A memory map in C++ refers to a structured view of the memory used by a process, which can be helpful for understanding memory allocation and debugging issues related to memory usage.
Here's a simple code snippet illustrating how to create a memory map of a process:
#include <iostream>
#include <fstream>
#include <string>
void printMemoryMap() {
std::ifstream memFile("/proc/self/maps");
std::string line;
while (std::getline(memFile, line)) {
std::cout << line << std::endl;
}
}
int main() {
printMemoryMap();
return 0;
}
Understanding Memory Mapping
What is Memory Mapping?
Memory mapping is a crucial programming technique that enables programs to interact with files or devices as if they are part of the virtual memory space. This mechanism enhances both performance and ease of file manipulation within C++. Memory mapping allows for the efficient loading of files, which can then be accessed and manipulated directly in memory rather than through traditional file I/O operations.
One of the key advantages of memory mapping over traditional file operations is how it simplifies the reading and writing process. Instead of using explicit read and write commands, memory mapping treats file data like an array in memory, thereby allowing for faster data access and manipulation.
How Memory Mapping Works
Memory mapping operates through the principles of virtual memory. When a program needs to access a file, the operating system (OS) can allocate a portion of the virtual memory to represent that file. The OS manages the physical memory using page tables, enabling efficient data retrieval while minimizing disk I/O operations.
When a program attempts to access a mapped region that is not currently loaded into memory, a page fault occurs. The OS responds by loading the necessary data from disk into physical memory, making it available for the program. This behind-the-scenes operation is seamless, allowing developers to focus more on application logic rather than low-level memory management.
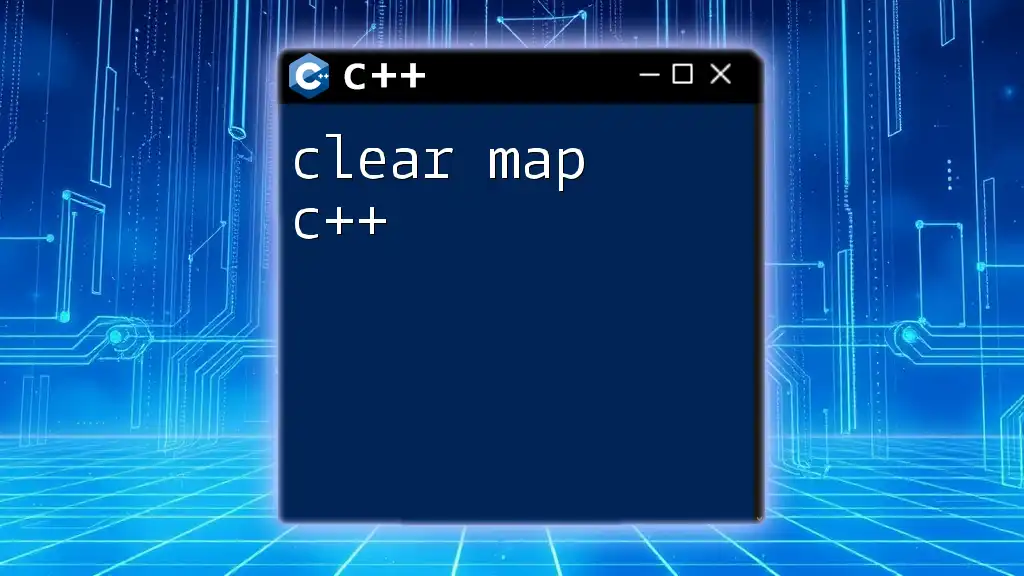
Why Use Memory Mapping in C++?
Performance Benefits
One of the primary reasons to use memory mapping in C++ is its performance. Traditional I/O operations may involve considerable overhead, requiring multiple system calls that can significantly slow down the execution of file-intensive applications. In contrast, memory mapping allows data to be accessed directly in memory with much lower latency.
Furthermore, large files can be accessed without loading them entirely into memory, which optimizes resource utilization. By mapping only the parts of a file that are needed at any given time, applications can handle substantial datasets efficiently—an essential feature in scenarios like database systems or data processing applications.
Convenience and Simplicity
Memory mapping simplifies code by eliminating the need for complex file handling procedures. Once a file is memory-mapped, operations like reading and writing become straightforward, resembling the manipulation of regular arrays or data structures. This is particularly useful for programmers who need to interact with large files without delving into cumbersome file management syntax.
Use Cases for Memory Mapping
Memory mapping is beneficial in several specific scenarios:
- Large data file processing: Applications such as databases benefit from memory mapping to efficiently manage data access without overwhelming system resources.
- Performance-critical applications: Game engines and real-time systems, where speed is essential, leverage memory mapping for rapid data access.
- Shared memory in multi-process applications: In scenarios where multiple processes need to read/write data concurrently, memory mapping can facilitate easy sharing and synchronization of that data.
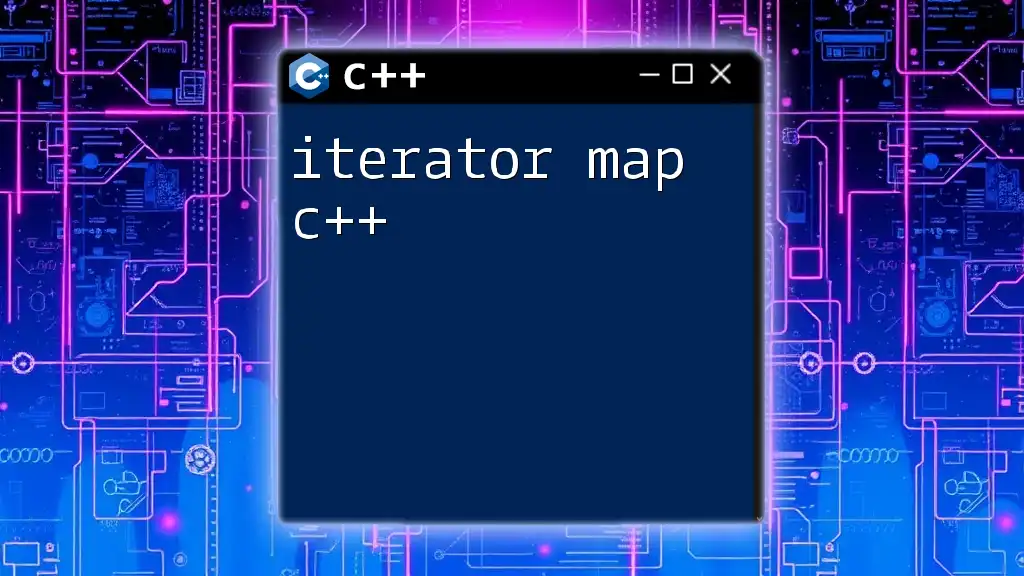
Memory Mapping Techniques in C++
Using the `mmap` Function
The Basics of `mmap`
The `mmap` function is a powerful C-style interface used to create memory mappings. It provides a mechanism to map files or devices into memory, allowing for direct access. Key parameters include:
- The address at which to start the mapping (usually `nullptr` for automatic choice)
- The length of the mapping
- Protection flags (e.g., `PROT_READ`, `PROT_WRITE`)
- Flags for sharing the mapping (e.g., `MAP_PRIVATE`, `MAP_SHARED`)
- The file descriptor of the target file
Code Example: Basic Memory Mapping
Here’s a basic example to demonstrate memory mapping with `mmap`:
#include <fcntl.h>
#include <sys/mman.h>
#include <sys/stat.h>
#include <unistd.h>
#include <iostream>
int main() {
// Open the file to be mapped
int fd = open("example.txt", O_RDONLY);
// Get the file size
struct stat sb;
fstat(fd, &sb);
size_t length = sb.st_size;
// Create a memory map
char *map = (char *)mmap(nullptr, length, PROT_READ, MAP_PRIVATE, fd, 0);
if (map == MAP_FAILED) {
std::cerr << "Mapping failed!" << std::endl;
return 1;
}
// Use the mapped memory
std::cout.write(map, length);
// Unmap and close the file
munmap(map, length);
close(fd);
return 0;
}
In this example, we open a file, create a memory-mapped region, and read its contents directly from memory. Once finished, it’s crucial to unmap and close the file to release resources.
Error Handling with `mmap`
Common Errors
Using `mmap` can lead to various errors, such as an invalid file descriptor or insufficient memory for mapping. Developers must handle these scenarios gracefully to prevent crashes or data corruption.
Implementing Error Checking
To ensure robust memory mapping, always check the return value of `mmap`. If it returns `MAP_FAILED`, it's essential to handle the error accordingly—such as logging it or attempting recovery.
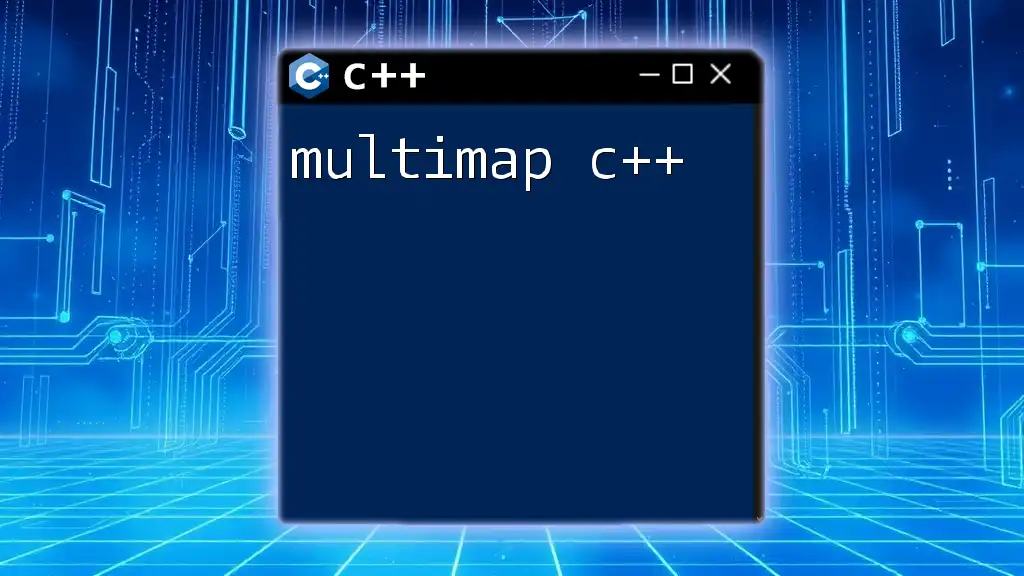
Advanced Memory Mapping Concepts
Using Memory Mapping with C++
Memory-Mapped Objects
Memory mapping in C++ extends beyond raw data access; it can also be used for creating objects within mapped memory. This leads to efficient large object handling without incurring the performance costs of copying data between buffers.
Example: Memory-Mapped Object Creation
This example showcases how to create an object in memory-mapped space:
// Define a struct for memory-mapped object
struct Data {
int id;
char name[256];
};
// Function to create a memory-mapped object
Data* createMmapObject(int fd, size_t length) {
return static_cast<Data*>(mmap(nullptr, length, PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0));
}
In this case, we define a `Data` structure, then map a file and utilize that region for object creation. The ability to use memory-mapped objects empowers developers to handle complex data structures efficiently.
Unmapping and Resource Management
Best Practices
Proper management of mapped memory is crucial. Always remember to call `munmap` once operations are complete. It’s a good idea to implement checks to ensure that the memory region is valid and that it has been previously mapped.
Memory Leaks and How to Avoid Them
Memory leaks can occur if mapped regions are not properly released. Employ tools like Valgrind to identify leaks during development. Establishing rigorous cleanup procedures, especially in complex applications where many mappings occur, is essential to maintain application health.
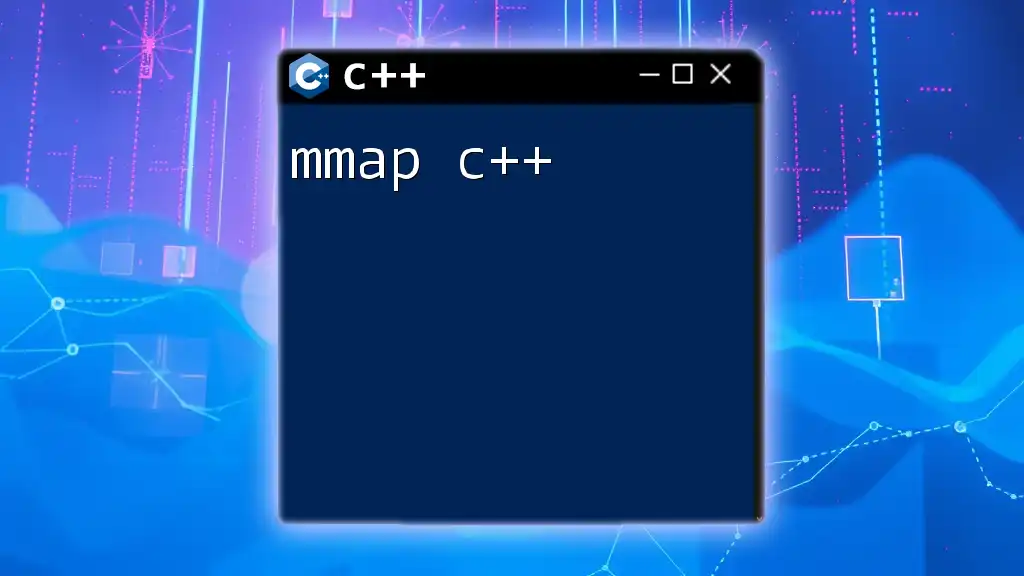
Conclusion
Recap of Memory Mapping in C++
Memory mapping in C++ is a powerful technique that enhances performance and simplifies file handling. With a clear understanding of how to implement `mmap`, handle errors, and leverage memory-mapped objects, developers can optimize applications that process large datasets efficiently.
Additional Resources and Further Reading
To deepen your understanding, consider exploring recommended books such as "The Linux Programming Interface" by Michael Kerrisk or online resources detailing advanced C++ memory management techniques. Participating in online courses focused on C++ may also provide valuable insights.
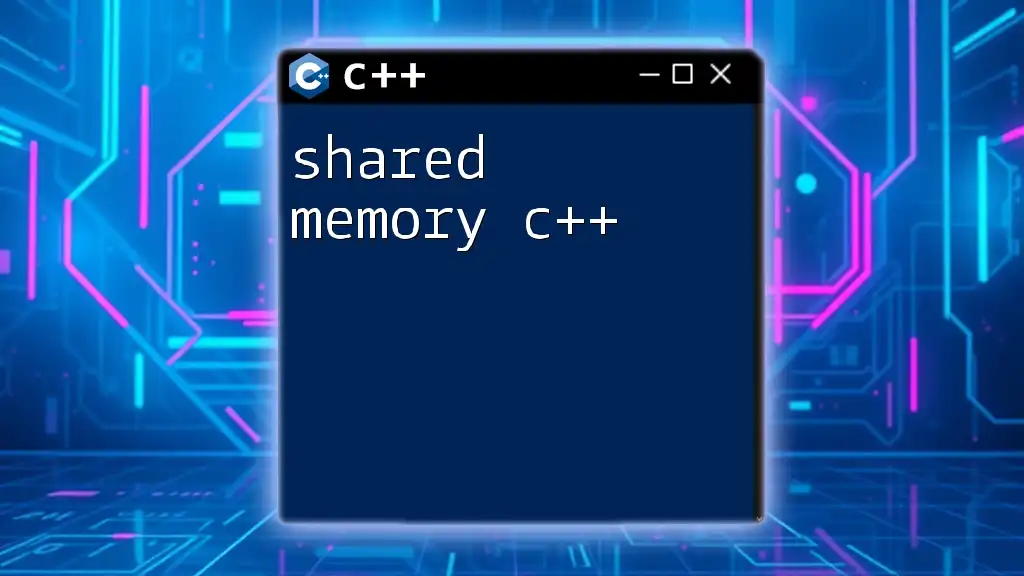
Call to Action
Don’t hesitate to dive into memory mapping in your projects! Experiment with the code samples provided, and consider joining our community for further insights and discussions on mastering C++. Share your experiences and challenges with memory mapping, and let’s learn together!