaces facilitate clearer responsibilities.
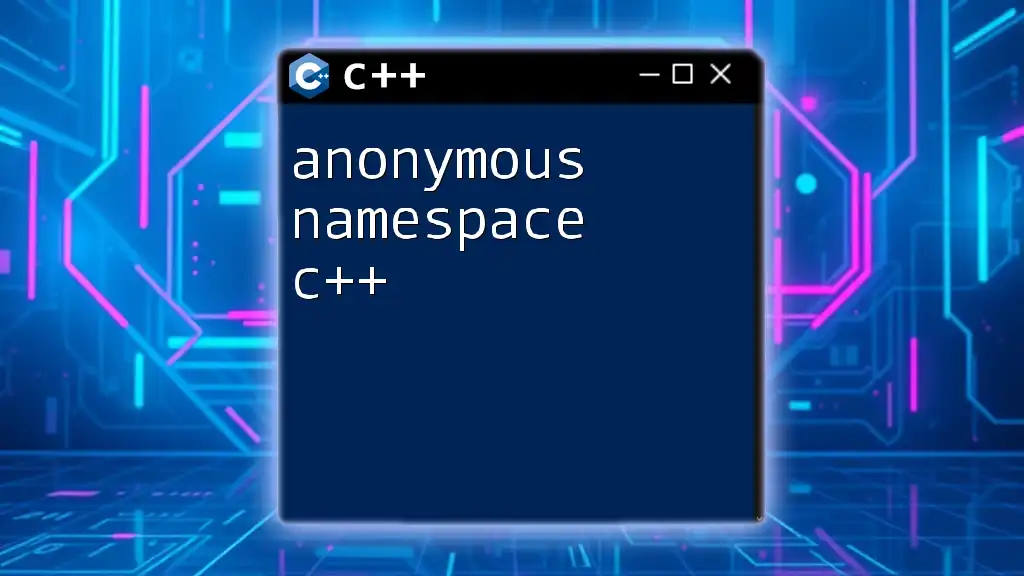
Using Namespace in C++
What Does 'Using Namespace' Mean?
The statement `using namespace` gives you the ability to access all the members of a namespace without needing to prefix them with the namespace’s name. This can significantly reduce the amount of typing required—making your code cleaner and easier to read.
However, it is essential to use this feature thoughtfully, as it can lead to ambiguities in larger projects with multiple libraries.
Common Namespaces in C++
C++ provides several standard namespaces, with the standard namespace (`std`) being the most commonly used. The `std` namespace contains all the built-in functionality provided by the C++ Standard Library, such as input/output stream objects and various utility functions.
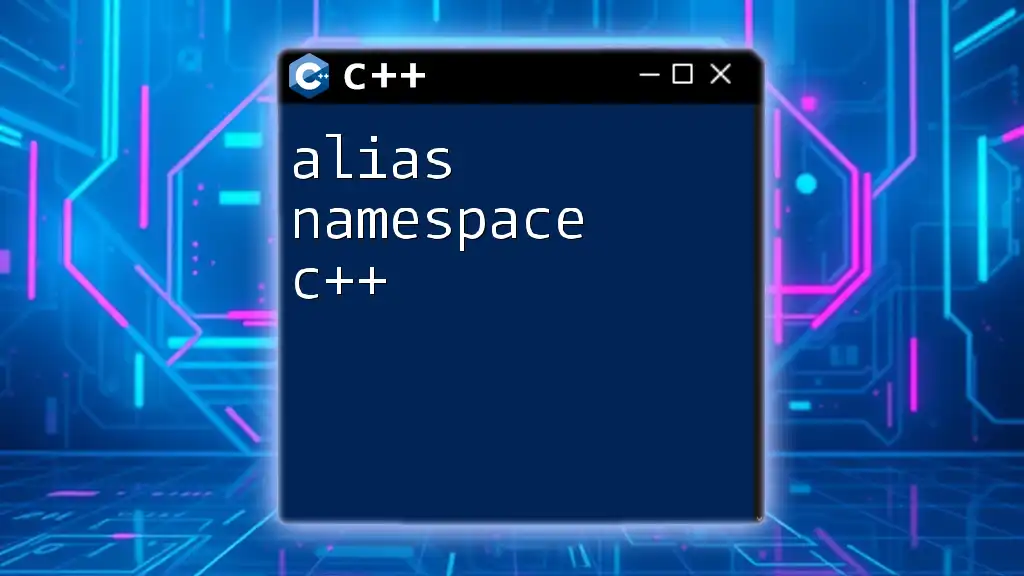
Syntax and Usage of `using namespace`
Basic Syntax of Using Namespace
The basic syntax for using a namespace is straightforward:
using namespace namespace_name;
For instance, to utilize the standard library, you would write:
using namespace std;
This declaration allows you to use standard library entities directly, without the prefix `std::`.
Applying Using Namespace in Practice
Example 1: Simplifying Standard Library Calls
The `using namespace` statement simplifies your code by allowing you to use standard functions without cumbersome prefixes. Here’s a basic example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!" << endl;
return 0;
}
In this snippet, `cout` and `endl` are used directly, thanks to `using namespace std`. Without this directive, you would have had to write `std::cout` and `std::endl`, which adds length without increasing clarity.
Example 2: Creating Personal Namespaces
You can also define your own namespaces to group related functions or classes. Here’s how you can create and use a personal namespace:
namespace MyNamespace {
void display() {
cout << "Hello from MyNamespace!" << endl;
}
}
using namespace MyNamespace;
int main() {
display();
return 0;
}
In this example, the function `display` is defined within `MyNamespace`, allowing you to call it directly without the namespace prefix because of the `using namespace MyNamespace;` directive.
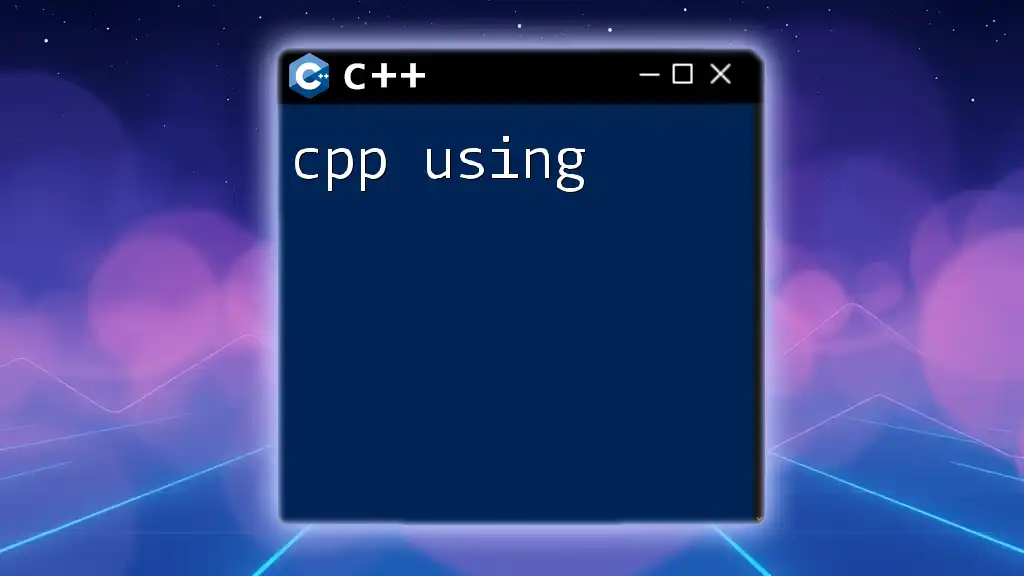
When to Use `using namespace`
Guidelines for Using Namespace
While the `using namespace` directive makes coding simpler, it's advisable to use it judiciously. The best practice is to apply it within the implementation files (`.cpp`) rather than in header files (`.h`). This is because declarations in header files get included in multiple translation units, which can lead to unintended name clashes.
Risks and Considerations
The primary risk of using `using namespace` indiscriminately is the potential for name clashes. For example, if two libraries contain a function named `calculate` and you use `using namespace` for both, the compiler won't know which `calculate` you are referring to.
To prevent this, consider using specific namespace declarations, or even explicitly referring to the namespace of the function you want to use. This conserves clarity while avoiding conflicts.
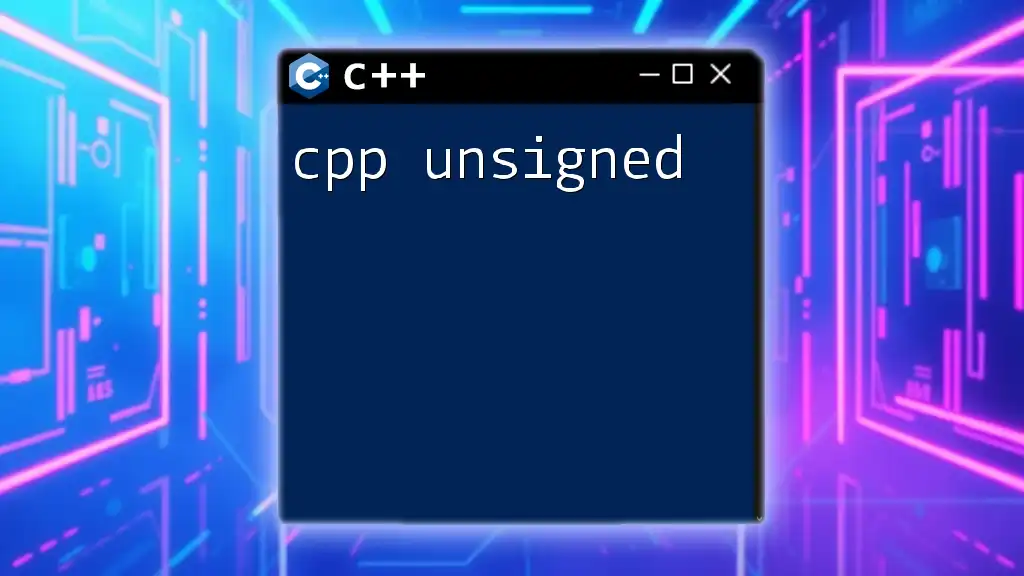
Alternatives to `using namespace`
Scoped Usage of Namespaces
When concerned about conflicts, using the scope resolution operator (`::`) can be a safer alternative. This method allows you to refer to namespaces explicitly, reducing ambiguity.
For example:
#include <iostream>
int main() {
std::cout << "Using scope resolution!" << std::endl;
return 0;
}
This code clearly indicates that `cout` and `endl` belong to the `std` namespace, providing explicit clarity with no risk of conflict.
Using Specific Names
Another option is to declare only the specific names you require. This minimizes the risk of conflicts while retaining the convenience of shorter code:
#include <iostream>
using std::cout;
using std::endl;
int main() {
cout << "Using specific names!" << endl;
return 0;
}
This approach allows you to use `cout` and `endl` directly, without pulling in everything from the `std` namespace.
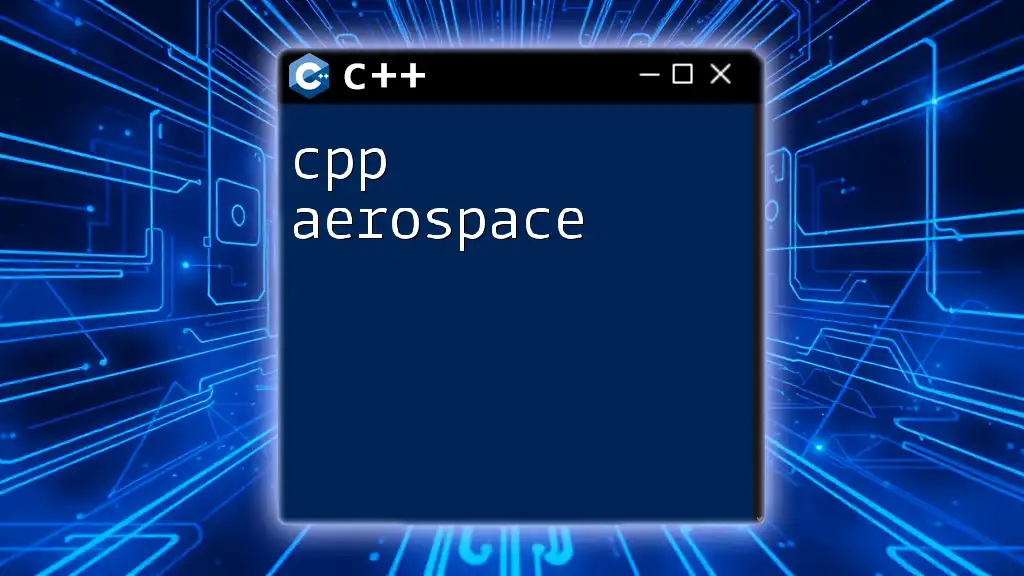
Conclusion
In summary, the cpp using namespace directive is a powerful tool that can enhance your coding experience by making it cleaner and simpler. However, like all powerful tools, it should be used thoughtfully. Striking a balance between convenience and maintaining clarity is crucial, especially in larger codebases.
Applying best practices—such as limiting its use in header files, exploring scoped usage, and favoring specific declarations—will help you avoid potential pitfalls while reaping the benefits.
Embrace the use of namespaces and the `using namespace` directive in C++ to organize your code effectively while preventing naming conflicts, leading to cleaner, more maintainable projects.
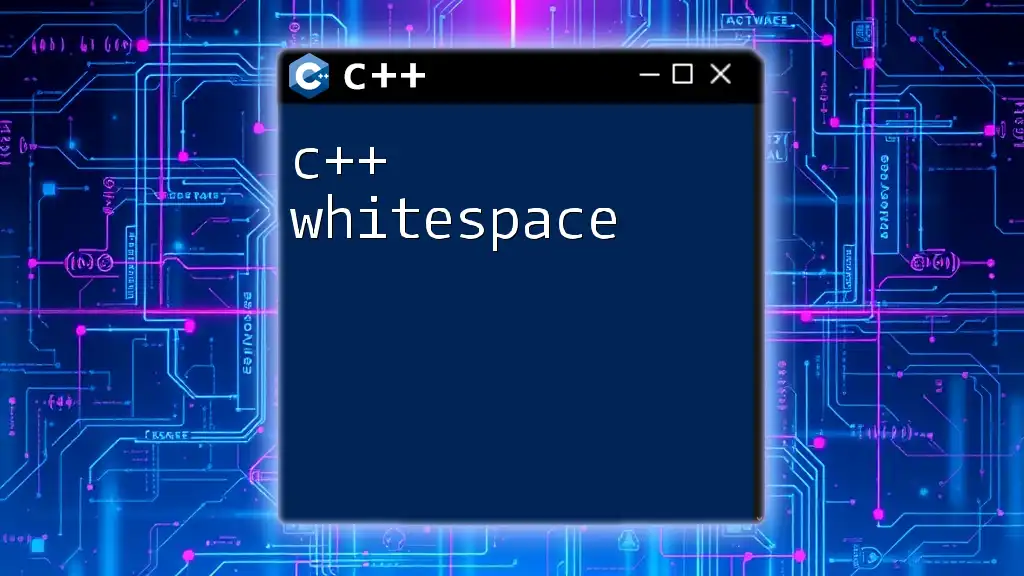
Additional Resources
For further learning, consider reading books like "The C++ Programming Language" by Bjarne Stroustrup, or exploring resources from the official C++ documentation. These will provide deeper insights into namespaces and effective C++ programming.