In C++, printing to the console is commonly done using the `std::cout` object along with the insertion operator (`<<`) to output text and variables.
Here's an example code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding CPP Printing
What is CPP Printing?
CPP printing refers to the process of generating output to the console using C++. It plays a crucial role in debugging and enhancing user interaction within applications. Understanding how to effectively use C++ printing commands is essential for developing efficient and user-friendly programs.
Overview of Printing in C++
In C++, printing involves utilizing the output stream to convey information to the user or debugging purposes. The basic syntax for printing is straightforward, typically involving the `std::cout` object and the insertion operator `<<`. The common use cases for CPP printing include displaying variable values, formatted outputs, and debugging information.
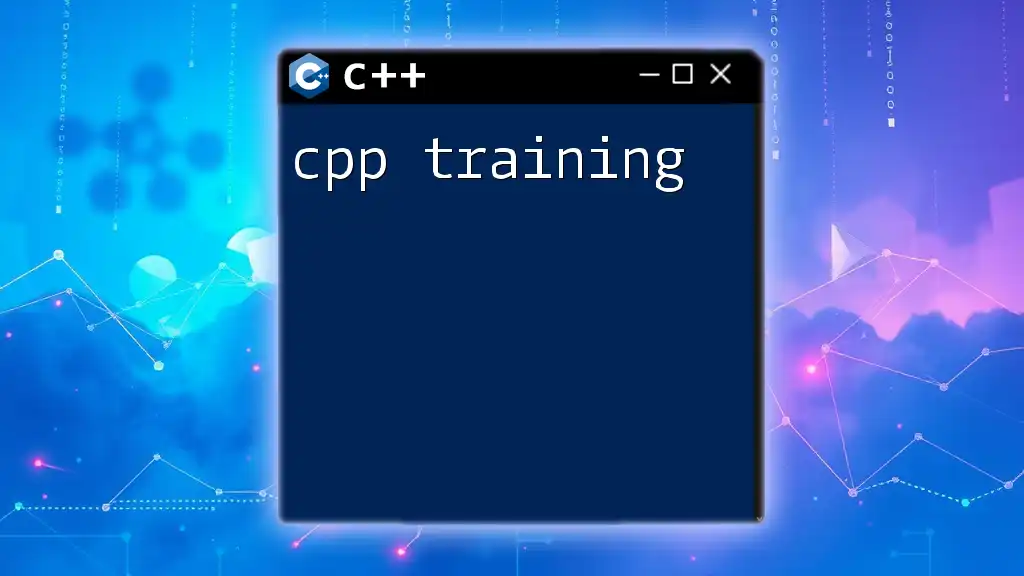
Using Standard Output Stream: `cout`
Introduction to `cout`
`cout` stands for "character output" and is part of the standard library in C++. It is utilized to send output to the standard output device, usually the console. By understanding `cout`, programmers can efficiently communicate information to users.
Basic Printing with `cout`
To begin printing with `cout`, the simplest example is as follows:
std::cout << "Hello, World!" << std::endl;
In this code, "Hello, World!" is printed to the console. The operator `<<` directs the string to the `cout` stream, and `std::endl` is used to flush the output buffer while also inserting a newline character.
Printing Variables and Data Types
Printing different data types in C++ can be done seamlessly using `cout`. Here’s an example for various data types:
int num = 10;
std::cout << "The number is: " << num << std::endl;
double pi = 3.14159;
std::cout << "The value of pi is: " << pi << std::endl;
char letter = 'A';
std::cout << "The first letter is: " << letter << std::endl;
std::string name = "Alice";
std::cout << "My name is: " << name << std::endl;
This demonstrates how to efficiently print integers, floating-point numbers, characters, and strings in a user-friendly manner. The ability to use the same syntax for different types enhances code readability.
Manipulating Output with Manipulators
C++ provides manipulators that allow programmers to format their output dynamically. Common manipulators include `std::endl` for newline, `std::fixed` for fixed-point notation, and `std::setprecision()` for controlling the number of decimal places. Here’s an example of using manipulators:
#include <iomanip>
double pi = 3.14159;
std::cout << std::fixed << std::setprecision(2) << pi << std::endl;
In this snippet, `std::fixed` ensures that the number is displayed in fixed-point notation, while `setprecision(2)` limits the output to two decimal places. This control allows for better clarity and readability in numerical outputs.
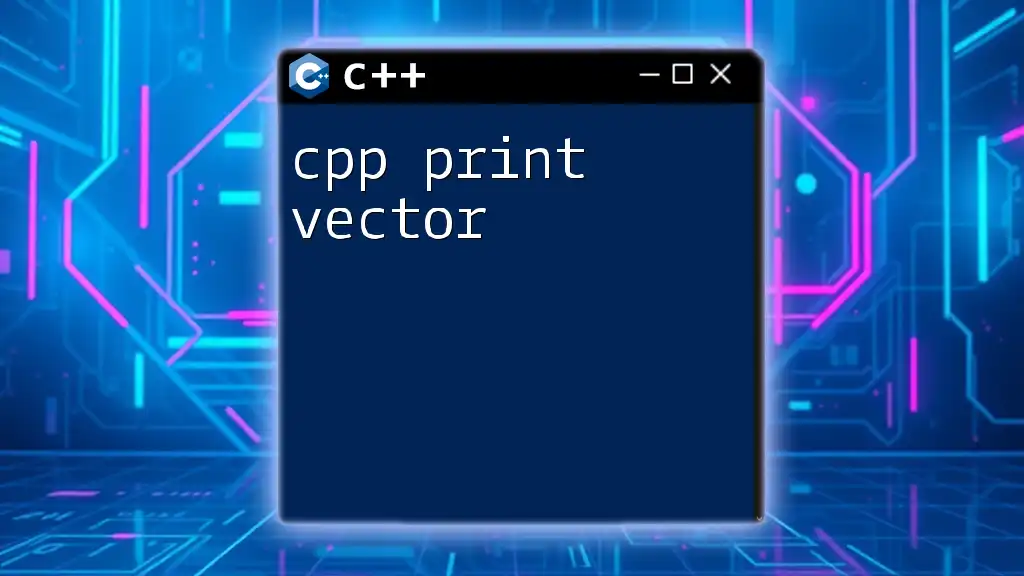
Advanced C++ Printing Techniques
Printing Arrays and Collections
Printing arrays can be a bit more complex than printing single variables, but it is essential for displaying collections of data. Here’s how to print a simple array:
int arr[] = {1, 2, 3, 4, 5};
for (int i : arr) {
std::cout << i << " ";
}
std::cout << std::endl;
This code demonstrates a range-based for loop to iterate through the array, outputting each element followed by a space.
When working with C++ Standard Template Library (STL) containers like `std::vector`, the approach remains similar:
#include <vector>
std::vector<int> vec = {1, 2, 3, 4, 5};
for (const auto &item : vec) {
std::cout << item << " ";
}
std::cout << std::endl;
Using a `vector` provides flexibility and ease of use when handling collections of data.
Printing Custom Objects
In C++, objects of user-defined types can be printed by overloading the insertion operator `<<`. This allows custom types to be directly output using `cout`. Here’s an example:
class Point {
public:
int x, y;
friend std::ostream& operator<<(std::ostream& os, const Point& p) {
os << "(" << p.x << ", " << p.y << ")";
return os;
}
};
// Usage
Point p1 = {3, 4};
std::cout << "Point coordinates: " << p1 << std::endl;
In this code, we define a `Point` class and overload the `<<` operator, which facilitates clear and intuitive printing of custom objects.
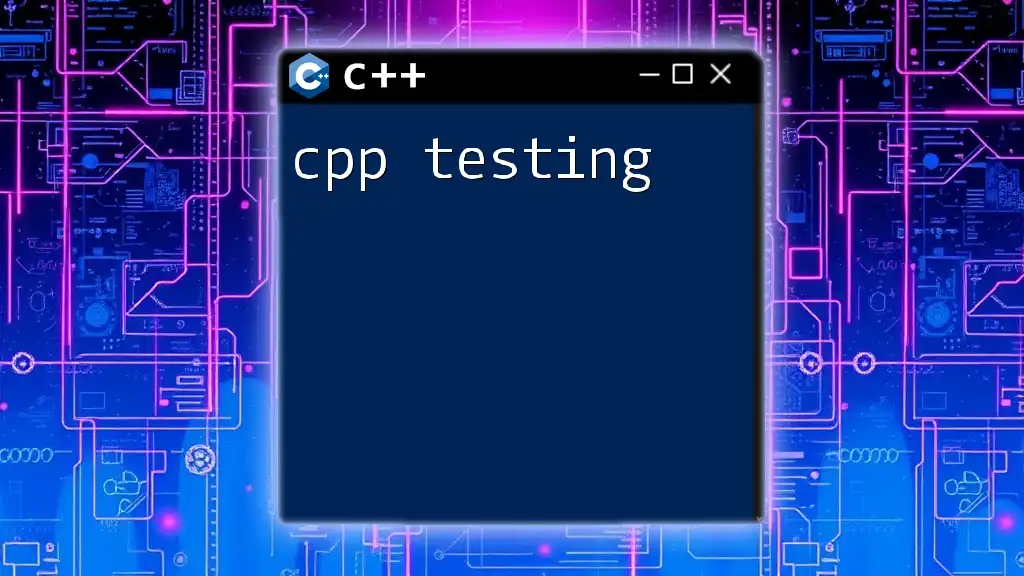
Error Handling in Printing
Understanding Output Errors
While outputting data, various errors can occur, such as outputting unsupported types or exceeding buffer limits. These issues can lead to unexpected behavior during runtime. Vigilance during the printing process is crucial.
Using Exception Handling for Robust Printing
To make outputs more robust, C++ allows the use of exception handling. Here’s how you can wrap your printing commands in a try-catch block to gracefully handle any errors:
#include <stdexcept>
try {
std::cout << "Some output" << std::endl;
// Simulated error
throw std::runtime_error("Error in printing");
} catch (const std::exception &e) {
std::cerr << e.what() << std::endl;
}
This example shows how to capture and respond to exceptions, ensuring that applications handle errors without crashing unexpectedly.
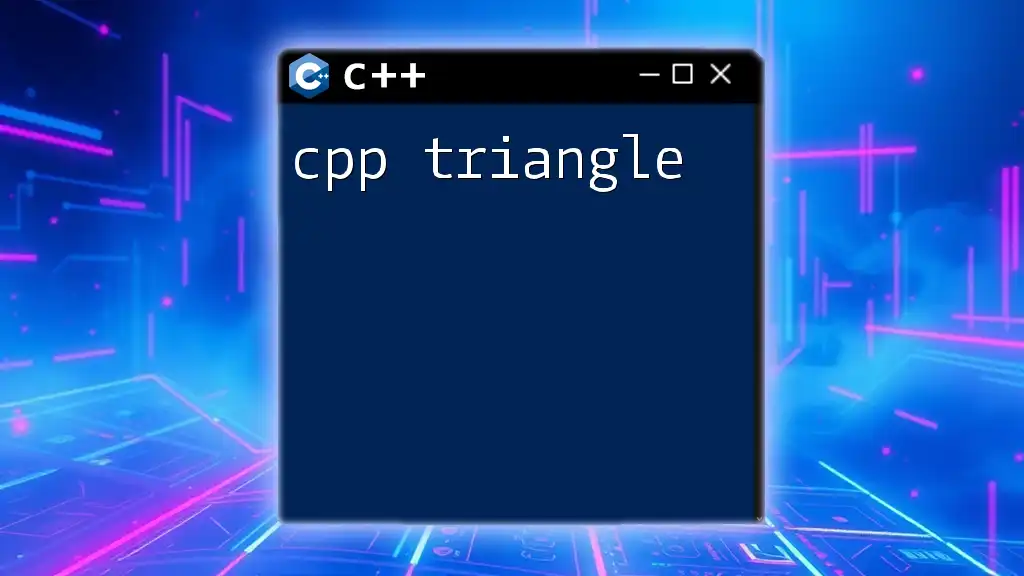
Best Practices for CPP Printing
Code Readability
When printing outputs, clear formatting enhances code readability. Using consistent spacing and indentation in your output strings can improve the user experience and debugging efficiency.
Performance Considerations
Excessive printing can slow down program execution. For applications that require frequent outputs, consider minimizing console printing, especially within loops, or use conditional logging to reduce the impact on performance.
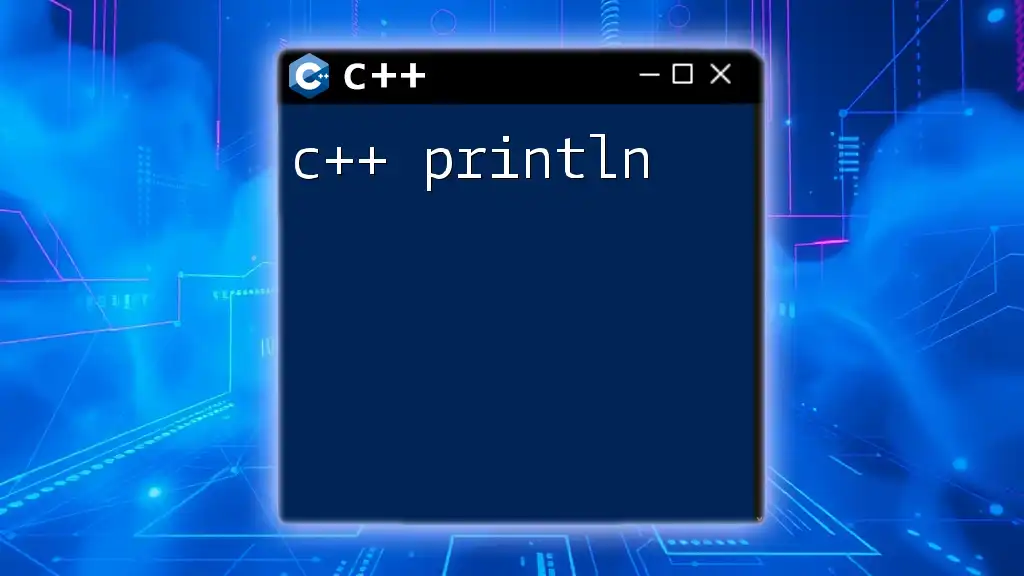
Conclusion
Summarizing CPP Printing Techniques
In summary, CPP printing is a powerful feature of the C++ language. By mastering `cout`, manipulators, and custom object outputs, developers can effectively convey information and debug their applications. Being aware of potential pitfalls in output and employing best practices ensure that CPP printing is both efficient and user-friendly.
Additional Resources and References
For those looking to dive deeper into C++ printing and output techniques, consider exploring more resources, including official documentation on `iostream`, tutorials specific to formatting output, and books on advanced C++ programming.