The `ofstream` class in C++ is used to create and manage output file streams for writing data to files.
#include <fstream>
int main() {
std::ofstream outfile("example.txt");
outfile << "Hello, World!" << std::endl;
outfile.close();
return 0;
}
Understanding ofstream in C++
What is ofstream?
ofstream stands for "output file stream" and is a part of the standard C++ library used to write data to files. The primary purpose of ofstream is to enable file output, allowing programmers to interact with the filesystem by creating and writing to files. File I/O is crucial in many applications, as it enables persistent data storage and retrieval.
How does ofstream work?
In C++, the concept of file streams enables you to handle input and output operations for files. The three primary types of file streams are ifstream (input file stream), ofstream (output file stream), and fstream (both input and output). ofstream focuses specifically on writing data to files.
Mechanism-wise, the ofstream operates by buffering data before writing it to the disk, ensuring efficient writing with minimal system calls.
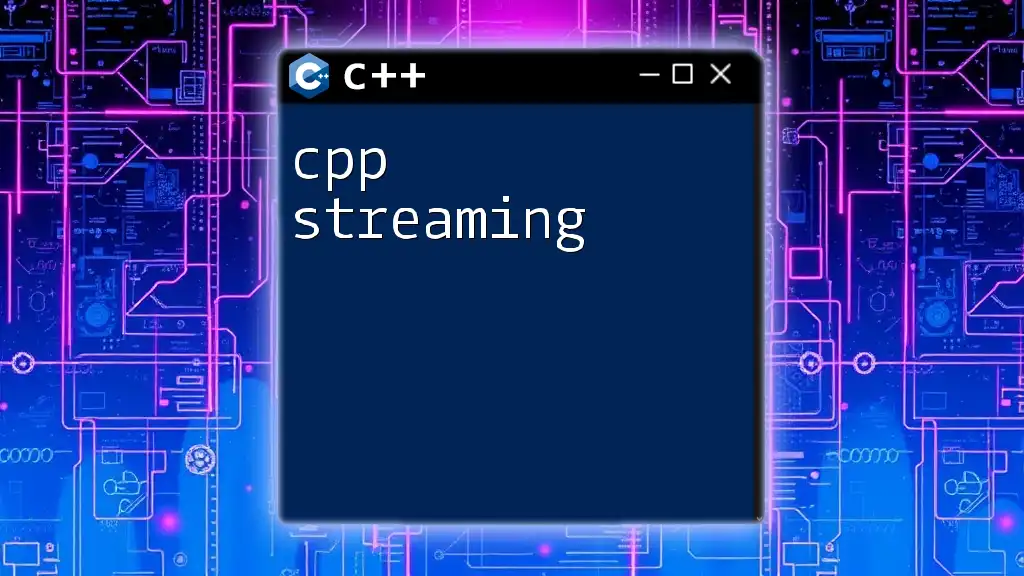
Setting Up ofstream in C++
Including the Necessary Header Files
To use ofstream, you need to include the appropriate header in your program. The following directive is essential:
#include <fstream>
This line ensures that you can utilize the ofstream functionalities.
Creating an Instance of ofstream
Creating an instance of ofstream is straightforward. You can initialize it with the name of the file you want to create or write to. The syntax is as follows:
ofstream outFile("example.txt");
In this example, an instance of ofstream named outFile is created, geared to write to a file named example.txt. If the file already exists, it will be truncated to zero length, meaning any existing data will be lost. If the file does not exist, it will be created automatically.
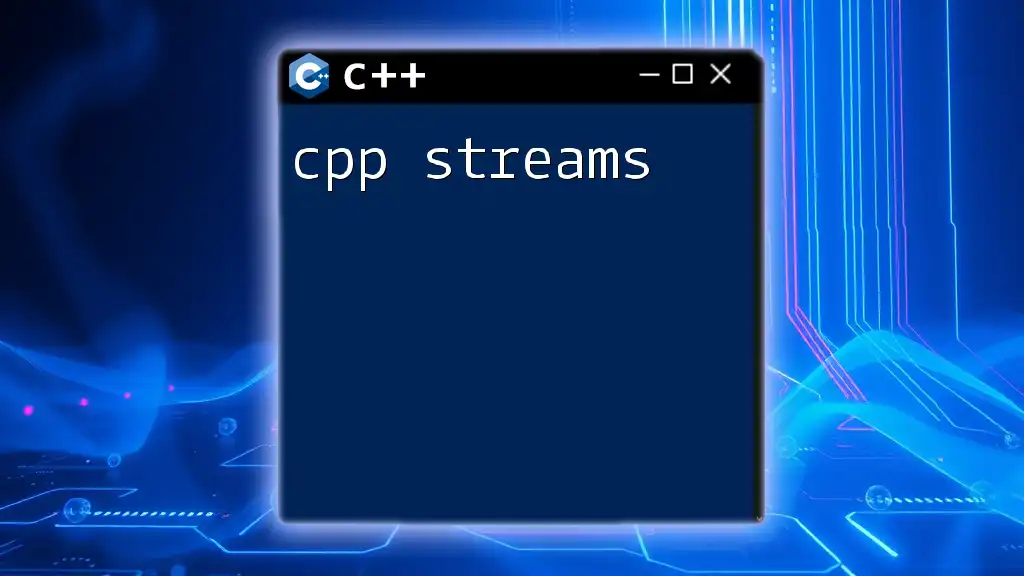
Writing Data to Files with ofstream
Basic Writing Operations
The simplest way to write data to a file using ofstream is by using the insertion operator (`<<`). For example:
outFile << "Hello, World!";
This command writes the text "Hello, World!" to the file. The ofstream instance will handle the necessary buffering and eventual writing to the disk.
Flushing and Closing Files
Writing to files may not always occur immediately, as data is often buffered for performance. Therefore, it is important to flush the buffers to ensure no data is lost. You can do this manually, but it is often best practice to close the file after writing:
outFile.close();
This command not only flushes any remaining data but also frees up system resources associated with the file.
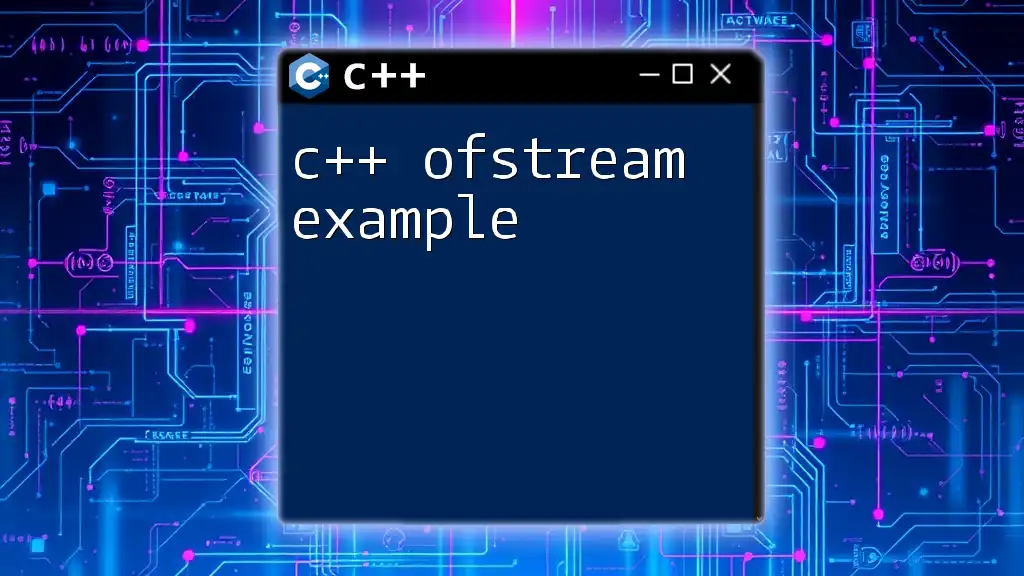
Advanced Features of ofstream
Working with Different Data Types
The ofstream class can handle various data types, making it versatile. For instance, you can write integers, characters, and strings seamlessly:
int age = 25;
outFile << "Age: " << age;
This command writes "Age: 25" to the file. Using the insertion operator with different data types demonstrates the flexibility of ofstream.
Formatting Outputs
Formatting your output is essential, especially when dealing with floating-point numbers or structured data. You can use manipulators from the `<iomanip>` library:
#include <iomanip>
outFile << std::fixed << std::setprecision(2) << 3.14159; // Output: 3.14
Here, std::fixed ensures the number is displayed in fixed decimal notation, while std::setprecision(2) limits it to two decimal places. Proper formatting enhances the readability of output files.
Error Handling in ofstream
Always ensure your ofstream instance points to a valid file before performing write operations. You can check if the file opened successfully using:
if (!outFile) {
std::cerr << "Error opening file!" << std::endl;
}
This snippet checks the ifstream instance and outputs an error message if the file could not be opened, which is crucial for robust programming practices.
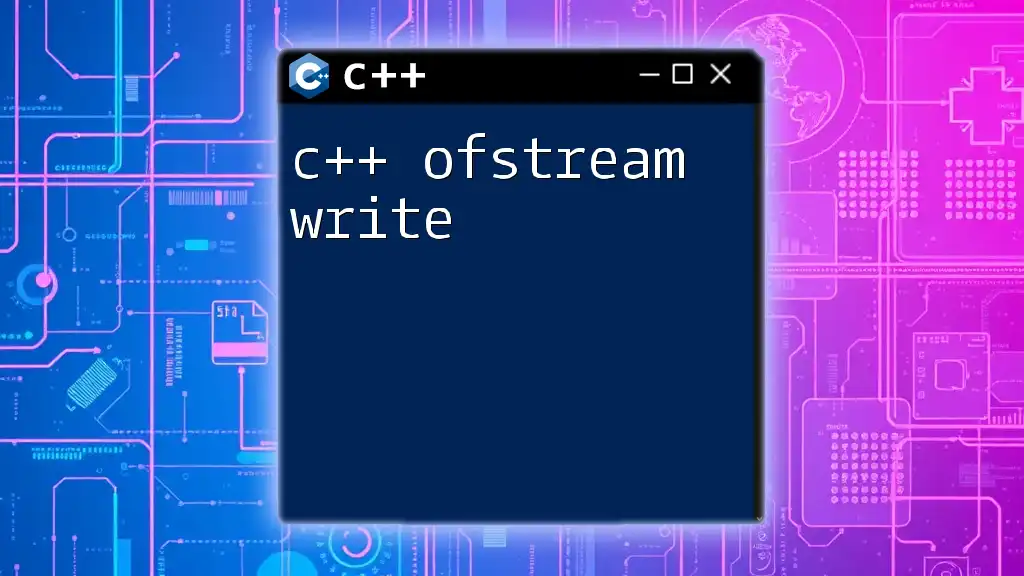
Practical Examples of Using ofstream
ofstream c++ Example: Creating a Simple Log File
Creating a log file is a practical scenario where ofstream shines. Here’s how to create a simple log file:
ofstream logFile("log.txt");
logFile << "Log Entry: Operation Successful." << std::endl;
logFile.close();
This code creates a log file named log.txt and writes an entry to it. This demonstrates a real-life application of ofstream in maintaining activity logs.
ofstream c++ Example: Writing User Input to File
Another common use for ofstream is to capture user input and write it to a file. Here’s how this could work:
string userInput;
cout << "Enter some text: ";
getline(cin, userInput);
outFile << userInput << std::endl;
In this example, the program prompts the user for input. The entered text is then written to the file, showcasing ofstream's capability to handle dynamic content.
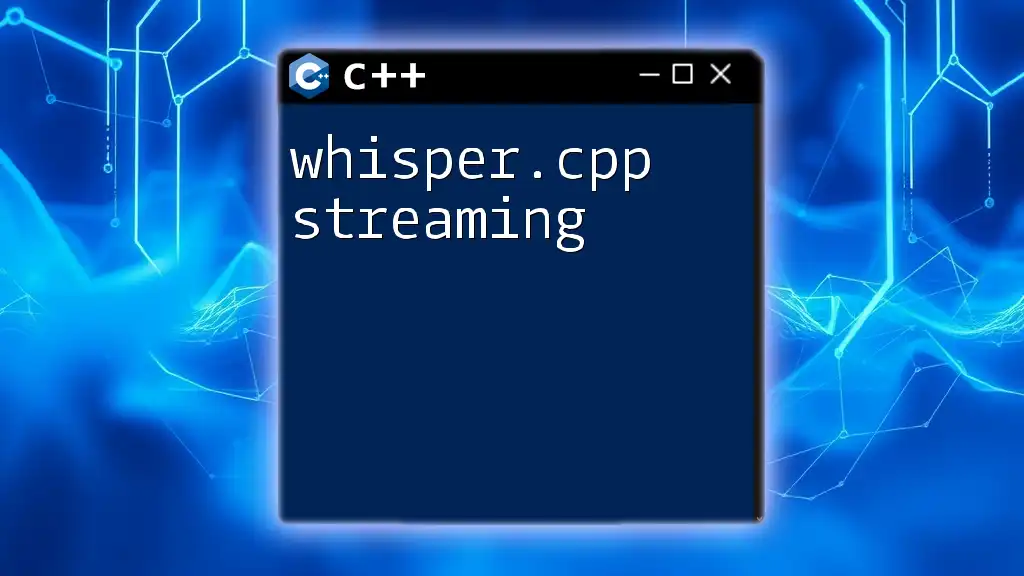
Best Practices for Using ofstream
Optimal File Naming Conventions
When creating files, consider clear and meaningful file names. This makes it easier to manage multiple files later. Using file extensions judiciously (e.g., `.txt`, `.log`) aids in identifying file types.
Resource Management and Cleanup
Always ensure that files are closed after you're done writing. Failing to do so can lead to resource leaks, which might eventually cause crashes or file corruption. Regularly practice good resource management habits in your coding.
Performance Considerations
While ofstream handles most performance concerns, understanding when to use buffered versus unbuffered output can enhance efficiency, particularly in large-scale data operations. Always benchmark your file I/O operations, especially in performance-sensitive applications.
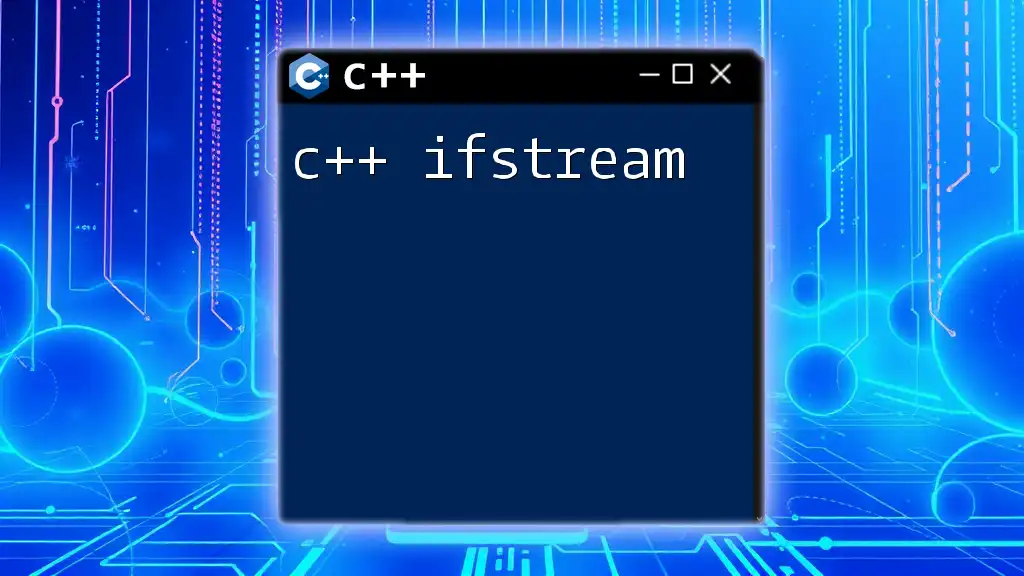
Conclusion
Recap of Key Points
The ofstream is an essential component in C++ when it comes to writing data to files. It offers various functionalities, including error handling, data formatting, and dynamic data handling. Understanding these key features is vital for effective file management in any C++ application.
Encouragement to Experiment
Now that you have a comprehensive understanding of cpp ofstream, I encourage you to experiment with these concepts. Practical experience is the best way to learn. Links to additional resources can further enhance your skills and understanding of file handling in C++.