C++ can be integrated with Lua to enhance game development and scripting capabilities, allowing for efficient execution of Lua scripts directly within a C++ application.
Here's an example of how to execute a simple Lua script from C++:
#include <iostream>
#include <lua.hpp>
int main() {
lua_State* L = luaL_newstate(); // Create a new Lua state
luaL_openlibs(L); // Open Lua standard libraries
luaL_dostring(L, "print('Hello from Lua!')"); // Execute a simple Lua script
lua_close(L); // Close the Lua state
return 0;
}
Understanding Lua
What is Lua?
Lua is a powerful, efficient, lightweight, and embeddable scripting language. Designed in Brazil in the early 1990s, it has gained popularity due to its simplicity and flexibility. Its primary purpose is to serve as a configuration language for applications and offer scripting capabilities, making it particularly well-suited for game development.
Why Choose Lua for C++ Projects?
Integrating Lua with C++ brings significant advantages:
-
Performance Considerations: Lua is known for its execution speed and small footprint. In scenarios where you require fast script execution alongside demanding C++ code, Lua seamlessly fills the gap without impacting performance adversely.
-
Flexibility in Scripting: Lua provides dynamic typing and an approachable syntax, enabling programmers to write scripts rapidly. Adjusting and tweaking game behavior or application flow becomes easier with Lua's scripting capabilities.
-
Rich Libraries and Community Support: The Lua ecosystem offers a plethora of libraries tailored for various needs. With a vibrant community behind it, finding resources, troubleshooting, and sharing knowledge is readily accessible.
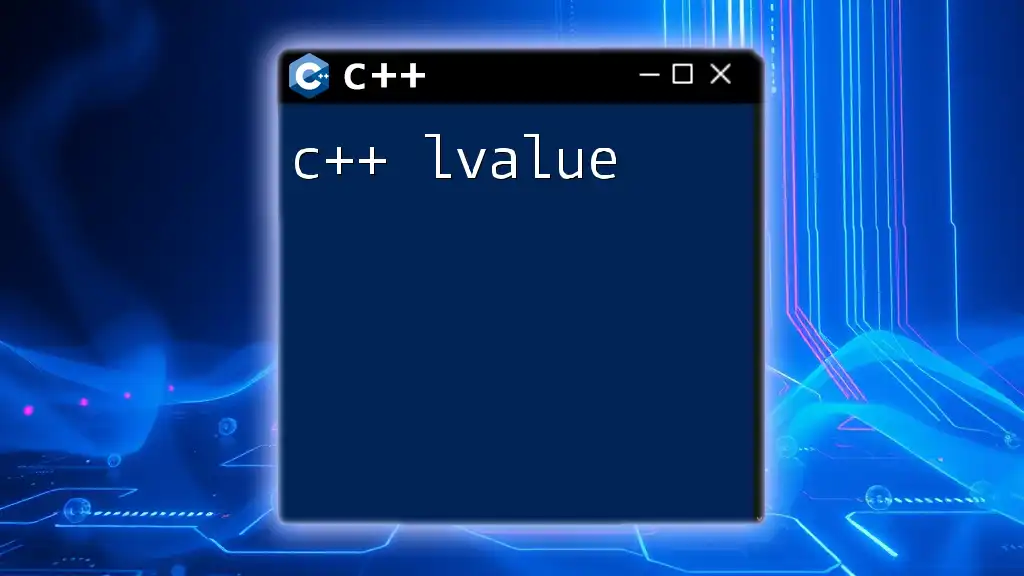
Setting Up Your Environment
Installing Lua
To get started with Lua, the first step is to install it on your machine. Follow these general steps based on your operating system:
-
For Windows, download the Lua binaries from the official Lua website. Extract the files and add the folder to your system's PATH environment variable.
-
For Linux, you can install Lua directly using your package manager, for example:
sudo apt-get install lua5.3
-
On Mac systems, you can use Homebrew to install Lua:
brew install lua
Integrating Lua with C++
Integrating Lua with your C++ project enables you to utilize Lua's scripting features. The most common method is using the Lua C API. Libraries like LuaBridge and Luabind simplify this process, providing an easier interface.
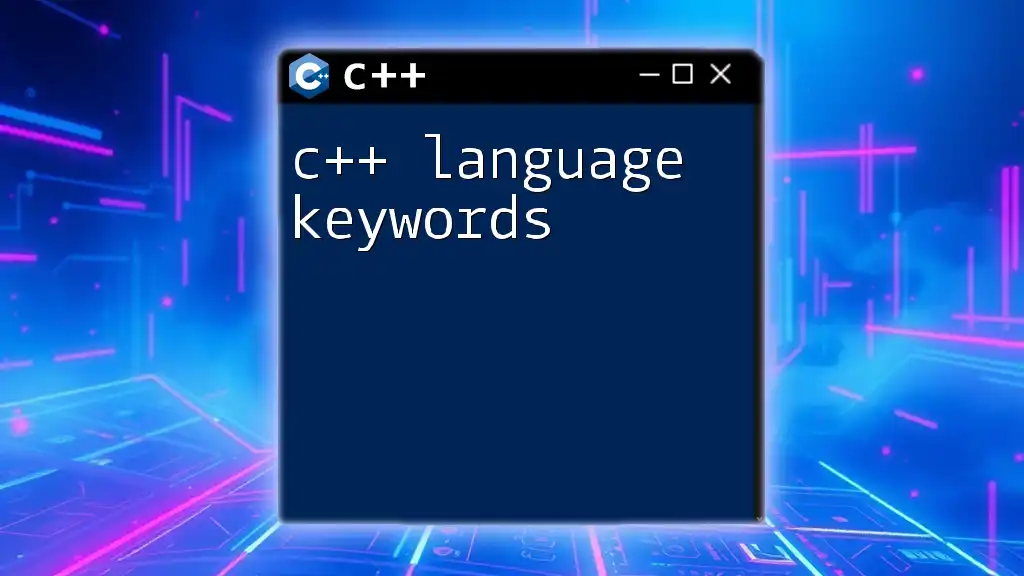
Basic Syntax and Features of Lua
Lua Basic Data Types
Lua supports several fundamental data types, which include:
- Numbers: Represent real numbers (both integers and floats).
- Strings: Immutable sequences of characters.
- Booleans: Represented as `true` or `false`.
- Tables: Lua’s primary data structure, akin to arrays and dictionaries in other languages.
Here’s a simple example demonstrating basic data types in Lua:
local number = 42
local text = "Hello, Lua!"
print(text .. " The answer is: " .. number)
Functions in Lua
Functions in Lua can be defined simply and effectively. You can create both local and global functions. Here’s a basic example:
function greet(name)
return "Hello, " .. name
end
print(greet("World"))
This example demonstrates how to define a function and call it with an argument.
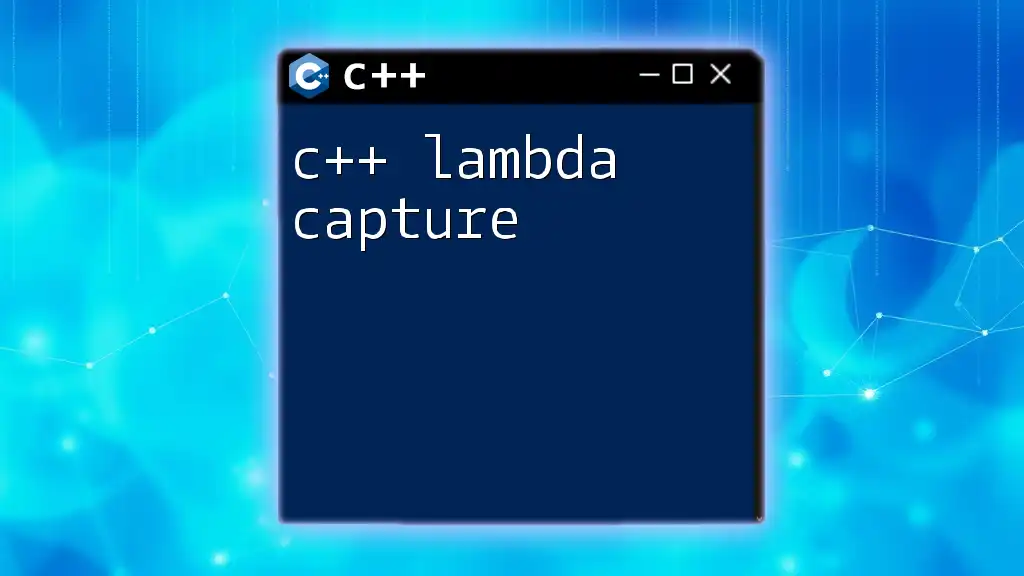
Linking C++ with Lua
The Lua C API
The Lua C API is a powerful interface allowing C++ code to interact with Lua scripts directly. Here’s how to create a Lua state in your C++ application:
#include <lua.hpp>
lua_State* L = luaL_newstate();
luaL_openlibs(L); // Load standard libraries
This piece of code initializes a new Lua state and opens the standard libraries, making Lua functions available for your scripts.
Calling Lua Functions from C++
You can execute Lua functions from C++ seamlessly. Here’s how:
- Load a Lua file containing the function.
- Retrieve the function from the global Lua namespace.
- Call the function using `lua_pcall`.
Example:
luaL_dofile(L, "script.lua"); // Load the Lua script
lua_getglobal(L, "function_name"); // Get the Lua function
lua_pcall(L, 0, 1, 0); // Call it
This approach allows you to interface with Lua's powerful scripting directly from your C++ code.
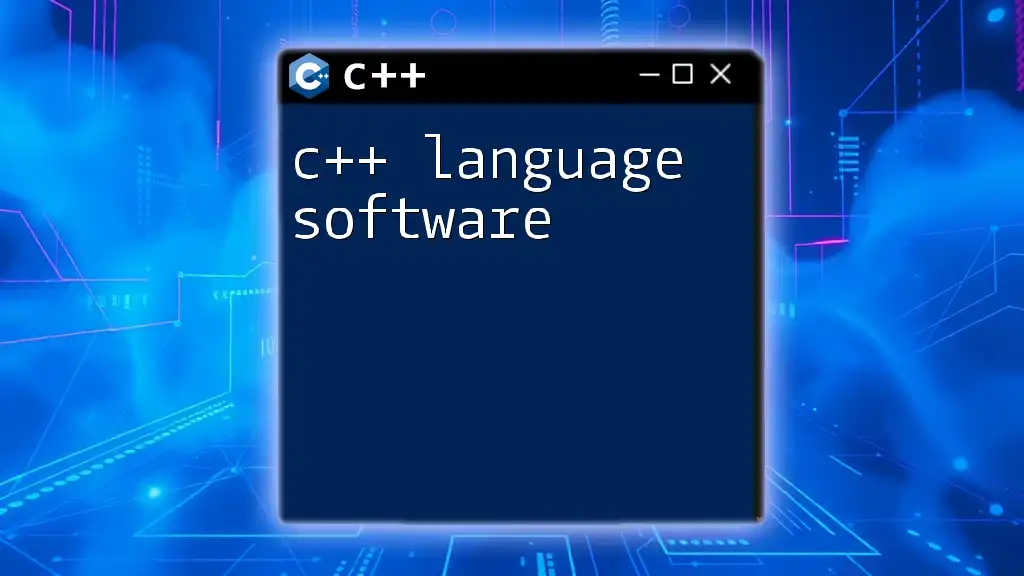
Manipulating Lua Variables in C++
Pushing and Popping Values
Lua operates on a stack-based system, which means you will often push values onto the stack before using them. Here’s an example:
lua_pushnumber(L, 10); // Push a number
lua_pushstring(L, "Hello!"); // Push a string
You can later retrieve these values in your Lua scripts.
Accessing Lua Tables from C++
Lua tables are versatile data structures, and manipulating them from C++ is straightforward. Here’s how you can interact with a Lua table:
lua_getglobal(L, "myTable"); // Access Lua table
lua_pushnumber(L, 1);
lua_pushstring(L, "Value");
lua_settable(L, -3); // Set value in the table
In this example, we push a value to a specific key (in this case, the integer 1) in the Lua table named `myTable`.
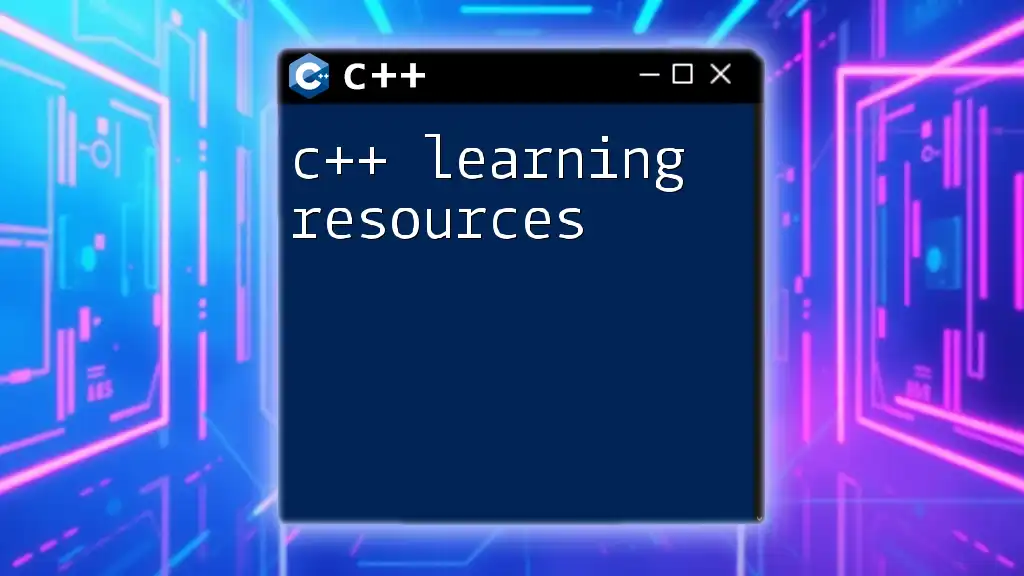
Advanced Topics
Error Handling in Lua
When working with Lua, it’s crucial to implement proper error handling to catch any issues that arise when executing Lua code. Here is a typical approach:
if (luaL_dofile(L, "script.lua") != LUA_OK) {
const char* errorMessage = lua_tostring(L, -1);
std::cerr << "Error: " << errorMessage << std::endl;
lua_pop(L, 1); // Remove error message from stack
}
This code checks for errors during script execution and prints an error message while cleaning up the Lua stack.
Using C++ Libraries with Lua
When using third-party C++ libraries in your Lua scripts, you may need to expose C++ classes or objects to Lua. This process typically involves creating bindings that Lua can access. Many libraries, such as LuaBridge, simplify this integration.
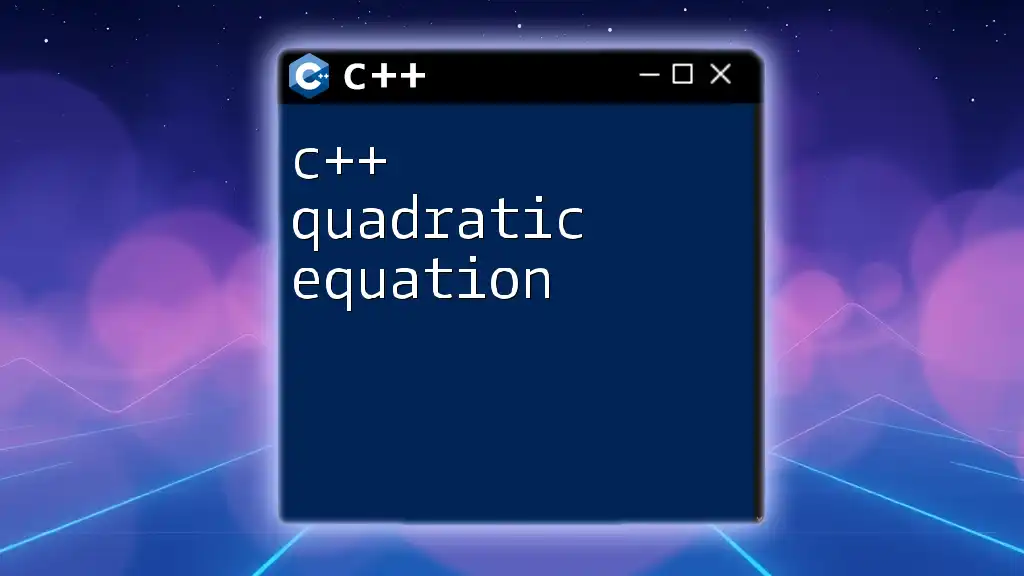
Best Practices for Using C++ with Lua
Performance Tips
To optimize performance while using C++ and Lua, consider the following:
-
Minimize Context Switching: Excessive switching between C++ and Lua can slow down performance. Try to batch operations in Lua to reduce calls to C++.
-
Profile Your Code: Use profiling tools to identify bottlenecks in your scripts and code. This ensures you optimize the right sections.
Maintainability Considerations
As your project grows, maintainability becomes crucial. Follow these strategies:
-
Modular Design: Keep your C++ and Lua code modular. Break down larger projects into smaller, manageable chunks.
-
Documentation: Maintain comprehensive documentation for your codebase, including inline comments, to help others understand your code structure and logic.
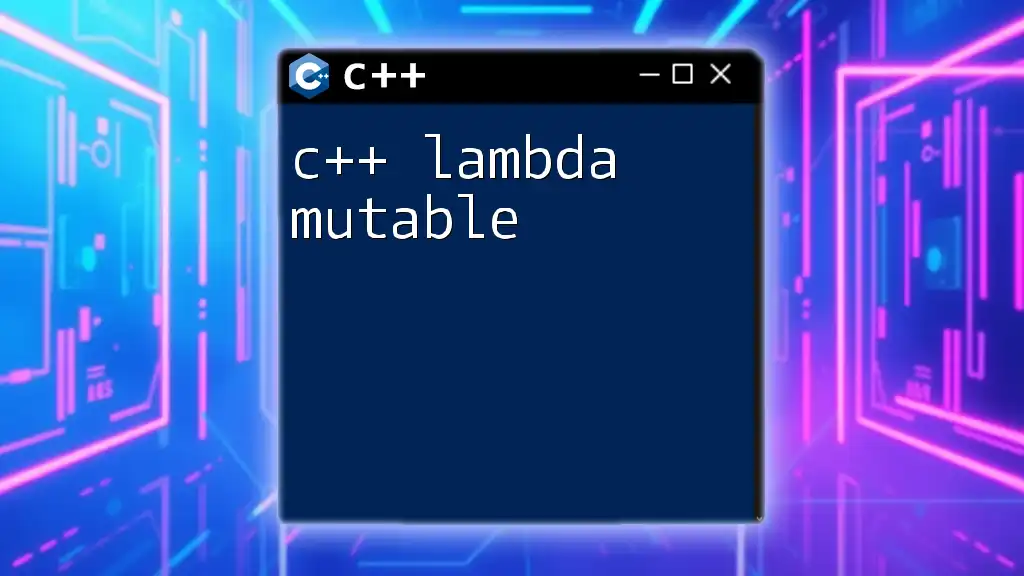
Conclusion
Integrating C++ and Lua provides flexibility and efficiency in software development. This comprehensive guide has highlighted the basics of Lua, how to set up an environment, and methods for linking it with C++.
As you explore more complex projects, embrace experimenting with various features that C++ and Lua offer. The melding of these two powerful programming languages can lead to innovative solutions, particularly in fields like game development, where dynamic behavior is essential.
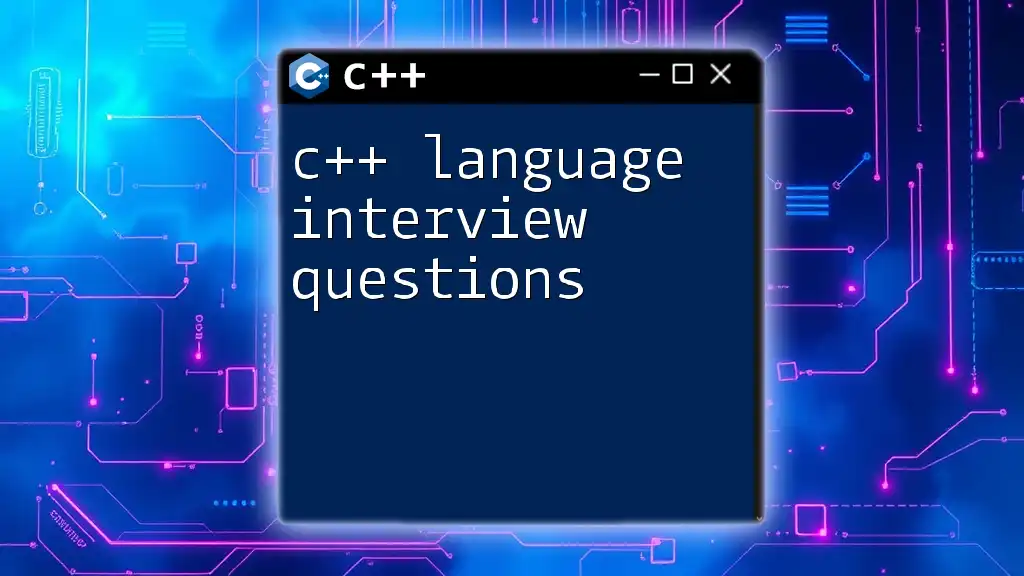
Further Resources
For continual learning, explore these resources:
- Books: Look for titles focused on Lua and C++, delving deeper into their integration.
- Websites: Many online platforms provide tutorials and libraries for both languages.
- Community Forums: Engage with communities around C++ and Lua to share knowledge and get support.
Embrace the synergy of C++ and Lua, and enjoy the journey of creating powerful applications!