In C++, a global variable is declared outside of any function, making it accessible from any function within the same file or across multiple files if properly referenced. Here’s an example:
#include <iostream>
int globalVar = 10; // Global variable
void printGlobal() {
std::cout << "Global variable: " << globalVar << std::endl; // Accessing global variable
}
int main() {
printGlobal();
return 0;
}
Introduction to C++ Global Variables
In C++, global variables are declared outside of any function or class and can be accessed from any part of the code. They have a program-wide scope, meaning that the same variable can be accessed by different functions, leading to easier data sharing across various sections of a program. Understanding the significance and use cases of global variables is crucial for effective programming.
Global variables can simplify certain tasks but may introduce complexity if not managed properly. They are often used to maintain state or configurations that need to be accessed by multiple functions. For instance, a global counter or configuration flag can be used throughout a program without passing it as a function argument.
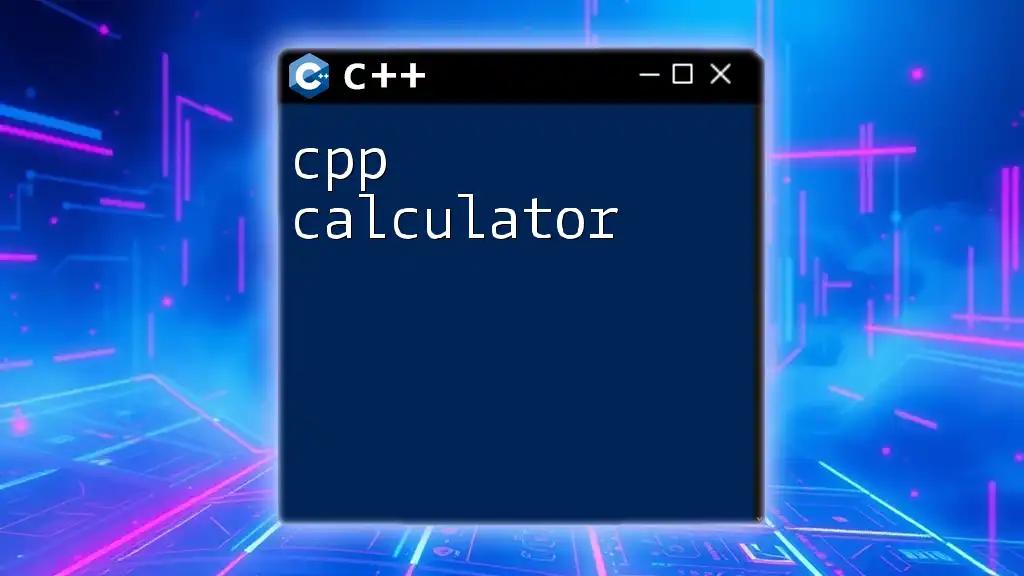
Understanding Scope and Variable Lifetime in C++
What is Scope?
Scope in programming refers to the visibility and accessibility of variables and functions within different regions of the code. In C++, variables have a scope that dictates where they can be accessed or modified.
Using global variables effectively requires an understanding of scope. Variables can have a local scope, meaning they are only visible within the function they are declared in, or a global scope, allowing visibility across multiple functions.
Types of Scope
Local Scope: Variables declared inside a function are said to have local scope. They cannot be accessed outside of that function.
Global Scope: Any variable declared outside of any functions or classes is part of the global scope and can be accessed by any function in the same file.
Variable Lifetime
Variable Lifetime relates to how long a variable exists in memory. Global variables remain in existence for the entire duration of the program, while local variables only exist during the execution of the function they are declared in.
Comparison of local and global scopes
Understanding the differences between local and global scopes is critical. Local variables can help prevent accidental interference with other functions, while global variables can simplify data management if used judiciously. However, excessive use of global variables can lead to a program structure that is hard to understand and maintain.
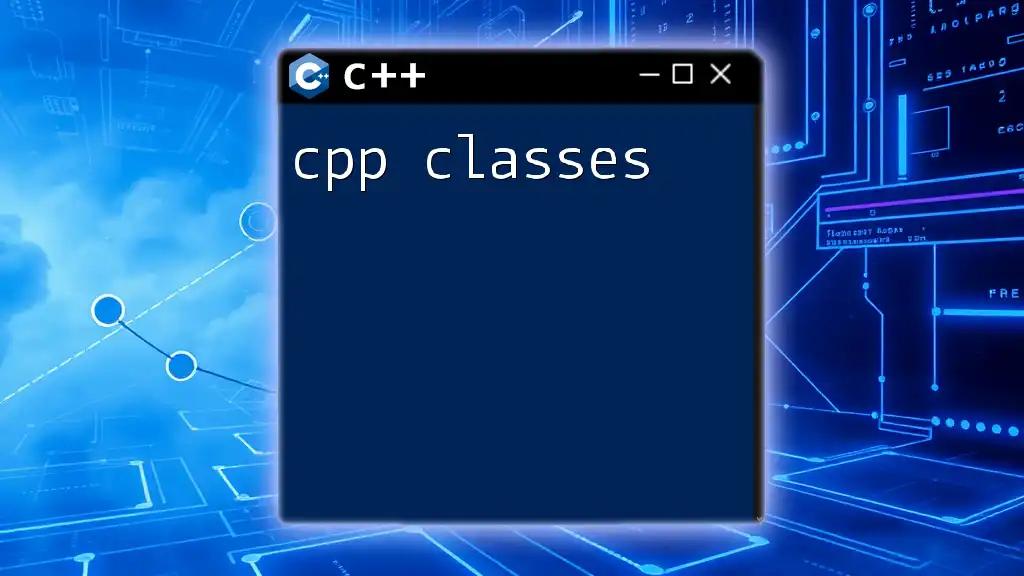
Declaring and Using Global Variables in C++
How to Declare Global Variables
Declaring a global variable is quite straightforward. The syntax for declaring a global variable is similar to local variables, but it must be placed outside any function or class.
Example of a global variable declaration:
int globalCounter = 0; // Global variable declaration
Initializing Global Variables
Global variables can be initialized explicitly or left to default values. If you choose to declare a global variable without an initial value, it will automatically be initialized to zero or to the equivalent of an "empty" state depending on its type.
Explicit initialization example:
double globalPi = 3.14159; // Explicitly initialized global variable
Accessing Global Variables
To access a global variable within functions, simply reference it by name. Here’s an example demonstrating how to increment a global variable:
void incrementCounter() {
globalCounter++; // Accessing global variable
}
In this function, the global variable `globalCounter` can be increased without requiring it to be passed as a parameter. This demonstrates one of the primary benefits of using global variables.
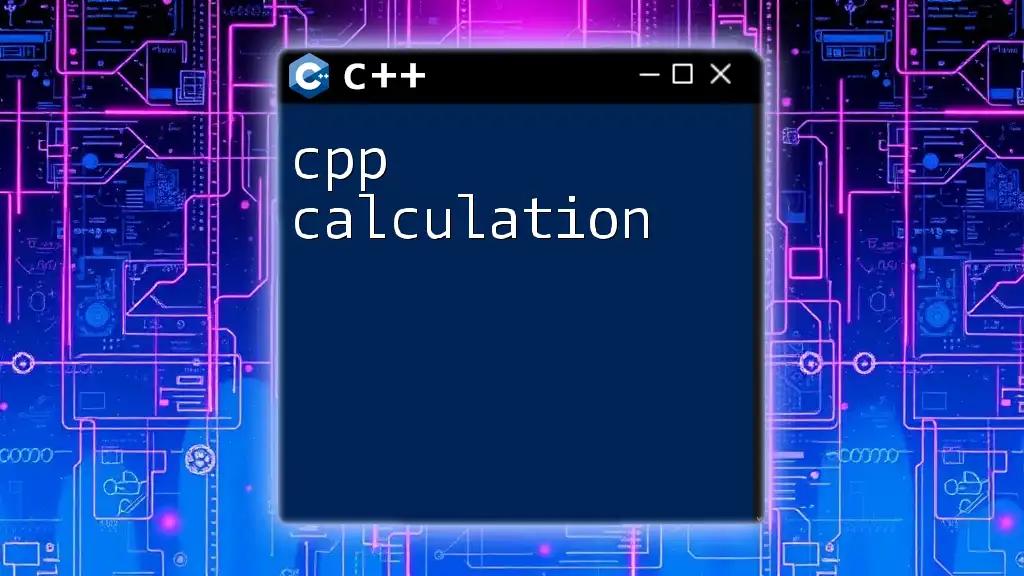
Best Practices for Using Global Variables
When to Use Global Variables
Global variables can be useful, but it’s essential to determine when it’s appropriate to use them. They are typically best employed in cases where state management needs to be shared across various functions without the overhead of passing multiple variables.
Avoiding Name Collisions
Using global variables can sometimes lead to naming conflicts with local variables of the same name. To mitigate this, using namespaces is a common strategy. Here’s an example:
namespace GlobalSpace {
int globalVar = 10; // Scoped global variable
}
This approach helps to clarify code scope and avoids confusion regarding which variable is being referenced.
Maintaining Code Readability
Readability is crucial in programming. Using global variables can sometimes make code harder to follow, especially in larger projects. Adopt consistent naming conventions and document your global variables clearly. For example, prefacing global variable names with `g_` (like `g_globalCounter`) helps indicate their global status, improving clarity.
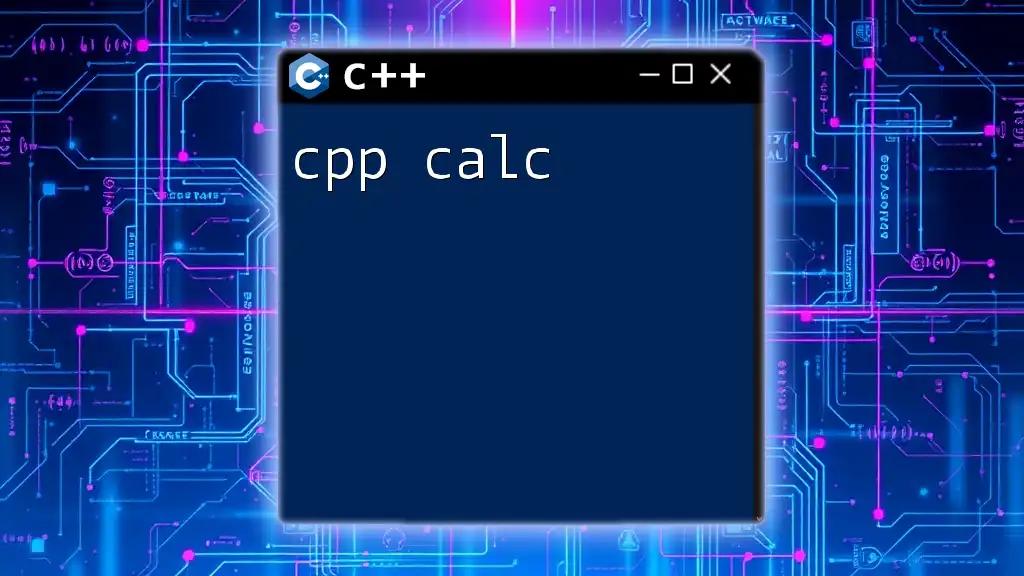
Common Pitfalls When Using Global Variables
Unintended Side Effects
One of the biggest challenges with global variables is unintended side effects. Modifying a global variable within a function can cause unexpected outcomes elsewhere in the program. For example:
void modifyGlobal() {
globalCounter += 10; // Unintentional side effect
}
Here, incrementing `globalCounter` may lead to issues if other parts of the program expect it to remain unchanged.
Thread Safety Concerns
In multi-threaded environments, global variables can pose thread safety concerns. They can be accessed by multiple threads simultaneously, leading to data inconsistency. To safeguard against this, implement synchronization mechanisms like mutexes or use thread-local storage to maintain separate copies of variables for each thread.
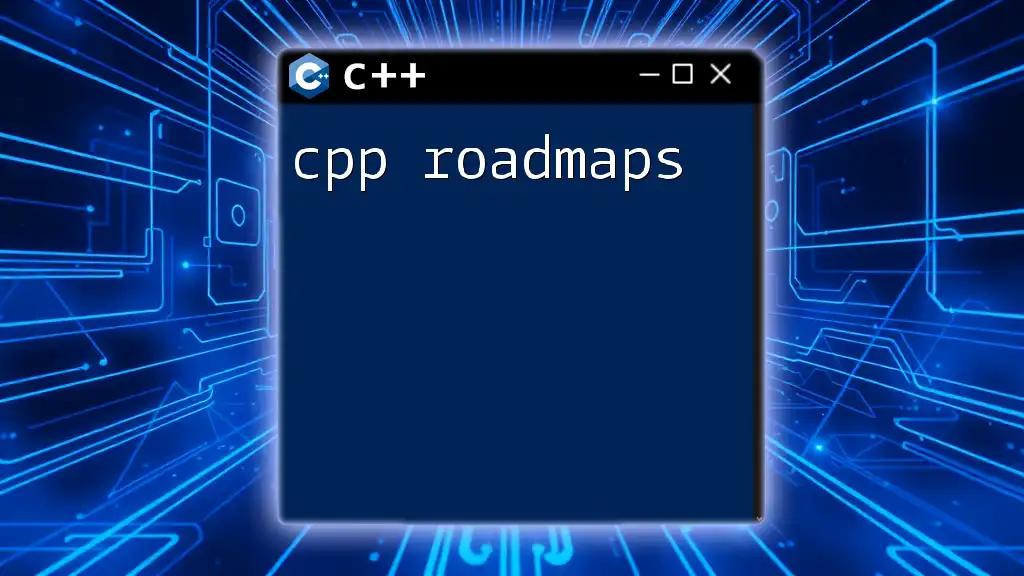
Alternatives to Global Variables
Static Variables
An alternative to global variables is static variables. These variables retain their values between function calls but are only accessible within the function they are declared in. This provides a level of encapsulation while maintaining state. Consider the following example:
void myFunction() {
static int staticCounter = 0; // Static variable retains value between calls
staticCounter++;
// ...
}
Here, `staticCounter` is preserved across different invocations of `myFunction`, but its access is limited to within the function.
Singleton Pattern
Another approach to managing variable state without global variables is using the Singleton design pattern. This pattern ensures a class has only one instance and provides a global point of access to it. Here’s an example:
class Singleton {
public:
static Singleton& getInstance() {
static Singleton instance; // Guaranteed to be destroyed
return instance;
}
private:
Singleton() {} // Constructor is private to prevent instantiation
};
Using a singleton reduces global state pollution while still providing a globally accessible instance of the class.
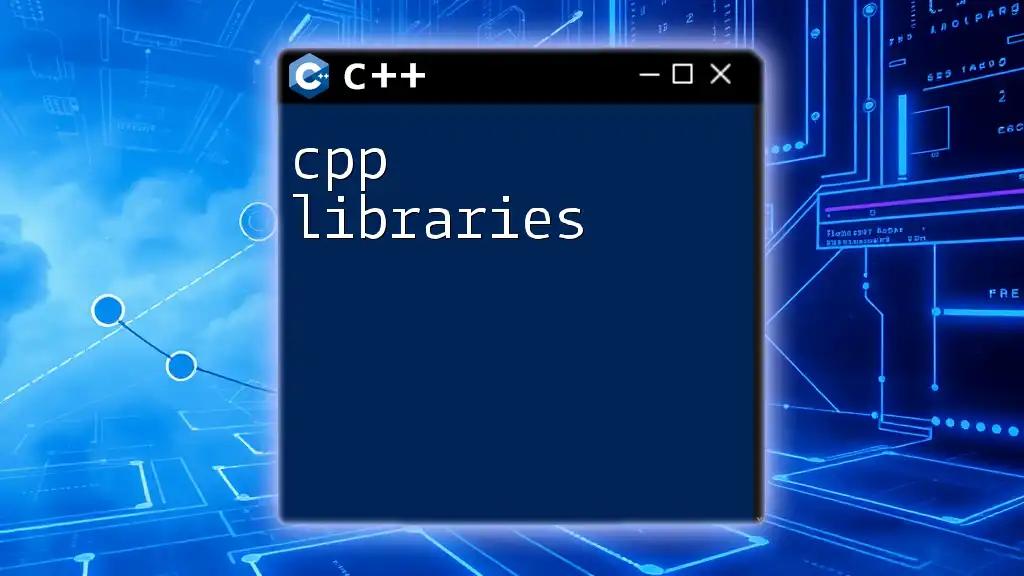
Conclusion
Global variables in C++ play an essential role in managing state and data across multiple functions. However, they come with both benefits and potential pitfalls. This guide has outlined the nature of global variables, best practices for their use, and alternatives to consider for maintaining state in your programs. By using global variables judiciously, you can create robust and maintainable C++ applications.
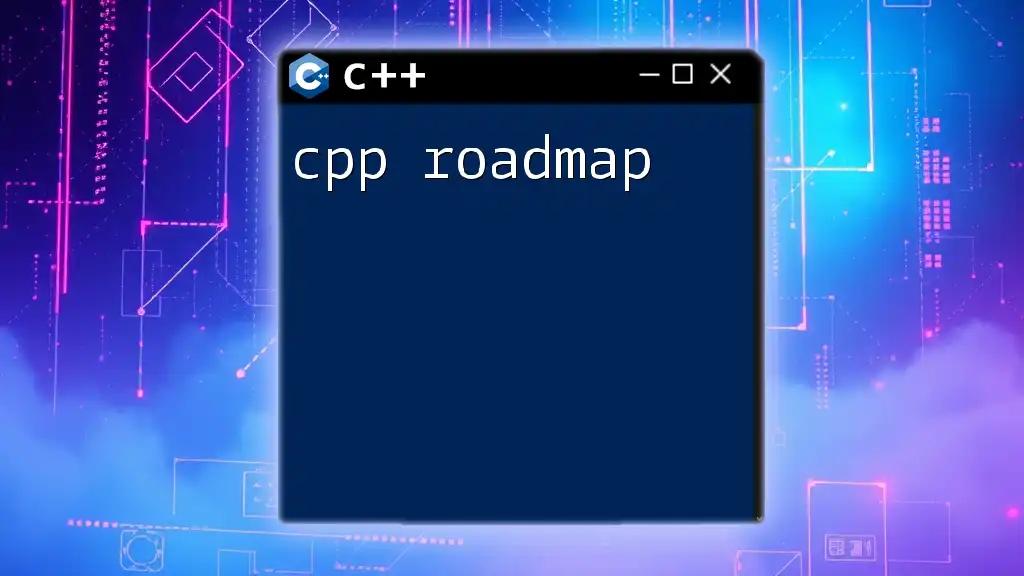
Call to Action
To explore more tips and tricks in C++ programming, subscribe to our newsletter and join our community for discussions and practical coding examples. Your journey toward mastering C++ starts here!