"CPP for students is a comprehensive guide designed to simplify C++ commands and concepts, enabling quick learning and effective coding."
Here's a basic code snippet that demonstrates a simple "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What is C++?
C++ is a general-purpose programming language developed by Bjarne Stroustrup in the early 1980s as an enhancement of the C programming language. It is widely recognized for its versatility in different types of software development, ranging from systems software to game development. C++ introduced features such as classes, inheritance, and polymorphism, making it an essential choice for object-oriented programming.
In today's digital world, C++ remains a foundational language, particularly in performance-critical applications and systems programming, rendering it an invaluable asset for students looking to build a career in technology.
Why Learn C++ as a Student?
Learning C++ presents numerous benefits for students:
- Career Advancement: Many technology firms seek proficiency in C++ for roles such as software developers, embedded systems engineers, and game developers.
- Broad Applications: C++ finds utility in a wide range of domains, including video games, real-time simulations, operating systems, and web browsers.
- Strong Community Support: With a long-lasting legacy, C++ boasts an extensive community that provides ample resources, tutorials, and forums, allowing students to learn and troubleshoot efficiently.
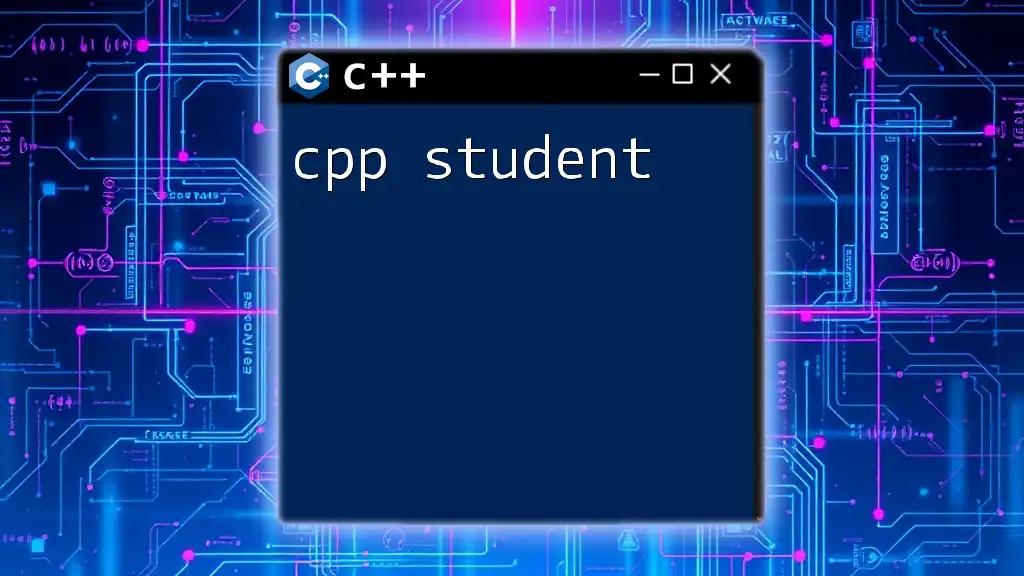
Setting Up Your Environment
Installing C++ Compiler
To start programming in C++, you need to install a compiler that translates your C++ code into machine-readable instructions. Some popular compilers include:
- GCC: A widely used open-source compiler available on multiple platforms.
- Clang: Known for its excellent diagnostics and modular architecture.
- MSVC: Microsoft's Visual C++ Compiler, which is robust and features-rich for Windows development.
Installation Steps
- For Windows: You can install MinGW for GCC or download Visual Studio for MSVC.
- For macOS: Clang is available by default; however, you can install GCC via Homebrew.
- For Linux: GCC can typically be installed using your package manager (e.g., `sudo apt install g++`).
Choosing an Integrated Development Environment (IDE)
An IDE can significantly enhance your programming experience. Here are a few popular choices:
- Visual Studio: Rich features and debugging capabilities, primarily for Windows.
- Code::Blocks: A lightweight, open-source IDE suitable for Windows, Mac, and Linux.
- Eclipse: A robust IDE that supports various programming languages, including C++.
When choosing an IDE, consider ease of use, available features, and community support.

Basic C++ Syntax
First Program: Hello World
The quintessential first program for beginners is to display "Hello, World!" on the screen. Here’s how you can do it in C++:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this code, `#include <iostream>` includes the library for input and output operations. The `main` function is where program execution begins. `cout` is used to print text to the console.
Variables and Data Types
C++ offers several built-in data types essential for variable declaration:
- int: For integers.
- float: For floating-point numbers.
- char: For single characters.
- string: For sequences of characters.
You can declare and initialize variables as follows:
int age = 20;
float salary = 3000.50;
char grade = 'A';
string name = "John Doe";
Understanding how to correctly use these data types is fundamental for effective coding in C++.
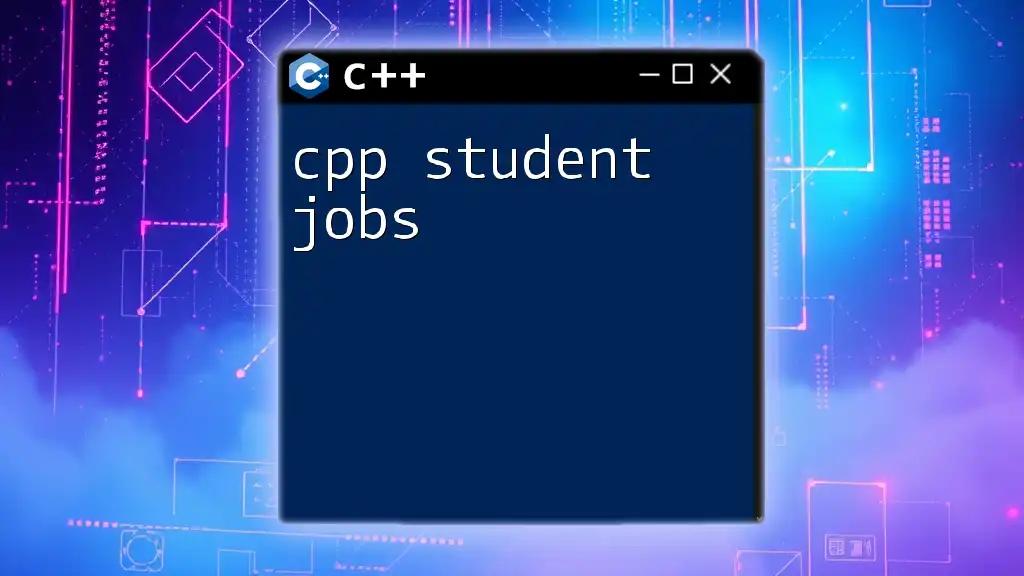
Control Structures
Conditional Statements
C++ allows you to implement logic through conditional statements, guiding the flow of execution based on conditions. The `if`, `else`, and `switch` statements are vital in this context.
For example, using an `if` statement:
if (age >= 18) {
cout << "Adult" << endl;
} else {
cout << "Minor" << endl;
}
This code checks if the `age` variable is 18 or more and prints the corresponding message.
Loops
Loops help in executing repetitive tasks efficiently. C++ provides several types of loops:
- for Loop: Best used when the number of iterations is known.
for (int i = 0; i < 10; i++) {
cout << i << " ";
}
- while Loop: Suitable when the number of iterations is unknown but relies on a condition.
int count = 0;
while (count < 5) {
cout << count << " ";
count++;
}
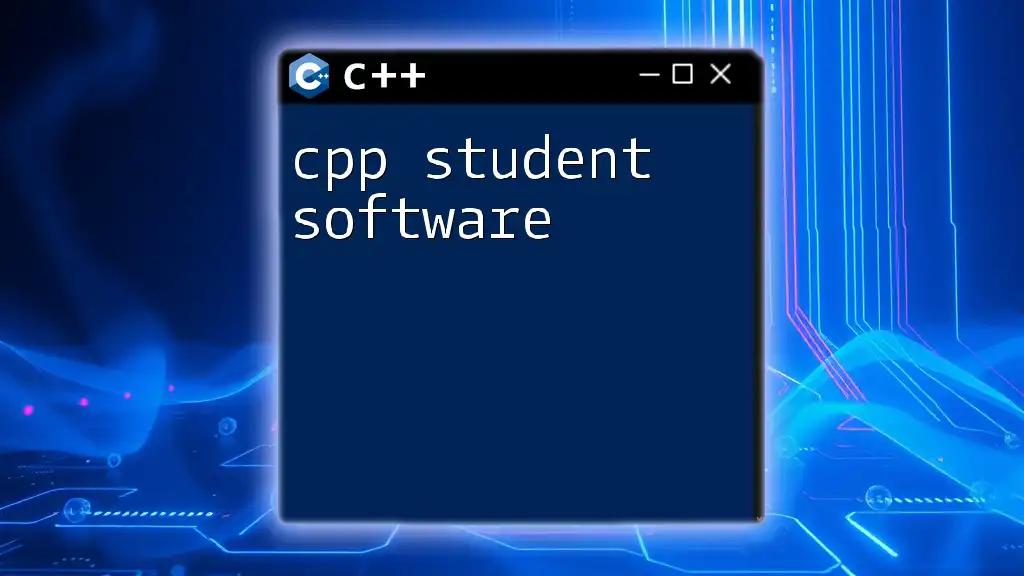
Functions in C++
Defining and Calling Functions
Functions modularize code, making it more organized and reusable. Here’s how to define a simple function:
int add(int a, int b) {
return a + b;
}
This code defines a function named `add` that takes two integer parameters and returns their sum.
Function Overloading
C++ allows multiple functions to have the same name as long as their parameter types differ or the number of parameters varies, known as function overloading.
int add(int a, int b);
double add(double a, double b);
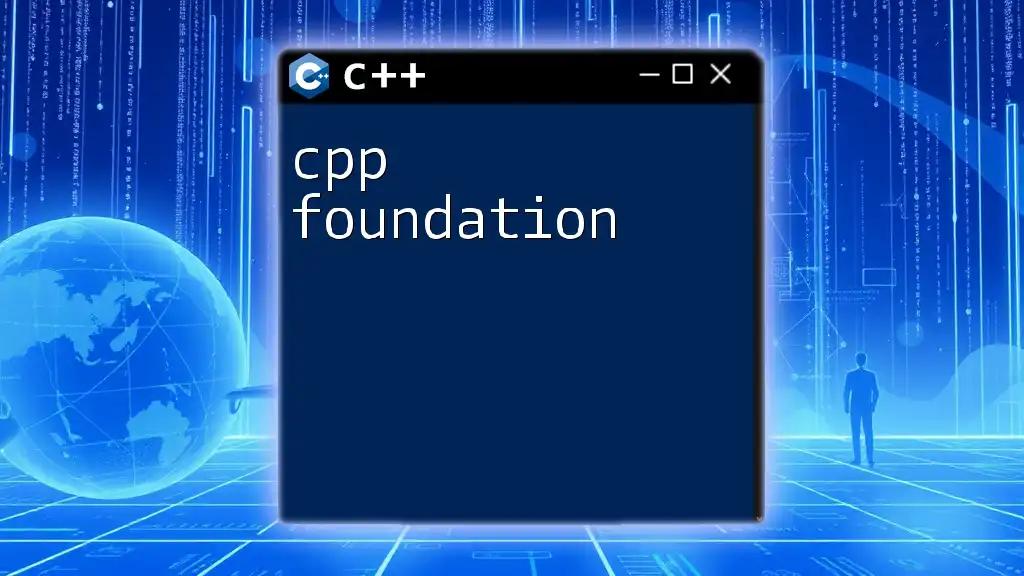
Object-Oriented Programming in C++
Understanding Classes and Objects
C++ is an object-oriented programming language, which means it expertly manages data through classes and objects. A class is a blueprint, while an object is an instance of that class.
Here’s an example:
class Dog {
public:
string breed;
int age;
void bark() {
cout << "Woof!" << endl;
}
};
In this code, the `Dog` class has two attributes (`breed` and `age`) and a method `bark`.
Constructors and Destructors
Constructors initialize objects when they are created, while destructors are called when objects are deleted. Understanding these concepts is essential to managing resource allocation effectively.
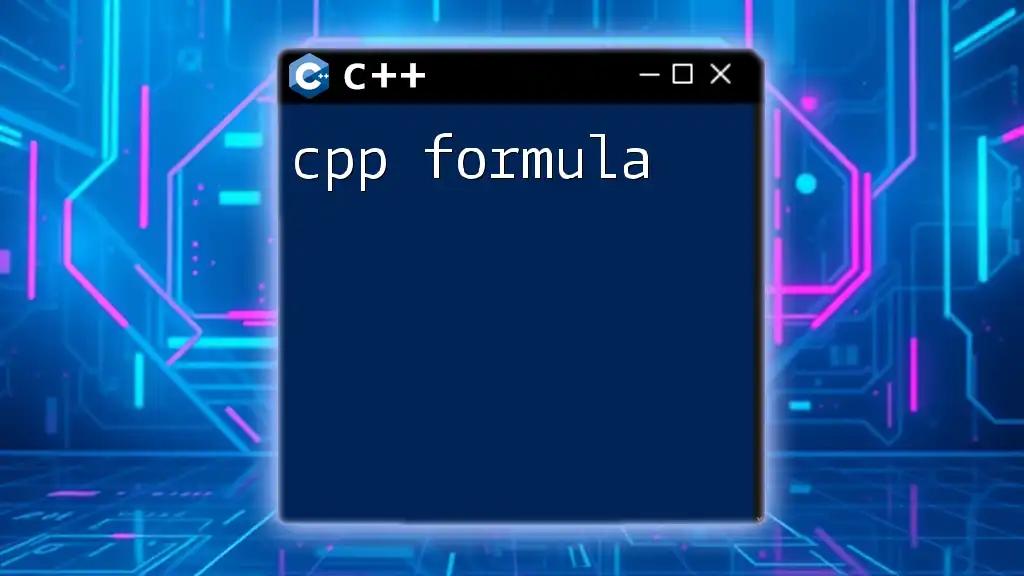
Advanced Concepts
Pointers and References
Pointers and references are advanced features in C++ that allow for direct memory management. A pointer holds the address of a variable:
int a = 10;
int* p = &a; // Pointer to a
This line of code declares an integer `a` and a pointer `p` that points to `a`'s memory address.
Templates
Templates provide a way to create generic and reusable code. By using templates, you can write functions or classes that can operate with any data type. Here’s an example of a function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function can now add integers, floats, or any other type that supports the `+` operator.

Common C++ Libraries
Introduction to STL (Standard Template Library)
The Standard Template Library (STL) is a powerful library that provides classes and functions for algorithms and data structures. From vectors to lists, it simplifies many programming tasks.
For example, using the `vector` class:
#include <vector>
using namespace std;
vector<int> numbers = {1, 2, 3, 4, 5};
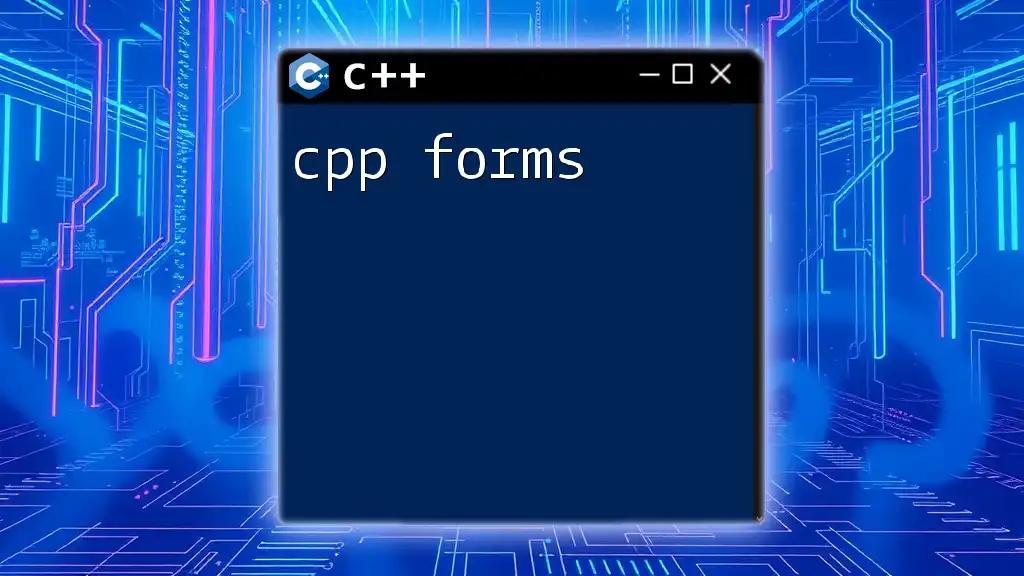
Best Practices for Students
Debugging Techniques
Effective debugging is crucial for developing robust C++ applications. Students should familiarize themselves with both compiler error messages and debugging tools like `gdb` or the integrated debugging features of their IDE.
Writing Readable Code
Clarity and organization are critical when writing code. Use meaningful variable names, adhere to consistent naming conventions, and comment on complex code sections. These practices enhance code readability and maintainability.
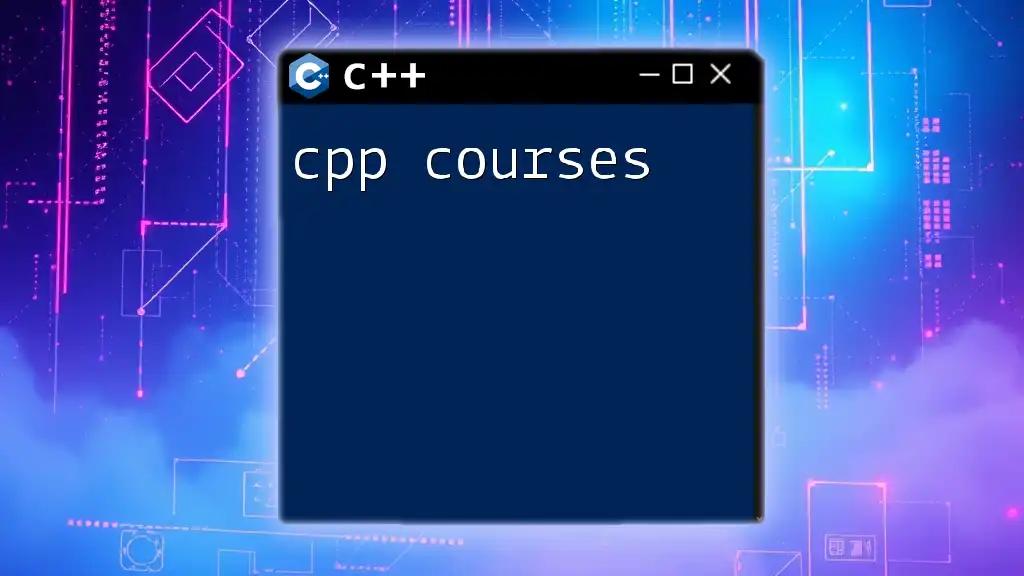
Resources for Further Learning
Recommended Books and Online Courses
Several resources aid in enhancing your understanding of C++:
- Books: Titles like "The C++ Programming Language" by Bjarne Stroustrup and "Effective C++" by Scott Meyers provide deep insights.
- Online Courses: Platforms like Coursera, Udemy, and Codecademy offer interactive C++ courses tailored for students.
Joining C++ Communities
Engaging with fellow learners and experts can quicken your learning curve. Consider joining platforms such as Stack Overflow, Reddit's r/cpp, or GitHub to discuss issues, ask questions, and collaborate on projects.

Conclusion
Mastering C++ serves as a powerful foundation for any aspiring technologist. Through diligent practice and leveraging the resources outlined in this guide, students can unlock the potential of C++ and pave their way for successful careers in programming.