Creating a CSV file in C++ can be done easily by using file output streams to write comma-separated values to a text file.
Here's a simple example:
#include <fstream>
#include <iostream>
int main() {
std::ofstream file("output.csv");
if (file.is_open()) {
file << "Name,Age,Occupation\n";
file << "Alice,30,Engineer\n";
file << "Bob,25,Designer\n";
file.close();
} else {
std::cerr << "Unable to open file";
}
return 0;
}
Understanding CSV Files
What is a CSV File?
A CSV file, or Comma-Separated Values file, is a simple text format used for storing tabular data. Each line in the file corresponds to a single row, and the values within that row are separated by commas, making it easy to import or export data across various applications. The structure is straightforward and typically consists of headers that define the data fields followed by rows of data entries.
Uses of CSV Files
CSV files are widely utilized in software applications for various purposes, such as:
- Data export and import between applications (e.g., spreadsheets, databases)
- Storing datasets for easy data manipulation and analysis
- Logging and archiving data due to their human-readable format
One of the primary advantages of using CSV over other file formats (like Excel) is its simplicity and ease of integration with programming languages, including C++. This makes it an excellent choice for data handling in C++ programs.
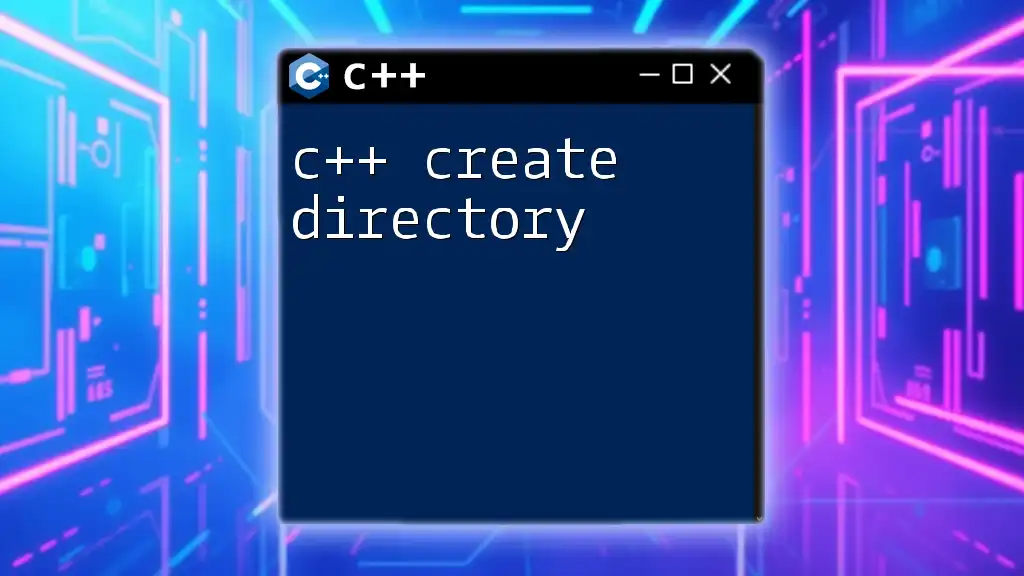
Setting Up the Environment
Required Tools
Before delving into the implementation, it's essential to set up the development environment appropriately. Some recommended IDEs for C++ development include:
- Visual Studio: A feature-rich IDE that provides extensive support for C++ development.
- Code::Blocks: A lightweight, open-source IDE that is suitable for C++.
In most cases, you'll rely on the standard C++ libraries, particularly `<fstream>`, which provides functionality to handle file streams.
Basic C++ Concepts Recap
To create a CSV file in C++, you should have a basic understanding of file handling concepts. You will typically use:
- `std::ofstream`: For writing to files.
- `std::ifstream`: For reading from files.
- Include necessary headers:
#include <iostream>
#include <fstream>
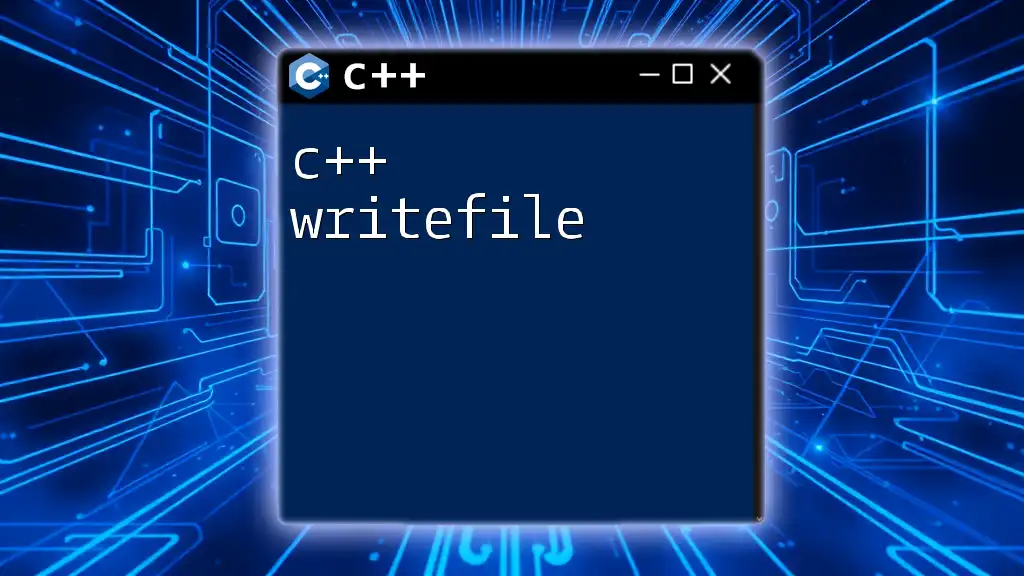
Implementing CSV File Creation in C++
Opening a File Stream
To create and write to a CSV file in C++, you need to open a file stream correctly. This is achieved through `std::ofstream` which enables writing data to files.
Writing to a CSV File
Creating and Opening a CSV File
Creating a CSV file is as simple as declaring an `std::ofstream` object and specifying the filename. Here's a sample code snippet:
std::ofstream csvFile("data.csv");
The `data.csv` file will be created in the current working directory if it doesn’t already exist.
Writing Data to the CSV File
Once the file is opened, you can start writing data to it. It’s essential to follow a structured format: you usually start with a header row that describes the data columns, followed by the subsequent data rows separated by commas. Here’s an example of how to write data:
if (csvFile.is_open()) {
csvFile << "Name,Age,Occupation\n"; // Header
csvFile << "Alice,30,Engineer\n"; // Data rows
csvFile << "Bob,25,Designer\n";
csvFile.close(); // Closing the file after writing
}
In this snippet, we first check if the file is open, then proceed to write the header and the data rows. It’s good practice to include a newline character (`\n`) to separate each entry properly.
Closing the File Stream
After writing your data, it's crucial to close the file stream using `csvFile.close()`. Failing to do this can lead to data loss or corruption. The operating system may not properly save the file contents if the application terminates unexpectedly. Closing the file properly ensures that all data is flushed from the buffer to the file.
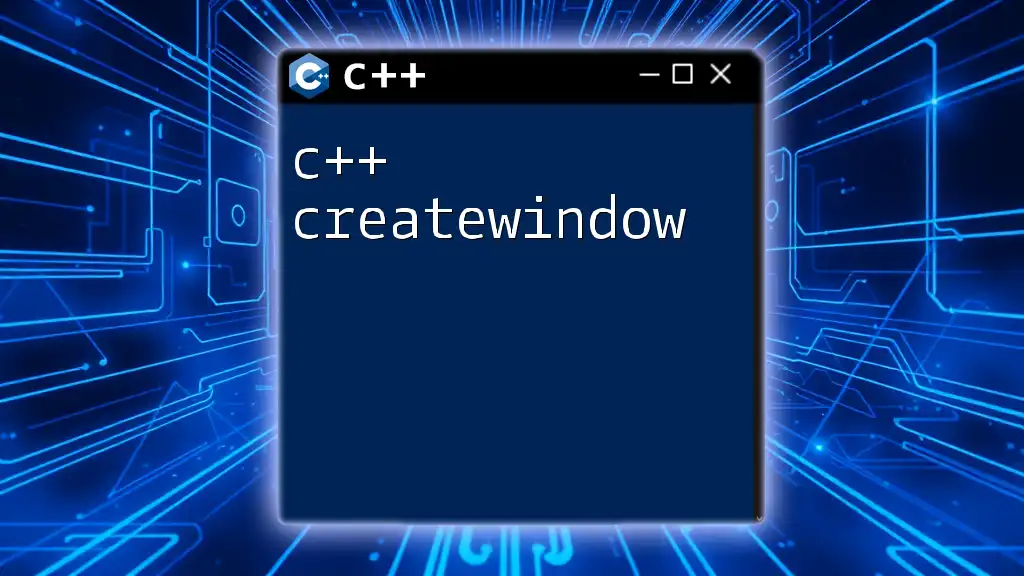
Handling Errors
Checking File Operations
To ensure robust code, always check if the file operations are successful. If the file fails to open, it’s important to handle that case gracefully. Here’s how you can implement this:
if (!csvFile) {
std::cerr << "Error opening file." << std::endl;
}
This conditional statement will inform you of any issues at the moment of file opening.
Error Handling Techniques
Error handling is a critical practice in programming. Use try-catch blocks to handle exceptions that may occur during file operations. Here's an example:
try {
std::ofstream csvFile("data.csv");
if (!csvFile) {
throw std::ios_base::failure("Failed to open file.");
}
// Write to the file...
} catch (const std::ios_base::failure& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
In this case, any issues that arise during file creation or writing will be caught, preventing the program from crashing unexpectedly.
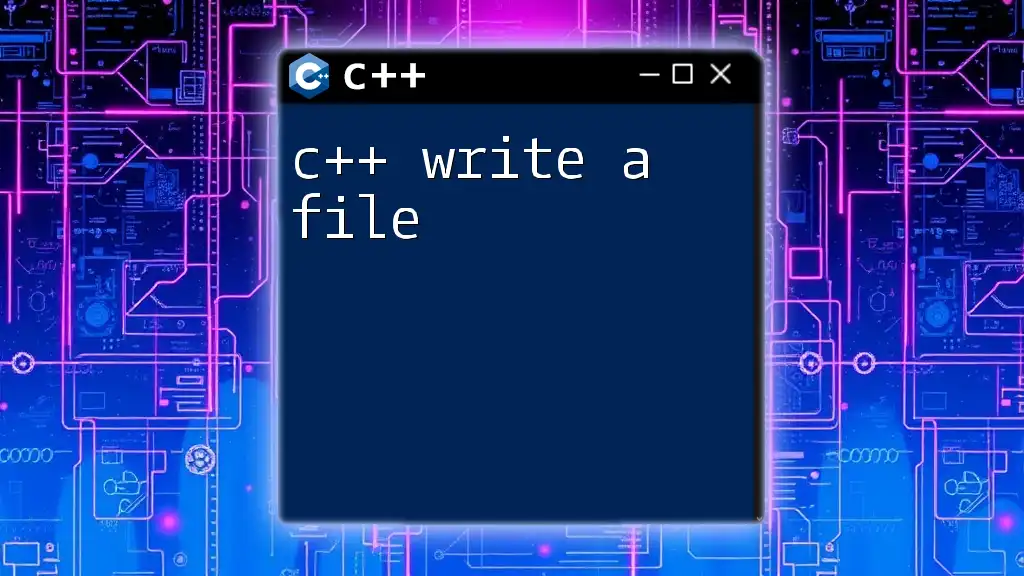
Reading from a CSV File (Bonus)
Introduction to Reading CSV Files
While creating a CSV file is essential, knowing how to read from one is equally valuable. This ability allows for the extraction and manipulation of data stored within the CSV format.
Code Example for Reading a CSV File
To read from a CSV file, utilize `std::ifstream`. Here's how to do it effectively:
std::ifstream csvInput("data.csv");
std::string line;
if (csvInput.is_open()) {
while (std::getline(csvInput, line)) {
std::cout << line << std::endl; // Print each line
}
csvInput.close();
} else {
std::cerr << "Unable to open file." << std::endl;
}
In this example, the program reads each line from the CSV file until it reaches the end, printing it to the console. Again, we check if the input file successfully opens before performing any read operations.
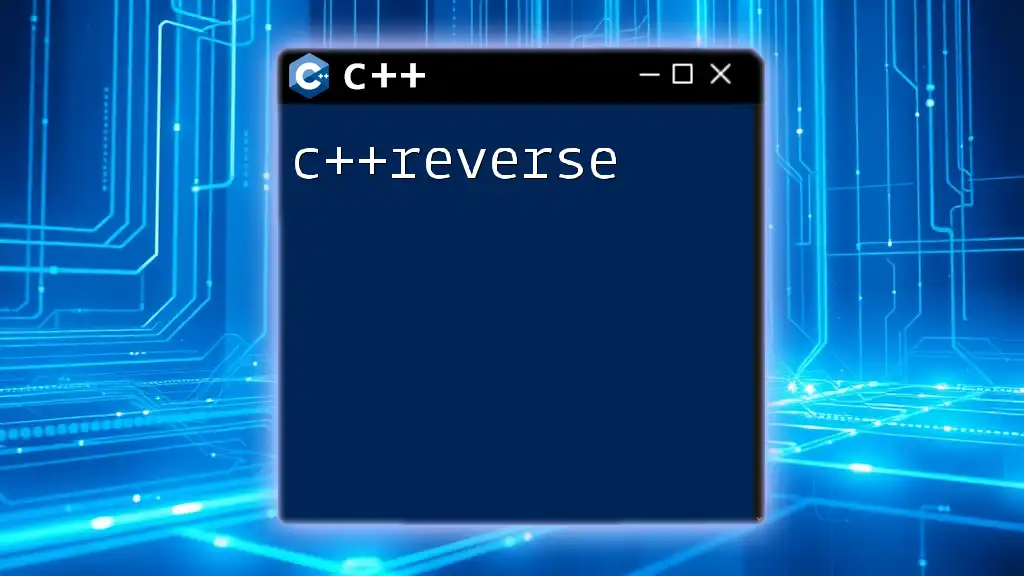
Best Practices for CSV File Handling
Organizing Data
When creating CSV files, always maintain consistent formatting. Headers should clearly define each data field and mirror the data types across columns. This organization allows for easier analysis and manipulation later on.
Performance Considerations
For larger datasets, processing efficiency becomes a concern. While CSV files are lightweight, handling significant volumes of data can be performance-intensive. In such cases, consider whether a more sophisticated storage option (e.g., databases) might be more appropriate.
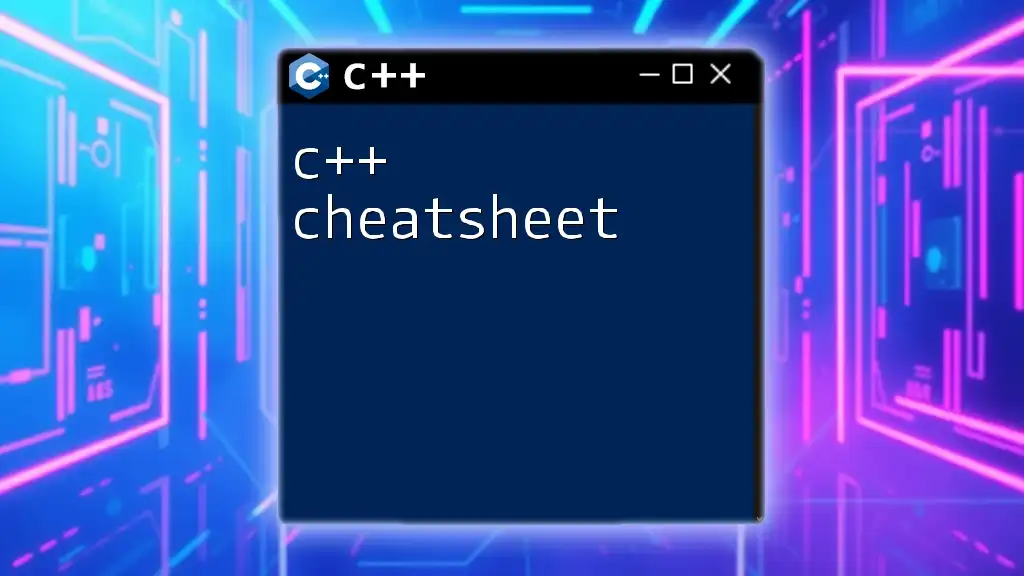
Conclusion
Creating a CSV file in C++ may seem straightforward, yet it encompasses various crucial concepts in file handling. By understanding how to create, write, and even read CSV files, programmers can efficiently manage and process data in their applications.
Practicing by creating your own CSV files and experimenting with error handling techniques will consolidate your understanding. As you move forward in your C++ journey, leveraging CSV files for data management will undoubtedly enhance your programming toolkit. Remember to share your experiences and challenges while working with CSV files in C++; your insights may help peers facing similar situations.
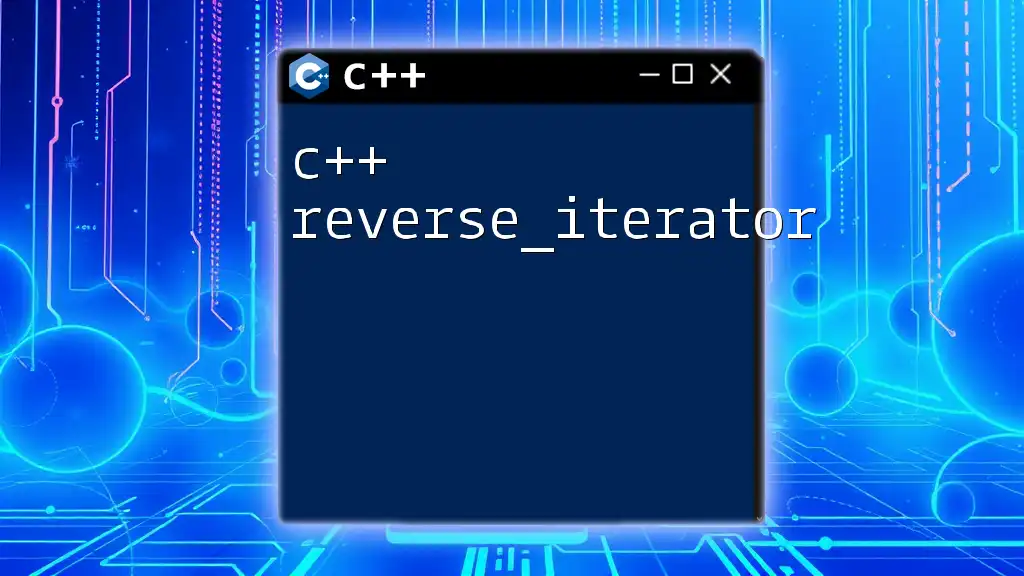
Additional Resources
For a deeper understanding of C++, refer to the official C++ documentation regarding file handling. There are also numerous online courses and tutorials designed to enhance your programming skills further. Explore these resources to solidify your knowledge in creating and managing CSV files effectively.