In C++, a `long unsigned int` is a data type that represents a non-negative integer with a larger range than a standard `unsigned int`, allowing for larger values without the risk of negative numbers.
#include <iostream>
int main() {
long unsigned int largeNumber = 4000000000; // Example of a long unsigned int
std::cout << "The large unsigned number is: " << largeNumber << std::endl;
return 0;
}
What is an Unsigned Long Int in C++?
Definition of Unsigned Long Int
A long unsigned int in C++ is an integer type that can hold only non-negative whole numbers. The term "unsigned" indicates that it cannot represent negative values, while "long" denotes its larger size compared to a regular unsigned int. The exact size of a long unsigned int may vary depending on the system, but it is commonly 64 bits on modern platforms, allowing it to store values ranging from 0 to 4,294,967,295 (2^32 - 1) or more on certain systems that support 64-bit integers.
Characteristics of Unsigned Long Int
The unsigned long int type in C++ has several important characteristics:
-
Memory Allocation: It typically takes up 4 bytes on 32-bit systems and 8 bytes on 64-bit systems. This larger memory allocation allows for a greater range of positive values compared to signed integers.
-
Comparison with Signed Long Integers: While a signed long int can represent both positive and negative numbers, an unsigned long int doubles the positive range but eliminates the ability to represent negative values. For example, a signed long can range from -2,147,483,648 to 2,147,483,647 (on a 32-bit system), while an unsigned long goes from 0 to 4,294,967,295.
![Understanding Unsigned Int in C++ [Quick Guide]](/images/posts/u/unsigned-int-cpp.webp)
Advantages of Using Unsigned Long Int
Using a long unsigned int has several advantages:
-
Memory Efficiency: Since an unsigned long can hold a larger range of values without using negative space, it is particularly useful in scenarios where large numbers are essential, like indexing large arrays or handling file sizes.
-
Avoiding Negative Values: In situations where only positive numbers are necessary—such as counting items, representing sizes, or addressing memory locations—using an unsigned long int prevents the potential errors associated with negative values.
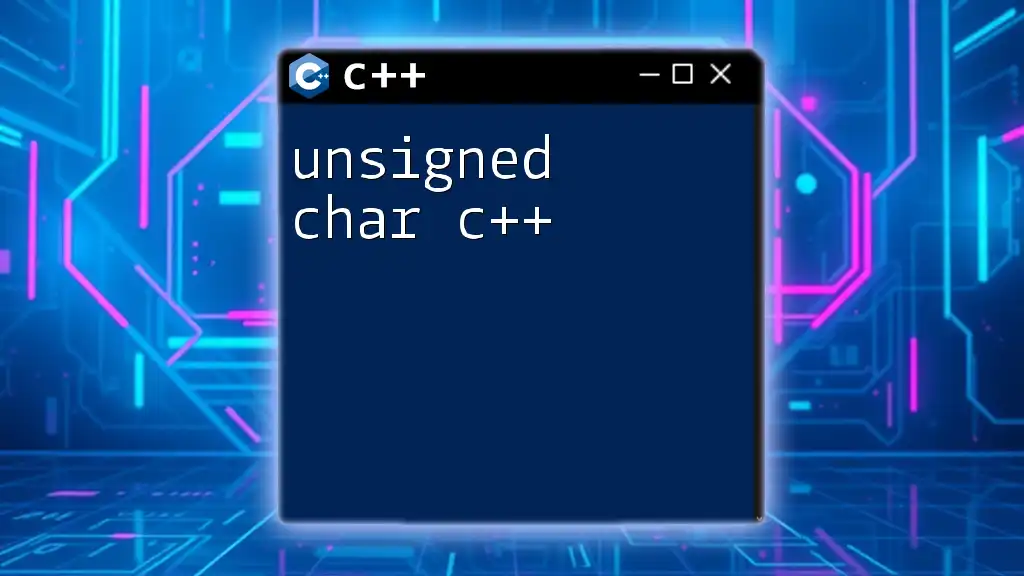
Declaring and Using Unsigned Long Int
Syntax for Declaration
To declare an unsigned long int, use the following syntax:
unsigned long myVariable;
Initialization Examples
You can initialize an unsigned long int in various ways:
- Simple Initialization:
unsigned long count = 1000;
- Initialization with Expressions:
Consider a scenario where you need to calculate a factorial:
unsigned long factorial = 1;
for (unsigned long i = 1; i <= 10; i++) {
factorial *= i; // Calculates 10! which is 3628800
}
This example illustrates how unsigned long can accommodate the rapid growth of factorial numbers without running into limits.
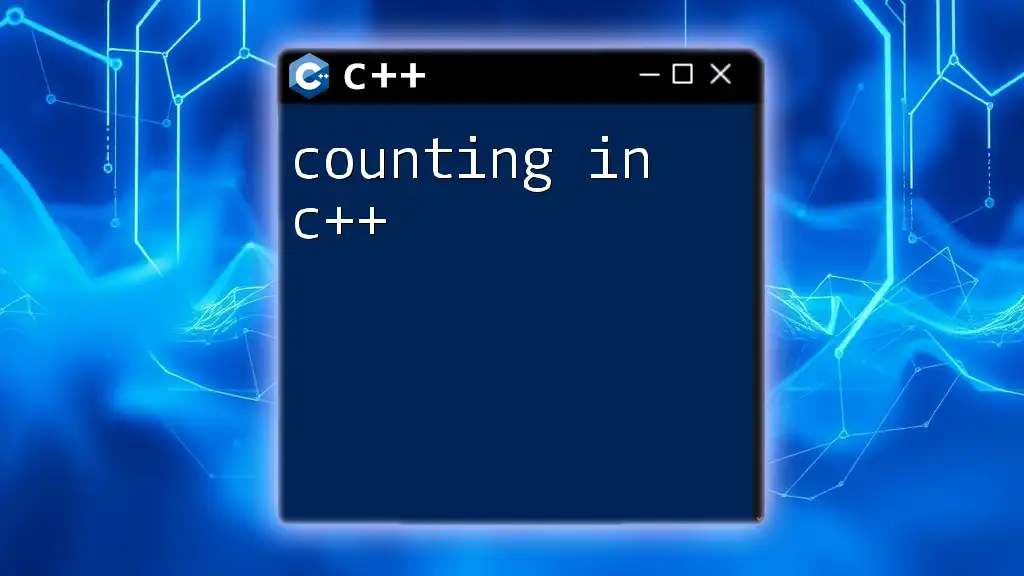
Common Operations with Unsigned Long Int
Arithmetic Operations
You can perform standard arithmetic operations with unsigned long int just as you would with traditional types. Here’s an example:
unsigned long a = 10;
unsigned long b = 20;
unsigned long sum = a + b; // sum now contains 30
Comparison Operations
Comparing unsigned long integers is straightforward. For example:
if (a < b) {
// Logic for when a is less than b
std::cout << "a is less than b" << std::endl;
}
This simple comparison is vital in many algorithms where the order of numbers matters.
Bitwise Operations
You can also perform various bitwise operations. For example:
unsigned long x = 5; // Binary: 101
unsigned long y = 3; // Binary: 011
unsigned long result = x & y; // Binary result: 001 (which is 1)
Bitwise operations can be useful in low-level programming and performance-critical situations.
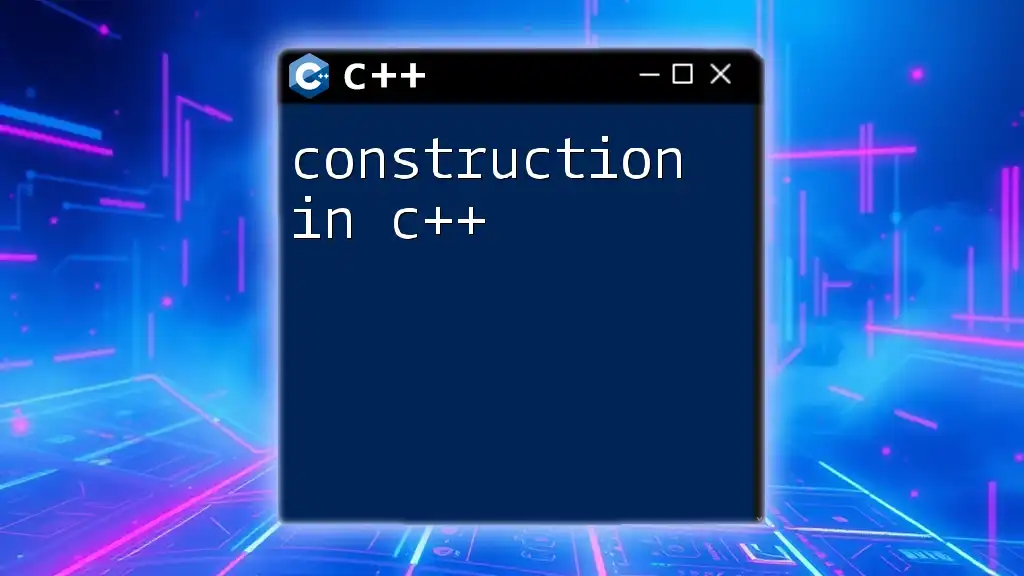
When to Use Unsigned Long Int
You should consider using a long unsigned int in scenarios requiring large positive numbers, such as:
- Finance Applications: Handling large transactions or quantities.
- Data Analysis: In statistical calculations where large integers are common.
- Graphics Programming: When working with pixel values and graphical elements, unsigned types can simplify calculations by ensuring non-negative values.
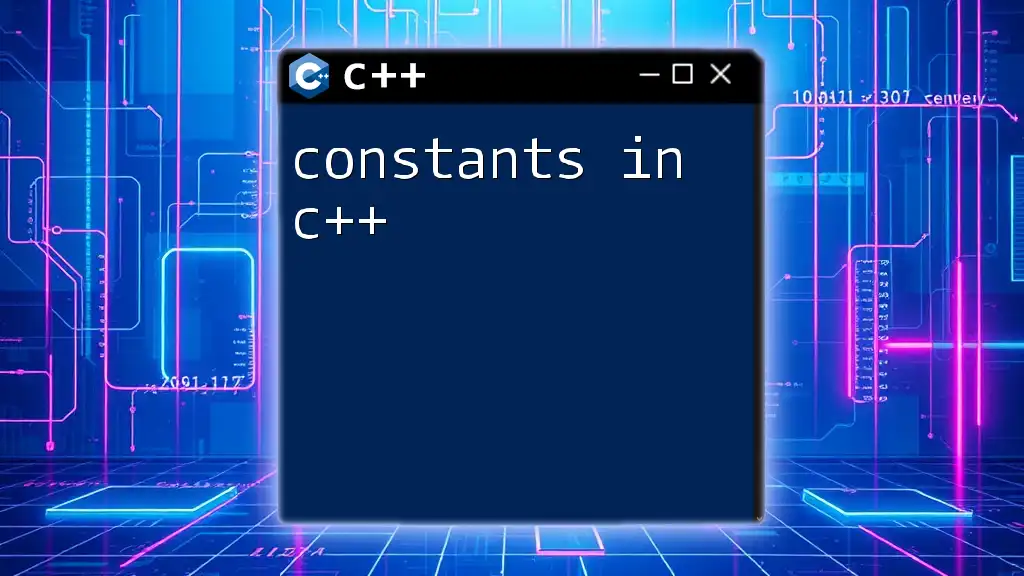
Overflow and Underflow in Unsigned Long Int
Understanding Overflow
An overflow occurs when a calculation exceeds the maximum limit of an unsigned long int. For instance:
unsigned long maxLimit = ULONG_MAX;
unsigned long overflow = maxLimit + 1; // Wraps around to 0
In this case, adding 1 to the maximum value results in wrapping around to 0. Such behavior can lead to unexpected outcomes, especially in algorithms where precise counts are essential.
Error Handling for Overflow
To prevent overflow, consider using error handling techniques, such as:
- Guard Clauses: Before performing calculations, check if adding to the variable will exceed the maximum value.
- Using Larger Data Types: For calculations that may exceed the range of unsigned long, consider using larger types like `__int128` or libraries designed for big integers.
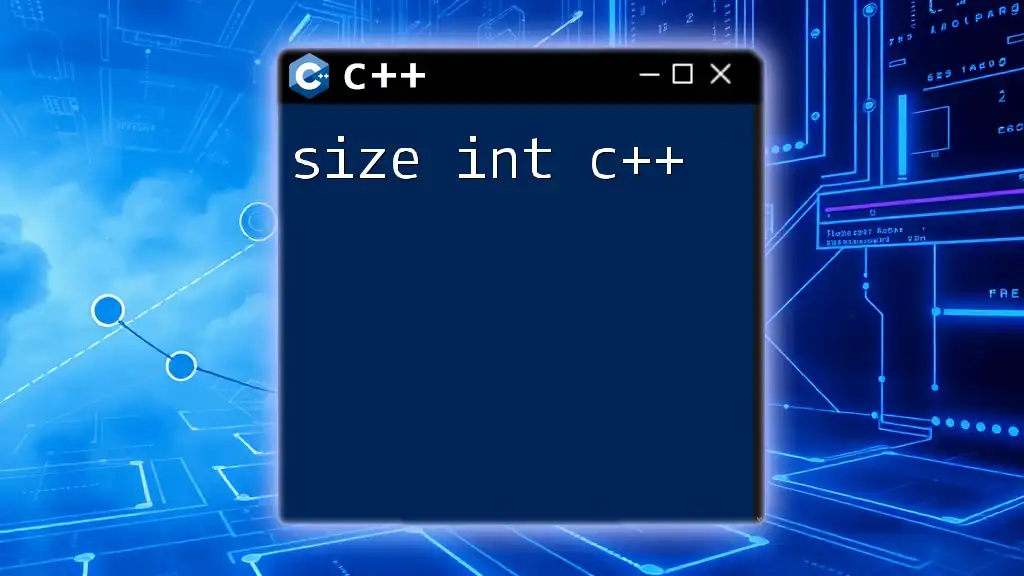
Differences Between Unsigned Long and Other Integer Types
Unsigned Long vs. Long
The key difference between long unsigned ints and regular long ints lies in how they handle negative values. While long ints can represent both signs, unsigned long ints eliminate the possibility of negative numbers, thereby increasing the upper limit of stored values.
Unsigned Long vs. Unsigned Int
Unsigned long allows storage of larger numbers compared to unsigned ints, but it comes with a trade-off in terms of memory size. An unsigned int typically takes 4 bytes and can represent values from 0 to 4,294,967,295 on 32-bit systems, while unsigned long may provide a greater upper bound, making it more suitable for high-capacity storage needs.
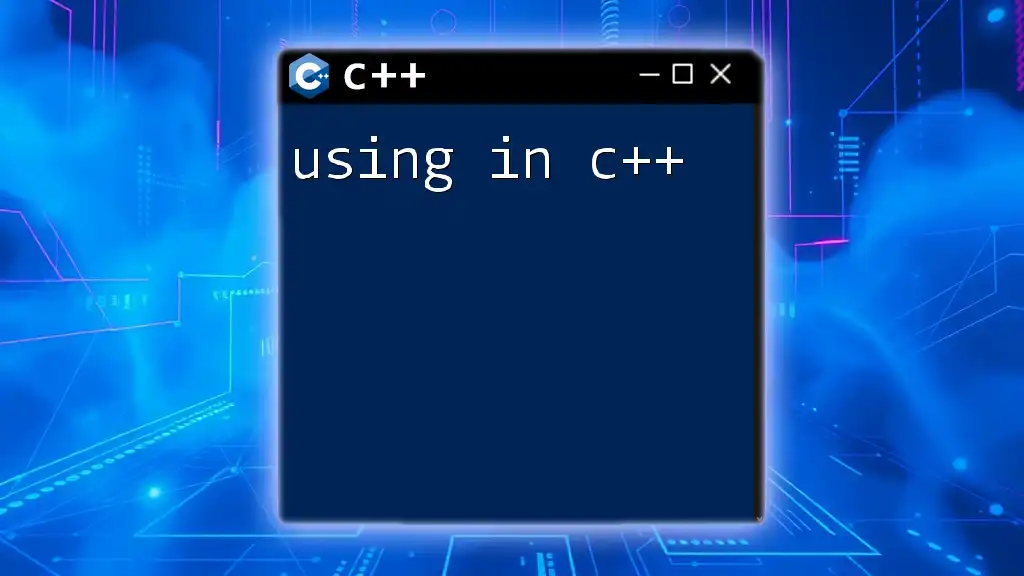
Best Practices for Using Unsigned Long Int in C++
Code Readability
To maintain code readability, always name your variables clearly. Avoid cryptic names like `var1` or `x` in favor of descriptive names, such as `itemCount` or `maxTransactionValue`.
Performance Considerations
Choosing the right data type based on your project’s requirements is crucial. If you're working with large quantities and performance is a concern, opt for long unsigned ints. In contrast, for smaller values, a regular unsigned int should suffice, conserving memory.
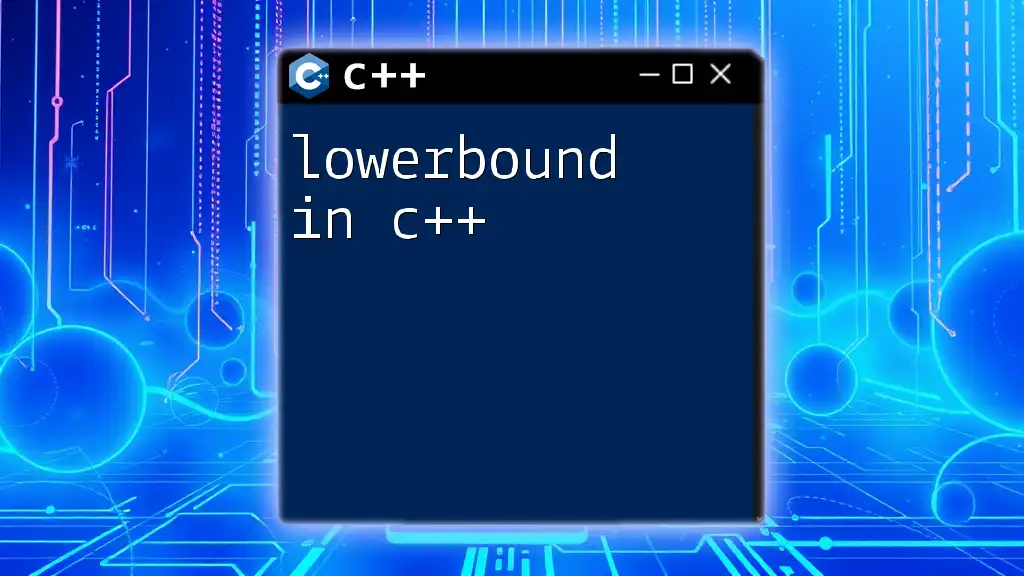
Conclusion
The long unsigned int in C++ is a powerful type that expands the possibilities of numerical computations by enabling the storage of large positive integers. Understanding its characteristics, advantages, and appropriate use cases enhances your programming skills and helps prevent common pitfalls associated with integer overflows. Embrace the power of long unsigned ints and incorporate them into your coding practices to write more efficient and error-free C++ applications.
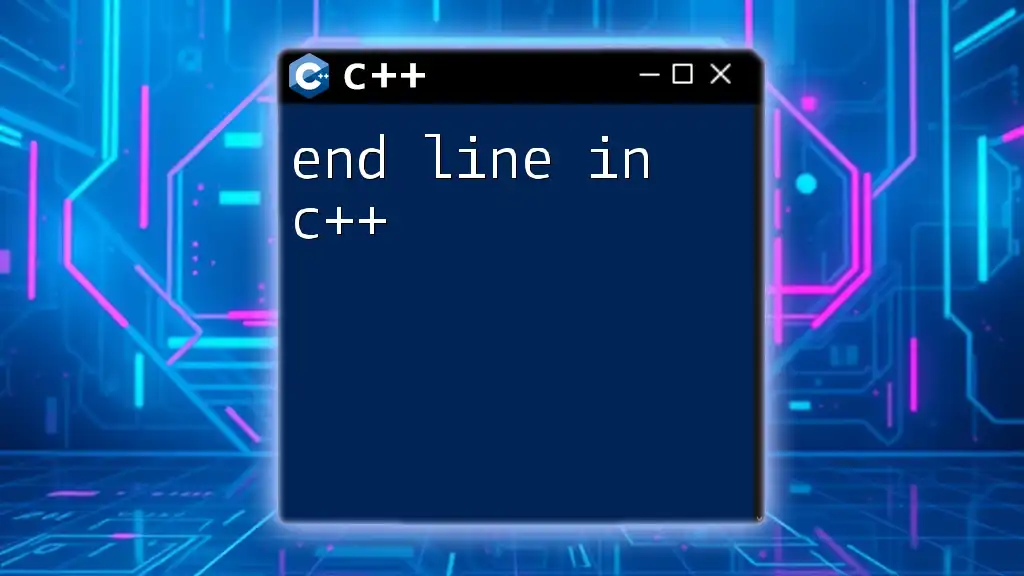
Additional Resources
For further reading, explore the official C++ documentation for comprehensive insights, or consider diving into C++ programming textbooks and online courses that delve deeper into data types and their functionalities.