`<cstdlib>` is a header file in C++ that provides functions for performing general utility operations such as memory allocation, random number generation, and process control.
Here’s a simple example demonstrating the use of the `rand()` function from `<cstdlib>` to generate a random number:
#include <iostream>
#include <cstdlib> // Include the cstdlib header for rand and srand
#include <ctime> // Include ctime for the time function
int main() {
std::srand(static_cast<unsigned int>(std::time(0))); // Seed the random number generator
int randomNumber = std::rand(); // Generate a random number
std::cout << "Random Number: " << randomNumber << std::endl; // Output the random number
return 0;
}
What is cstdlib?
The `cstdlib` header file is part of the C++ Standard Library and provides functions for performing general utilities, including memory management, conversions, and process control. It serves as a bridge between C and C++ programming, making it easy to utilize C-style functions within C++ code. Understanding `cstdlib` is crucial for developers who want to effectively manage resources and perform operations that rely on standard library functions.
Importance of cstdlib in C++
Including the `cstdlib` library is important because it expands a programmer's toolkit, offering a variety of useful functions that streamline coding tasks. Utilizing these standard functions can help in:
- Memory Management: Efficient handling of dynamic memory allocation.
- Data Conversion: Conversion of data types, especially when interfacing with C-style strings.
- Random Number Generation: Easily generating random numbers for various applications.
- Error Handling: Gracefully managing errors and exiting programs when necessary.
Core Functions of cstdlib
Memory Management Functions
One of the most critical areas where `cstdlib` shines is in memory management, allowing for dynamic allocation and deallocation of memory. The primary functions used are `malloc()` and `free()`.
- malloc(): Allocates uninitialized memory block.
- free(): Deallocates memory that was previously allocated with `malloc()`.
Here’s an example demonstrating memory allocation and deallocation:
#include <cstdlib>
#include <iostream>
int main() {
// Allocate memory for an array of integers
int* arr = (int*)malloc(5 * sizeof(int));
if (arr) {
for (int i = 0; i < 5; ++i) {
arr[i] = i; // Initialize array elements
std::cout << "Element " << i << ": " << arr[i] << std::endl;
}
// Deallocate memory
free(arr);
}
return 0;
}
In this example, we allocate memory for an array of integers using `malloc()`. It's crucial to check if the allocation was successful before utilizing the memory. After we’re done, we use `free()` to prevent memory leaks.
Conversion Functions
`cstdlib` provides several conversion functions such as `atoi()`, `atof()`, and `strtol()`. These functions are essential when dealing with C-style strings, allowing you to convert them into numerical data types.
- atoi(): Converts a C-string to an integer.
- atof(): Converts a C-string to a floating-point number.
- strtol(): Converts a C-string to a long integer with error checking.
Here’s a simple example using `atoi()`:
#include <cstdlib>
#include <iostream>
int main() {
const char* str = "1234";
int num = atoi(str);
std::cout << "The integer is: " << num << std::endl;
return 0;
}
This code snippet illustrates the usage of `atoi()`, where we convert a string representing a number into an integer. Such conversions are crucial when handling user input or parsing data from text files.
Random Number Generation
Generating random numbers is often required in various applications, from simulations to randomized algorithms. `cstdlib` provides two essential functions:
- rand(): Generates a pseudo-random integer.
- srand(): Seeds the random number generator, ensuring different sequences of random numbers on each run.
Here’s an example demonstrating these functions:
#include <cstdlib>
#include <iostream>
#include <ctime>
int main() {
// Seed the random number generator
srand((unsigned)time(0));
// Generate and print a random number
std::cout << "Random Number: " << rand() << std::endl;
return 0;
}
In this code, we first seed the generator with the current time to ensure a different starting point for random numbers each time the program is executed. The `rand()` function then provides a random integer.
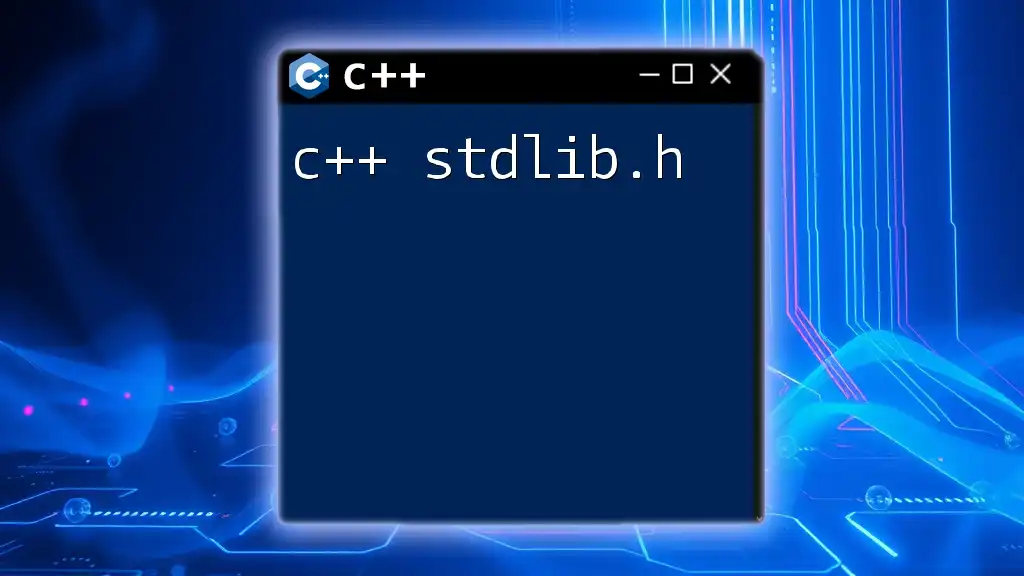
Error Handling in C++
Exiting the Program
When a program encounters a critical issue or needs to terminate, `cstdlib` offers `exit()` and `abort()` as methods to manage program exits gracefully.
- exit(): Terminates the program and returns a status code.
- abort(): Causes abnormal termination and does not unwind the stack.
Consider the following usage of `exit()`:
#include <cstdlib>
#include <iostream>
int main() {
std::cout << "Exiting the program gracefully." << std::endl;
exit(0); // Successful termination
// Code after exit() will not execute
std::cout << "This line will never be executed." << std::endl;
return 0;
}
Using `exit(0)` signifies a successful exit from the program. In contrast, `abort()` is more abrupt and should be used in scenarios where the program faces an unrecoverable error.
Dealing with Errors
When working with external resources like files, managing errors efficiently is paramount. The functions `perror()` and `strerror()` found in `cstdlib` help retrieve meaningful error messages.
Here’s how you might use `perror()` for error handling:
#include <cstdlib>
#include <iostream>
#include <cstdio>
int main() {
// Attempt to open a non-existent file
FILE* file = fopen("nonexistent.txt", "r");
if (!file) {
perror("Error opening file"); // Print the error message
}
return 0;
}
In this instance, if the file cannot be opened, `perror()` prints a descriptive error message, simplifying debugging and ensuring smooth error management.
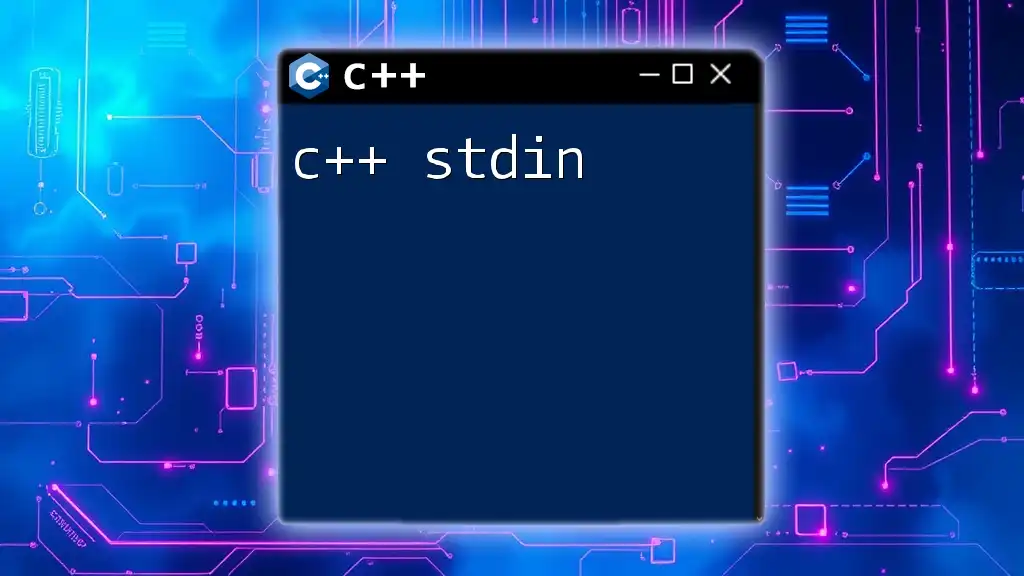
Performance Considerations
When incorporating functions from `cstdlib`, it is important to consider their impact on performance. Standard library functions are often optimized and offer a level of reliability. However, excessive use of dynamic allocation can lead to fragmentation and performance overhead.
-
Efficient Function Selection: Choose the correct functions based on your use case. For example, prefer using `std::vector` for dynamic arrays in C++ as it manages memory more efficiently than raw pointers.
-
Optimize for Reuse: Reuse allocated memory instead of frequently allocating and deallocating to reduce overhead.
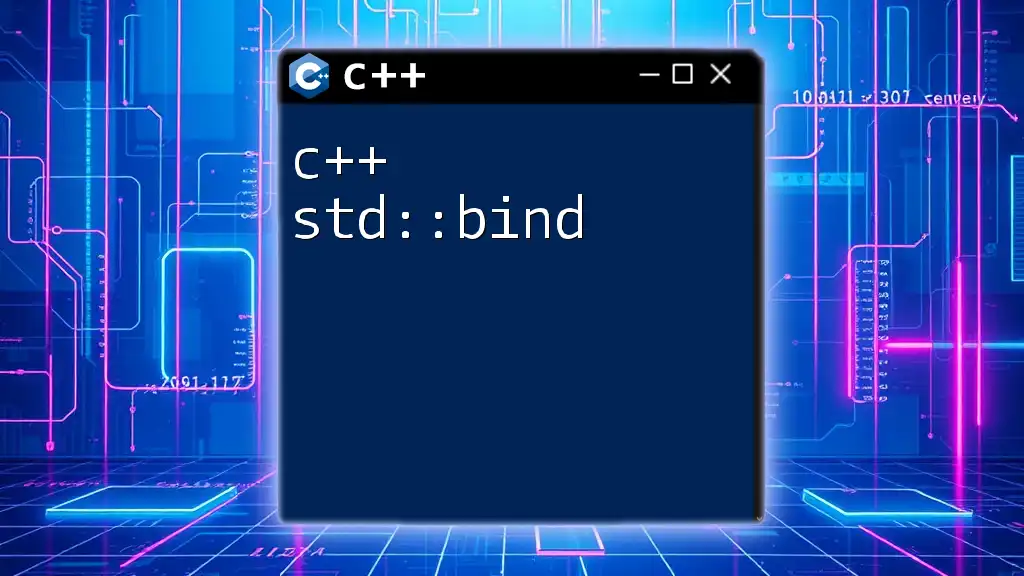
Best Practices
When to Use cstdlib in C++
- Use `cstdlib` for C Compatibility: When developing applications where C functions are needed, always depend on `cstdlib` for managing memory and using conversions.
- Avoid Memory Leaks: Always pair `malloc()` with `free()`, and consider modern C++ practices for resource management.
Staying Up-to-Date
To ensure your skills remain relevant, keep abreast of any changes and enhancements to the `cstdlib` library or the broader C++ standard. Explore online resources such as tutorials, documentation, and community forums to deepen your understanding and capabilities.
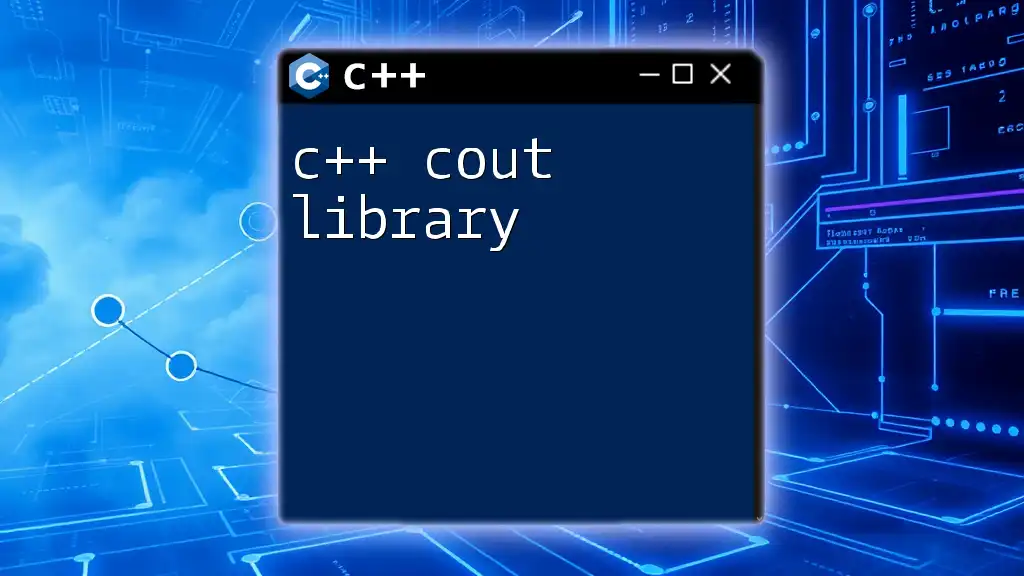
Conclusion
Understanding `c++ cstdlib` allows developers to leverage a wide array of useful functions that enhance programming efficiency. From memory management to data conversion and error handling, `cstdlib` serves as a vital component in any C++ programmer's toolkit. It’s recommended to start incorporating these tools in your coding practices to unlock greater potential in your programming endeavors.