C++ logging allows developers to output messages to the console or log files, which can be crucial for debugging and tracking application behavior.
Here's a simple example of how to implement basic logging in C++:
#include <iostream>
#include <fstream>
#include <string>
void logMessage(const std::string& message) {
std::ofstream logFile("log.txt", std::ios_base::app); // Open log file in append mode
if (logFile.is_open()) {
logFile << message << std::endl; // Write message to log file
logFile.close();
} else {
std::cerr << "Unable to open log file!" << std::endl; // Error handling
}
}
int main() {
logMessage("Application started.");
// Other code...
logMessage("Application finished.");
return 0;
}
What is Logging?
Logging is a critical component in software development that allows developers to track the flow of a program and record important events. It serves as a valuable tool for troubleshooting and monitoring applications during both development and production phases.
While debugging involves stepping through code and inspecting state at runtime, logging provides a persistent record of events and actions. When errors arise or unexpected behavior occurs, logs can be invaluable in diagnosing issues.
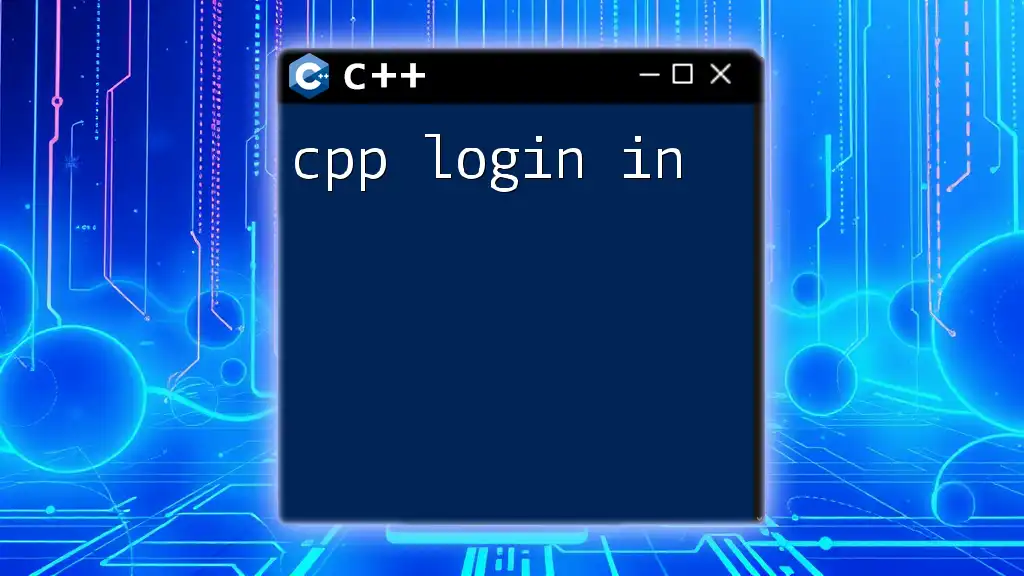
Overview of C++ Logging
C++ logging has evolved over time, with numerous libraries offering a variety of features. Today, developers have access to sophisticated logging mechanisms that aid in producing high-performance applications.
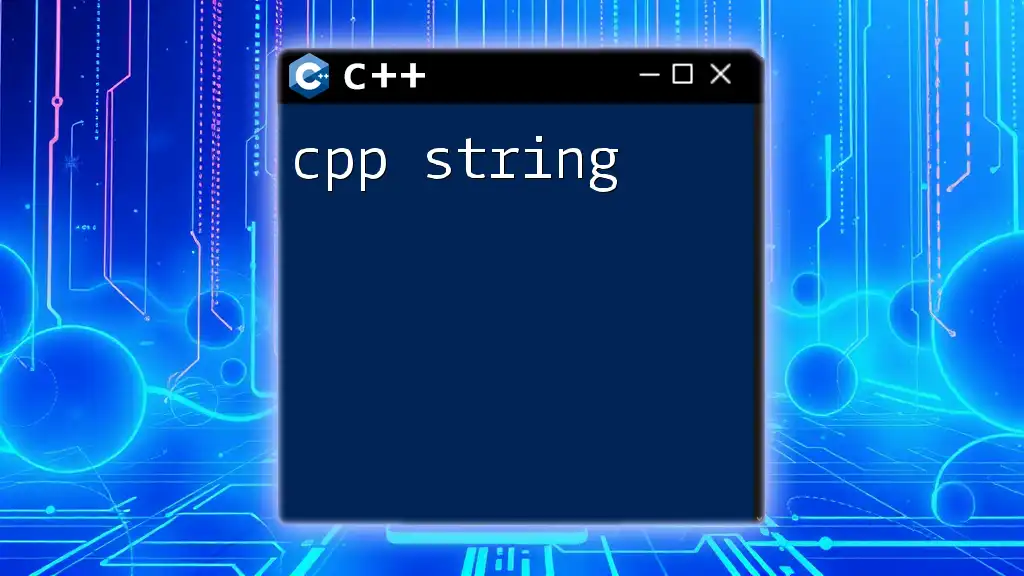
What is a C++ Logger?
A C++ logger is a component or a library that helps developers log messages in a structured way. The purpose of a logger is to record information regarding the application's operation, making it easier to monitor its performance and address issues.
There are two primary types of loggers in C++:
-
Basic Loggers: These typically offer minimal functionality and are suitable for simple applications. They log messages directly to the console or a file without advanced features.
-
Advanced Loggers: These libraries provide a rich set of features, including customizable log levels, asynchronous logging, and integration with various output sinks. They are designed to manage complex applications efficiently.
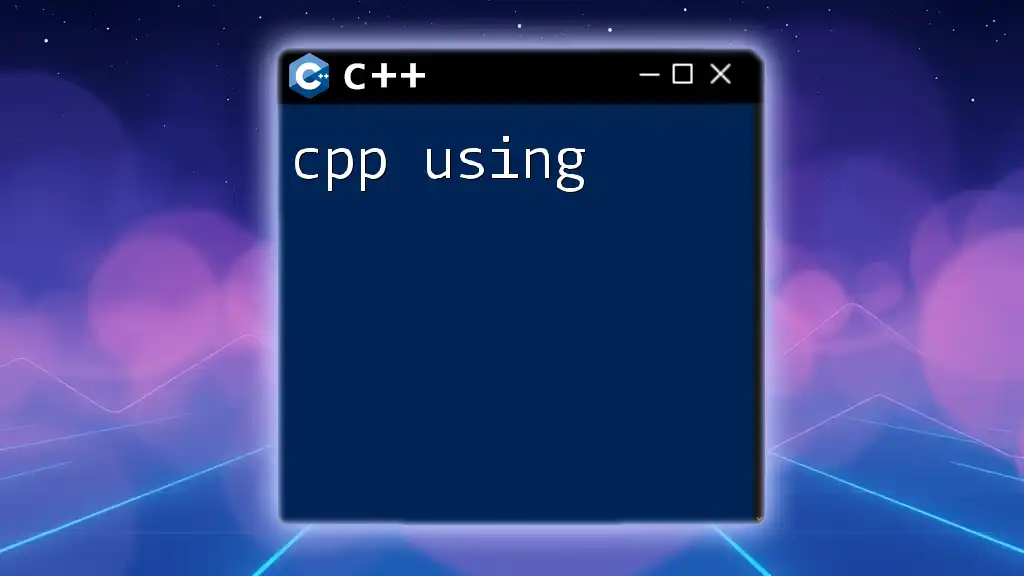
Advantages of Using a Logging Library in C++
Using a logging library in C++ can enhance both development speed and application performance. The advantages include:
-
Efficiency and Performance: Well-designed logging libraries implement optimized mechanisms, ensuring that logging has a minimized performance impact on applications.
-
Scalability and Maintainability: With standardized logging practices, applications can be scaled more easily. Logging libraries often come with robust features that help maintain large code bases.
Popular C++ Logging Libraries
There are several popular logging libraries in C++, each with its strengths:
-
spdlog: A fast C++ logging library that is easy to use and supports various formatting options and asynchronous logging.
-
Boost.Log: Part of the Boost Libraries, it provides highly configurable logging with a steep learning curve but offers extensive features.
-
glog: Google’s logging library, suited for applications requiring advanced logging features such as stack traces.
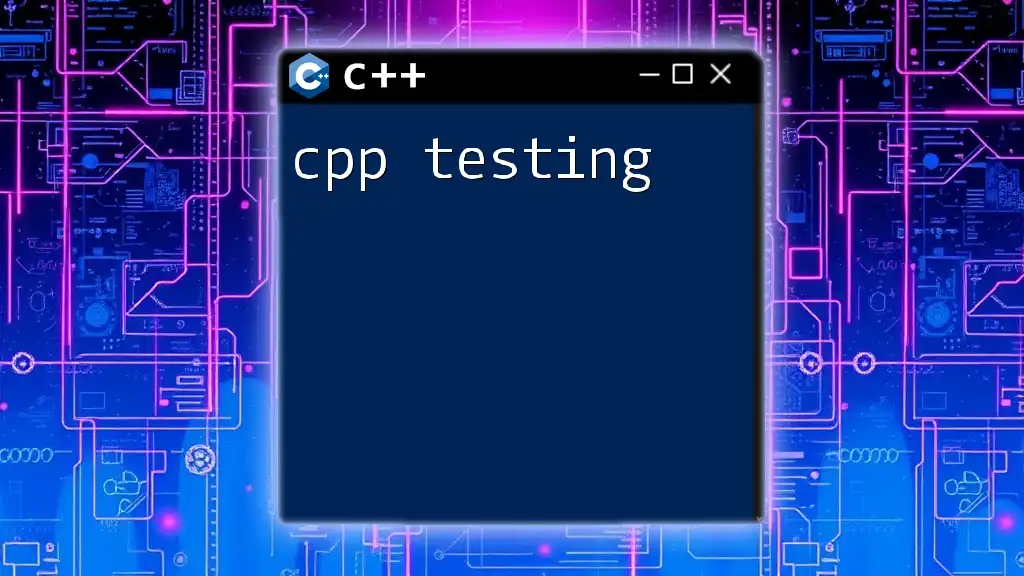
Getting Started with C++ Logging
To introduce logging into your C++ application, you first need to set up a logging library. Let's take `spdlog` as an example.
Setting Up a C++ Logging Library
Installation of spdlog can be done via package managers or by including it in your project manually. Below is a sample code snippet to get your logger ready:
#include <spdlog/spdlog.h>
int main() {
auto logger = spdlog::stdout_color_mt("console");
logger->info("Welcome to C++ Logging!");
return 0;
}
This code initializes a logger that outputs messages with color formatting directly to the console.
Basic Logging Usage
Once your logger is set up, you can start logging messages. Here's a simple log example:
logger->info("This is an info message.");
logger->error("This is an error message.");
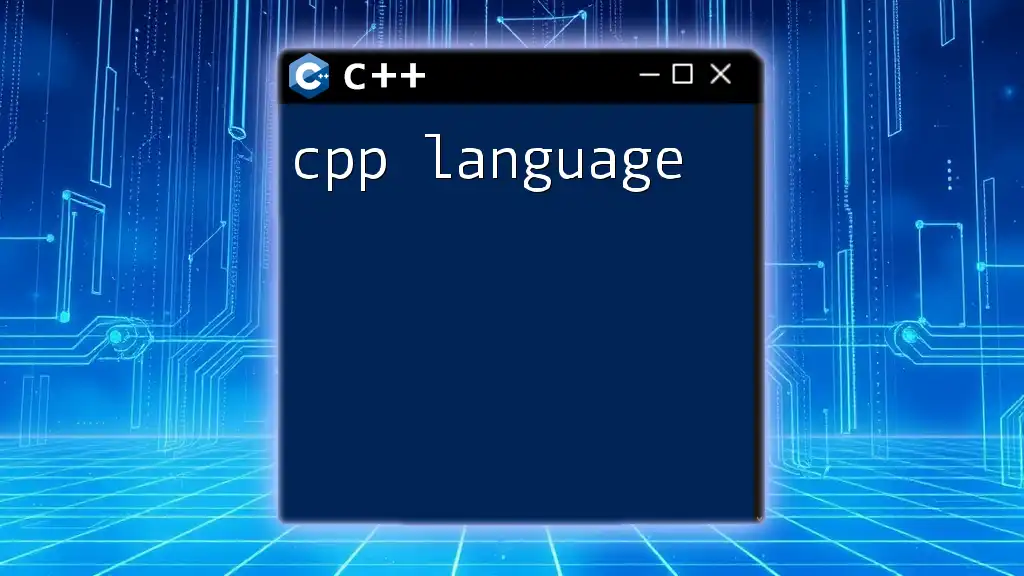
Understanding Log Levels
Logging messages is more than just writing texts; it involves categorizing messages with different log levels.
Definitions of Various Log Levels:
- Trace: Detailed information typically used for diagnosing problems.
- Debug: Information used for debugging purposes.
- Info: Standard messages that describe the normal functioning of the application.
- Warn: Indications of potentially harmful situations.
- Error: Logging of problematic situations that prevented a specific operation from being executed.
- Critical: Severe error events that might prevent the application from continuing to run.
Choosing the Right Log Level
Choosing the correct log level is crucial to maintain clarity in output. Use `info` for typical operations, `warn` for conditions that might lead to issues, and `error` for exceptions or faults that should be addressed. For example:
logger->debug("This is a debug message.");
logger->warn("This is a warning message!");
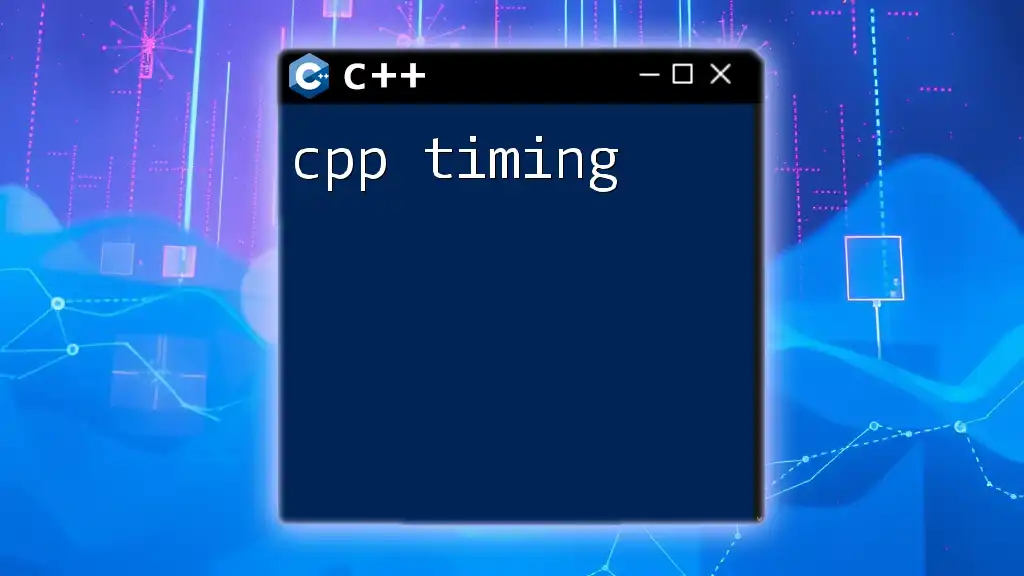
Advanced Logging Configuration
Setting Log Formats
Customizing log messages enhances readability. For example, using a pattern for your logs will refine your output:
spdlog::set_pattern("%^[%T] %n: %v%$"); // Set pattern for output
File Logging
For persistent logging, configuring file output is essential. Here’s how to log messages to a file:
auto file_logger = spdlog::basic_logger_mt("file_logger", "logs.txt");
file_logger->info("This will be logged to a file.");
Asynchronous Logging
Asynchronous logging can greatly improve performance by allowing the logging process to run in a separate thread. Configure logging as follows:
logger = spdlog::create_async<spdlog::sinks::simple_file_sink_mt>("async_file_logger", "async_logs.txt");
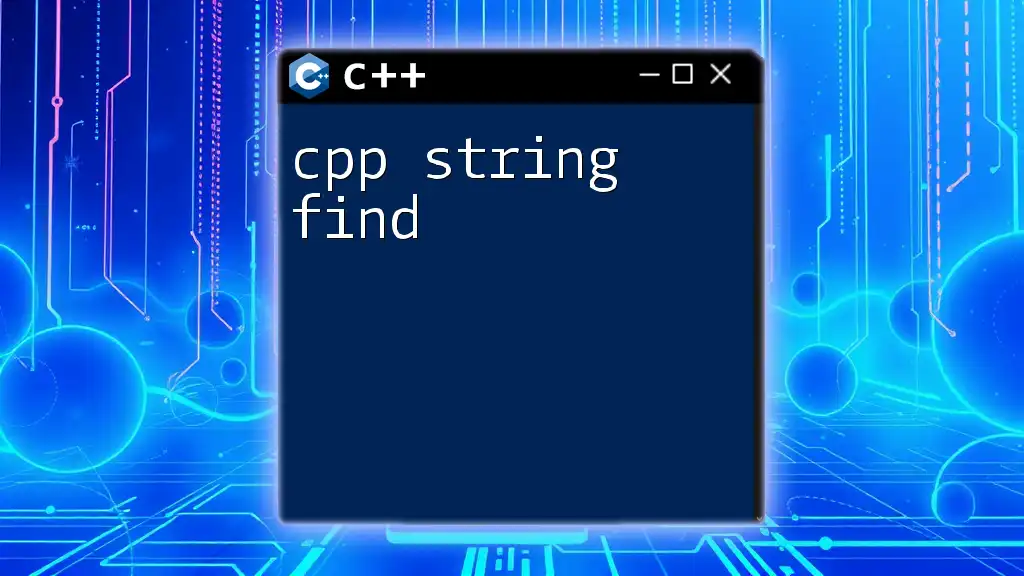
Handling Log Messages
Formatting Messages
Effective logging often involves formatted messages to ensure clarity. Use special formatting syntax to embed variable values within your logs, making them more informative.
Including Contextual Information
Incorporating contextual data, such as timestamps and thread identifiers, aids in tracking application state. Here's an example:
logger->info("Thread ID: {} - Log message", std::this_thread::get_id());
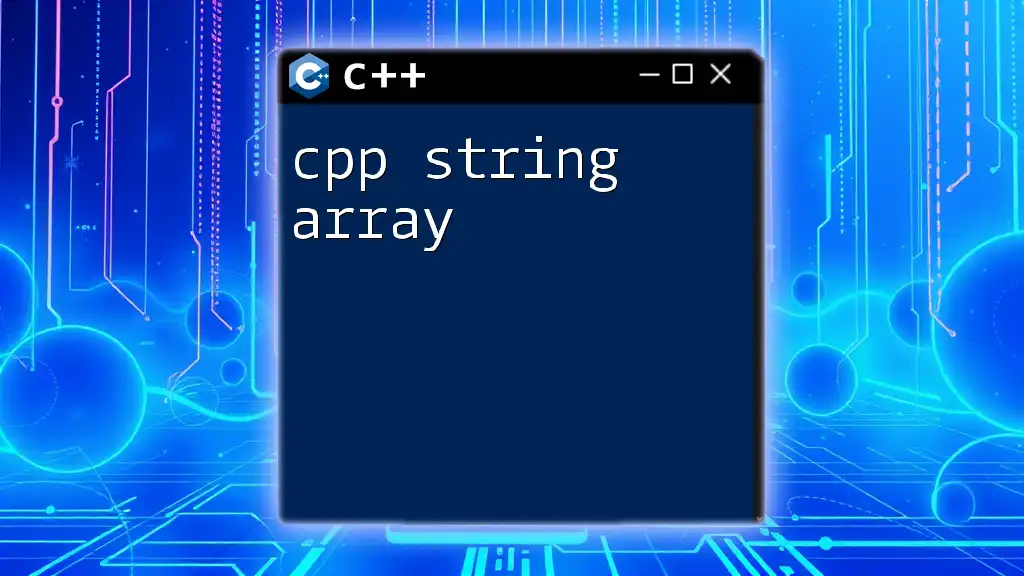
Error Handling in C++ Logging
Best Practices for Error Logging
It’s critical to catch exceptions and log them accordingly to prevent silent failures. For instance:
try {
// code that may throw
} catch (const std::exception &ex) {
logger->error("Exception caught: {}", ex.what());
}
Logging Application State
Logging relative application states can provide insights when troubleshooting issues. Regularly log key states to ease retrospective analysis.
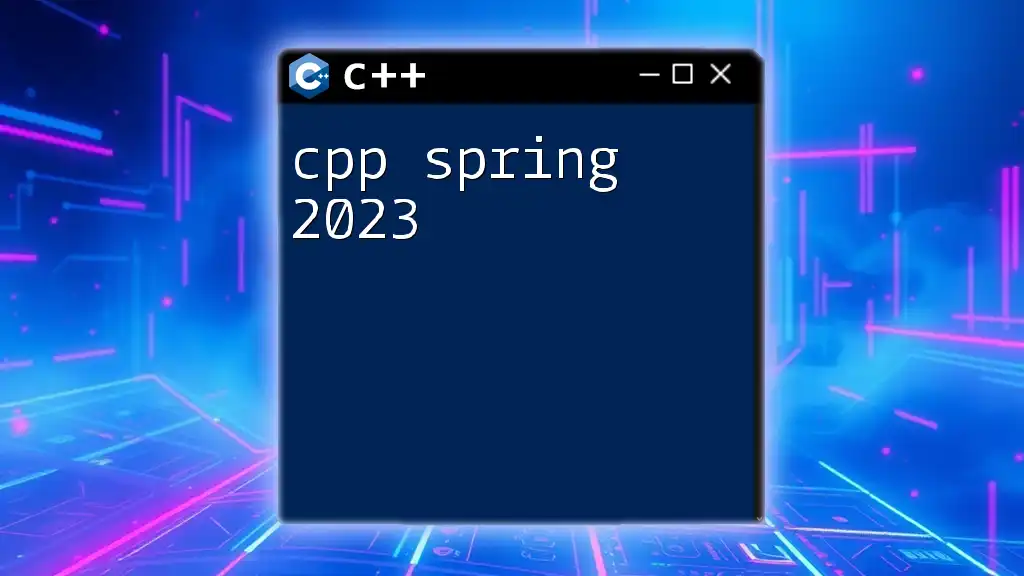
Customizing the C++ Logger
Creating Custom Loggers
If your application has unique logging requirements, creating a custom logger class may be beneficial. Here's an example:
class MyLogger {
public:
MyLogger() {
logger = spdlog::stdout_color_mt("MyLogger");
}
void info(const std::string& message) {
logger->info(message);
}
private:
std::shared_ptr<spdlog::logger> logger;
};
Custom loggers can enhance readability and encapsulate logging functionality.
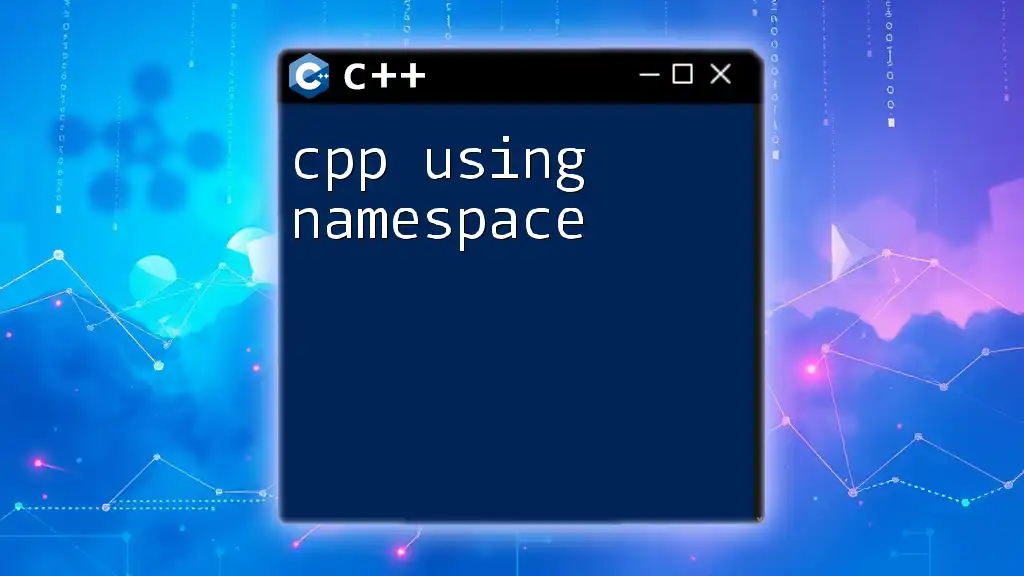
Performance Considerations in C++ Logging
Impact of Logging on Performance
While logging is essential, it can introduce latency if not managed effectively. Logging operations, particularly synchronous, can block your application if performed excessively or incorrectly.
Performance Optimization Tips
- Use asynchronous logging when possible.
- Limit log verbosity in production environments; debug logs should generally be turned off.
- Consider log rotation for files to avoid large log sizes impacting performance.
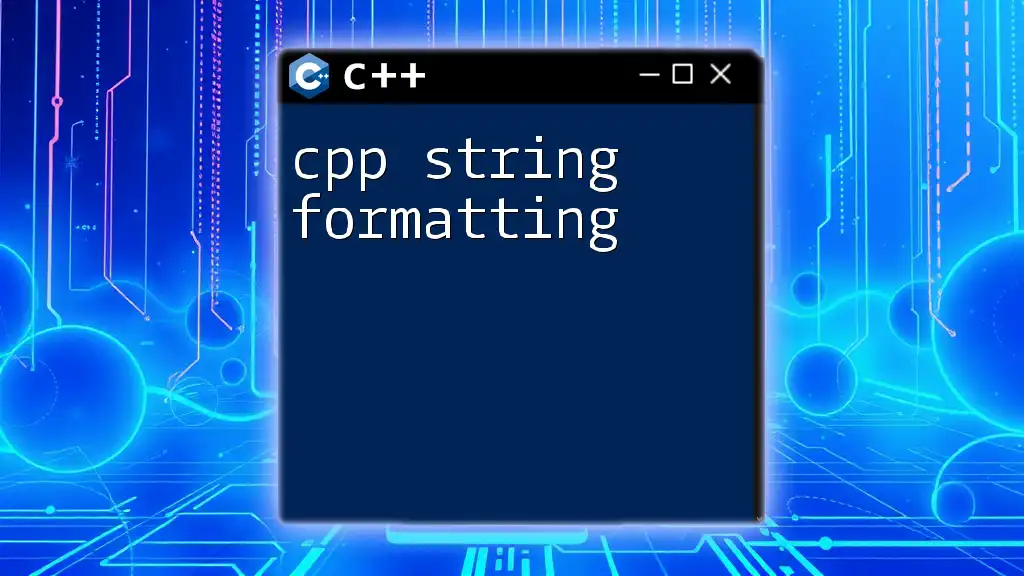
Conclusion
C++ logging is an invaluable practice for developing efficient applications. By implementing a logging library, you can gain detailed insights into your application’s flow and identify potential problems quickly. Always follow best practices to ensure that logging contributes positively to your development workflow.
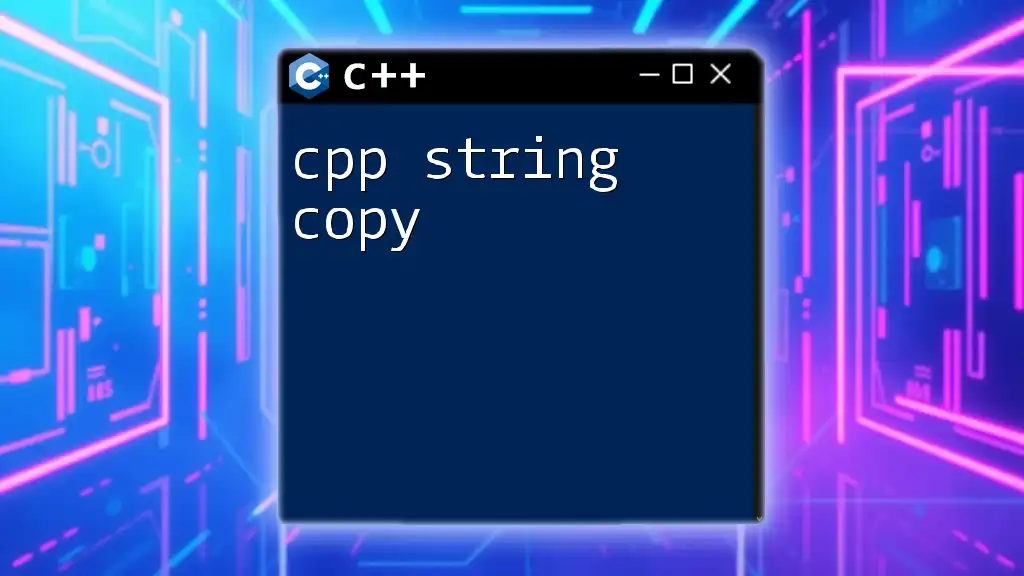
Further Reading and Resources
To deepen your knowledge, explore the documentation of popular logging libraries, such as `spdlog`, and engage with community forums for discussion on best practices and use cases. Embracing logging in your C++ projects will not only enhance your understanding but also significantly improve application reliability.