In C++, the `try`, `catch`, and `finally` constructs handle exceptions, allowing you to execute code to manage errors during program execution, with `catch` handling the exception, while `finally` can be used in conjunction with other constructs in languages like Java but requires implementation through RAII in C++.
Here's a code snippet demonstrating `try` and `catch` in C++:
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("An error occurred");
} catch (const std::runtime_error& e) {
std::cout << "Caught: " << e.what() << std::endl;
}
// Note: Finally equivalent must be handled using destructors or custom functions in C++
return 0;
}
Understanding Try, Catch, and Throw
Exception handling in C++ is primarily managed using three key constructs: try, catch, and throw. These constructs are essential for dealing with runtime errors gracefully, allowing developers to control the flow of the program when things do not go as intended.
Overview of Exception Handling Constructs
-
try: This keyword defines a block of code that may potentially throw an exception. It is the first line of defense in catching errors.
-
catch: This block follows a try block and is responsible for handling exceptions that have been thrown. The catch block can specify the type of exception it’s willing to handle, making it versatile in managing various error scenarios.
-
throw: This keyword is used to signal that an exception has occurred. Upon encountering a throw statement, control is transferred to the appropriate catch block.
Basic Structure of a Try-Catch Block
The most basic implementation of a try-catch block in C++ follows this syntax:
try {
// Code that may throw an exception
} catch (const exceptionType& e) {
// Code to handle the exception
}
This structure allows for effective error management, where operations that might fail are wrapped in the try block, while the corresponding error handling logic resides in the catch block.

Dive Deeper into Try Blocks
Best Practices for Using Try Blocks
When utilizing try blocks, it’s essential to maintain a few best practices for optimal functionality:
-
Keep the code minimal: Only include the code that might throw an exception within a try block. This practice enhances performance and makes debugging easier.
-
Avoid resource-heavy operations: Heavy computations or resource allocations should be handled outside the try block if possible, as this can lead to unintended exceptions being thrown and might obscure the root cause of an error.
Example of a Try Block in Use
Consider the following example where a function may potentially throw an exception based on the input value:
try {
int result = riskyOperation();
cout << "Operation successful: " << result << endl;
} catch (const std::exception &e) {
cout << "Exception caught: " << e.what() << endl;
}
Here, the `riskyOperation()` is performed within a try block, and should it throw an exception, it is caught by the catch block, allowing for proper error reporting.
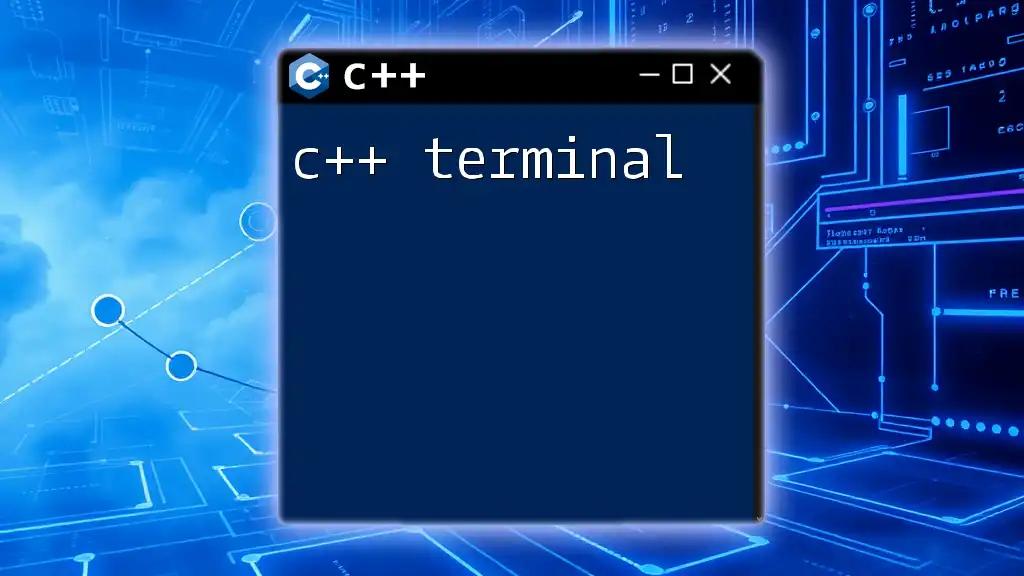
Exploring Catch Blocks
Syntax and Functionality of Catch
The catch block serves as the mechanism to process exceptions thrown by the try block. By specifying different catch handlers, developers can handle individual exception types accordingly, leading to more precise error handling.
Using Multiple Catch Statements
In scenarios where multiple types of exceptions may be thrown, multiple catch statements can be beneficial. This allows tailored responses based on the specific exception encountered. Here's a demonstration:
try {
// Code that might throw different types of exceptions
} catch (const std::invalid_argument& e) {
cout << "Invalid argument: " << e.what() << endl;
} catch (const std::out_of_range& e) {
cout << "Out of range: " << e.what() << endl;
}
In the code above, different catch blocks handle `std::invalid_argument` and `std::out_of_range` exceptions, providing tailored feedback based on the type of exception thrown.
Catching and Rethrowing Exceptions
Sometimes, it may be prudent to handle an exception while also allowing it to propagate further. This can be accomplished through rethrowing, making debugging and logging easier:
try {
riskyOperation();
} catch (const std::exception &e) {
cout << "Handling exception: " << e.what() << endl;
throw; // Rethrowing the exception
}
By using `throw;` without an operand, the current exception is re-thrown, allowing further handling upstream.

The Concept of Finally in C++
Understanding the Need for Finally
The finally construct is not natively part of C++ like in some other languages (e.g., Java, C#). However, its main purpose—ensuring cleanup operations are executed regardless of whether an exception occurred—remains crucial in C++ programming.
Implementing Finally-Like Behavior in C++
To mimic the behavior of a finally block, C++ developers often leverage RAII (Resource Acquisition Is Initialization). RAII involves tying resource management to object lifetime, thereby ensuring that resource cleanup occurs automatically when an object goes out of scope.
Example Using Smart Pointers or Custom Cleanup Classes
An excellent way to implement RAII is through smart pointers, which automatically manage memory:
#include <memory>
class Resource {
public:
Resource() { /* Acquire resource */ }
~Resource() { /* Cleanup resource */ }
};
void functionThatUsesResource() {
Resource r; // Automatically cleaned up at the end of the scope
// Perform operations that might throw exceptions
}
In this example, the `Resource` class’s destructor is called when the variable `r` goes out of scope, ensuring that cleanup is handled even in the case of exceptions.
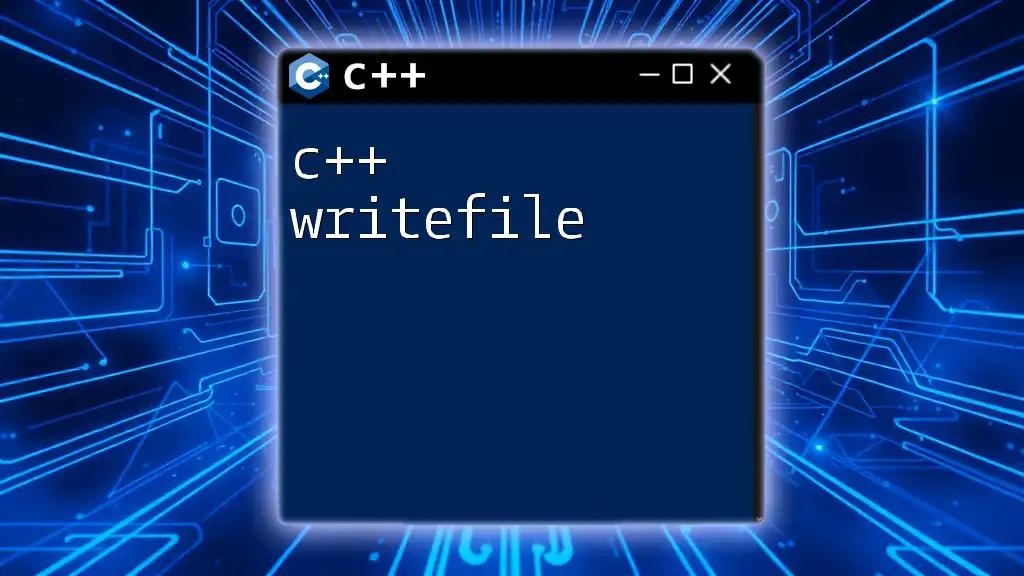
Advanced Exception Handling Techniques
Custom Exception Classes
While the standard exception classes provided by the C++ Standard Library are robust, creating custom exceptions can offer greater flexibility and readability in code. Custom exception classes enable you to encapsulate specific error information relevant to your application.
Example of Creating and Using a Custom Exception Class
class MyCustomException : public std::exception {
public:
const char* what() const noexcept override {
return "My custom exception occurred!";
}
};
Utilizing a custom exception can enhance the clarity of the error handling in the codebase. You can throw this custom exception based on specific conditions:
void someFunction() {
// some operation
if (someErrorCondition) {
throw MyCustomException();
}
}
Using std::exception Hierarchy
Understanding the `std::exception` hierarchy can also aid in effective exception management, allowing developers to leverage built-in exception types effectively while creating robust applications.
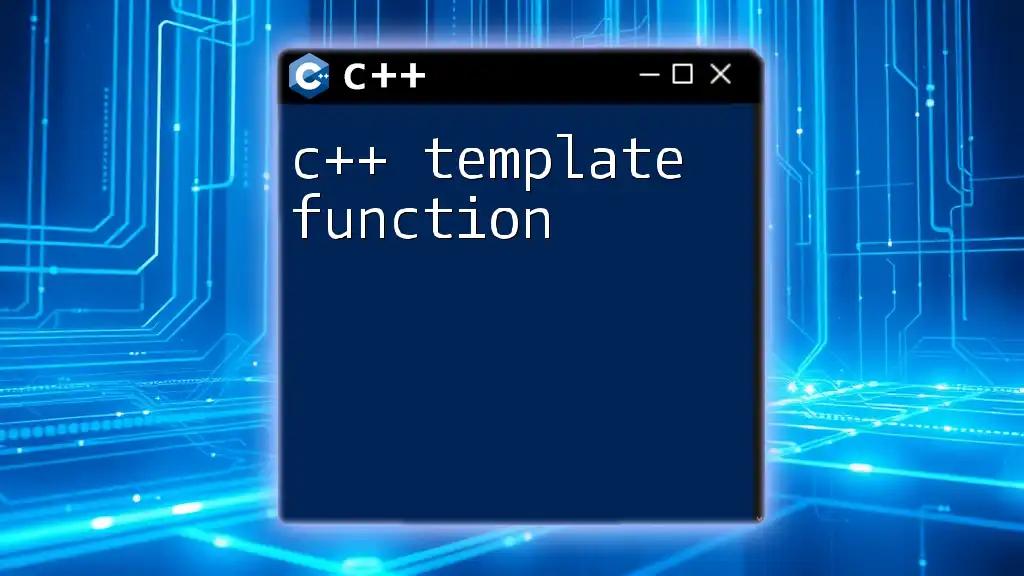
Conclusion
The c++ try catch finally paradigm, while not featuring a direct finally construct, relies on well-established practices and constructs to provide robust exception handling. Understanding and implementing the try-catch mechanism allows developers to create applications that are resilient to errors and maintain a better user experience.
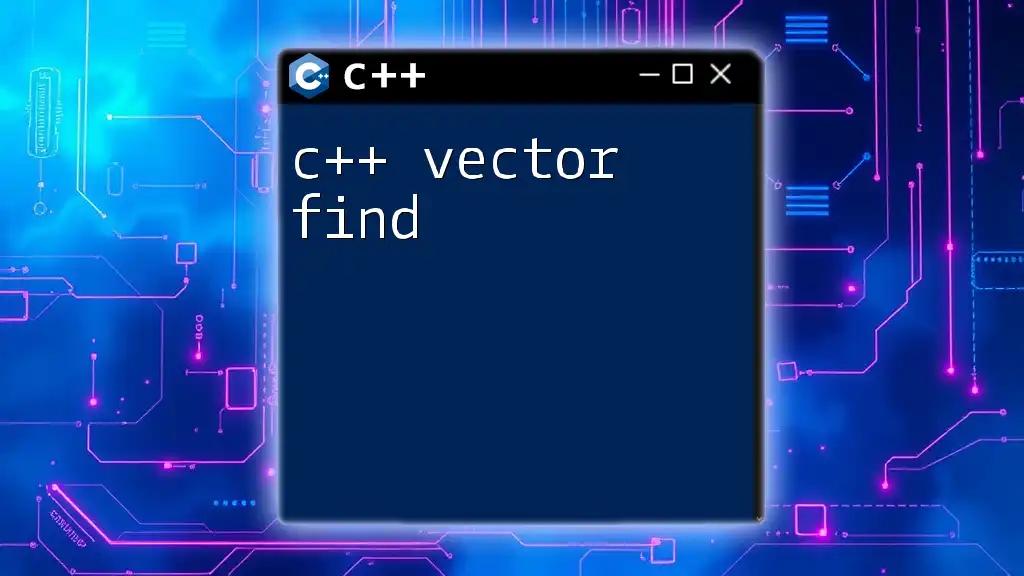
Resources for Further Learning
For those interested in deepening their knowledge, numerous books, online courses, and official documentation covering C++ exception handling techniques are available. Engaging with these resources will aid in mastering exception handling and crafting solid, error-resilient C++ applications.
Examples of Good Practices
Reviewing code snippets showcasing proper error handling techniques will not only reinforce the concepts discussed but also provide practical insights into implementation.
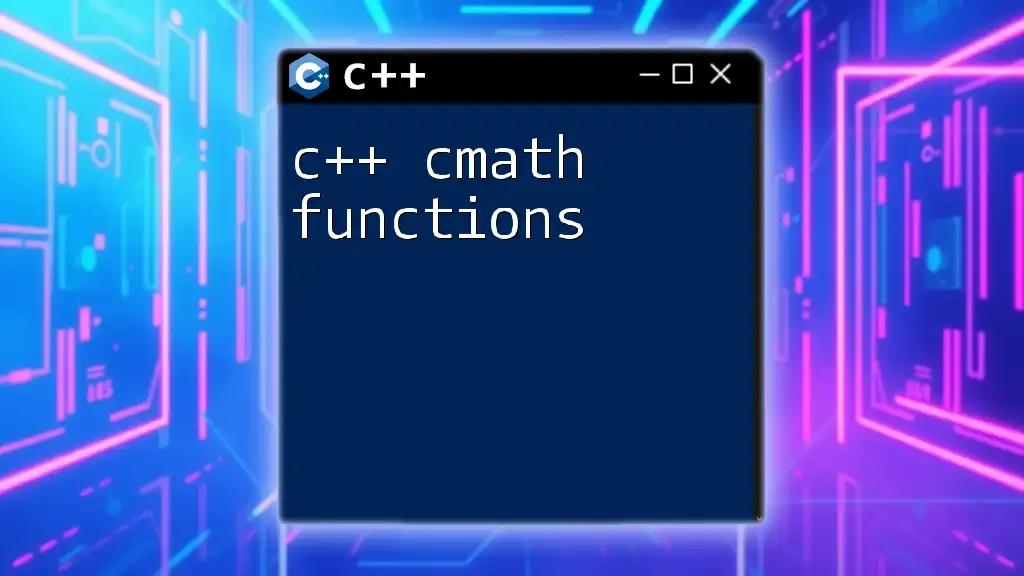
Call to Action
Continue honing your skills in C++ and stay tuned for future posts and lessons that delve even deeper into C++ programming topics. Experiment with the techniques covered here, and explore their application in your projects!