The Audio C++ library enables developers to manage audio playback, recording, and processing tasks efficiently within their C++ applications.
Here's a simple example of using the Audio C++ library to play an audio file:
#include <SFML/Audio.hpp>
int main() {
sf::Music music;
if (!music.openFromFile("audio_file.ogg")) {
return -1; // error
}
music.play();
while (music.getStatus() == sf::SoundSource::Playing) {
// Keep the program running while the music is playing
}
return 0;
}
What is a C++ Audio Library?
An audio C++ library serves as a packaged set of tools and functions that facilitate audio processing in C++ applications. These libraries streamline the complex task of audio manipulation by handling low-level tasks such as file handling, streaming, and audio playback, allowing developers to focus on higher-level functionality. Utilizing an audio library ensures improved efficiency, maintainability, and a more robust development process over implementing audio functionality from scratch.
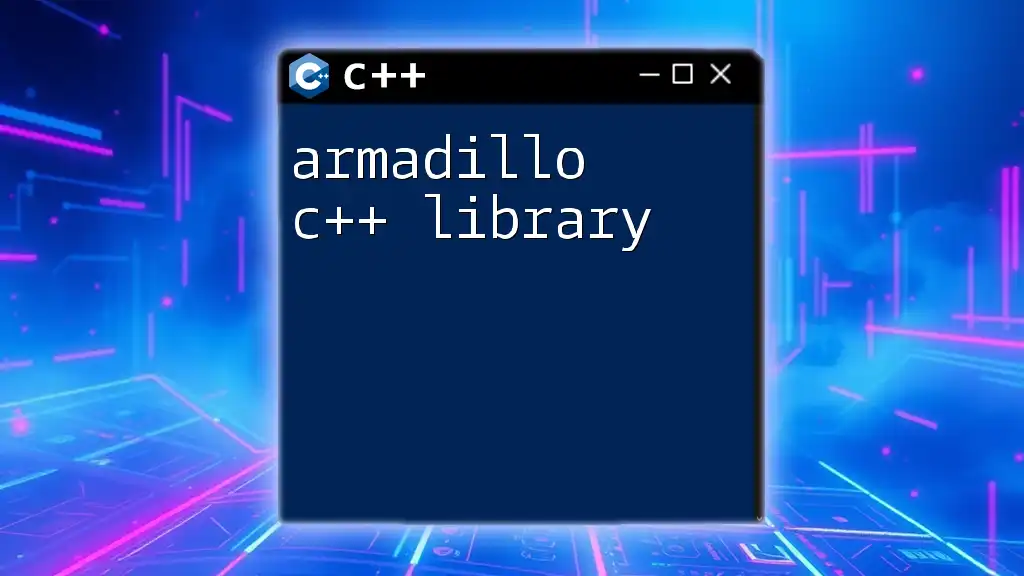
Popular C++ Audio Libraries
Several reputable audio C++ libraries are available, each offering unique features and functionalities:
- PortAudio: This is a cross-platform library that provides a simple API for audio input and output. It's widely used for both recording and playing sound.
- SFML Audio: Part of the Simple and Fast Multimedia Library, SFML Audio enables simple audio playback and manipulation with straightforward APIs.
- RtAudio: This high-level C++ wrapper provides an easy interface for real-time audio input/output across different platforms.
Choosing the Right Library
When selecting an audio library, consider the following factors:
- Ease of Use: A library with a simple-to-understand API will accelerate the learning curve.
- Compatibility: Check if the library supports your target platforms and audio formats.
- Performance: Evaluate the library's performance characteristics, especially for real-time audio applications.
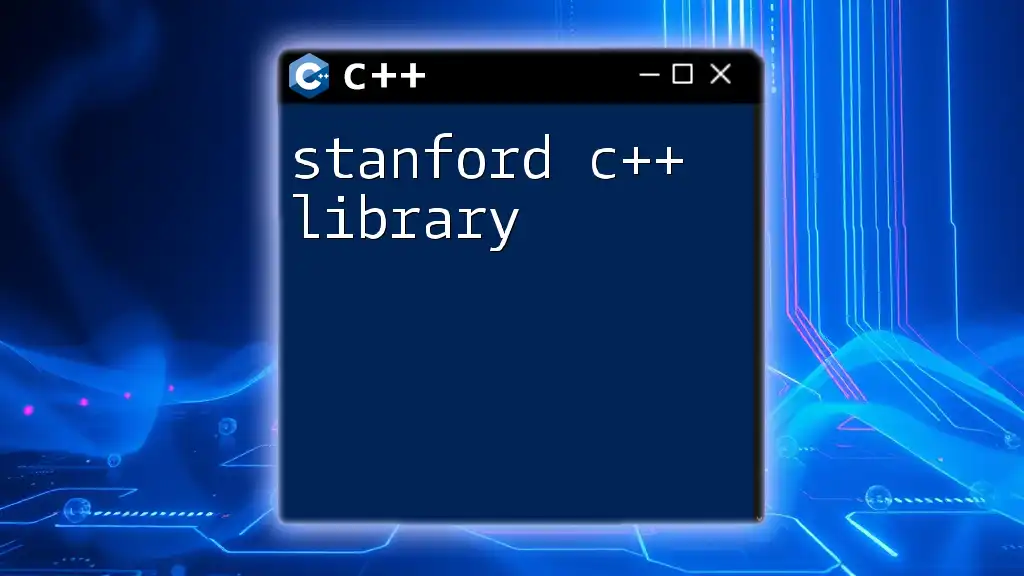
Setting Up Your C++ Audio Library
Installation and Setup
For this guide, let’s focus on PortAudio. Follow these steps to install and set it up:
- Download PortAudio: Visit the [PortAudio website](http://www.portaudio.com/) and download the latest version.
- Extract the files and read the README or INSTALL files for platform-specific instructions.
- Compile the library: Depending on your platform, you may need to run specific build scripts or use a build system like CMake.
Basic Configuration
Once installed, configure your development environment:
- Ensure the PortAudio headers and library files are included in your project settings.
- Link against the appropriate PortAudio library files.
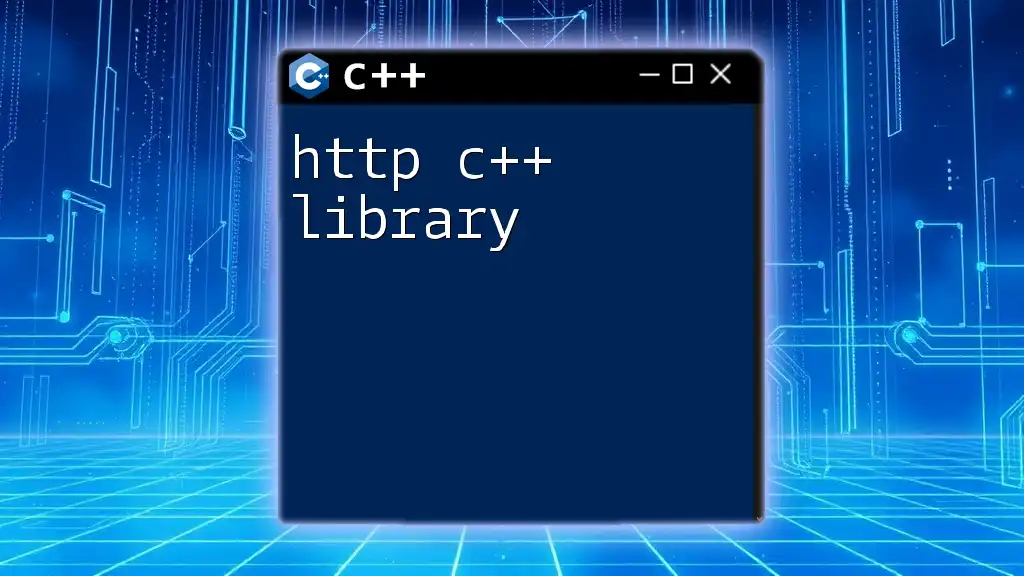
Core Concepts of Audio Processing
Audio Formats and Encoding
Understanding audio formats and encoding is crucial for effective audio processing. Common formats include:
- WAV: An uncompressed format that retains high sound quality.
- MP3: A compressed format optimized for space, but may reduce quality.
- OGG: A free, open-source format that provides good quality and compression.
Choosing the right format can impact audio quality and processing efficiency significantly.
Understanding Audio Streams
An audio stream refers to a flow of audio data. You can have:
- Input Streams: Used for capturing audio from a microphone.
- Output Streams: Used for playing audio through speakers.
To create a simple audio stream in PortAudio, consider the following example:
#include <iostream>
#include <portaudio.h>
int main() {
Pa_Initialize();
// Your input/output stream code here
Pa_Terminate();
return 0;
}
In this code snippet, we initialize PortAudio, setting the stage for audio processing.
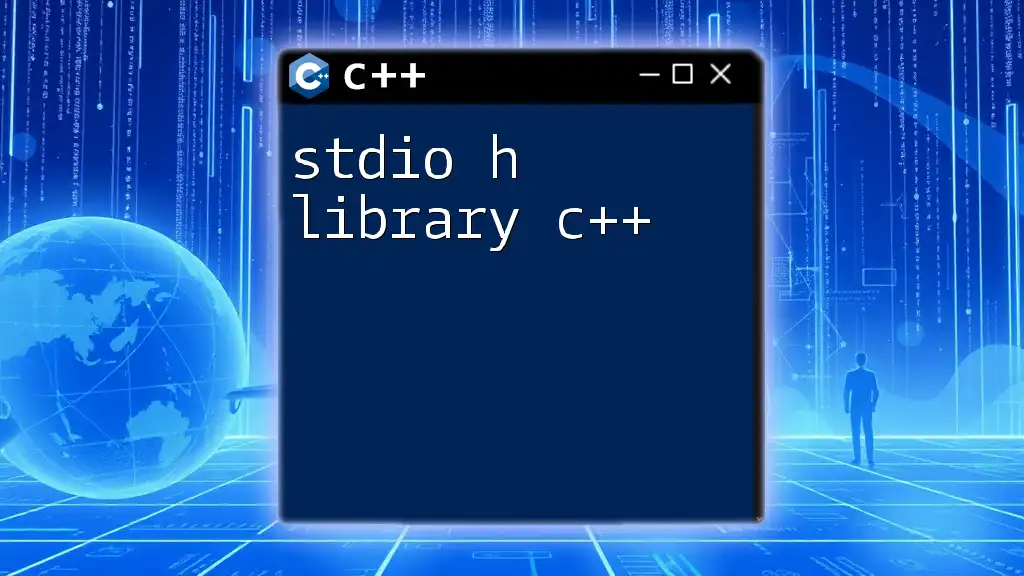
Using the C++ Audio Library: Basic Functionality
Playing Sound
To load and play a sound file using PortAudio, the code snippet below demonstrates the procedure:
#include <iostream>
#include <portaudio.h>
static int playCallback(const void* inputBuffer, void* outputBuffer,
unsigned long framesPerBuffer,
const PaStreamCallbackTimeInfo* timeInfo,
PaStreamCallbackFlags statusFlags) {
// Fill outputBuffer with audio data, e.g., read from a file or buffer
return paContinue;
}
int main() {
PaError err;
err = Pa_Initialize();
// Handle error
PaStream* stream;
err = Pa_OpenDefaultStream(&stream, 0, 2, paFloat32,
44100, paFramesPerBufferUnspecified,
playCallback, NULL);
// Start the stream, and handle the playback logic
Pa_Terminate();
return 0;
}
This example illustrates how to set up a playback stream with a callback function that will be triggered to fill the output buffer with audio data.
Recording Audio
Recording audio with PortAudio is also straightforward. Here’s how you can implement it:
static int recordCallback(const void* inputBuffer, void* outputBuffer,
unsigned long framesPerBuffer,
const PaStreamCallbackTimeInfo* timeInfo,
PaStreamCallbackFlags statusFlags) {
// Process the input buffer (e.g., save to file)
return paContinue;
}
int main() {
PaError err;
err = Pa_Initialize();
// Handle error
PaStream* stream;
err = Pa_OpenDefaultStream(&stream, 1, 0, paFloat32,
44100, paFramesPerBufferUnspecified,
recordCallback, NULL);
// Start the stream and handle recording logic
Pa_Terminate();
return 0;
}
In this snippet, the recordCallback function allows you to process the input audio data directly from the microphone.
Audio Effects and Manipulation
Applying effects to audio can enrich the user experience. Consider a simple echo effect. Here’s an illustrative example of processing audio data in a callback:
static int echoEffectCallback(const void* inputBuffer, void* outputBuffer,
unsigned long framesPerBuffer,
const PaStreamCallbackTimeInfo* timeInfo,
PaStreamCallbackFlags statusFlags) {
float* in = (float*)inputBuffer;
float* out = (float*)outputBuffer;
// Apply echo effect here
for (unsigned long i = 0; i < framesPerBuffer; i++) {
out[i] = in[i] * 0.5; // Simple echo effect (adjust to taste)
}
return paContinue;
}
This example demonstrates how to manipulate the audio data in real-time.
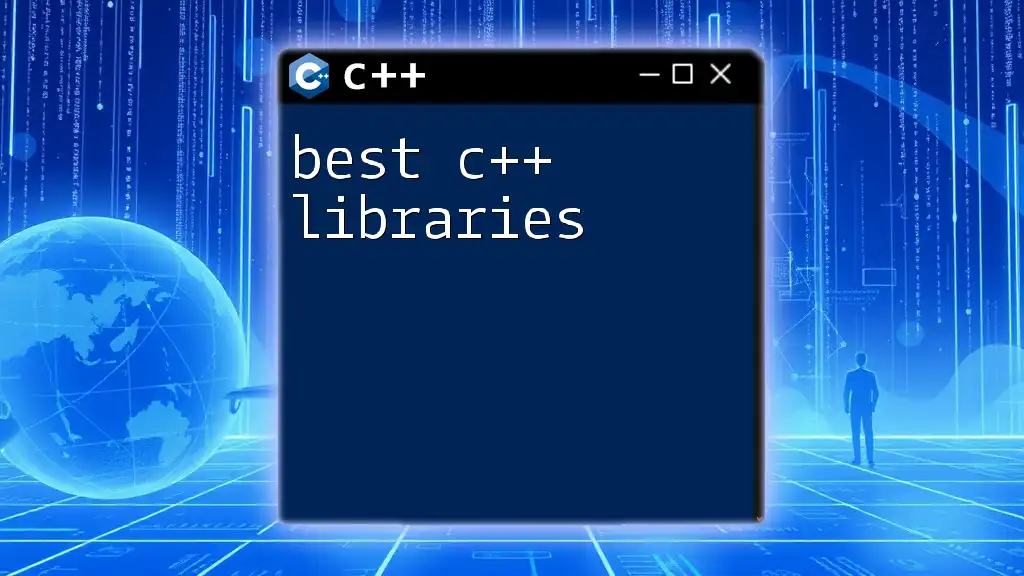
Advanced Features of C++ Audio Libraries
MIDI Support
MIDI (Musical Instrument Digital Interface) is essential for music applications. An audio C++ library often provides MIDI functionality, allowing developers to send or receive MIDI messages. Here’s a basic setup:
// Pseudocode for handling MIDI
void midiCallback(/* MIDI parameters */) {
// Process MIDI messages
}
Using the library’s MIDI functions, you can set up a listener that invokes `midiCallback` when messages are received.
Streaming Audio
Audio streaming is critical for applications that require real-time audio playback. Here is an example demonstrating how to implement audio streaming:
// Pseudocode for streaming audio
while (streaming) {
// Read data from a source
// Write data to the audio output
}
Real-time processing requires careful management of audio buffers to avoid dropouts.
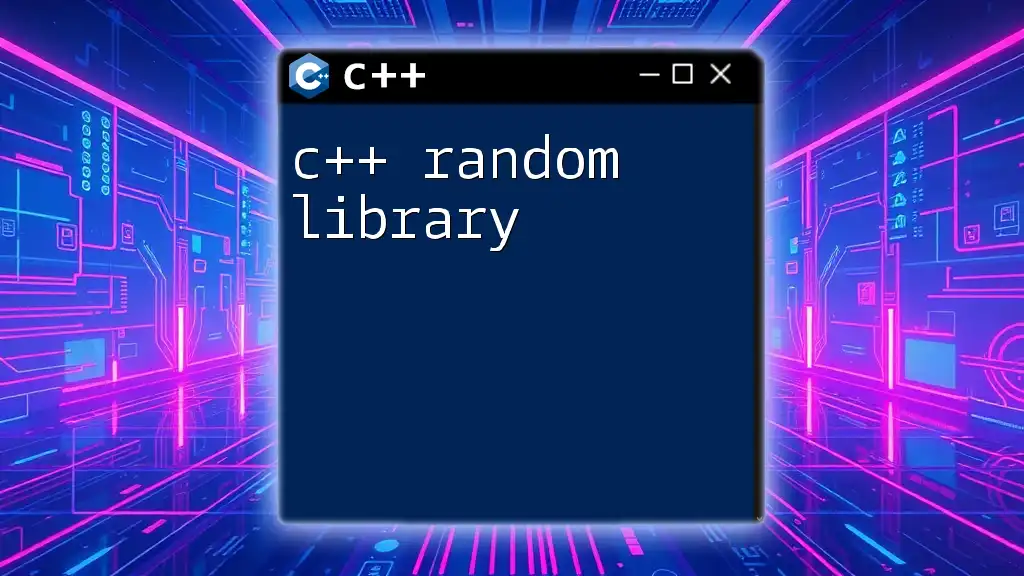
Best Practices in C++ Audio Programming
Memory Management
Efficient memory management is crucial in audio programming. Consider these tips:
- Avoid memory leaks: Use smart pointers or ensure proper cleanup of resources.
- Minimize allocations: Allocate as much as possible at the beginning to avoid runtime overhead.
Handling Errors and Debugging
Common pitfalls in audio programming include:
- Ignoring errors from audio library calls. Always check return values.
- Creating too many audio instances can lead to performance degradation.
Utilize logging and debugging tools to track issues effectively. Consider using frameworks like `gdb` or integrated debugging tools in IDEs.
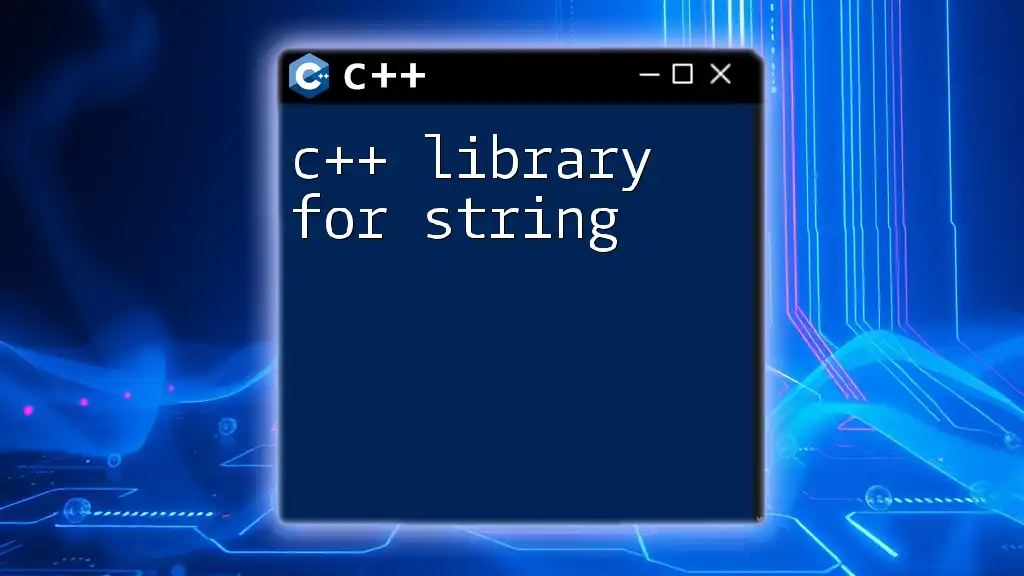
Case Studies: Real-World Applications
Gaming Applications
Audio libraries power sound engines in games, providing immersive experiences through background music, sound effects, and real-time audio feedback. A game might employ PortAudio to manage various audio channels simultaneously.
Music Software
For music production applications, audio libraries can handle multi-track playback and effects processing, enabling composers and producers to work seamlessly.
Voice Assistants
Voice recognition systems leverage audio libraries for capturing and processing audio input. Using C++, these systems can handle complex audio signals efficiently.
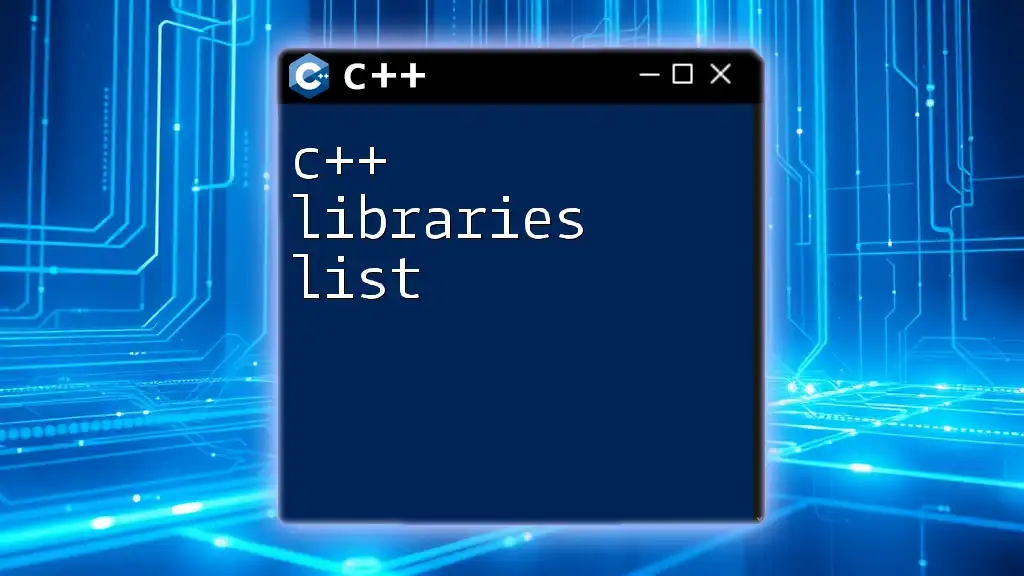
Conclusion
In summary, audio C++ libraries serve as invaluable tools in the development of audio-rich applications. By understanding their functionalities and integrating them into your projects, you can create engaging and sophisticated audio experiences. Exploring the libraries covered in this guide can lead to a deeper comprehension and innovative applications in C++ programming.
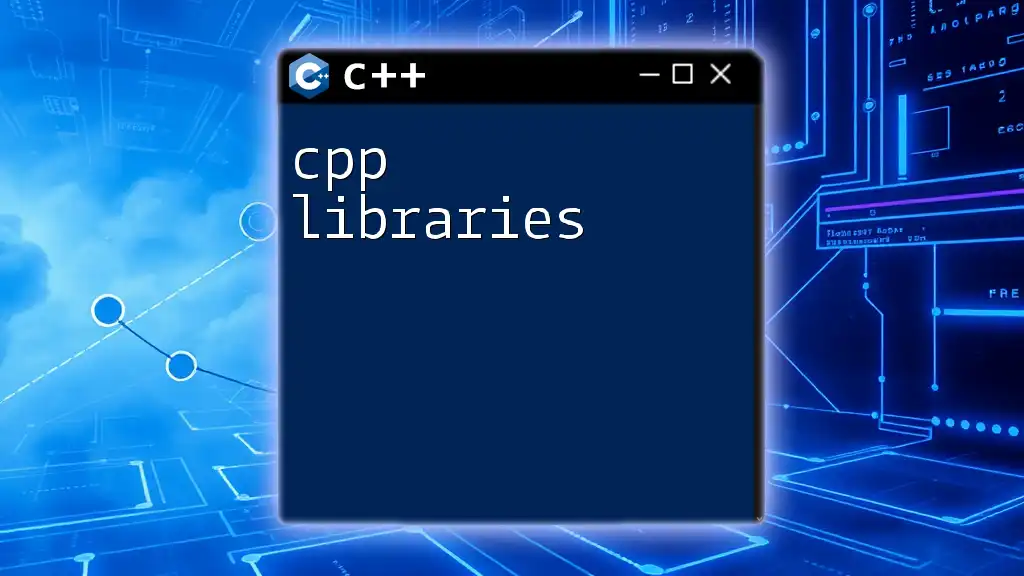
Further Learning Resources
To further enhance your knowledge, consider exploring recommended books, online tutorials, and forums dedicated to C++ programming and audio processing. Engage in communities where you can share insights and gain feedback on your projects related to the audio C++ library.
This framework sets the stage for developing exciting applications in audio programming, and I encourage you to experiment and innovate as you dive deeper into these concepts!