In C++, the `unordered_map` allows you to store key-value pairs and the `insert` method is used to add elements to the map efficiently; here's how you can do it:
#include <unordered_map>
#include <iostream>
int main() {
std::unordered_map<std::string, int> myMap;
myMap.insert({"apple", 1});
myMap.insert(std::make_pair("banana", 2));
std::cout << "apple: " << myMap["apple"] << ", banana: " << myMap["banana"] << std::endl;
return 0;
}
Understanding `unordered_map`
What is `unordered_map`?
`unordered_map` is part of the C++ Standard Library, defined in the header `<unordered_map>`. It is a container that stores elements in key-value pairs and allows for fast retrieval based on keys. The key distinction of `unordered_map` is that it does not maintain the order of its elements; instead, it uses a hash table implementation.
The primary benefit of using `unordered_map` is its average-case constant time complexity for insertion, deletion, and access operations, making it highly efficient for operations where quick lookups are desired.
Use Cases for `unordered_map`
`unordered_map` is especially useful when:
- The order of elements is not crucial: If you do not need to access elements in a specific sequence, `unordered_map` is the ideal choice.
- Performance is a priority: In situations requiring rapid access to large datasets (e.g., caching, counting occurrences, index-based lookups), the efficiency of `unordered_map` becomes invaluable.
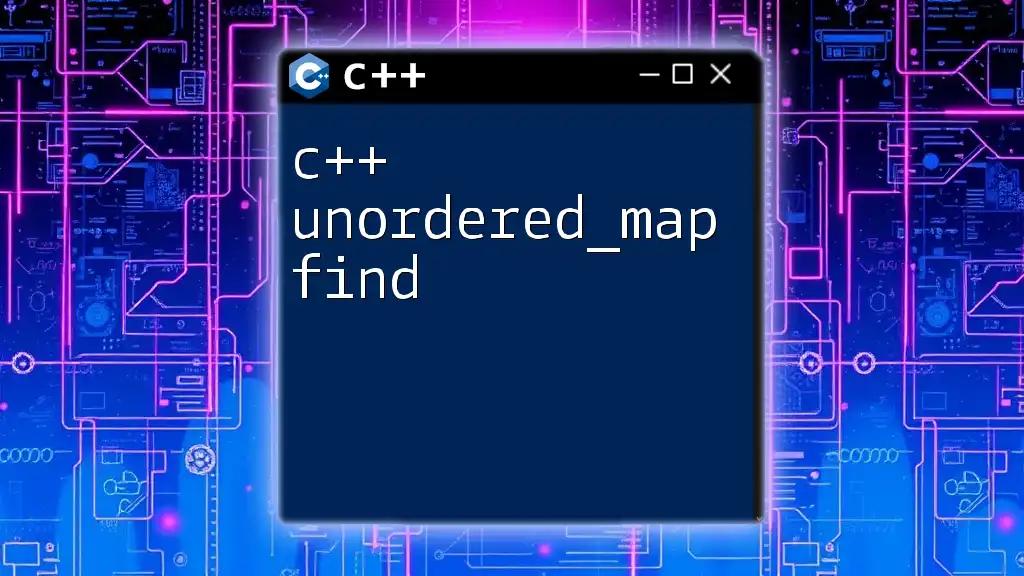
Basic Structure of `unordered_map`
Key Characteristics
An `unordered_map` is built on the principle of hashing. Each element is stored as a key-value pair, and the actual storage is handled in buckets based on the hash of keys. The time complexities for different operations are as follows:
- Insertion: O(1) average case, O(n) worst case due to rehashing.
- Deletion: O(1) average case, O(n) worst case.
- Access: O(1) average case, O(n) worst case.
Syntax of `unordered_map`
Creating an `unordered_map` involves specifying the types for keys and values:
#include <unordered_map>
std::unordered_map<KeyType, ValueType> myMap;
Here, replace `KeyType` and `ValueType` with the appropriate types for your use case (e.g., `int` for keys and `std::string` for values).

Inserting Elements into an `unordered_map`
Using `insert()` Function
One of the foundational methods for inserting into an `unordered_map` is through the `insert()` function.
Syntax
std::pair<std::unordered_map<KeyType, ValueType>::iterator, bool> insert(const value_type& val);
This method returns a pair consisting of an iterator to the element and a boolean value indicating success or failure of the insertion.
Example
std::unordered_map<int, std::string> myMap;
myMap.insert({1, "One"});
In this example, the key `1` has the associated value `"One"`. If the key was already present, the `insert()` function would not add a duplicate, and the boolean returned would be `false`.
Using `operator[]`
The `operator[]` can also be used to insert elements, serving as a shorthand for adding or updating a key-value pair.
Syntax
ValueType& operator[](const KeyType& key);
Example
myMap[2] = "Two";
In the above case, if the key `2` does not exist, it is created with the value `"Two"`. If it does exist, its value will be updated. This behavior can lead to unintended entries, so one must be cautious when using `operator[]` if wanting to avoid overwriting existing keys.
Using `emplace()`
`emplace()` is another method available for handling insertions. Unlike `insert()`, which takes an existing object to construct elements, `emplace()` constructs elements in-place.
Syntax
template< class... Args >
std::pair<iterator,bool> emplace( Args&&... args );
Example
myMap.emplace(3, "Three");
Using `emplace()`, the key `3` is inserted with the value `"Three"`. It can efficiently avoid unnecessary copies or moves, which is beneficial for performance when dealing with large objects.
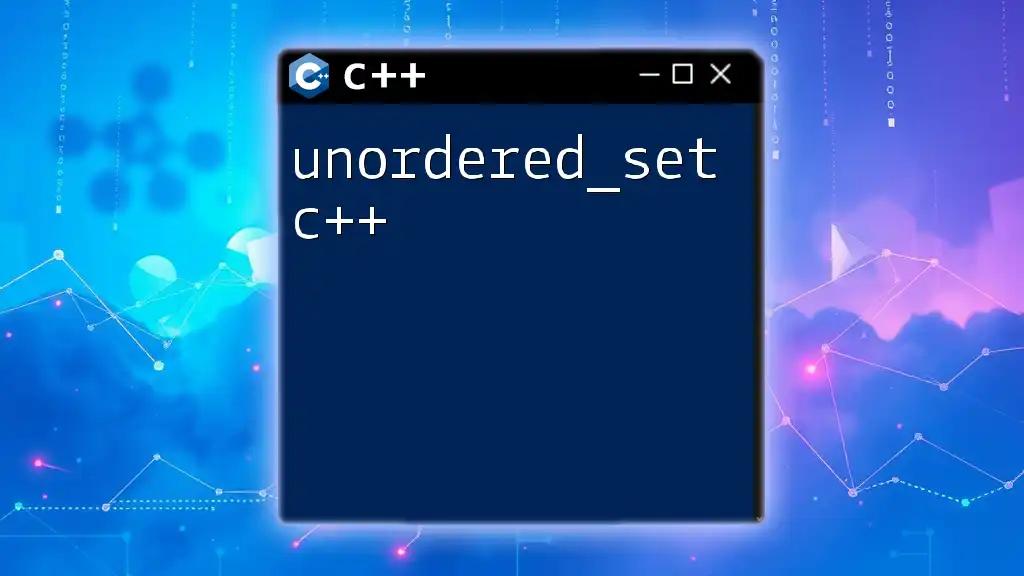
Handling Duplicate Keys
Behavior of `insert()`
When you attempt to insert a key that already exists in the map, `insert()` will not add a new entry. Instead, the function returns an iterator to the existing element and `false` to denote that the insertion was unsuccessful.
Overwriting Values with `operator[]`
If you want to overwrite the value of an existing key using `operator[]`, you can simply do so without any special handling. For example:
myMap[1] = "Changed One";
Here, if the key `1` already existed, its value will be updated without generating an error.

Best Practices for Using `unordered_map` Insertion
Avoiding Performance Pitfalls
To ensure consistent performance with `unordered_map`, especially concerning hash collisions:
- Choose appropriate hash functions: If you are using custom key types, implement hash functions that minimize collisions.
- Understand load factors and resizing: An `unordered_map` will resize itself (rehash) once the load factor exceeds a certain threshold. This means that your `unordered_map` may temporarily degrade in performance, so planning the reserve size before insertion can be beneficial.
Memory Management
C++ manages the memory allocation for `unordered_map`, but it's important to understand that every insertion could increase the memory footprint. Be aware of how much data you are working with and consider reserving space ahead of time to optimize performance:
myMap.reserve(100); // Pre-allocate for 100 elements

Real-World Applications
Case Study: Counting Frequency of Elements
A practical use case for `unordered_map` is counting the frequency of occurrences of elements in a dataset. Here's a simple implementation:
std::vector<std::string> words = { "apple", "banana", "apple", "orange", "banana", "banana" };
std::unordered_map<std::string, int> frequencyMap;
for (const auto& word : words) {
frequencyMap[word]++;
}
In this example, each word's frequency is tracked efficiently using simple insertions, showcasing how `unordered_map` can effectively handle data dynamically.
Another Case: Caching with `unordered_map`
`unordered_map` also excels in caching results of computations, which can drastically improve performance. For instance, if you have a function that performs an expensive computation, you can cache its results like this:
std::unordered_map<int, int> cache;
auto expensiveFunction(int input) {
auto it = cache.find(input);
if (it != cache.end()) {
return it->second; // Return cached result
}
// Perform the expensive calculation
int result = /* computation */;
cache[input] = result; // Cache the result
return result;
}
This strategy ensures that repeated calls with the same input avoid unnecessary recomputation.
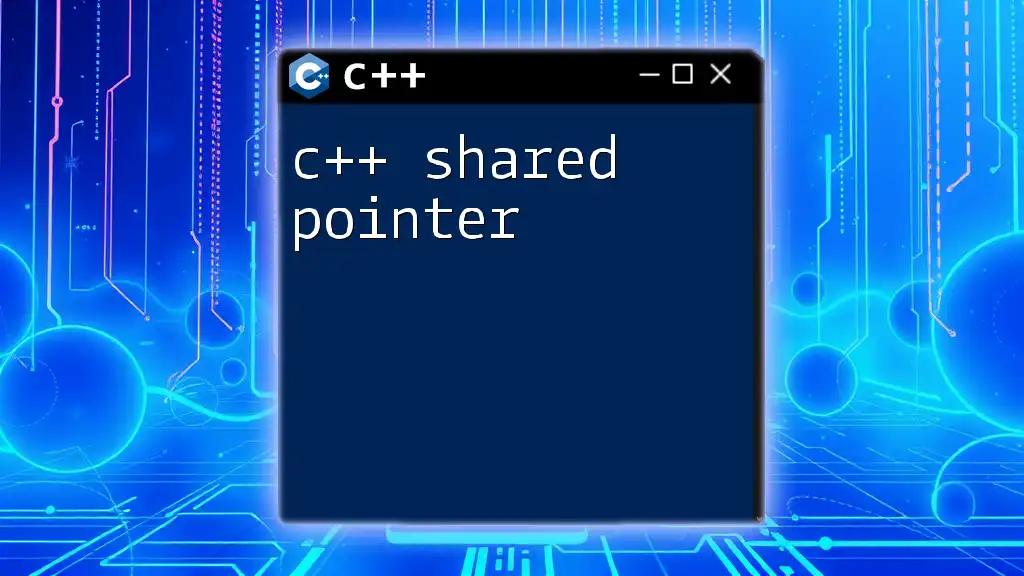
Conclusion
The `c++ unordered_map insert` functionality is pivotal for anyone looking to leverage efficient data handling in C++. Understanding the various methods for insertion, the implications of key duplication, and best practices for performance can empower developers to utilize C++ maps more effectively in their applications. By incorporating these techniques into your coding practices, you can ensure that your C++ applications run smoothly and efficiently.

Further Reading
For further understanding of `unordered_map`, consider exploring the official C++ documentation, as well as looking into other topics related to C++ containers such as `map` and `set`. These resources can deepen your knowledge and enhance your programming skill set.