The `-o` option in the C++ compiler command allows you to specify the name of the output file that will be generated after compilation.
g++ -o my_program my_source_file.cpp
Understanding the C Preprocessor
What is the C Preprocessor?
The C preprocessor acts as a crucial component of C/C++ programming, functioning to prepare your source code before it undergoes compilation. It handles preprocessing directives, which are commands that can modify the code or include files. Understanding the preprocessor's role helps programmers streamline their code and enhance its efficiency.
How the Preprocessor Works
Before any C or C++ code is compiled, the preprocessor takes charge. It processes directives and comments, expanding macros, including header files, and defining symbols as specified. Common directives include:
- `#include`: For including external files or libraries
- `#define`: For defining macros
This processing phase allows for cleaner code and better organization, as developers can break their code into manageable chunks through headers and modular components.
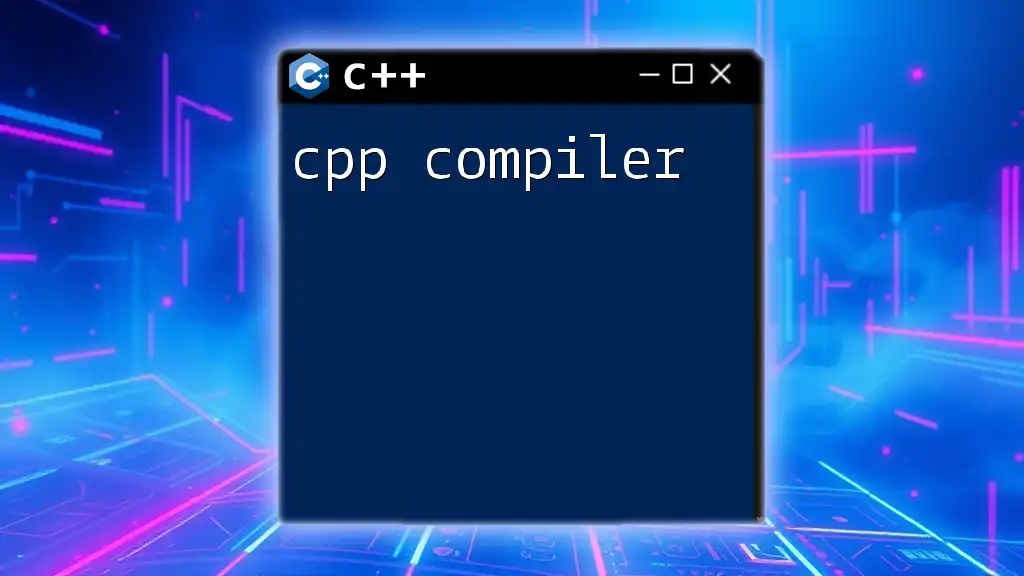
Introduction to the `-o` Option
What Does `-o` Stand For?
The `-o` option is a command-line flag commonly used with the `cpp` command, where it stands for output. This option lets users specify the name of the file that will store the preprocessed output.
Purpose of the `-o` Option
Specifying an output file using `-o` is crucial for effective management of your code files. Without the `-o` option, the preprocessor would output a file with a default name, making it challenging to keep track of various versions or processed files. By using `-o`, developers can maintain a clear organization of their work, which is particularly beneficial in larger projects or collaborative environments.
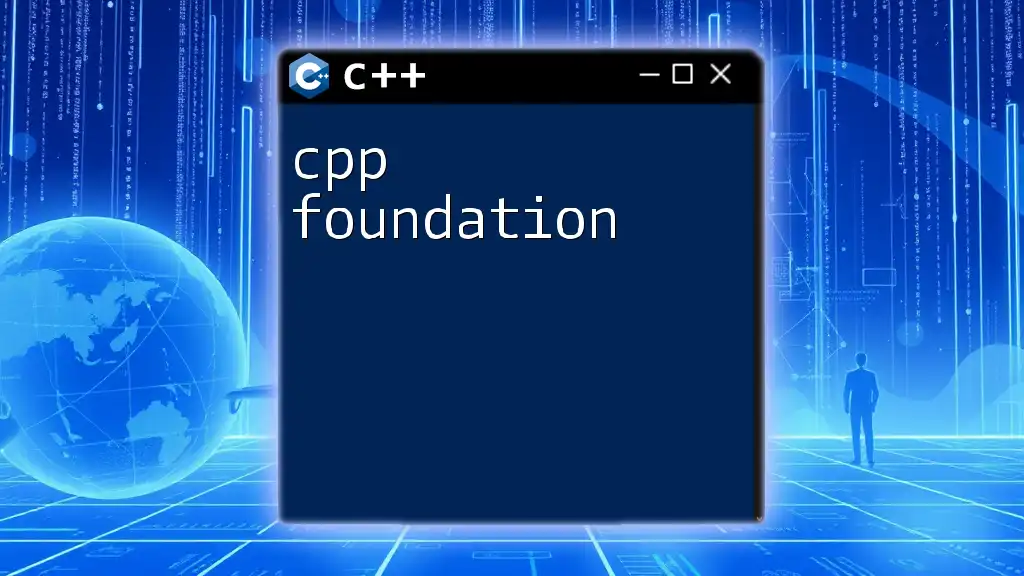
Usage of `cpp -o`
Basic Syntax of the `cpp` Command
The basic syntax for using the `cpp` command with the `-o` option is structured as follows:
cpp [options] [input file] [output file]
Example of `cpp -o` Usage
To clarify its application, consider the following command:
cpp -o output_file.cpp input_file.cpp
In this example:
- `output_file.cpp` is the name you want to assign to the preprocessed output.
- `input_file.cpp` is the original C/C++ source code that needs preprocessing.
Verifying Output
After executing the `cpp -o` command, it's essential to check that the output file has been created successfully. You can do this by running the following command:
cat output_file.cpp
If the command is executed without errors, you will see the preprocessed content displayed in the terminal. This confirms that the `cpp -o` command has performed its job correctly.
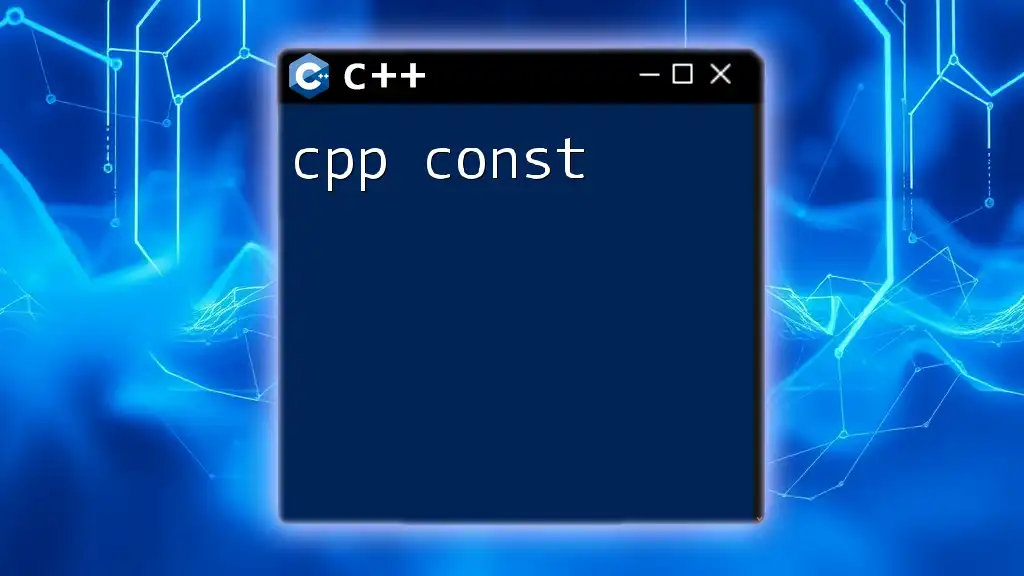
Practical Examples
Example 1: Basic Usage
Imagine you have a simple C program saved as `input_file.c`:
// input_file.c
#include <stdio.h>
#define GREETING "Hello, World!"
int main() {
printf("%s\n", GREETING);
return 0;
}
You can preprocess this file using the following command:
cpp -o preprocessed_file.c input_file.c
Expected Output: After running this command, `preprocessed_file.c` will contain a version of the original code, with the `#include` directive processed and the macro `GREETING` expanded to "Hello, World!". This file would look similar to the following:
// preprocessed_file.c
int main() {
printf("Hello, World!\n");
return 0;
}
Example 2: Complex Code
Now consider a more complex scenario with macros and standard libraries. You have `input_file.c` as follows:
// input_file.c
#include <math.h>
#define PI 3.14
int main() {
double radius = 5;
double area = PI * radius * radius;
return 0;
}
Using the `cpp -o` command to preprocess this file looks like this:
cpp -o preprocessed_file.c input_file.c
Expected Output: The `preprocessed_file.c` would show the entire code, where the definition of `PI` has been processed, and the standard library `math.h` included, further clarifying how macros are expanded.
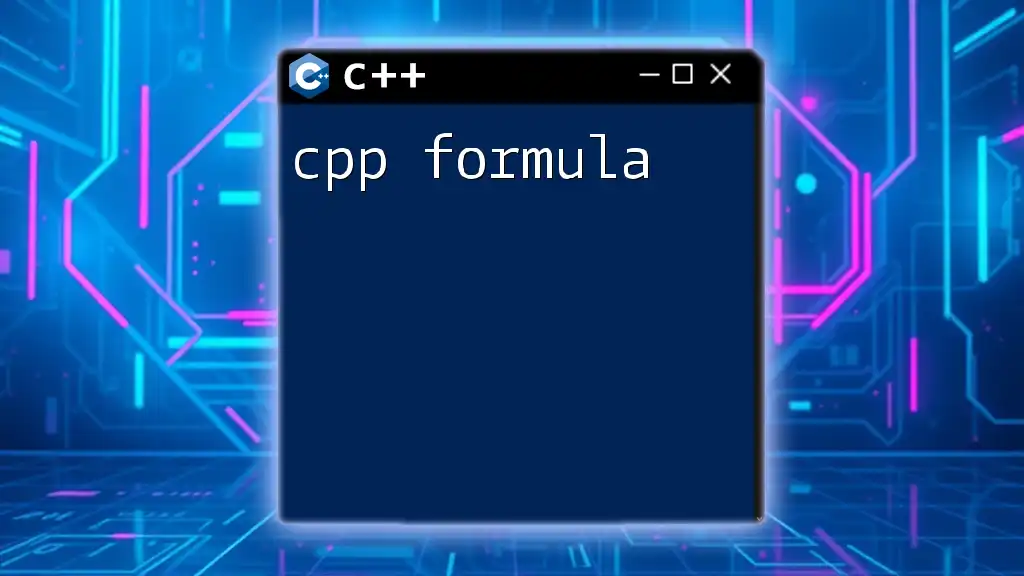
Common Errors and Troubleshooting
Missing Input File Error
A common issue arises when the specified input file does not exist. If you run the command with an incorrect file name, you will see an error message indicating the file could not be found. To solve this, double-check the input file name for accuracy and ensure that it is located in the directory from which you're executing the command.
Invalid Output File Name
While the `-o` option allows for custom naming of output files, certain restrictions apply. For instance, special characters or spaces in file names can lead to errors. Adopting best practices for naming—using alphanumeric characters, underscores, and avoiding spaces—can help in avoiding these issues.
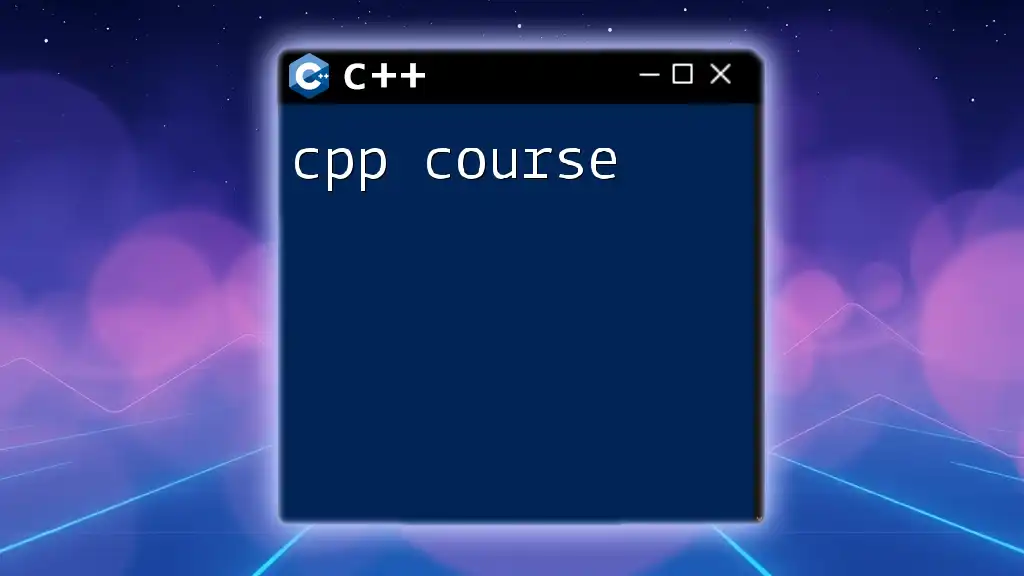
Conclusion
Recap of `cpp -o`
In summary, the `cpp -o` command plays a vital role in the effective preprocessing of C/C++ source code. By understanding its functionality and employing it correctly, developers can enhance their coding efficiency and maintain better organization within their projects.
Final Tips for Using `cpp`
As you continue to explore the capabilities of the `cpp` command, don't hesitate to experiment with different flags and options. Familiarity will foster skill and expertise in preprocessing your C/C++ code.
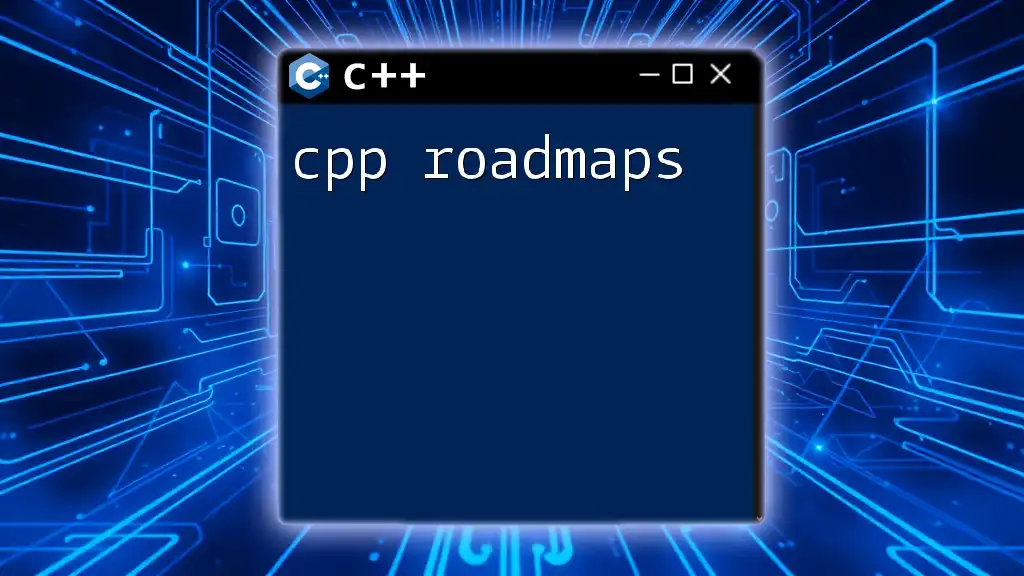
Additional Resources
For further learning, refer to official C and C++ documentation, as well as recommended books and online courses that delve deeper into the intricacies of the C preprocessor. These resources will help reinforce your understanding and broaden your programming skill set.