In C++, an `unsigned short` is a data type that can store non-negative integer values typically ranging from 0 to 65,535, using 16 bits of memory.
unsigned short age = 25;
Understanding Data Types in C++
In C++, data types play a crucial role in how data is stored, manipulated, and processed. Integral types such as integers, characters, and booleans are commonly used. Among these, short int and unsigned short are specific types of integer data types that serve different purposes, particularly when it comes to value ranges and memory consumption.
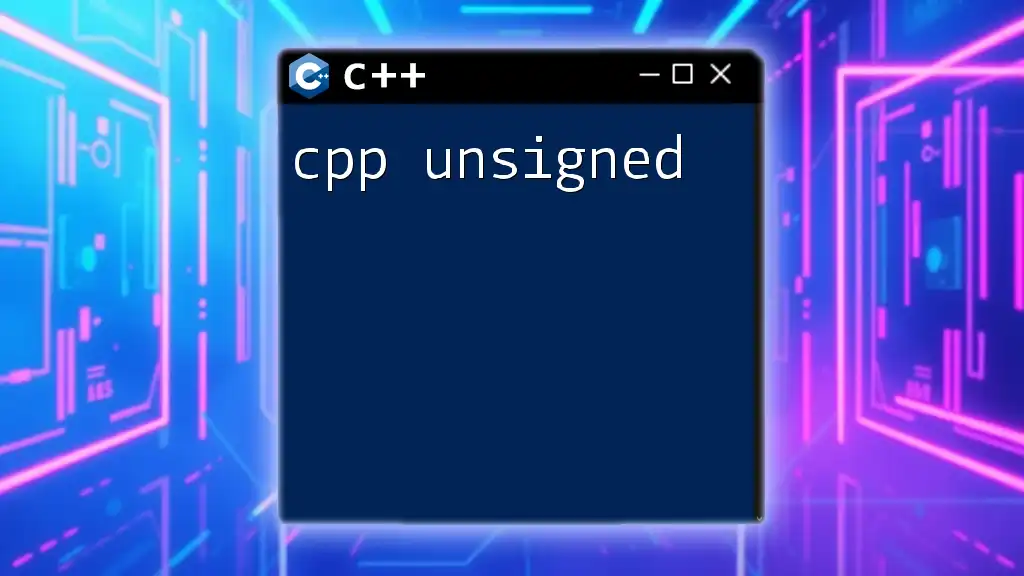
What is an Unsigned Short in C++?
An unsigned short is a data type in C++ that is used to store non-negative integer values. Unlike the standard `short int`, which can hold both positive and negative values, the unsigned short restricts its range to only non-negative integers.
The key difference lies in the value range:
- Short int has a range typically from -32,768 to +32,767 (for a 16-bit system).
- Unsigned short expands this range from 0 to 65,535 because it does not account for negative numbers.
Using an unsigned short is beneficial when you know that the variable will never need to represent negative values, such as when counting objects, or dealing with colors in graphics programming.
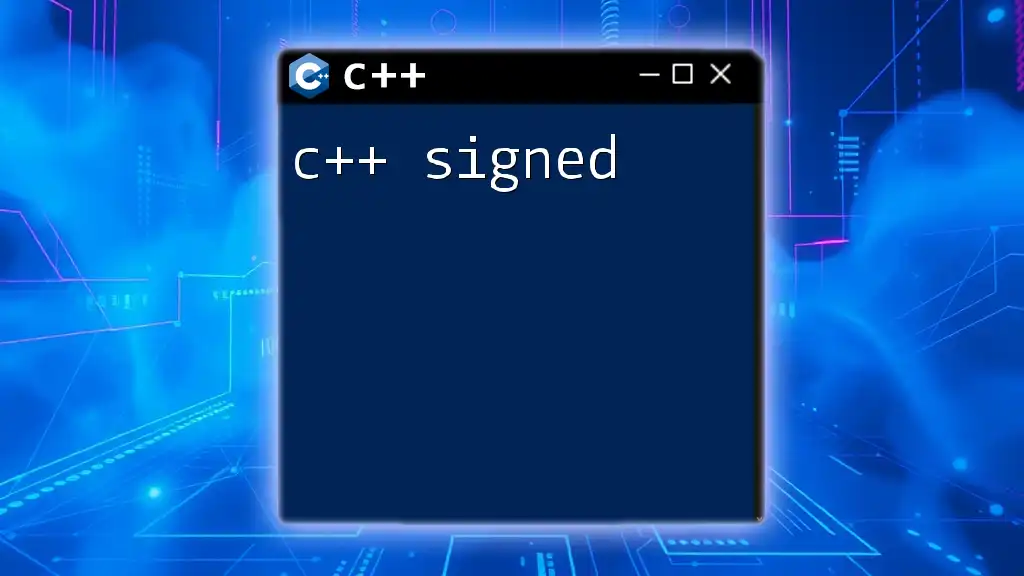
Characteristics of Unsigned Short
Memory Allocation
In C++, the unsigned short data type typically occupies 2 bytes (16 bits) of memory. This allows for efficient memory usage, especially in scenarios that involve extensive arrays or objects where space is a constraint.
Value Range
The range of an unsigned short is from 0 to 65,535. This is derived from the formula:
Values = 2^(n) where n is the number of bits.
For an unsigned short (16 bits), this gives us:
- Minimum: 0
- Maximum: 65,535
Performance Considerations
Using an unsigned short can offer performance benefits over other data types due to its smaller size. In performance-critical applications, especially in embedded systems or real-time graphics, choosing the appropriate data type may lead to faster processing and reduced memory load.
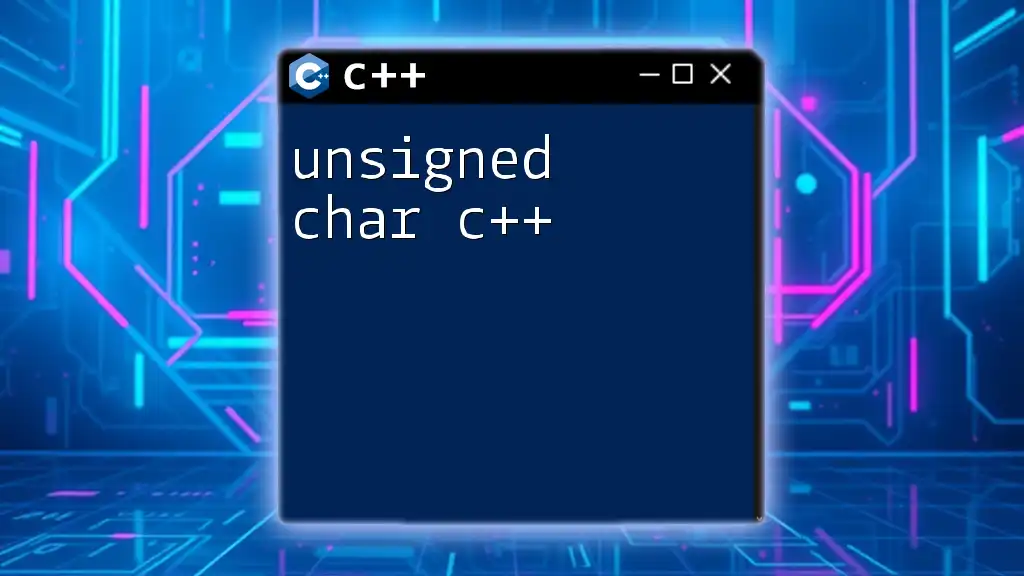
Declaring Unsigned Short Variables
To declare an unsigned short variable, you'll use the following syntax:
unsigned short myValue;
It's also crucial to initialize your unsigned short variables effectively. For instance:
unsigned short myValue = 300;
This assignment ensures that your variable starts with a known value, preventing uninitialized memory access issues.
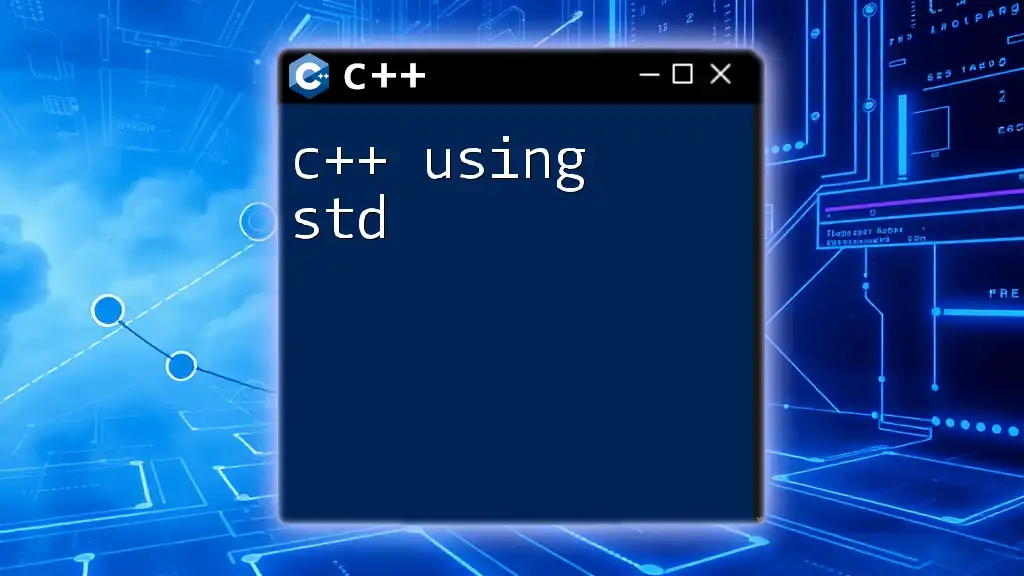
Operations with Unsigned Short
Arithmetic Operations
Unsigned shorts support basic arithmetic operations such as addition, subtraction, multiplication, and division.
Here’s an example demonstrating an addition:
unsigned short a = 500;
unsigned short b = 300;
unsigned short sum = a + b; // Result: 800
Comparison Operations
Comparison operators can be applied to unsigned shorts, just like any other numeric types. Consider the following example:
unsigned short a = 600;
unsigned short b = 400;
if (a > b) {
// code here to execute if condition is true
}
This functionality allows you to make decisions in your program based on comparisons of unsigned short values.
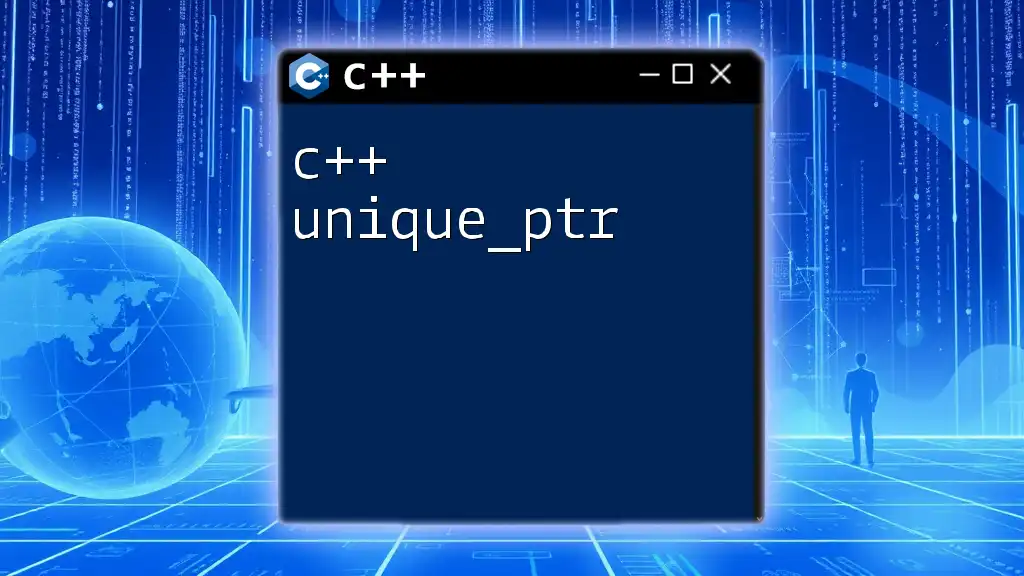
Conversions between Signed and Unsigned Short
In C++, conversions between signed and unsigned types can happen implicitly or explicitly. Awareness of these conversions is vital to avoid unexpected behaviors.
Consider this implicit conversion:
short signedNum = -1; // Implicit conversion to unsigned short
unsigned short unsignedNum = signedNum; // Results in large positive value
In this case, attempting to store a negative number in an unsigned short leads to an unexpected wrap-around to a large positive value, which can create logic errors in your program.
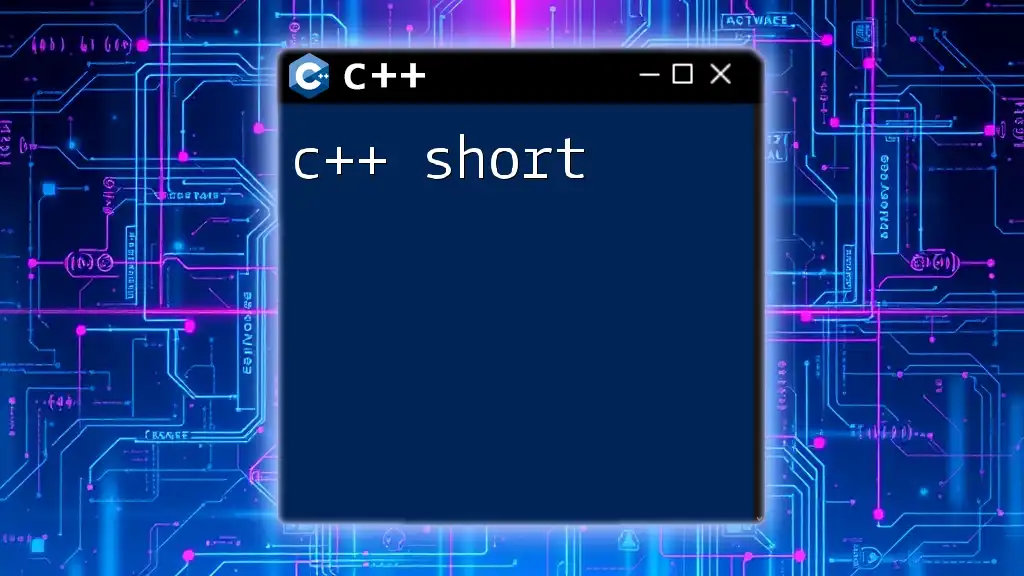
Common Errors with Unsigned Short
While unsigned short types are powerful, they can lead to common pitfalls. One major issue is overflow. For example:
unsigned short num = 65535; // Maximum value
num += 1; // Results in 0 due to overflow
This scenario demonstrates how exceeding the bounds of an unsigned short reverts the value back to zero due to overflow. Always ensure your calculations stay within the defined limits.
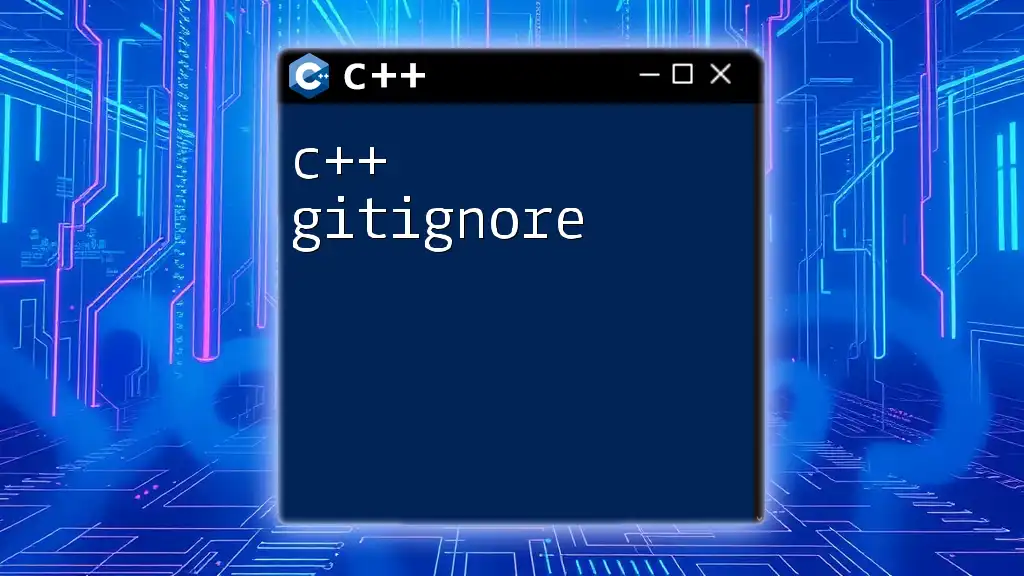
Practical Applications of Unsigned Short
There are numerous scenarios where an unsigned short is preferred. One prime example is within memory-constrained systems where every byte counts. For instance, when manipulating color values in graphics programming, using unsigned shorts allows you to represent color channels effectively without wasting memory.
Additionally, unsigned shorts are helpful in counting items, managing arrays, and limiting results where negatives are logically nonsensical.
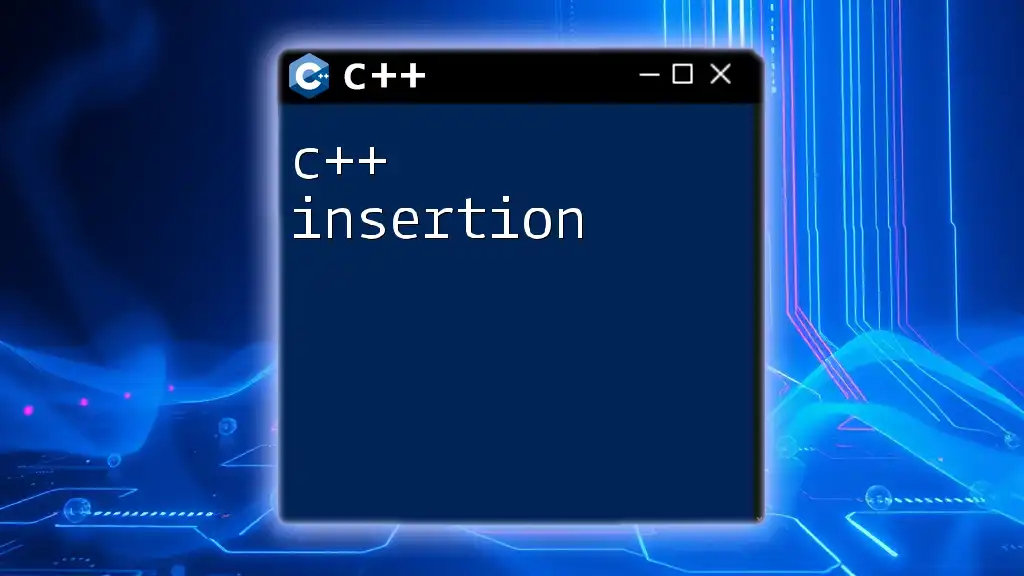
Best Practices for Using Unsigned Short in C++
When using an unsigned short, consider the following best practices:
- Use for Non-Negative Values: Only use this data type when you are certain that the value will never go negative.
- Check for Overflow: Always implement checks to avoid overflow scenarios.
- Use Caution with Conversions: Be vigilant when converting between signed and unsigned types to prevent unexpected behavior.
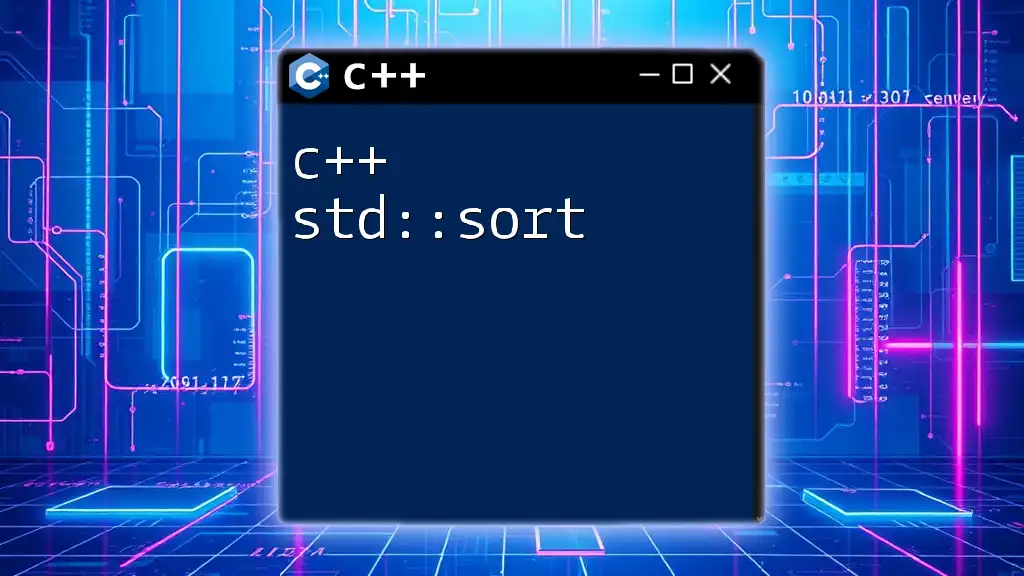
Conclusion
In this guide, we explored the C++ unsigned short data type, its characteristics, operations, common pitfalls, and practical applications. Understanding how to leverage the unsigned short effectively can enhance your programming toolbox, particularly in scenarios where memory efficiency and clear value constraints are essential.