A memory diagram in C++ visually represents how different data types and objects are stored in memory, including stack and heap allocation.
Here’s a simple example demonstrating memory allocation in C++:
#include <iostream>
int main() {
int stackVar = 5; // Stored on the stack
int* heapVar = new int(10); // Stored on the heap
std::cout << "Stack variable: " << stackVar << "\n";
std::cout << "Heap variable: " << *heapVar << "\n";
delete heapVar; // Freeing heap memory
return 0;
}
Understanding Memory Layout in C++
The Basics of Memory Segments
C++ programs manage memory through two main areas: stack and heap. Understanding these areas is crucial to mastering memory management.
Stack Memory operates in a Last In, First Out (LIFO) manner. This means that variables allocated on the stack are automatically deallocated once their scope ends, making stack memory management efficient and easy. However, the size of stack memory is limited, and trying to allocate too much can lead to a stack overflow.
Conversely, Heap Memory allows for dynamic memory allocation. It provides a flexible way to allocate memory during runtime using operators like `new` and `delete`. While this flexibility is advantageous, it also brings responsibilities; developers must manually manage the memory to avoid issues like memory leaks and fragmentation.
Memory Segment Breakdown
Understanding where different types of data reside is critical for effective programming in C++. Here is a breakdown of the memory layout:
Code Segment: This is where your executable code resides. It is read-only and cannot be modified during the execution of your program, which helps prevent accidental modifications.
Global Segment: Also known as the data segment, this area stores global and static variables. These variables persist for the lifetime of the program, which means their values can be accessed anytime throughout the execution.
Heap Segment: This is where dynamically allocated memory lives. When you use `new` to allocate memory, it resides here. It persists until you use `delete` to free it, making careful management crucial to avoiding memory-related issues.
Stack Segment: The stack segment is reserved for function calls, including local variables and return addresses. Each time a function is called, a new stack frame is created, which includes its local variables. Once the function exits, this memory is released.
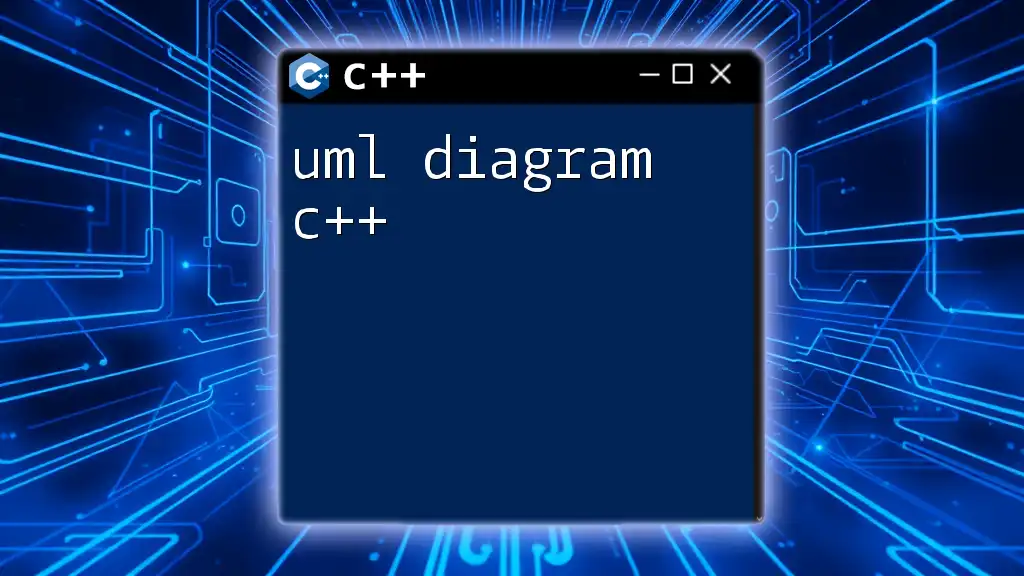
Visualizing Memory Diagrams
Introduction to Memory Diagrams
A memory diagram is a visual representation of how memory is laid out for a particular program. Understanding how variables are mapped within memory helps with debugging and optimizing code. Memory diagrams can simplify the grasp of complex interactions between different memory segments.
Creating a Simple Memory Diagram
Creating a memory diagram starts with defining the variables and understanding their lifetimes and scope. Let's consider the following code snippet:
int a = 10; // Local variable
int *b = new int; // Dynamic variable
static int c = 5; // Static variable
In this example:
- Variable `a` would be stored on the stack.
- Variable `b` points to a memory location on the heap as it represents a dynamically allocated integer.
- Variable `c` resides in the global segment as a static variable, accessible throughout the program.
When you draw the memory layout, it would resemble:
-
Stack:
| a | --> allocated automatically, deallocated at function exit
-
Heap:
| b | --> points to dynamically allocated space for an integer
-
Global Segment:
| c | --> static variable, persists for the program's entire lifetime
Example of a Memory Diagram
To illustrate further, consider the below example:
void exampleFunction() {
int localVar = 20;
int* dynamicVar = new int(30);
static int staticVar = 40;
}
In this function, we have:
- `localVar` on the stack — it will be automatically deleted when `exampleFunction` exits.
- `dynamicVar` points to an integer on the heap — you are responsible for freeing this memory.
- `staticVar` utilizes the global segment, remaining accessible across function calls.
A corresponding memory diagram would depict:
-
Stack:
| localVar | --> allocated and deallocated with function scope
-
Heap:
| dynamicVar | --> points to an integer in the heap, requires manual deallocation
-
Global Segment:
| staticVar | --> persists throughout the entire program execution
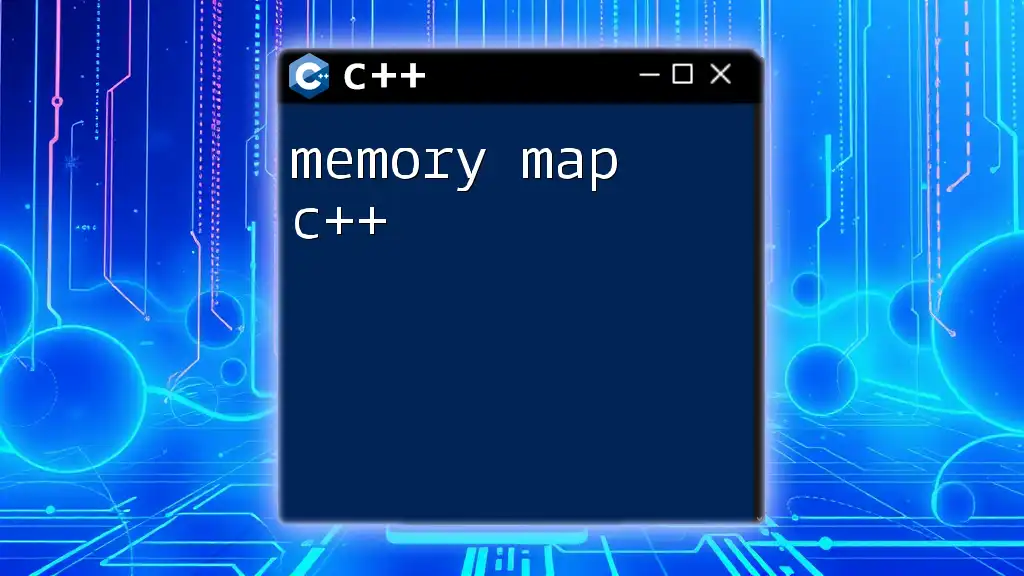
Common Memory Management Issues in C++
Memory Leaks
A memory leak occurs when allocated memory is not released back to the system. This often happens when you use `new` to allocate memory but fail to use `delete`. For example:
void memoryLeakExample() {
int* leak = new int(50);
// Forgot to delete
}
In the above code, `leak` consumes 4 bytes (or more, depending on the architecture), and since we do not delete it, it remains allocated even after the function exits, thus leaking memory.
Dangling Pointers
Dangling pointers arise when memory has been freed but the pointer still points to the back (freed memory). This can lead to undefined behavior if you attempt to access the memory afterwards. For example:
void danglingPointerExample() {
int* danglingPtr = new int(10);
delete danglingPtr;
// danglingPtr is now a dangling pointer
}
Using `danglingPtr` after it has been deleted can lead to crashes or corrupted data.
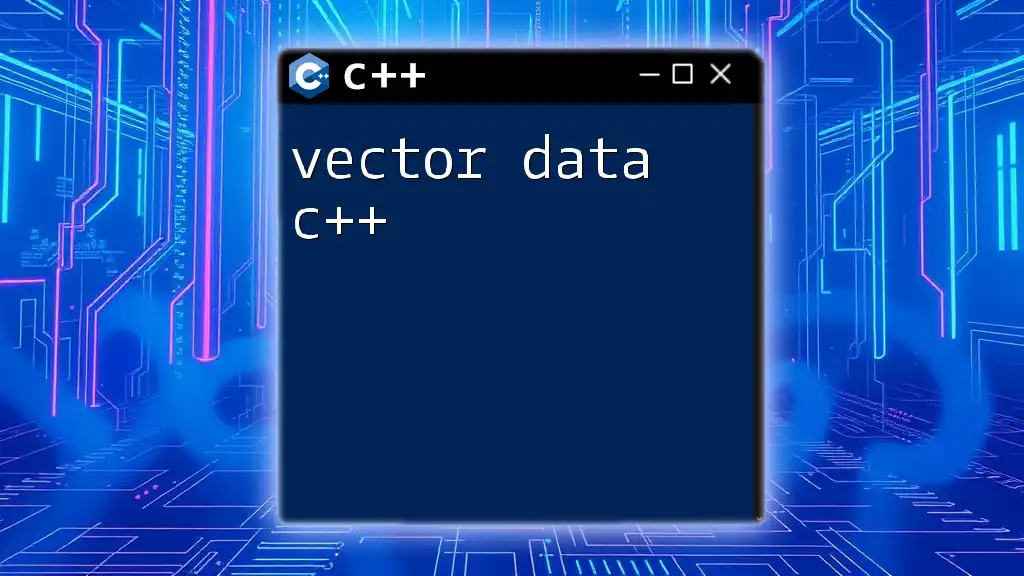
Best Practices for Memory Management
Proper Allocation and Deallocation
To avoid common pitfalls in memory management, consider using smart pointers provided by the C++ Standard Library. Smart pointers automate memory management, significantly reducing the risk of memory leaks and dangling pointers.
- `std::unique_ptr`: Automatically deletes the allocated memory when it goes out of scope.
- `std::shared_ptr`: Shares ownership of memory with other pointers and manages deallocation automatically when no references exist.
std::unique_ptr<int> smartPtr(new int(20));
RAII (Resource Acquisition Is Initialization)
RAII is a powerful principle that ties resource management to object lifetime. By ensuring that resources are acquired and released using constructors and destructors, you can effectively manage memory without resorting to manual calls for allocation and deallocation.
Debugging Memory Issues
To enhance your programming practices, utilize memory debugging tools such as Valgrind and AddressSanitizer. These tools can detect memory leaks, memory misuse, and corruption, thereby informing you of potential problems in your code.
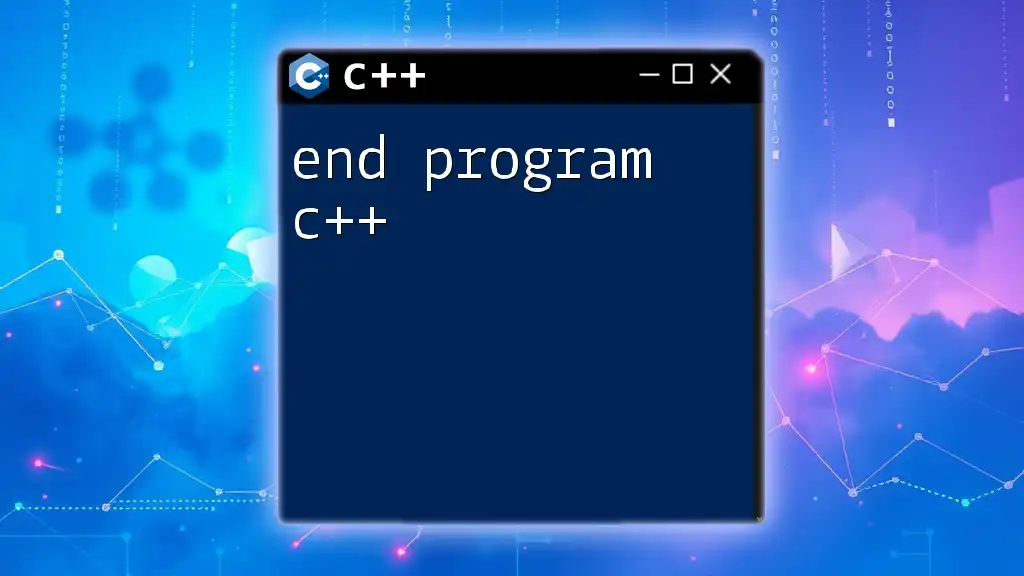
Conclusion
Understanding memory management in C++ is vital for crafting efficient, robust applications. By mastering memory diagrams, you can visualize how your variables interact within the memory layout, ultimately leading to improved performance and fewer bugs. Implementing best practices, like using smart pointers and RAII, combined with utilizing debugging tools, ensures that your C++ applications run smoothly while minimizing memory-related issues. Engage with this knowledge to elevate your programming skills and efficiency!