The "Hello World" program in C++ is a simple example that demonstrates the basic syntax for outputting text to the console, often used as an introductory exercise for beginners.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Hello World Program in C++
What is the Hello World Program?
The Hello World program is often the very first program written by beginners when learning a new programming language. It serves as an introductory exercise that helps familiarize users with the basic syntax and structure of the language. In C++, the Hello World program is particularly important because it lays the groundwork for understanding how C++ handles input and output operations.
Components of a C++ Program
Before diving into the code, it’s essential to understand the fundamental components that constitute a C++ program. A basic C++ program typically includes header files, a `main` function, and statements that execute upon running the program.
- Header Files: These are files that contain declarations of functions and macros. For our Hello World program, we will use the `<iostream>` header, which allows us to utilize input and output streams.
- Main Function: Every C++ program must have a `main` function. This is the starting point of execution. The program's logic is executed within this block.
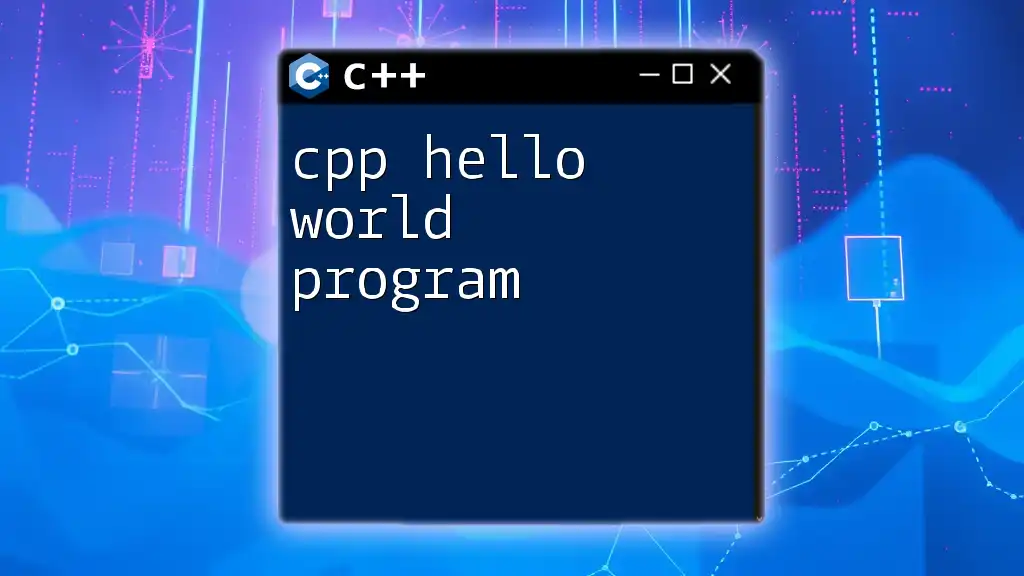
Writing Your First Hello World Program
Let’s move on to writing the Hello World C++ code. Here is a simple yet effective example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Code Breakdown
Now, let’s break down the code snippet to understand how it works.
-
`#include <iostream>`: This line instructs the compiler to include the standard input-output stream library, which is necessary for performing input and output operations in C++.
-
`int main()`: This defines the `main` function where the execution starts. It returns an integer value, which indicates the termination status of the program.
-
`std::cout`: Here, `std::cout` represents the standard output stream. It allows us to print data to the console. The prefix `std::` is necessary because `cout` is contained within the C++ standard library namespace, named `std`.
-
`<< "Hello, World!"`: The `<<` operator is known as the output operator. It directs what follows (in this case, the string "Hello, World!") to the output stream.
-
`std::endl`: This is used to insert a newline character and flush the output buffer, ensuring that everything has been displayed on the console before continuing with any further operations.
-
`return 0;`: This signifies that the program has executed successfully. The return value of `0` typically indicates that no errors occurred.
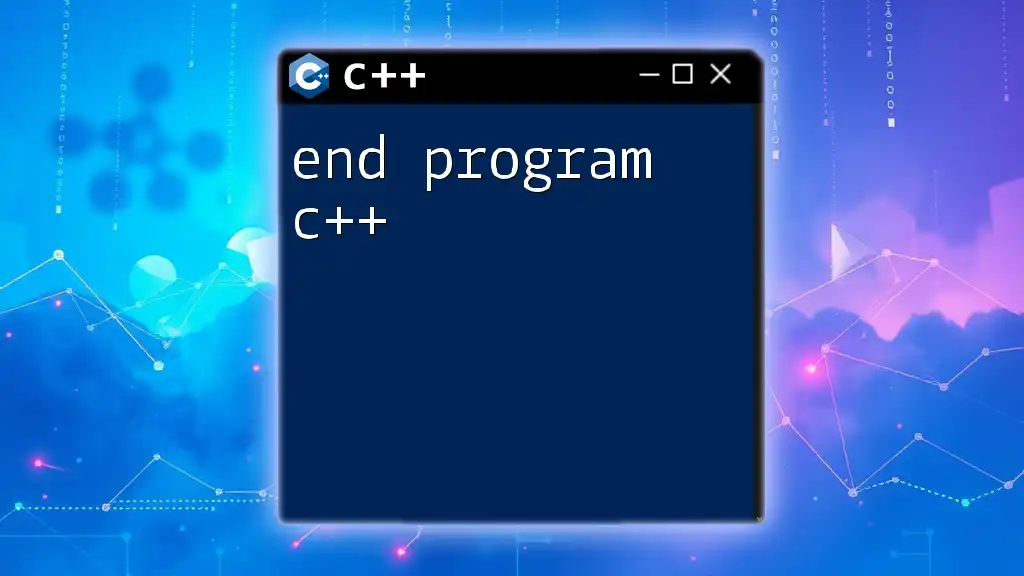
Compiling and Running the Hello World Program
Setting Up Your Environment
Before running the Hello World program, it’s crucial to set up your development environment. You can choose from a number of Integrated Development Environments (IDEs) and compilers for C++. Popular choices include:
- Code::Blocks
- g++ compiler (often used via the command line)
- Visual Studio
How to Compile and Execute the Code
To compile and execute your code, follow these step-by-step instructions based on your setup:
Using a Command Line Interface
- Open your command line interface.
- Navigate to the directory where your C++ file (let’s say `hello.cpp`) is saved.
- Compile the program using the following command:
g++ hello.cpp -o hello
- Run the compiled program with:
./hello
Using an IDE
If you are using an IDE like Code::Blocks or Visual Studio, simply create a new project, paste the Hello World code into the main file, and use the built-in buttons to compile and run the application.
Common Errors and Troubleshooting
When starting with C++, you might come across some common errors:
- Syntax Errors: Pay close attention to semicolons, parentheses, and braces.
- Header File Issues: Ensure you include the correct headers. Using `#include <iostream>` is crucial for output operations.
- Main Function Return Type: Make sure your `main` function is defined with an `int` return type and contains a `return 0;` statement.
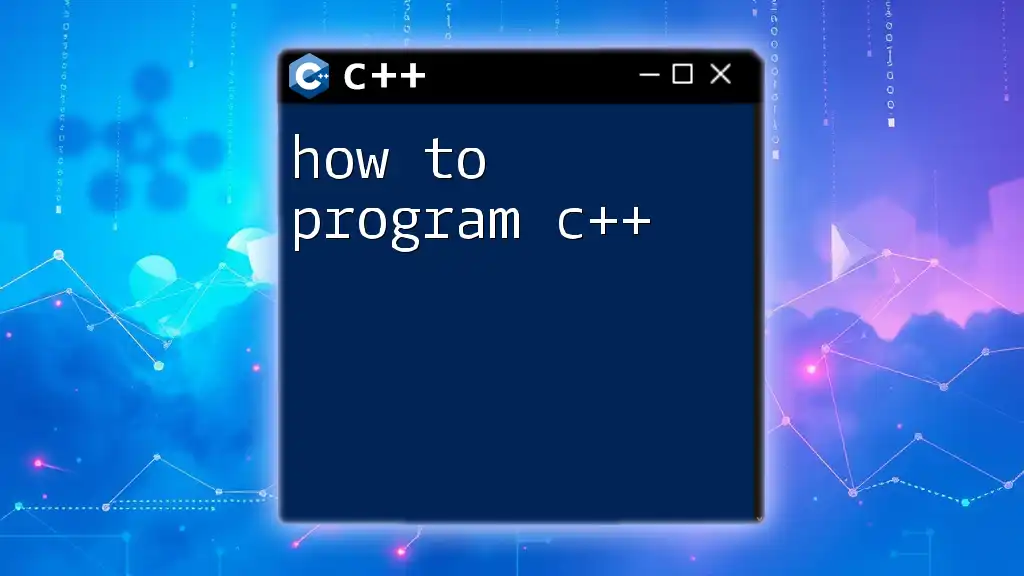
Expanding on the Hello World Concept
Variations of Printing Hello World
After successfully running your Hello World program, you may want to experiment with variations of outputting text. Below is how you might modify the output statement:
std::cout << "Hello, Universe!" << std::endl;
You can further combine expressions with strings:
std::cout << 10 + 5 << " Hello World!" << std::endl; // Output: 15 Hello World!
Using Different Data Types in C++
In C++, the Hello World program can be extended by including different data types. For example, you can output integers, strings, and characters as follows:
int number = 42;
std::cout << "The answer is: " << number << std::endl;
This snippet illustrates how variables can be introduced and used in your output, showcasing the versatility of C++ syntax.
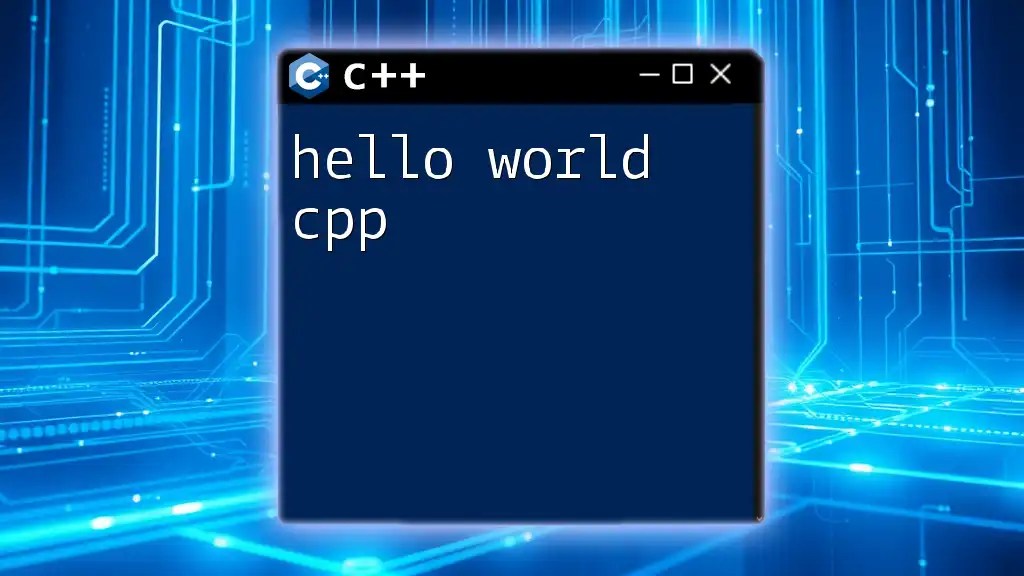
Conclusion
The Hello World program in C++ is a fundamental stepping stone for anyone eager to delve into this powerful programming language. It introduces you to basic concepts, syntax, and the structure of C++ programs while providing a hands-on approach to coding.
By understanding and executing simple programs like this, you build the foundation necessary to tackle more complex C++ coding tasks. We encourage you to explore additional resources and continue your programming journey, enhancing your skills and confidence.
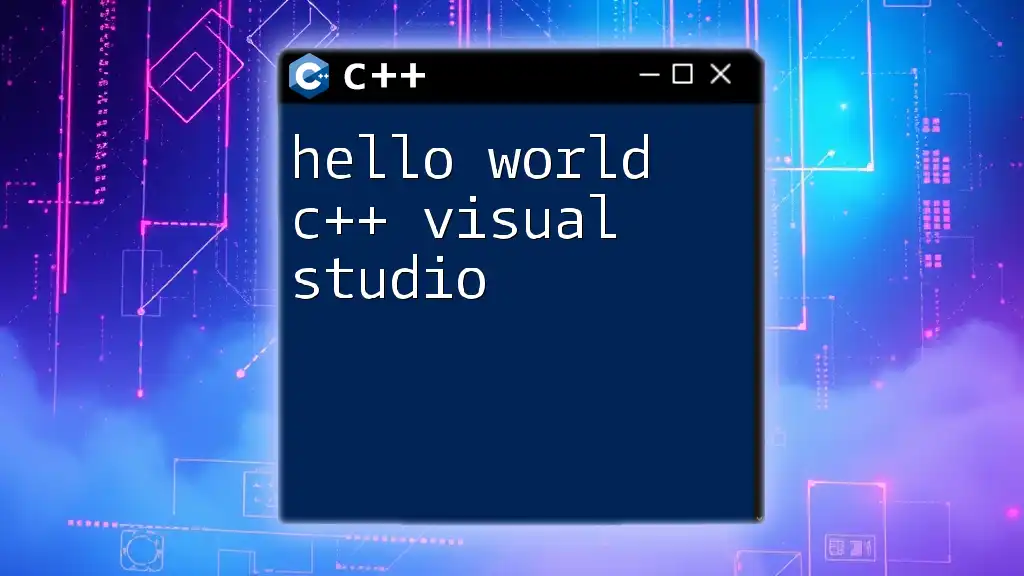
Additional Resources
Recommended Books and Online Resources
To further your C++ knowledge, consider exploring these well-regarded books and online platforms geared toward programming enthusiasts:
- "C++ Primer" by Stanley Lippman
- "Effective C++" by Scott Meyers
- Online courses on platforms like Udemy and Coursera
Further Reading
Dive deeper into specific C++ concepts with related articles focusing on advanced topics, coding tips, and best practices for efficient programming.
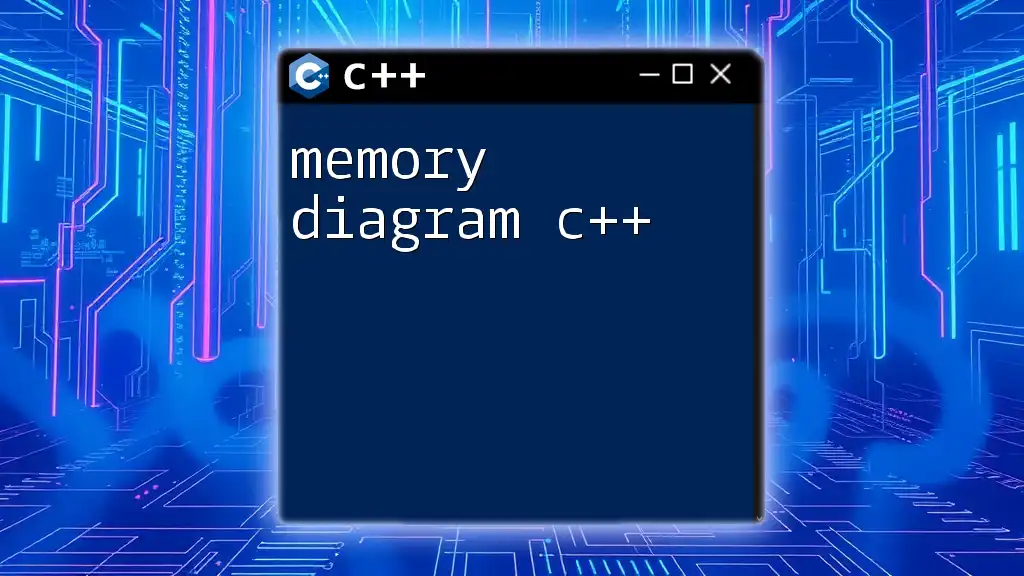
FAQ Section
Why do we start with "Hello, World!" in programming?
This tradition stems from its simplicity as a first program. It allows beginners to grasp fundamental programming concepts without overwhelming details.
Can I customize the message in the Hello World program?
Absolutely! You can change the string inside the output statement to any text of your choosing.
What are some common challenges when learning C++?
Common challenges include understanding memory management, mastering pointers and references, and navigating the complexities of C++ syntax and structure.