The "cpp version" refers to the specific version of the C++ programming language being used, which can affect available features, functionality, and syntax.
Here’s an example of how to check the currently used C++ version in code:
#include <iostream>
int main() {
#if __cplusplus == 201703L
std::cout << "C++17 is being used." << std::endl;
#elif __cplusplus == 201402L
std::cout << "C++14 is being used." << std::endl;
#elif __cplusplus == 201103L
std::cout << "C++11 is being used." << std::endl;
#else
std::cout << "An older version of C++ is being used." << std::endl;
#endif
return 0;
}
Understanding C++ Versions
Evolution of C++
C++ has undergone a significant evolution since its inception in the early 1980s. Originally designed by Bjarne Stroustrup as an enhancement to the C programming language, C++ introduced concepts that allowed for better abstraction and more complex software design. Each revision brought forth new features to enhance the language's capability and support for modern programming paradigms.
The major iterations of the C++ standard include:
- C++98: The first standardized version.
- C++03: A maintenance release focusing on bug fixes.
- C++11: A landmark revision introducing several modern programming features.
- C++14: An incremental update that added more refinements.
- C++17: Brought robust enhancements and utilities.
- C++20: Introduced major features aimed at simplifying and improving the efficiency of coding practices.
- C++23: Upcoming features that aim to further modernize the language.
The role of the ISO C++ Committee is fundamental, as it oversees the standardization process and works on proposals from the community to improve the language continuously.
Why C++ Versions Matter
Understanding the nuances of different C++ versions is crucial for developers. Each version introduces new features, optimizations, and best practices that can significantly impact code quality, maintainability, and performance.
Moreover, these differences can affect backward compatibility, meaning that code written in older versions may not compile or function correctly in newer environments without modifications. Keeping an eye on compiler support is equally essential, as not all compilers fully implement every feature from newer standards immediately.
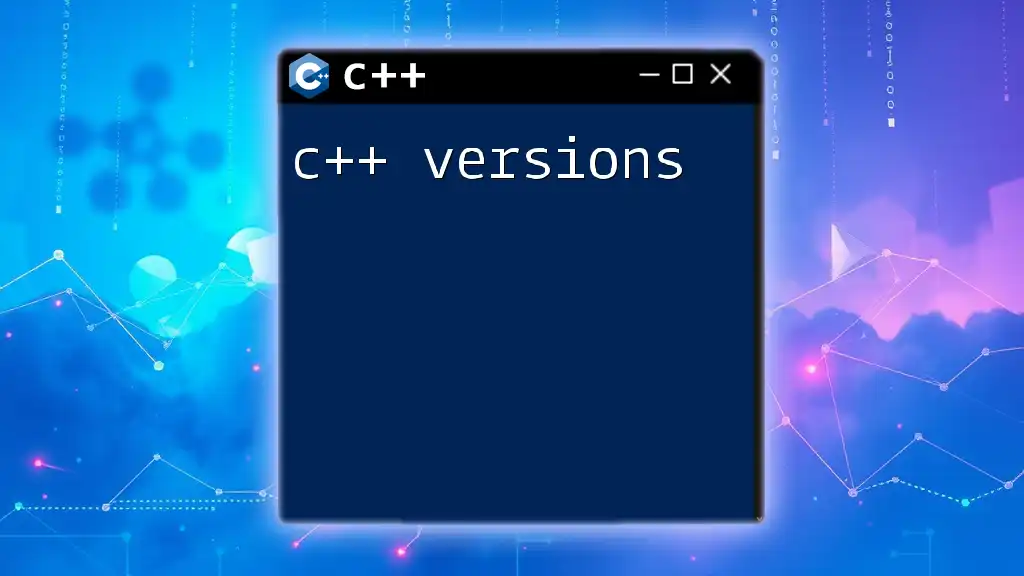
Major C++ Versions Overview
C++98: The First Standard
C++98 was the first version to establish formal standards for C++. This version introduced essential features like templates, which allow for generic programming, and the Standard Template Library (STL), which provides a framework for creating and using data structures and algorithms.
Example: Using Templates
#include <iostream>
using namespace std;
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
cout << "Sum: " << add<int>(5, 10) << endl; // Outputs: Sum: 15
return 0;
}
C++03: Minor Updates
C++03 served primarily as a bug-fix release for C++98, addressing issues without introducing significant new features. This version ensured that existing codebases could be maintained more reliably and consistently.
C++11: The Game Changer
C++11 revolutionized the language by introducing numerous impactful features that modernized programming in C++. Among its significant enhancements are:
- Auto Keyword and Type Inference: Simplifies variable declarations.
- Lambda Expressions: Enables inline function definitions.
- Smart Pointers: Reduces memory leakage with automatic memory management.
- Move Semantics: Optimizes resource transfer on object copying.
Example: Using Lambda Expressions
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(numbers.begin(), numbers.end(), [](int n) {
std::cout << n << " ";
});
return 0;
}
C++14: The Incremental Update
Building upon C++11, C++14 introduced various minor improvements for enhanced usability, such as:
- Generic Lambdas: Allow lambda expressions to accept any parameter type.
- Return Type Deduction: Automatic inference of function return types.
Example: Generic Lambdas
#include <iostream>
#include <vector>
int main() {
auto print = [](auto container) {
for (const auto& item : container) {
std::cout << item << " ";
}
};
std::vector<int> vec = {1, 2, 3, 4, 5};
print(vec);
return 0;
}
C++17: More Enhancements
C++17 added several new functionalities, enriching its utility and expressiveness. Key features include:
- Structured Bindings: A convenient way to unpack tuples and pairs.
- Optional and Variant Types: New types for better handling of default values and data types.
- Parallel Algorithms: Harnesses multi-threading capabilities.
Example: Using Structured Bindings
#include <iostream>
#include <tuple>
int main() {
std::tuple<int, char> t(1, 'A');
auto [i, c] = t; // Structured binding
std::cout << "Integer: " << i << ", Char: " << c << std::endl;
return 0;
}
C++20: The Latest Resolution
C++20 represents a significant leap forward, introducing groundbreaking features that facilitate modern programming practices:
- Concepts: Predicates that simplify generic programming.
- Ranges: A new way to work with collections.
- Coroutines: Support for writing asynchronous code more intuitively.
- Modules: Help organize and encapsulate code better.
Example: Using Concepts
#include <iostream>
#include <concepts>
template <typename T>
concept Integral = std::is_integral<T>::value;
template <Integral T>
T add(T a, T b) {
return a + b;
}
int main() {
std::cout << "Result: " << add(5, 10) << std::endl; // Outputs: Result: 15
return 0;
}
Upcoming Features in C++23
As C++23 approaches, the community is looking forward to enhancements that will continue to evolve the language. While formal specifications are still being discussed, some anticipated features include:
- More powerful constexpr capabilities.
- New library components for better software engineering practices.
- Enhanced pattern matching functionalities.
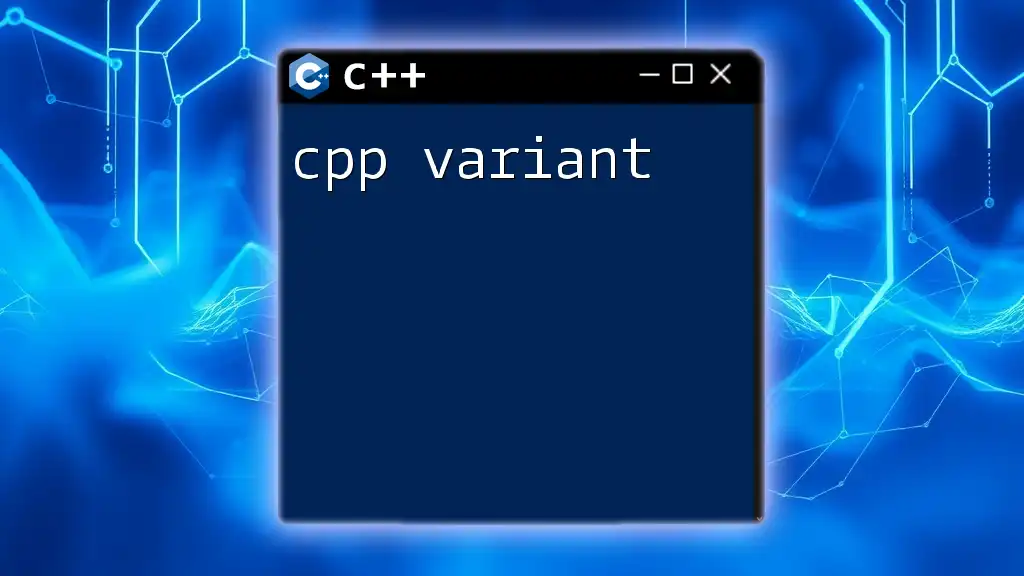
How to Check Your C++ Version
Common Methods
You can easily check your C++ version using the command line. For instance, if you are using g++, you can compile a simple program that includes version-checking macros.
Example: Check Version via g++
#include <iostream>
int main() {
std::cout << __cplusplus << std::endl; // This will print the version
return 0;
}
Compiler Compatibility
Not all compilers support all the features introduced in the latest C++ standards. Therefore, it is imperative to check the compatibility of your compiler and verify which version of the language it supports:
- g++: Generally, it has good support for most C++ standards, especially recent ones.
- MSVC: Microsoft’s compiler has been catching up with the standards, but discrepancies may remain.
- Clang: Known for its excellent support and compliance with the latest standards.
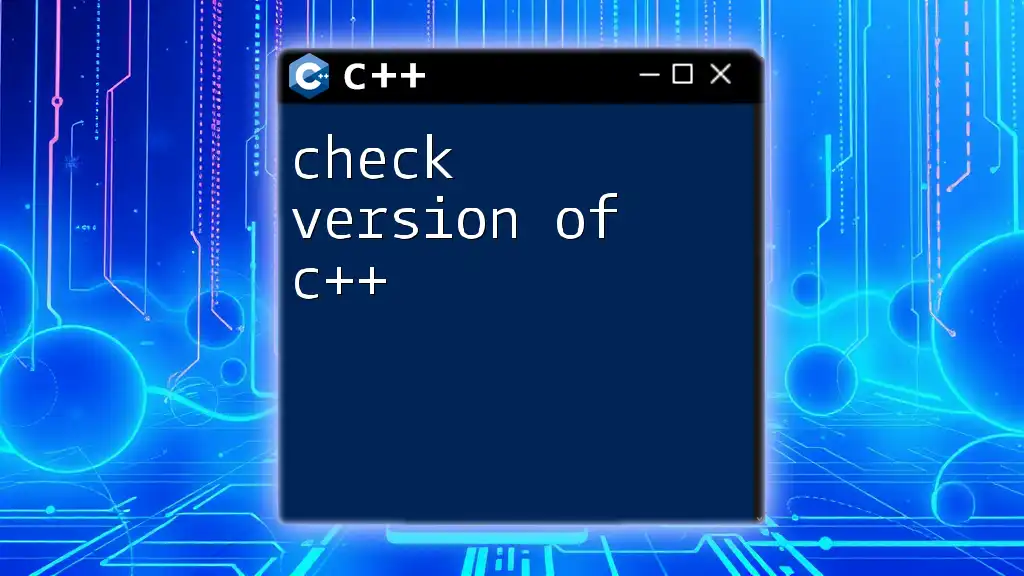
Conclusion
Understanding the different C++ versions is integral to progressing as a developer. Each iteration brings enhancements that can significantly alter how we write and manage our code. As newer standards emerge, staying informed and adaptive will not only improve your coding practices but also keep you aligned with industry best practices.
Frequently Asked Questions (FAQ)
What are the main differences between C++11 and C++14? The primary difference is that C++14 built upon C++11 by streamlining certain features and introducing minor enhancements. For example, generic lambdas and return type deduction were added.
How do I migrate an older C++ codebase to a newer standard? Start by compiling your code with the new standard flag, address any compiler errors or warnings, and leverage modern features progressively without overwhelming changes.
Is it necessary to use the latest C++ version? While it's not mandatory to use the latest version, leveraging new features can enhance code quality, performance, and maintainability. It's advisable to adopt suitable features that align with the project's needs.
What resources are available for learning modern C++? Look into books like "C++ Primer" by Lippman et al. or "Effective Modern C++" by Scott Meyers, as well as online courses and forums dedicated to C++ programming.