In C++, `unsigned char` is a data type that represents a character or a small integer in the range of 0 to 255, allowing for the storage of larger positive values compared to a regular `char`.
Here's a code snippet demonstrating its usage:
#include <iostream>
int main() {
unsigned char myChar = 250;
std::cout << "Value of myChar: " << static_cast<int>(myChar) << std::endl;
return 0;
}
What is `unsigned char` in C++?
`unsigned char` is a fundamental data type in C++. It represents a single byte of data without sign, thus allowing it to store positive values only. While the standard `char` can hold values from -128 to 127 (if signed), or 0 to 255 (if unsigned), the `unsigned char` can exclusively handle the range of 0 to 255. This makes it particularly useful when you need to store raw binary data or when performance and memory usage are concerns.
![Understanding Unsigned Int in C++ [Quick Guide]](/_next/image?url=%2Fimages%2Fposts%2Fu%2Funsigned-int-cpp.webp&w=1080&q=75)
Use Cases for `unsigned char`
In various scenarios, using `unsigned char` can be significantly advantageous:
- Raw Data Representation: Often utilized in low-level programming where manipulation of individual bytes is necessary.
- Binary File Handling: When reading or writing binary files, `unsigned char` can accurately represent the byte values without interpreting them as characters.
- Graphics Programming: In applications involving pixel manipulation, such as image processing, each color channel, like Red-Green-Blue (RGB), can be efficiently stored as an `unsigned char`, enabling you to represent color values without overflow.
Applications in Data Compression
In data compression algorithms, `unsigned char` is frequently used to represent a collection of bits compactly, ensuring that the memory footprint is reduced while maintaining data integrity.
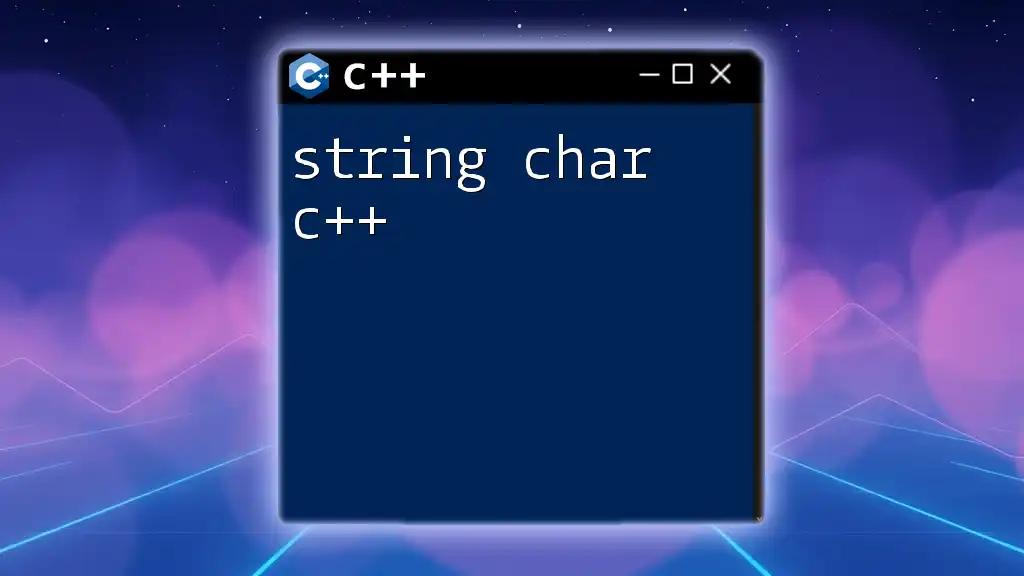
Characteristics of `unsigned char`
Range and Memory
The `unsigned char` data type can hold values from 0 to 255, giving it a total of 256 unique values. Just like `char`, it occupies 1 byte (8 bits) of memory, making it a memory-efficient choice for various applications involving sets of data that can only be positive.
Comparison Table
Data Type | Signed Range | Unsigned Range | Size |
---|---|---|---|
`char` | -128 to 127 | 0 to 255 | 1 Byte |
`signed char` | -128 to 127 | N/A | 1 Byte |
`unsigned char` | N/A | 0 to 255 | 1 Byte |
Code Example: Basic Declaration and Initialization
unsigned char myChar = 200;
In this example, we declare an `unsigned char` variable named `myChar` and initialize it with the value 200. This action is straightforward and safe, given that 200 is within the valid range for `unsigned char`.
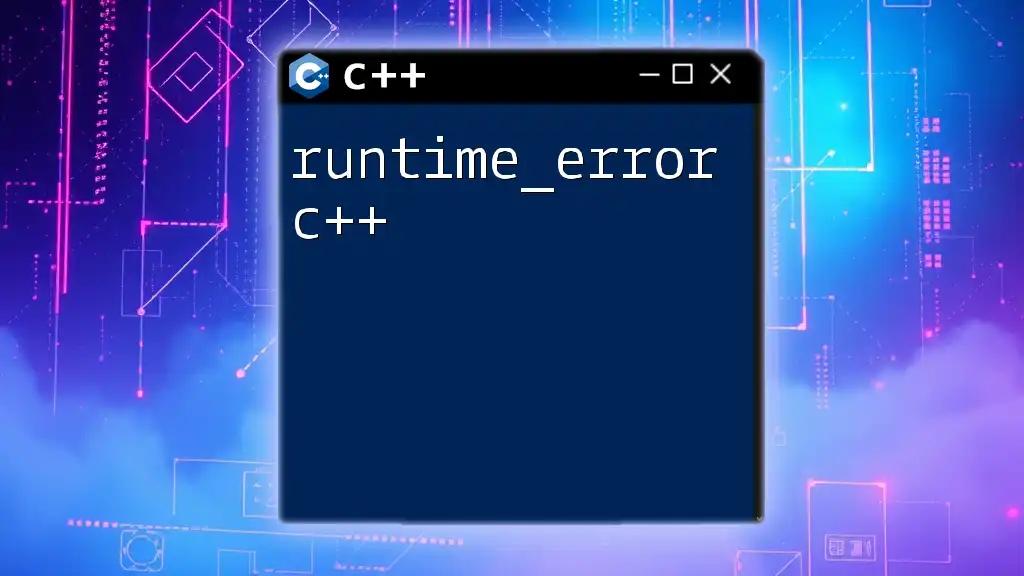
Operations with `unsigned char`
You can perform a variety of operations on `unsigned char`, including arithmetic and bitwise operations. However, caution is required, particularly due to the potential for overflow when performing arithmetic operations.
Arithmetic Operations
Arithmetic operations with `unsigned char` can lead to interesting scenarios:
#include <iostream>
int main() {
unsigned char a = 200;
unsigned char b = 100;
unsigned char sum = a + b; // May cause an overflow
std::cout << "Sum: " << (int)sum << std::endl; // Cast to int for display
return 0;
}
In this snippet, adding `a` and `b` would logically result in 300. However, since 300 exceeds the maximum range for `unsigned char`, the result wraps around to 44. Casting to `int` provides a clear output of the intended value.
Bitwise Operations
`unsigned char` also supports bitwise operations, such as AND, OR, NOT, etc. Here’s an example:
unsigned char x = 5; // Binary: 0101
unsigned char y = 3; // Binary: 0011
unsigned char result = x & y; // Bitwise AND
std::cout << "Result of AND: " << (int)result << std::endl; // Output will be 1 (0001)

Common Pitfalls and Best Practices
While using `unsigned char`, several pitfalls can arise, especially concerning implicit type conversions. When mixing `unsigned char` with other numeric types, always be aware of potential unintended consequences.
Implicit Conversion Pitfall
unsigned char data = 260; // Implicit conversion may lead to unexpected results
std::cout << "Data: " << (int)data << std::endl; // Output may not be 260
In the above example, 260 cannot be represented within the range of `unsigned char`, which results in an implicit conversion that might lead to confusion. Always ensure values assigned to `unsigned char` are within the appropriate range.

Performance Considerations
When optimizing for performance, `unsigned char` can yield benefits in specific contexts. Its ability to store positive integers with a reduced memory footprint is essential in scenarios where memory is constrained.
Memory vs. Speed Trade-offs
If you're structuring large datasets, choosing `unsigned char` can provide better overall performance by minimizing memory usage, which can lead to reduced cache misses. Benchmark your applications to determine the best data type for your specific use case.

Conclusion
Understanding the significance of `unsigned char` in C++ is crucial, especially for applications involving low-level data manipulation, graphics, and performance-critical software. The use of this data type allows developers to operate efficiently within the constraints of C++ while maximizing performance and ensuring data integrity. Experimentation with the provided code examples will further enhance your practical knowledge and proficiency in using `unsigned char`.

Further Reading
For those looking to deepen their understanding of data types and their applications in C++, exploring the official C++ documentation is highly recommended. There are also many great books and online resources that provide comprehensive coverage of data handling in C++.
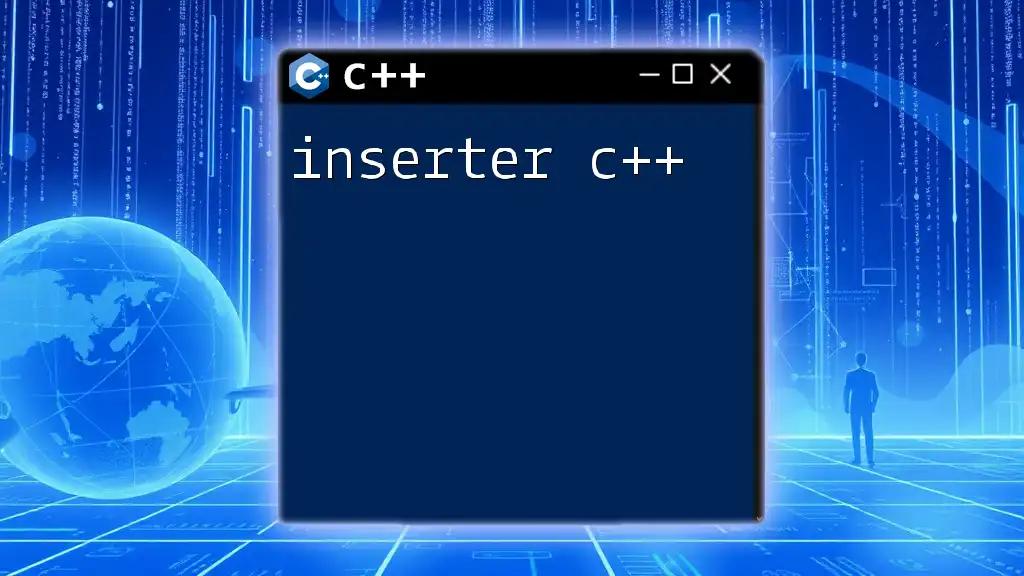
FAQs
-
What is the difference between `char`, `signed char`, and `unsigned char`?
`char` can be implemented as either signed or unsigned depending on the compiler. `signed char` holds negative values, while `unsigned char` is strictly non-negative. -
Can `unsigned char` hold negative values?
No, `unsigned char` can only represent values in the range 0 to 255. -
How to convert `unsigned char` to other numeric types?
Direct assignment works for types within the valid range, but when using larger types, such as `int`, a cast may be necessary to prevent overflow issues.