C++ regex match allows you to check if a string matches a given regular expression pattern using the `<regex>` library, which provides functionalities for creating and searching through regex patterns.
Here’s an example code snippet demonstrating how to use `std::regex_match`:
#include <iostream>
#include <regex>
int main() {
std::string text = "example@example.com";
std::regex pattern(R"((\w+)(\.|_)?(\w*)@(\w+)(\.\w+)+)");
if (std::regex_match(text, pattern)) {
std::cout << "Valid email address." << std::endl;
} else {
std::cout << "Invalid email address." << std::endl;
}
return 0;
}
Understanding Regex Syntax
Regex (short for regular expressions) is a powerful tool used for searching, matching, and manipulating strings. It's vital in various programming tasks, including data validation, parsing, and transformation. In C++, you can harness regex functionalities by including the `<regex>` header from the Standard Template Library (STL).
Basic Components of Regex
Regex consists of literal characters and metacharacters. Literal characters are ordinary characters that match themselves, while metacharacters are special characters that have a unique meaning. Here are some essential metacharacters:
- `.`: Matches any single character (except newline).
- `*`: Matches zero or more occurrences of the preceding character.
- `+`: Matches one or more occurrences of the preceding character.
Character Classes
Character classes allow you to define a set of characters that you want to match. For example:
- `[abc]`: Matches any single character that is either `a`, `b`, or `c`.
- `[^abc]`: Matches any single character not in the set, meaning it won't match `a`, `b`, or `c`.
Anchors in Regex
Anchors are crucial for specifying positions in the string you want to match:
- `^`: Signifies the start of a string.
- `$`: Signifies the end of a string.
For instance, the regex pattern `^Hello` would only match strings that start with "Hello", while `World$` would match strings ending with "World".
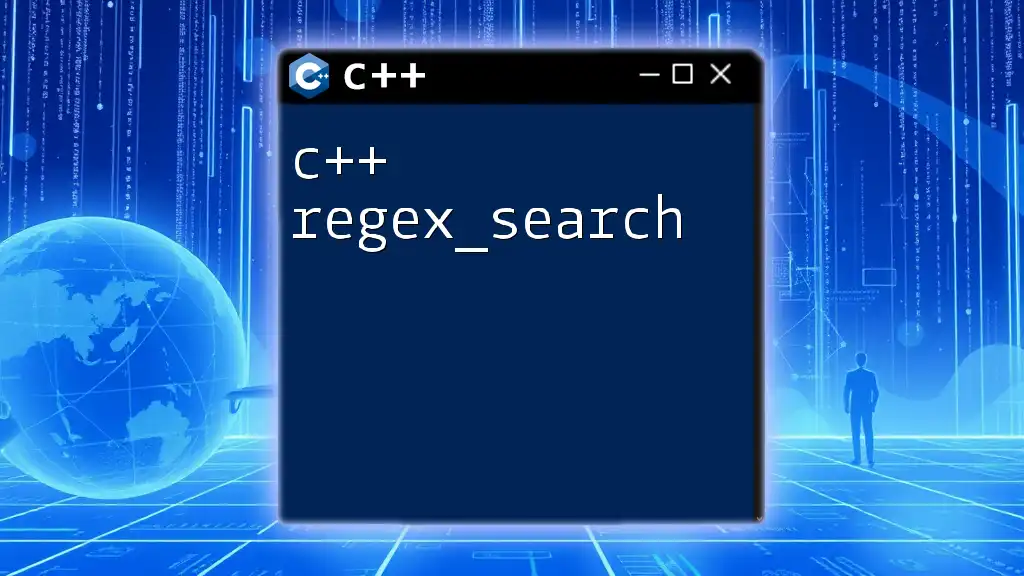
C++ Regex Functions Overview
The C++ regex library provides essential functions that simplify regex operations:
- `std::regex_match()`: Checks if the entire string matches the regex pattern.
- `std::regex_search()`: Searches a string for a match to the regex pattern and can find matches occurring anywhere in the string.
- `std::regex_replace()`: Replaces occurrences of the regex pattern with specified strings.
Understanding Each Function
Each of these functions serves different purposes:
- `std::regex_match()` is ideal when you want to verify that an entire string conforms to a specified pattern.
- `std::regex_search()` is useful when you want to find occurrences of a pattern within a longer string, regardless of the string's format.
- `std::regex_replace()` allows you to replace matched patterns dynamically, making it suitable for formatting or data cleansing tasks.
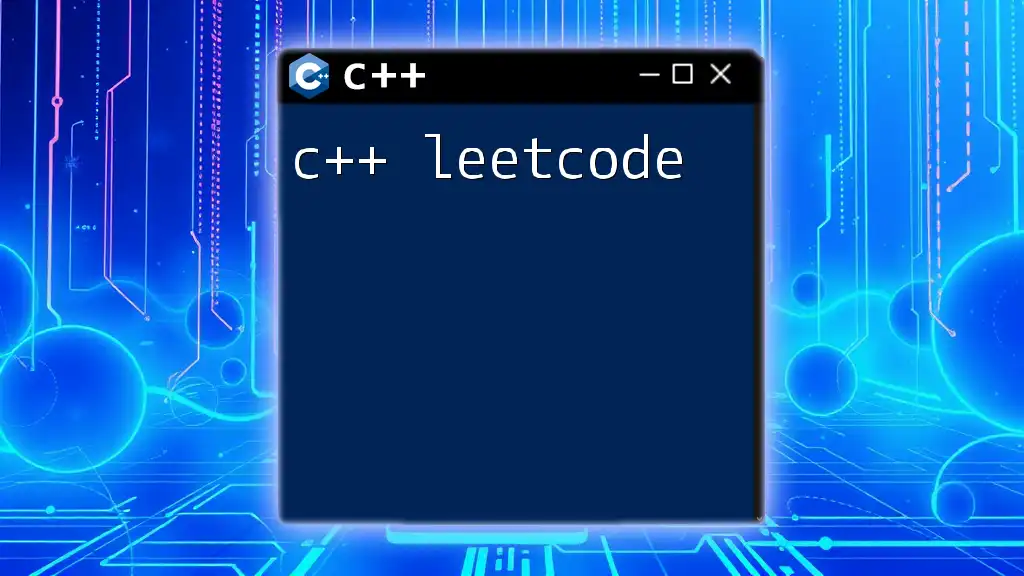
Using std::regex_match() in C++
Function Definition and Parameters
The function declaration for `std::regex_match()` looks as follows:
bool regex_match(const std::string& str, const std::regex& pattern);
- Parameters:
- `str`: The string to be checked against the regex pattern.
- `pattern`: The regex pattern that defines the match criteria.
Example 1: Basic Match
Here’s a simple example of using `std::regex_match()` to check if a given string exactly matches a predefined pattern:
#include <iostream>
#include <regex>
int main() {
std::string str = "HelloWorld";
std::regex pattern("HelloWorld");
if (std::regex_match(str, pattern)) {
std::cout << "Match found!" << std::endl;
} else {
std::cout << "No match." << std::endl;
}
return 0;
}
In this example, the output will be "Match found!" because the entire string exactly matches the regex pattern.
Example 2: Using Capturing Groups
Capturing groups can be useful when you need to extract parts of a matched string. Here’s an example:
#include <iostream>
#include <regex>
int main() {
std::string str = "Name: John, Age: 30";
std::regex pattern("Name: (\\w+), Age: (\\d+)");
std::smatch matches;
if (std::regex_match(str, matches, pattern)) {
std::cout << "Name: " << matches[1] << ", Age: " << matches[2] << std::endl;
} else {
std::cout << "No match." << std::endl;
}
return 0;
}
This code captures the name and age from the string, displaying them as output. The `\\w+` captures the name, while `\\d+` captures the age.
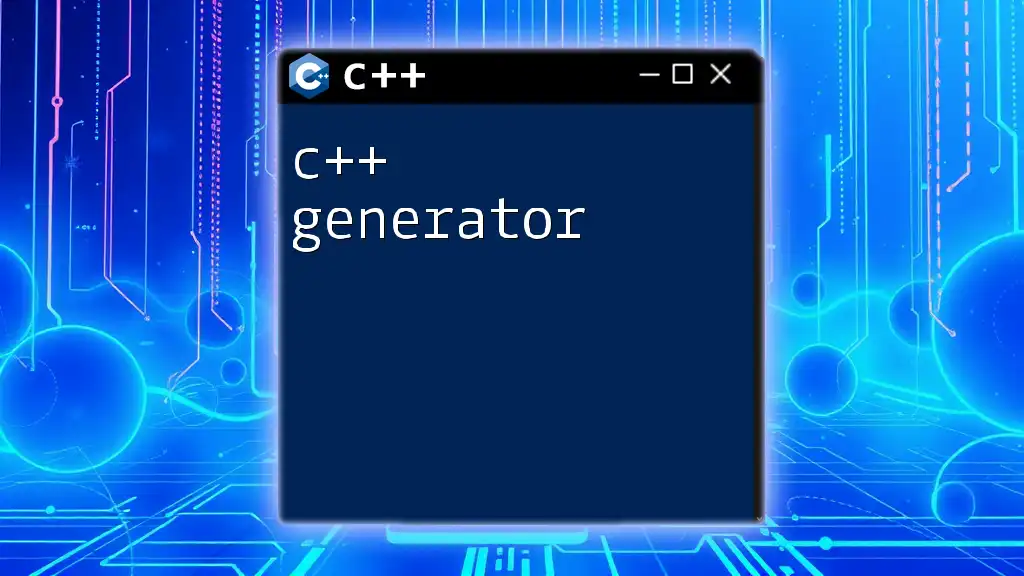
Practical Applications of C++ Regex Match
Form Validation
Validation is one of the most common uses of regex. Here’s how you can validate an email address:
Email Validation Example
#include <iostream>
#include <regex>
int main() {
std::string email = "user@example.com";
std::regex pattern(R"(^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,})$");
if (std::regex_match(email, pattern)) {
std::cout << "Valid email." << std::endl;
} else {
std::cout << "Invalid email." << std::endl;
}
return 0;
}
This example checks if the provided string is a valid email format.
Phone Number Validation
Validating phone numbers can also be achieved using regex. Here’s a simple example:
#include <iostream>
#include <regex>
int main() {
std::string phone = "(123) 456-7890";
std::regex pattern(R"(\(\d{3}\) \d{3}-\d{4})");
if (std::regex_match(phone, pattern)) {
std::cout << "Valid phone number." << std::endl;
} else {
std::cout << "Invalid phone number." << std::endl;
}
return 0;
}
This regex checks for the standard format of a U.S. phone number.
Data Extraction
Regex can also be instrumental in extracting information from larger texts. For example, collecting URLs from a passage:
#include <iostream>
#include <regex>
int main() {
std::string text = "Visit our site at https://example.com and http://abc.com";
std::regex pattern(R"((http|https)://[^\s]+)");
std::smatch matches;
while (std::regex_search(text, matches, pattern)) {
std::cout << "Found URL: " << matches[0] << std::endl;
text = matches.suffix();
}
return 0;
}
This code searches and extracts all URLs from a given text block.
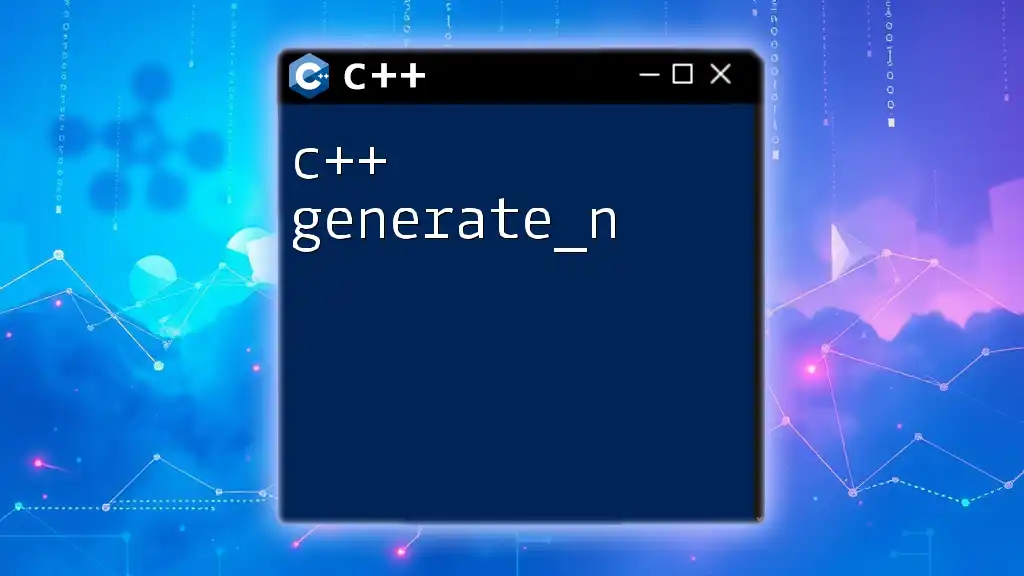
Performance Considerations
When working with regex, it's essential to be aware of its efficiency.
Efficiency of Regex Matching
Regex matching can become computationally expensive, especially with complex patterns. To enhance performance:
- Simplify patterns: Avoid unnecessary complexity.
- Understand backtracking: Regex engines may backtrack in certain situations, leading to slower performance.
Common Pitfalls
Some common pitfalls include:
- Overly complex patterns: These can confuse both the developer and the regex engine itself.
- Improperly escaping metacharacters: Failing to escape necessary characters can lead to unexpected outcomes.
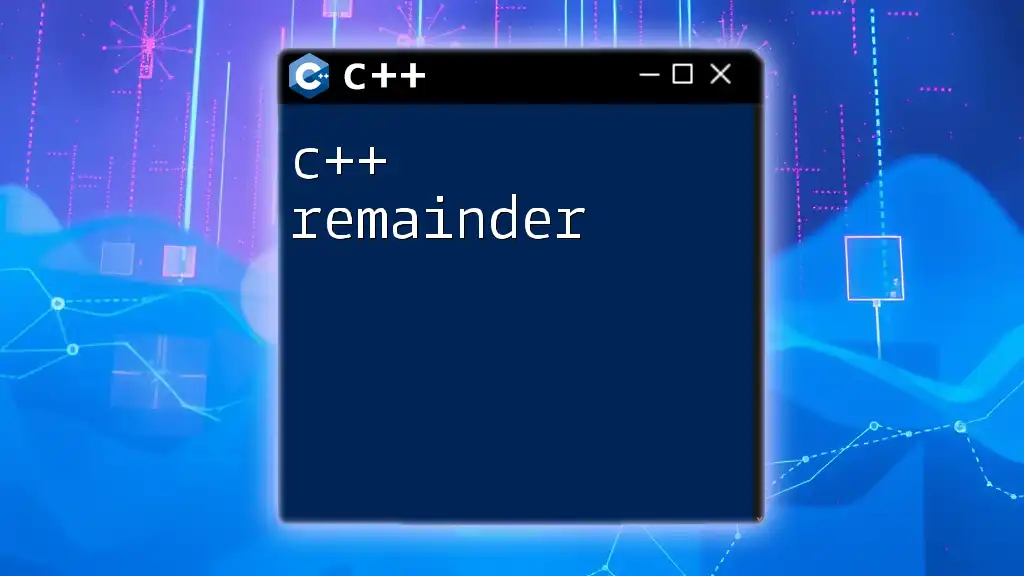
Debugging Regex Patterns
Debugging regex can sometimes be challenging, but recognizing common errors will help:
Common Errors and Solutions
- Misunderstood syntax: Ensure that you’re familiar with regex syntax to avoid misinterpretations.
- Matches that fail unexpectedly: Analyze your patterns and test against various strings to ensure functionality.
Tools for Testing Regex
Several online tools can help debug and refine your regex patterns, such as regex101.com. These tools provide instant feedback and explanations for your patterns.
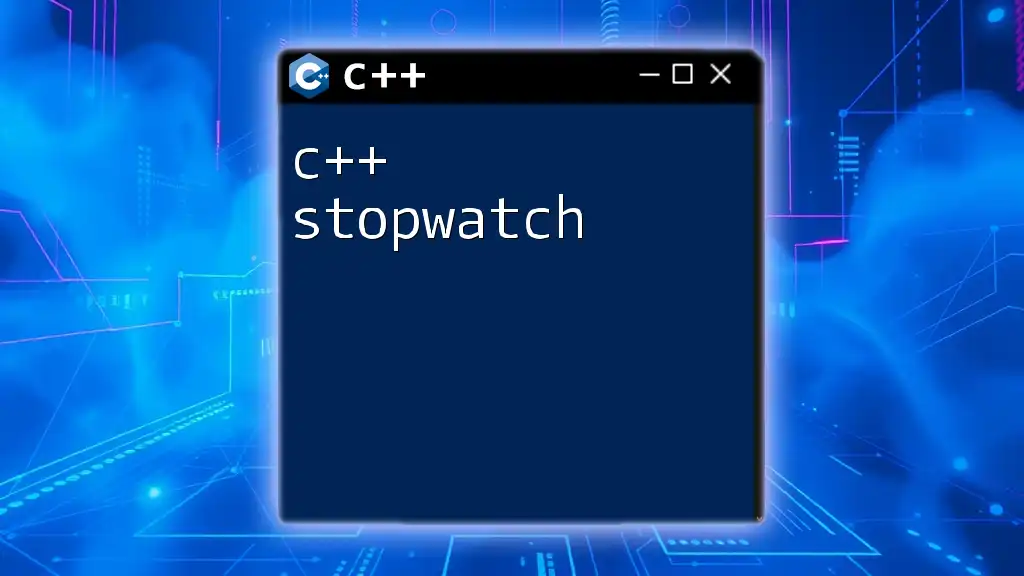
Conclusion and Further Resources
In summary, C++ regex match capabilities are a powerful ally in text processing and validation. By mastering the various functions and patterns, you can significantly enhance the robustness of your string handling in C++.
For further reading, consider exploring the comprehensive C++ standard library documentation, and sites like GeeksforGeeks and Stack Overflow, where you can find additional regex patterns and use cases for practical applications. Mastering regex can open up numerous possibilities in data parsing and validation, making it an invaluable skill for any C++ developer.