The iostream library in C++ provides functionalities for input and output operations, allowing you to read from standard input and write to standard output using streams.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Outputs "Hello, World!" to the console
return 0;
}
What is the iostream Library?
The iostream library in C++ is a powerful component that facilitates input and output operations through a consistent and efficient framework. This library enables data to flow between the program and the standard input/output devices, such as the keyboard and screen.
The significance of the iostream library lies in its ability to manage data streams seamlessly. It allows programmers to interact with users, display output on the console, and read input data effortlessly.
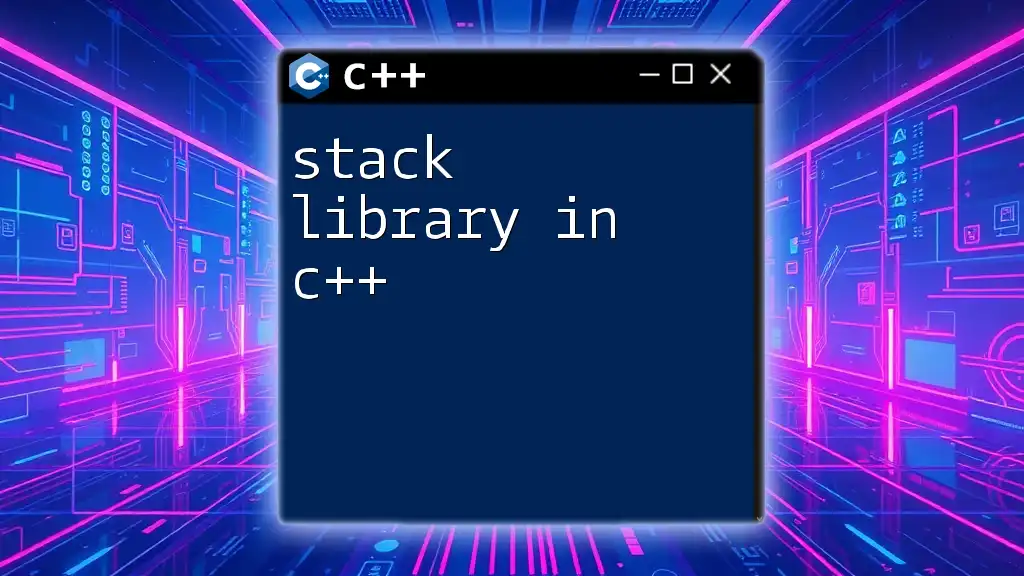
Components of the iostream Library
Standard Input and Output Streams
cin: Handling Console Input
The `cin` object is used for reading input from the standard input stream, which is typically the keyboard. It utilizes the stream extraction operator (`>>`) to retrieve data from the user.
For example, the following snippet illustrates how to read an integer value from user input and display it back:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
In this example, when the user types a number and presses Enter, `cin` captures it and stores it in the variable `number`, which is then printed to the console using `cout`.
cout: Handling Console Output
The `cout` object is used to display output to the standard output stream, typically the console. It employs the stream insertion operator (`<<`) to send data to the output.
This example demonstrates basic usage of `cout`:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
cout << "Number: " << 42 << ", Float: " << 3.14 << endl;
return 0;
}
Here, multiline strings, an integer, and a float are sent to the console, showcasing the versatility of `cout`. The `endl` manipulator is used to insert a newline character and flush the output buffer.
Other Important Streams
cerr: Writing Error Messages
The `cerr` object is specifically designed for outputting error messages. It is unbuffered, meaning it displays messages immediately, which is beneficial for debugging.
For instance, consider this simple error output example:
#include <iostream>
using namespace std;
int main() {
cerr << "An error occurred!" << endl;
return 1;
}
When this code is executed, it prints an error message directly to the console, allowing for immediate visibility of errors.
clog: Logging Information
Unlike `cerr`, `clog` is buffered, making it suitable for logging purposes that don’t require immediate feedback. It allows for easier management of output over time.
Here’s how to use `clog`:
#include <iostream>
using namespace std;
int main() {
clog << "This is a log message." << endl;
return 0;
}
Using `clog` helps in maintaining a record of events that occur during program execution without overwhelming the user with constant output.
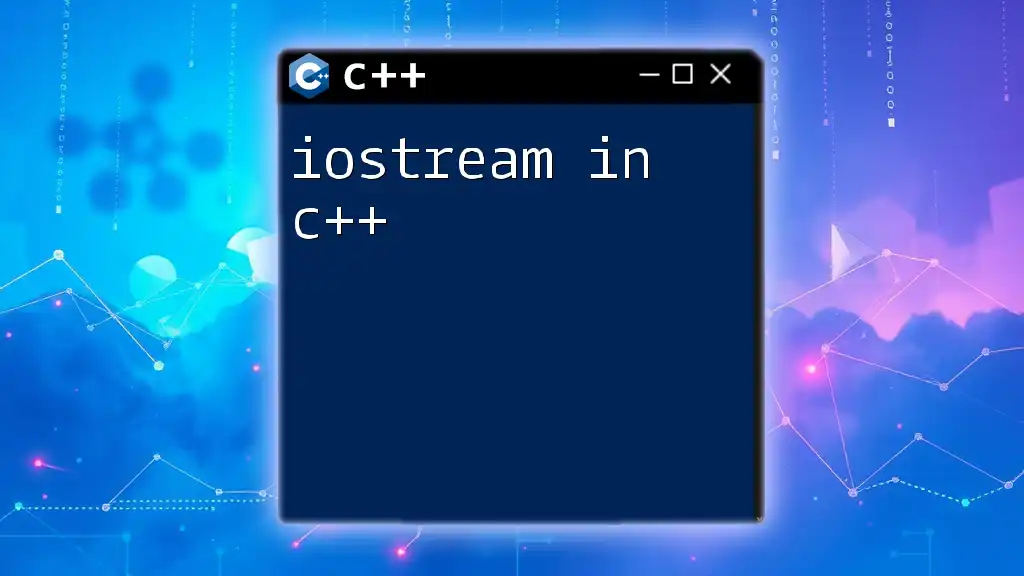
Working with the iostream Library
Input/Output Operators
Stream Insertion Operator (<<)
The stream insertion operator (`<<`) is employed with `cout` to send output. This operator can be chained to include multiple pieces of information within a single output statement.
cout << "My age is " << 25 << " and I live in " << "New York." << endl;
Stream Extraction Operator (>>)
Conversely, the stream extraction operator (`>>`) is utilized with `cin` to retrieve user input. It can also be chained, permitting multiple inputs in one line.
int a, b;
cout << "Enter two numbers: ";
cin >> a >> b;
Manipulating Output
C++ offers several manipulators that can modify the behavior and formatting of output.
- endl: Inserts a newline and flushes the output buffer.
- setw: Sets the width of the output field.
- setprecision: Controls the number of digits displayed for floating-point numbers.
For example, using `setw` for formatted output:
#include <iostream>
#include <iomanip>
using namespace std;
int main() {
cout << setw(10) << "Name" << setw(5) << "Age" << endl;
cout << setw(10) << "Alice" << setw(5) << 30 << endl;
return 0;
}
In this snippet, `setw` aligns the output into formatted columns, making the information visually clear and organized.
Error Handling with Streams
While reading input or producing output, various issues can arise. Understanding how to handle these errors is crucial.
You can check for input errors using the `fail()` function, which determines whether the last operation was successful.
Here's a practical example:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
while (!(cin >> number)) {
cin.clear(); // clear the error flag
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // ignore incorrect input
cout << "Invalid input. Please enter an integer: ";
}
cout << "You entered: " << number << endl;
return 0;
}
This loop continues until a valid integer is entered, helping to maintain program stability and providing a better user experience.
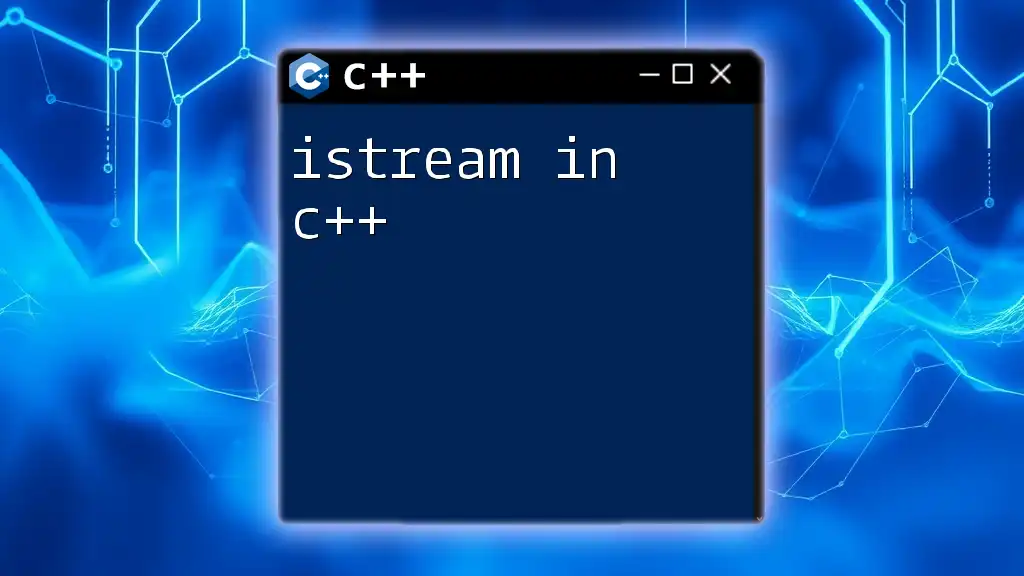
Best Practices for Using the iostream Library
When utilizing the iostream library in C++, consider the following best practices:
- Always use the `std::` prefix to avoid ambiguity, or include a `using namespace std;` statement to prevent potential naming conflicts.
- For large-scale applications, favor the use of `cerr` and `clog` for error reporting and logging rather than cluttering the primary program output with error messages.
- Optimize console I/O by minimizing the number of operations and using manipulators selectively to enhance performance.
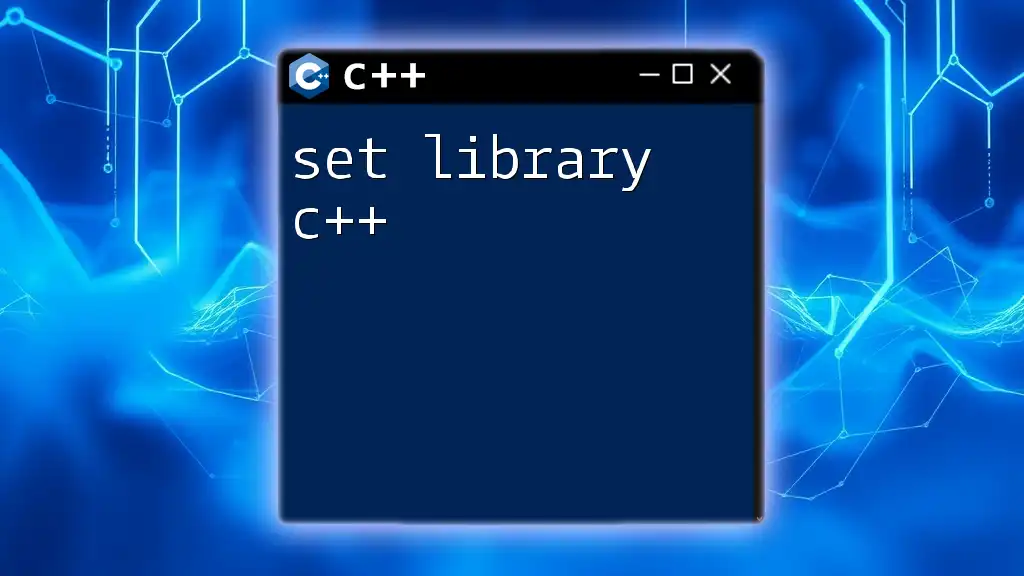
Conclusion
In summary, mastering the iostream library in C++ is foundational for effective programming. Its input/output management capabilities are pivotal for interacting with users and handling data efficiently. By practicing the techniques discussed and utilizing the examples provided, you’ll be well-equipped to implement effective I/O operations in your C++ programs.
For further exploration, consider diving deeper into additional resources and documentation on the iostream library to expand your knowledge and skills in C++.