In 2024, C++ continues to evolve with enhanced features and improvements, making it essential for developers to stay updated on the latest syntax and commands.
Here’s a simple example showcasing how to declare and initialize a variable in C++:
#include <iostream>
int main() {
int number = 2024; // Declaring and initializing an integer variable
std::cout << "The year is " << number << std::endl;
return 0;
}
What’s New in C++ 2024?
Overview of Major Features
C++ 2024 introduces several major advancements primarily designed to streamline the coding experience and enhance the language’s capabilities. It is crucial to understand these features in the context of the historical evolution of C++. There are essential enhancements in the standard library, language features, performance, and more that will help developers write cleaner, more efficient code.
Enhanced Standard Library
The standard library in C++ 2024 has undergone significant upgrades. New libraries and functionalities have been introduced to provide better toolsets for developers. For instance, newer data structures and algorithms can significantly simplify daily programming tasks. Integration of these libraries is straightforward and requires minimal adjustments to existing codebases, allowing developers to seamlessly adopt them into their projects.
Example of Using New Libraries: To leverage new functionalities, simply include the necessary headers and begin using the new tools available:
#include <new_library>
Coroutines Improvements
Coroutines, introduced in C++20, received critical improvements in C++ 2024. These enhancements simplify asynchronous programming, making it more intuitive for developers. The keywords `co_await`, `co_return`, and `co_yield` demonstrate how to handle asynchronous programming more efficiently.
Code Snippet:
#include <iostream>
#include <coroutine>
struct ExampleCoroutine {
struct promise_type {
ExampleCoroutine get_return_object() { return {}; }
auto initial_suspend() { return std::suspend_always(); }
auto final_suspend() noexcept { return std::suspend_always(); }
void unhandled_exception() {}
void return_void() {}
};
};
ExampleCoroutine coroutineFunction() {
// Asynchronous logic
co_return;
}
This illustrates the ease with which you can create and utilize asynchronous functions, thereby improving application responsiveness.
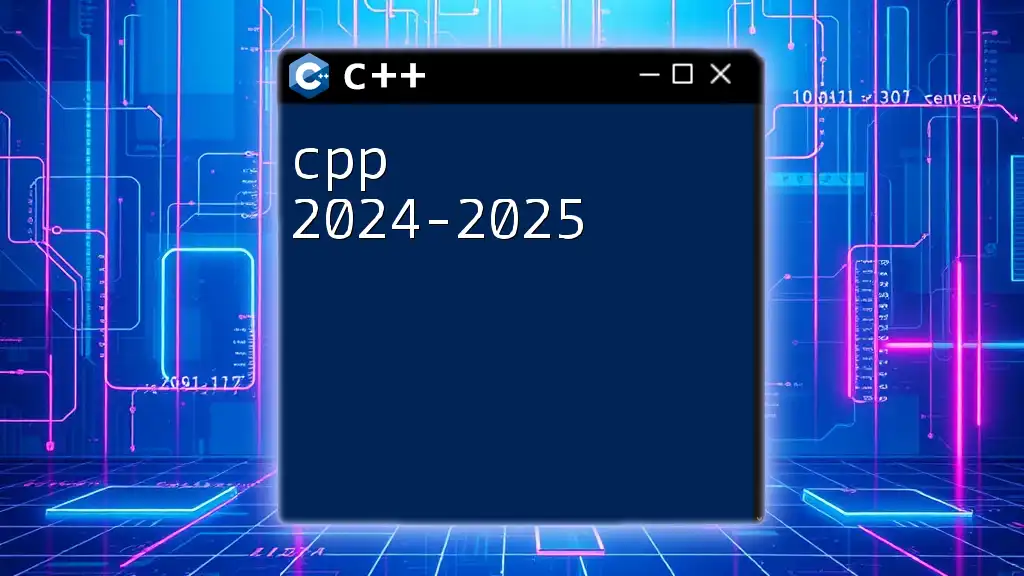
Language Features
Module System Refinements
The module system originally introduced in C++20 has refined capabilities in C++ 2024. This innovation promotes modular programming by enabling faster compilation times and better code organization. Prior to modules, header files often caused inconsistencies and lengthy compile times; C++ 2024 addresses these issues effectively.
Code Snippet:
export module math;
export int add(int a, int b) {
return a + b;
}
Utilizing modules allows you to encapsulate code functions effectively, ensuring better reusability across your codebase.
Pattern Matching
With the evolution of C++ 2024, pattern matching becomes more robust and integral to the language. This feature facilitates clearer and more concise logic, allowing developers to handle conditional operations effectively.
Code Snippet:
switch (value) {
case A: processA(); break;
case B: processB(); break;
default: processDefault(); break;
}
Harnessing pattern matching simplifies control flow in complex scenarios, resulting in more readable and maintainable code.
Enhanced Type Deduction
C++ 2024 dramatically improves type deduction mechanisms, allowing the compiler to infer types more intuitively. This feature accelerates the development process and minimizes human error.
auto myVar = 42; // Type deduced as int
This streamlined approach not only enhances developer experience but also eliminates the need for repetitive type declarations.
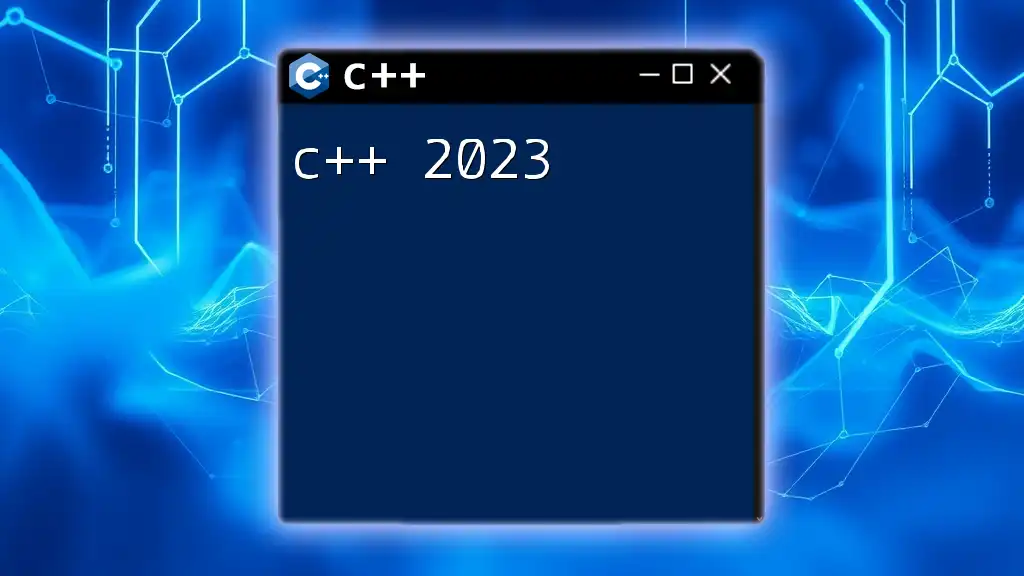
Performance Improvements
Compiler Optimizations
C++ 2024 introduces various compiler optimizations that streamline the performance of your applications. These optimizations focus on both execution time and memory usage, providing noticeable real-world benefits.
Real-world benchmarks indicate that programs compiled using the latest standard exhibit marked improvements in performance metrics, such as reduced load times and memory overhead.
Better Support for Concurrent Programming
As developers increasingly rely on concurrent programming techniques, C++ 2024 provides new tools and libraries designed specifically for this purpose. The updated thread support enhances the ease of managing asynchronous tasks, allowing for better multitasking.
Code Snippet:
#include <thread>
void performTask() {
// Task implementation
}
int main() {
std::thread t1(performTask);
t1.join(); // Wait for the thread to finish
}
This example demonstrates how to utilize threads effectively, making it easier than ever to manage concurrent operations.
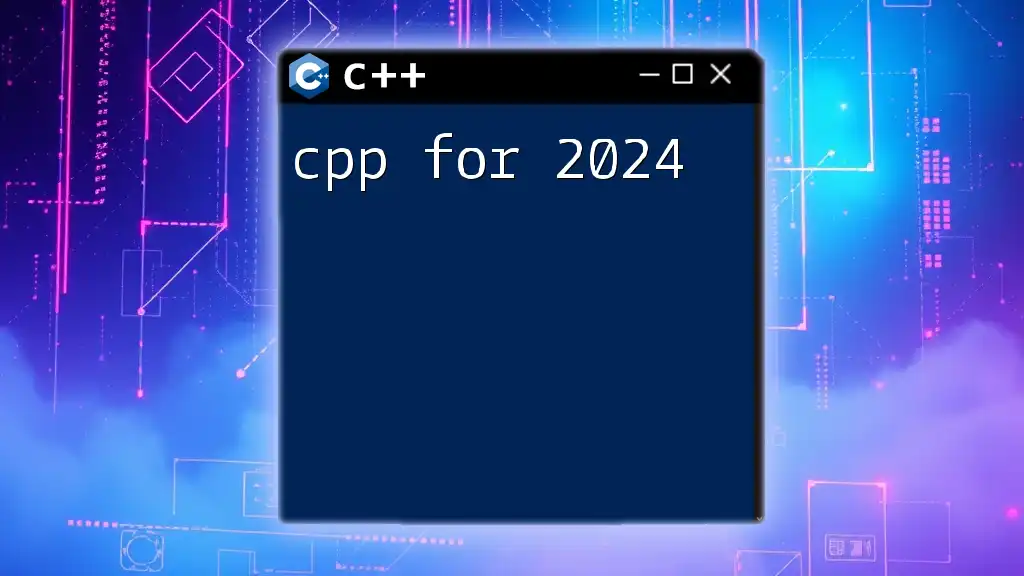
Ecosystem Updates
Modern Development Tools
The ecosystem surrounding C++ 2024 has expanded significantly, with numerous modern Integrated Development Environments (IDEs) and tools being updated to fully support the latest features. IDEs such as Visual Studio, CLion, and Eclipse provide extensive support for exploiting the new capabilities of C++ 2024.
Efficiently integrating these new development tools into your existing systems enhances your workflow, reducing friction for the developers using them.
Community Insights
Community contributions remain vital to the continuous evolution of C++. C++ 2024 benefits from widespread feedback and suggestions from developers worldwide. Open-source collaborations have led to robust libraries and frameworks that enrich the programming ecosystem, ensuring that the language evolves based on user needs and industry demands.
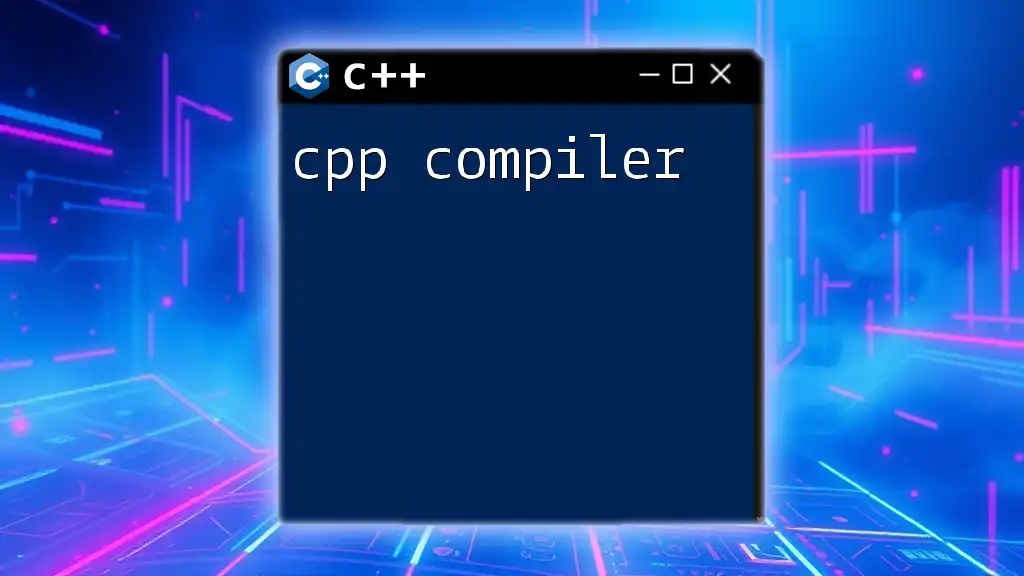
Best Practices for Leveraging C++ 2024
Code Structure and Organization
Adopting an organized code structure is paramount when transitioning to C++ 2024. Properly structuring your program improves readability and allows for better maintenance over time.
Encouraging developers to adhere to style guidelines and structured code practices will result in cleaner code and a more efficient programming environment.
Error Handling Improvements
C++ 2024 enhances error handling capabilities, creating more robust applications. The previous mechanisms for catching and handling exceptions have been refined, promoting a more streamlined approach to managing errors.
Code Snippet:
try {
riskyFunction();
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
The above code illustrates the simplicity and clarity of error handling, allowing developers to manage exceptions without cluttering their code.
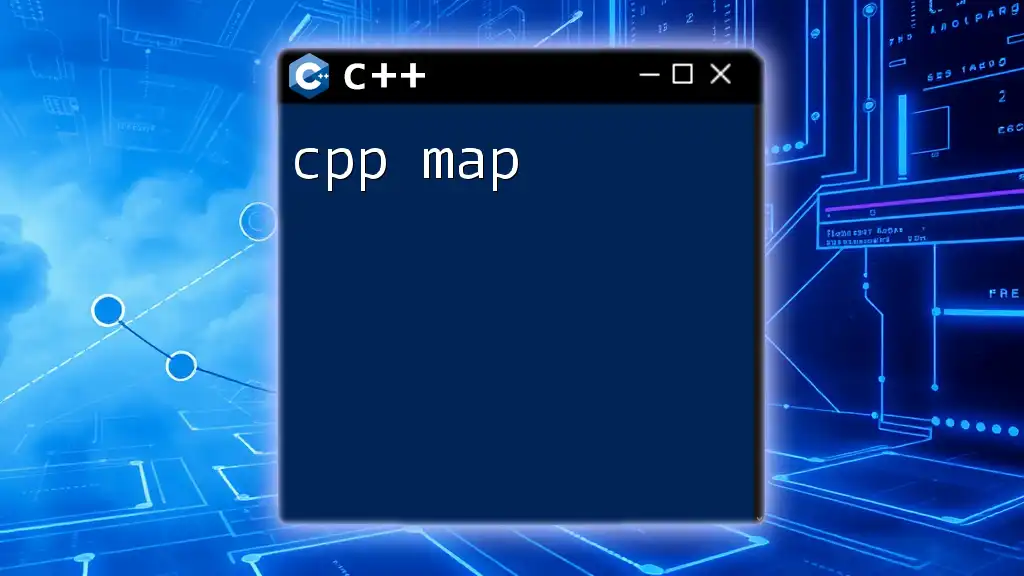
Resources for Further Learning
Books and Online Courses
To further your understanding of C++ 2024, consider exploring recommended texts that dive into advanced topics and new features. Online platforms like Coursera, Udemy, and Codecademy offer comprehensive courses tailored for C++ 2024, granting practical insights and hands-on experience.
Code Repositories and Practice Environments
Engagement with various code repositories that showcase examples and applications of C++ 2024 will significantly enhance your learning experience. Utilizing platforms like GitHub for practice and experimentation can help solidify your understanding of the new features introduced in this latest version.
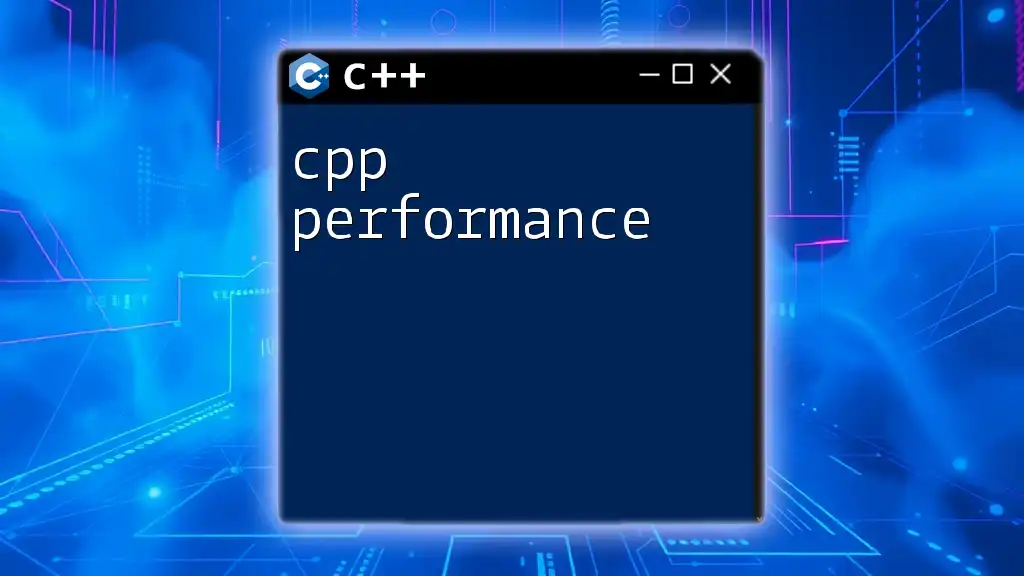
Conclusion
C++ 2024 marks a significant milestone in the language's evolution, introducing a host of new features and tools designed to streamline development and improve performance. By understanding and leveraging these advancements, developers can enhance their productivity and create more sophisticated applications. The future of C++ looks promising, and embracing these changes will position developers for success.
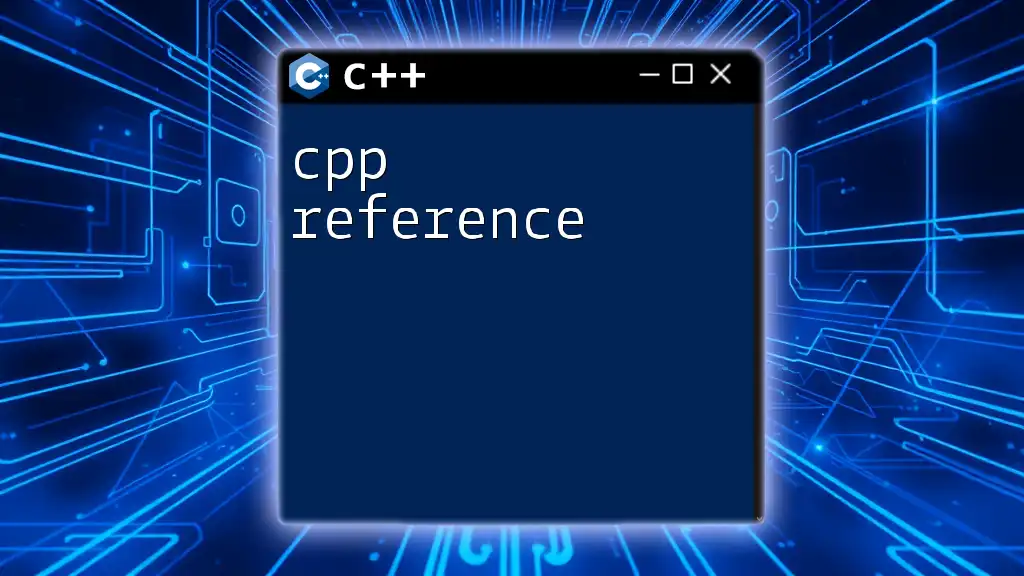
FAQs
Many common questions arise regarding C++ 2024, particularly concerning how to transition from older versions and adapt existing code. Frequently asked questions may involve clarifications on specific features, the implementation of new best practices, and the most efficient paths to learning about C++ 2024 features. Be sure to engage with the community for insights and best practices as you delve into the new developments of C++.