The `isdigit` function in C++ checks whether a given character is a decimal digit (0-9), returning a non-zero value if true and 0 if false.
#include <iostream>
#include <cctype>
int main() {
char ch = '5';
if (isdigit(ch)) {
std::cout << ch << " is a digit." << std::endl;
} else {
std::cout << ch << " is not a digit." << std::endl;
}
return 0;
}
Understanding `isdigit`
What is `isdigit`?
The `isdigit` function is a built-in C++ function designed to determine if a given character represents a decimal digit (i.e., one of the characters '0' through '9'). This functionality is essential for validating input and ensuring that characters processed in your program fall within expected ranges.
Where is `isdigit` Found?
To use the `isdigit` function, you must include the `<cctype>` header. This standard library header file provides facilities for character classification and conversion, ensuring you can handle character data effectively. Unlike C, where you might also encounter `<ctype.h>`, C++ prefers the former for its type safety and namespace management.
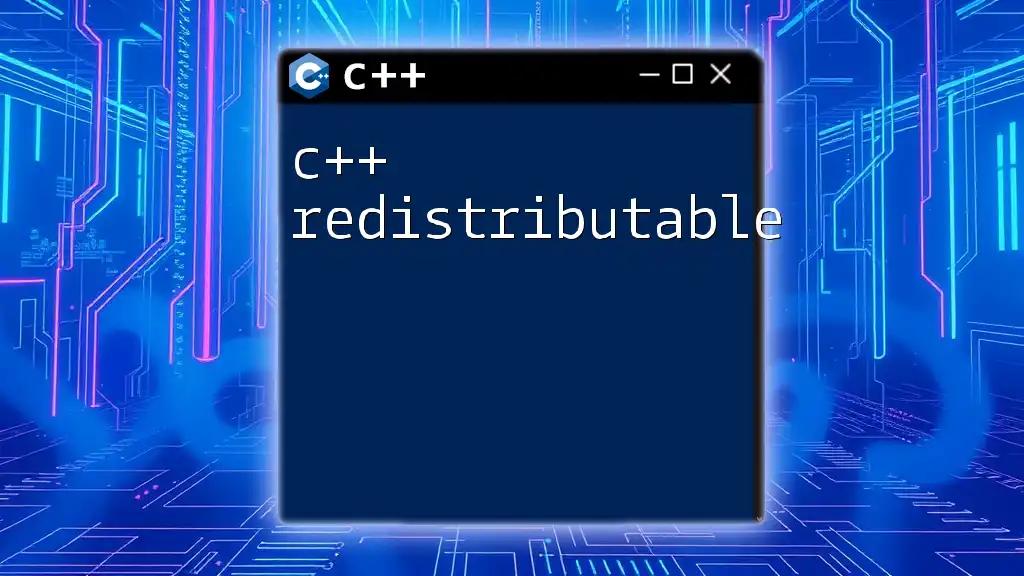
Syntax of `isdigit`
Basic Syntax
The basic syntax of the `isdigit` function can be outlined as follows:
int isdigit(int ch);
This signature indicates that the function takes an `int` as an argument, which typically represents a character, and returns an integer.
Parameters and Return Value
The `isdigit` function accepts a single parameter, `ch`, which represents the character that you want to check. The return value is also of type `int`. Here’s how it works:
- Returns a non-zero value (true) if the input character is a digit.
- Returns 0 (false) if the input character is not a digit.
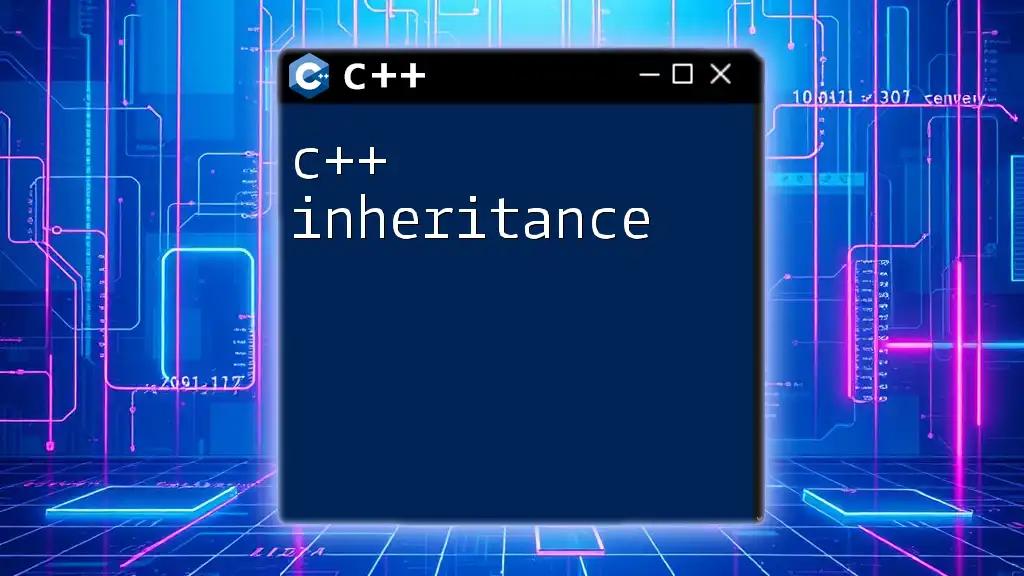
How to Use `isdigit`
Basic Example
To demonstrate how the `isdigit` function works, consider the following straightforward example:
#include <iostream>
#include <cctype>
int main() {
char ch = '5';
if (isdigit(ch)) {
std::cout << ch << " is a digit." << std::endl;
} else {
std::cout << ch << " is not a digit." << std::endl;
}
return 0;
}
In this code, we check if the character '5' is a digit. If it is, we print a confirmation; otherwise, we indicate that it's not. This example showcases the basic functionality of `isdigit`.
Checking Multiple Characters
You often need to validate strings or multiple characters in practice. Here’s how you can loop through a string to check each character:
#include <iostream>
#include <cctype>
int main() {
std::string input = "C0d3;!5";
for (char ch : input) {
if (isdigit(ch)) {
std::cout << ch << " is a digit." << std::endl;
}
}
return 0;
}
In this example, we traverse through the string "C0d3;!5" character by character, validating which characters are digits and printing them out. This method is invaluable when dealing with user inputs or data which may contain mixed characters.
User Input Validation
Validating User Input with `isdigit`
A common application of `isdigit` is to validate user input to ensure it consists of digits only. Here’s an example that demonstrates this concept:
#include <iostream>
#include <cctype>
#include <string>
int main() {
std::string userInput;
std::cout << "Enter a number: ";
std::getline(std::cin, userInput);
bool isValid = true;
for (char ch : userInput) {
if (!isdigit(ch)) {
isValid = false;
break;
}
}
if (isValid) {
std::cout << "Valid number." << std::endl;
} else {
std::cout << "Invalid input. Please enter digits only." << std::endl;
}
return 0;
}
Here, we prompt the user to enter a number and check each character using `isdigit`. If we encounter any non-digit characters, we notify the user of invalid input. This practice greatly enhances the robustness of your applications, ensuring that only valid numeric input is processed.

Common Mistakes and Troubleshooting
Using Non-Character Types
One common mistake when using `isdigit` is attempting to pass non-character types as arguments. The `isdigit` function expects an `int`, typically representing a character code. For example, using `isdigit(10)` would not produce a meaningful result since 10 does not correspond to a digit character. Always ensure that you are passing a valid character or convert it appropriately.
Misunderstanding Return Values
Another frequent misunderstanding arises from the nature of the return value. Remember that `isdigit` returns a non-zero value for true (indicating a digit) and returns 0 for false. Misinterpretation of these values can lead to erroneous program behavior, especially in conditional statements.
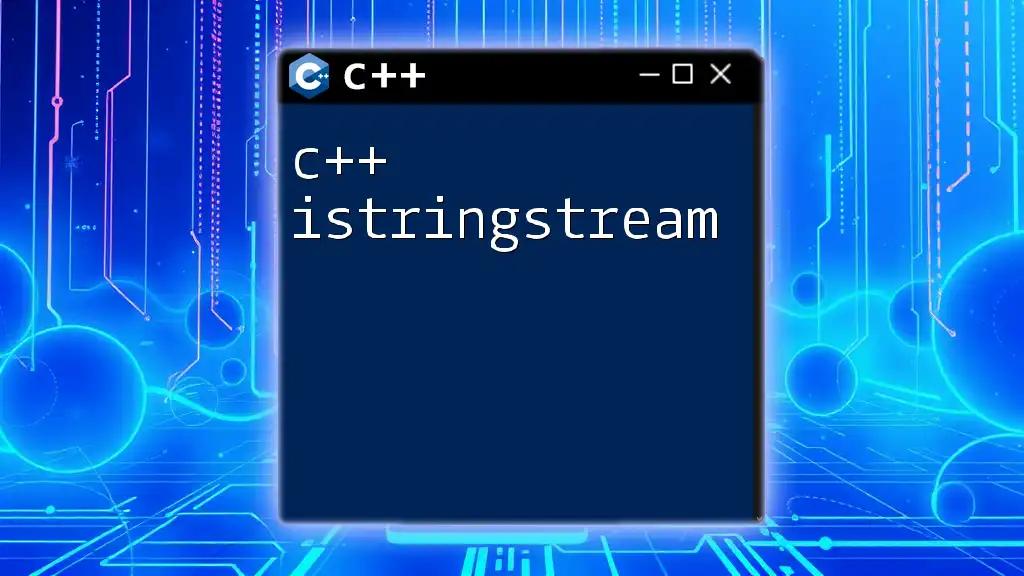
Performance Considerations
The Efficiency of `isdigit`
The `isdigit` function is highly optimized and designed for quick character classification. Using it in your programs not only simplifies logic but also enhances performance in scenarios involving extensive character validation. It's recommended to leverage `isdigit` whenever you need to validate numeric characters to ensure efficient and accurate processing.
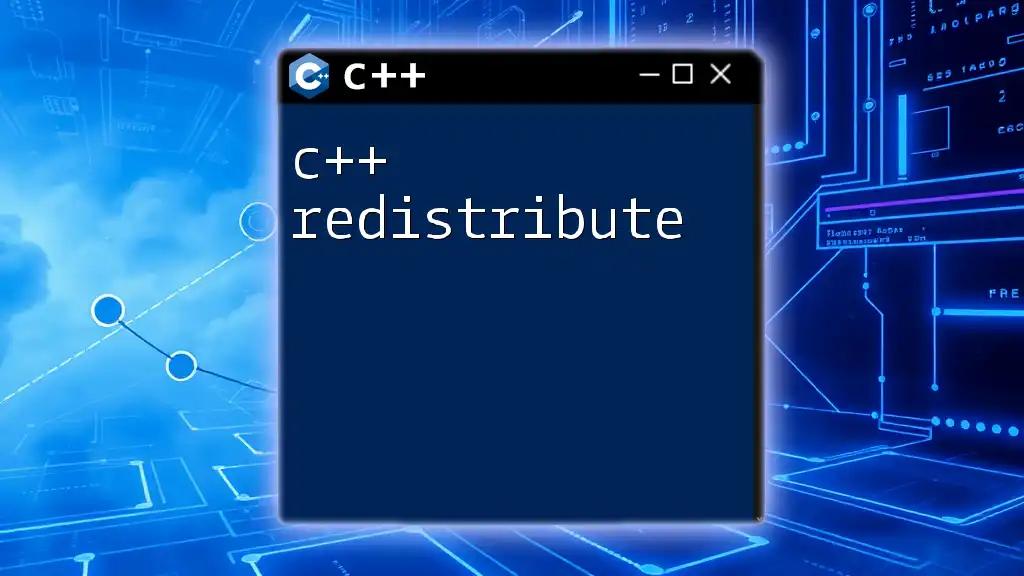
Alternatives to `isdigit`
Other Character Checking Functions
While `isdigit` is a powerful function for checking digit characters, C++ offers several related functions that aid in character classification:
- `isalpha`: Checks if a character is alphabetic (a-z or A-Z).
- `isalnum`: Determines if a character is alphanumeric (either a digit or an alphabetic character).
- `isspace`: Checks if a character is a whitespace character (spaces, tabs, newlines, etc.).
These functions can be used in combination with `isdigit` to create comprehensive validation logic for user input or data processing.
When to Use Custom Functions
Despite the effectiveness of `isdigit`, there may be instances where custom validation functions could be more appropriate. Consider creating custom logic if you have special requirements that go beyond simple digit checking, such as validating formatted numbers (e.g., currency or phone numbers).

Conclusion
In summary, the C++ `isdigit` function is an indispensable tool for character validation, particularly for ensuring that inputs are numeric. Whether checking single characters or validating strings, `isdigit` streamlines the process, making your code more readable and maintainable. Mastering the use of `isdigit` can significantly improve the quality and reliability of your C++ applications.
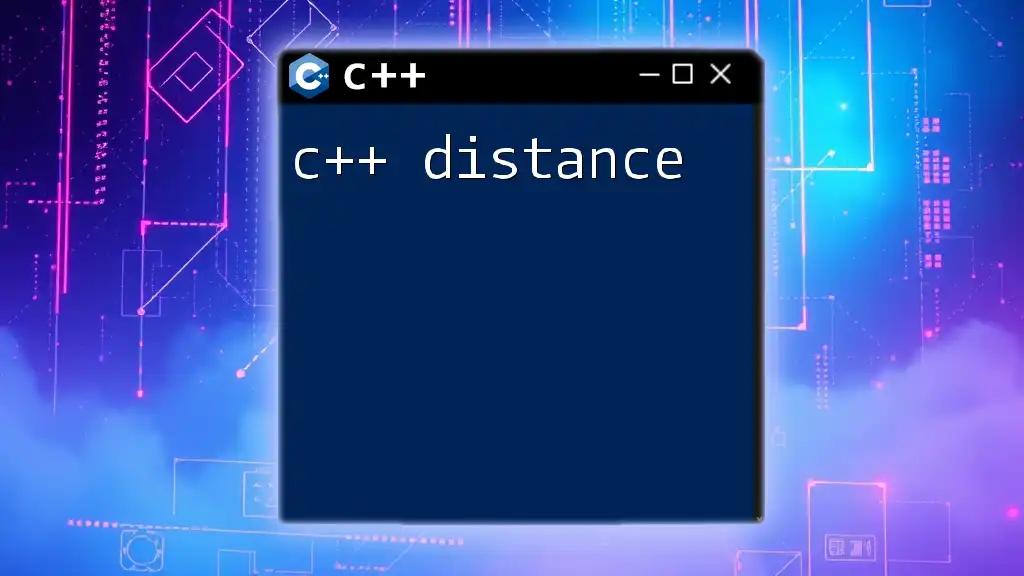
Additional Resources
References and Further Reading
For further learning, consider exploring authoritative resources such as:
- C++ Standard Library documentation for `isdigit` and related functions.
- Online programming communities and forums for practical tips and examples.
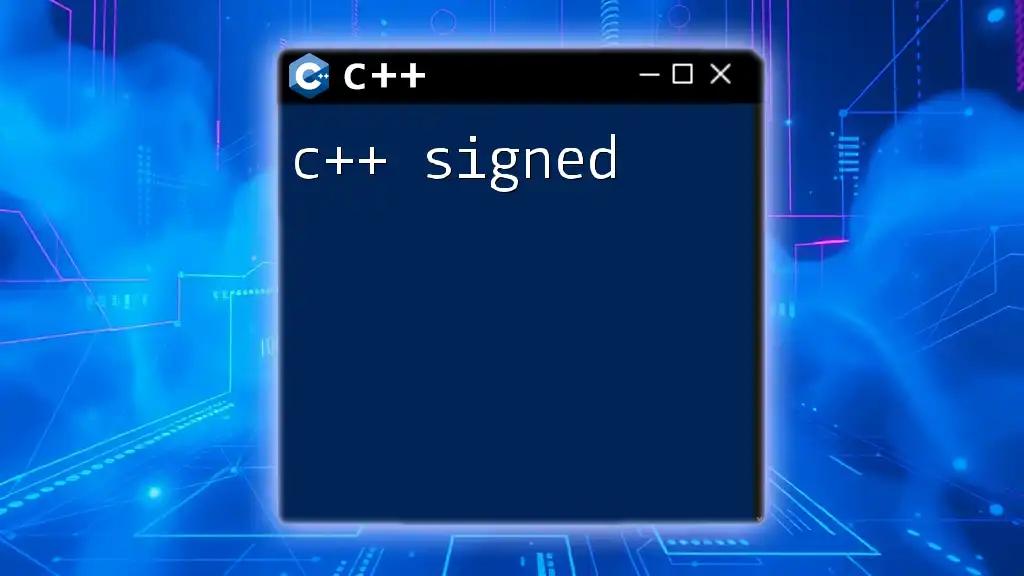
Call to Action
Practice implementing `isdigit` in your own projects, and share your experiences! Engaging with the material and experimenting with code will deepen your understanding and enhance your programming skills in C++.