An LRU (Least Recently Used) cache in C++ is a data structure that efficiently stores a limited number of items and evicts the least recently used item when capacity is reached.
Here’s a simple implementation of an LRU cache using C++:
#include <iostream>
#include <list>
#include <unordered_map>
class LRUCache {
public:
LRUCache(int capacity) : cap(capacity) {}
int get(int key) {
auto it = cache.find(key);
if (it == cache.end()) return -1; // Key not found
// Move key to the front (most recently used)
order.splice(order.begin(), order, it->second);
return it->second->second; // Return value
}
void put(int key, int value) {
auto it = cache.find(key);
if (it != cache.end()) {
// Update existing key and move to the front
it->second->second = value;
order.splice(order.begin(), order, it->second);
return;
}
if (cache.size() == cap) {
// Remove least recently used item
int lruKey = order.back().first;
order.pop_back();
cache.erase(lruKey);
}
// Insert new key-value pair
order.emplace_front(key, value);
cache[key] = order.begin();
}
private:
int cap;
std::list<std::pair<int, int>> order; // List of key-value pairs
std::unordered_map<int, decltype(order.begin())> cache; // Map of key to list iterator
};
This code demonstrates a basic implementation of an LRU cache using a combination of a doubly linked list and an unordered map to achieve O(1) time complexity for both get and put operations.
Understanding the LRU Cache
What is an LRU Cache?
The Least Recently Used (LRU) cache is a mechanism often employed in computing for managing resource allocation efficiently. At its core, an LRU Cache keeps track of a set amount of cached items and evicts the least recently used ones when the limit is exceeded. This strategy is especially useful in scenarios where memory or storage allocation needs to optimize access to frequently used data.
Why Use an LRU Cache in C++?
Implementing an LRU Cache in C++ has significant advantages. First and foremost, it improves overall performance by minimizing access times when retrieving data. This is particularly important for applications where speed is critical, such as web services and database management. Furthermore, an LRU Cache contributes to better memory management. Compared to standard collections, this method ensures that only the most relevant data is retained, which leads to efficient use of memory resources.

How an LRU Cache Works
Key Components of an LRU Cache
An LRU Cache generally comprises two vital structures:
- HashMap: This allows for quick retrieval of items stored in the cache. By mapping keys directly to their respective data, the time complexity for accessing items is reduced to O(1).
- Doubly Linked List: This structure maintains the order of items based on usage. Each time an item is accessed, it is moved to the front of the list, while the least recently used items fall to the back, ready for eviction when necessary.
Basic Operations
The main operations of an LRU Cache include:
- Get Operation: When fetching an item, if it exists in the cache, it is retrieved and marked as recently used (moved to the front of the list).
- Put Operation: Adding a new item or updating an existing one. If the cache exceeds its designated capacity, the least recently used item is evicted to make space for the new entry.

Implementing an LRU Cache in C++
Setting Up the Environment
Before diving into coding, make sure to include the necessary C++ libraries. You will commonly use:
#include <unordered_map>
#include <list>
These libraries are essential for using hash maps and lists efficiently. To compile and run your C++ code, use a compatible IDE or a terminal with the appropriate C++ compiler installed.
LRU Cache Class Structure
To encapsulate the functionality of an LRU Cache, you will start by declaring a class named `LRUCache`. The core design includes private member variables to maintain the cache's capacity, a hash map for fast lookups, and a linked list for item ordering.
class LRUCache {
private:
int capacity; // Maximum capacity of the cache
std::list<int> lruList; // Doubly linked list to track usage order
std::unordered_map<int, std::pair<int, decltype(lruList.begin())>> cacheMap;
// Key, Pair of Value and Iterator pointing to the list
public:
LRUCache(int capacity);
int get(int key);
void put(int key, int value);
};
Code Implementation
Constructor
The constructor initializes the cache with a specified capacity.
LRUCache::LRUCache(int capacity) {
this->capacity = capacity;
}
Get Method
In the `get` method, the cache checks if the requested key is present. If it is, the corresponding value is returned, and the item's position in the linked list is updated.
int LRUCache::get(int key) {
auto it = cacheMap.find(key);
if (it == cacheMap.end()) {
return -1; // Not found
} else {
// Move the accessed item to the front of the list
lruList.splice(lruList.begin(), lruList, it->second.second);
return it->second.first; // Return value
}
}
Put Method
The `put` method handles insertion or updating items in the cache. If the key already exists, it updates the value and adjusts the order in the linked list. If the cache has reached its limit, it evicts the least recently used item.
void LRUCache::put(int key, int value) {
auto it = cacheMap.find(key);
if (it != cacheMap.end()) {
// Update value and move item to the front
lruList.splice(lruList.begin(), lruList, it->second.second);
it->second.first = value; // Update value
} else {
if (cacheMap.size() >= capacity) {
// Evict the least recently used item
int lruKey = lruList.back(); // Get least recently used key
lruList.pop_back(); // Remove from list
cacheMap.erase(lruKey); // Remove from map
}
// Insert new key-value pair at the front
lruList.push_front(key);
cacheMap[key] = {value, lruList.begin()};
}
}
Complete Example of LRU Cache Implementation
In your main function, you can create an instance of the LRU Cache and perform operations to observe its functionality.
int main() {
LRUCache cache(2); // Capacity of 2
cache.put(1, 1); // Cache is {1=1}
cache.put(2, 2); // Cache is {1=1, 2=2}
std::cout << cache.get(1); // Returns 1
cache.put(3, 3); // Evicts key 2
std::cout << cache.get(2); // Returns -1 (not found)
cache.put(4, 4); // Evicts key 1
std::cout << cache.get(1); // Returns -1 (not found)
std::cout << cache.get(3); // Returns 3
std::cout << cache.get(4); // Returns 4
}
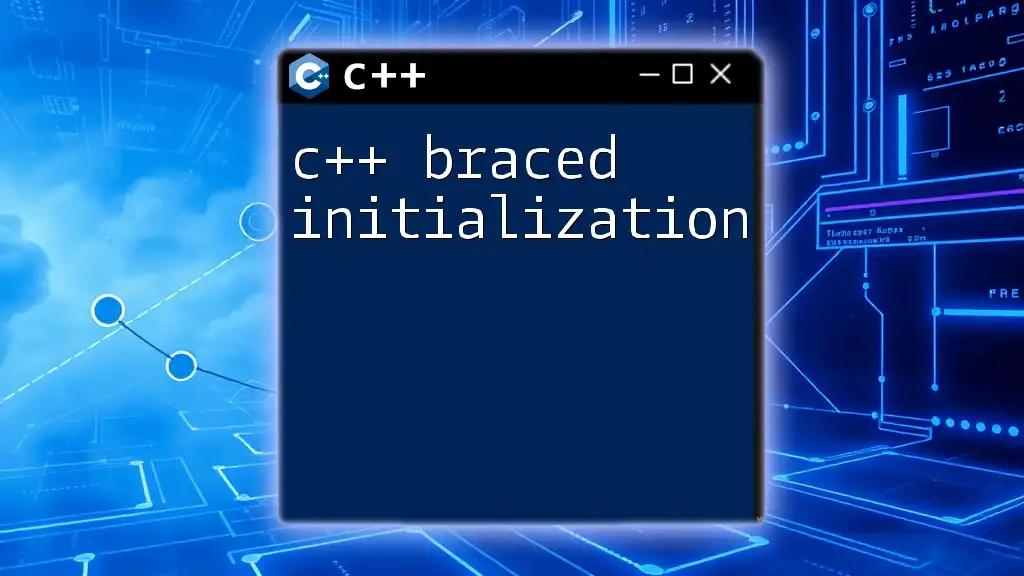
Testing the LRU Cache
Unit Testing LRU Cache
Testing is a crucial part of ensuring your LRU Cache works as expected. You can use testing frameworks like Google Test to create unit tests for your functionality.
TEST(LRUCacheTest, BasicOperations) {
LRUCache cache(2);
cache.put(1, 1);
ASSERT_EQ(cache.get(1), 1);
cache.put(2, 2);
ASSERT_EQ(cache.get(2), 2);
cache.put(3, 3); // Evicts key 1
ASSERT_EQ(cache.get(1), -1);
}
Performance Metrics
When assessing the efficiency of your LRU Cache implementation:
- Time Complexity: Both the `get` and `put` operations should ideally run in O(1) time due to the combination of the hash map and the linked list.
- Space Complexity: The space used depends heavily on the amount of data stored, leading to an O(n) space complexity, where n is the capacity of the cache.
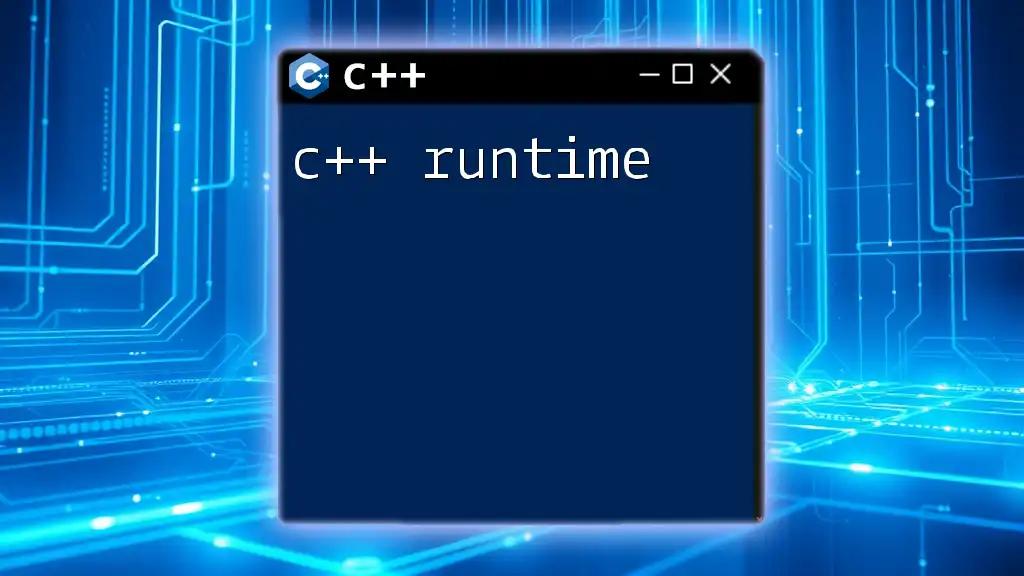
Conclusion
Summary of Key Points
Implementing a C++ LRU Cache can vastly improve data access speeds in applications requiring real-time data retrieval. By utilizing a combination of a hash map and a doubly linked list, this caching strategy effectively manages memory and optimizes performance.
Next Steps for Learners
For those looking to deepen their understanding of caching mechanisms, consider exploring additional resources in data structures and algorithms. Read books or articles that expand on advanced caching strategies, data organization methods, and performance optimization techniques.
Call to Action
We encourage you to implement your own LRU Cache using the guidelines provided and experiment with various data sets. If you have questions or feedback, feel free to reach out!