The "Hello World" example in C++ demonstrates the basic syntax of a C++ program by printing the phrase "Hello, World!" to the console. Here’s the code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful, high-level programming language that is widely used for developing system software, game development, and applications that require high performance. Developed in the early 1980s by Bjarne Stroustrup, C++ is an extension of the C programming language, incorporating object-oriented features, making it a versatile choice for many developers.
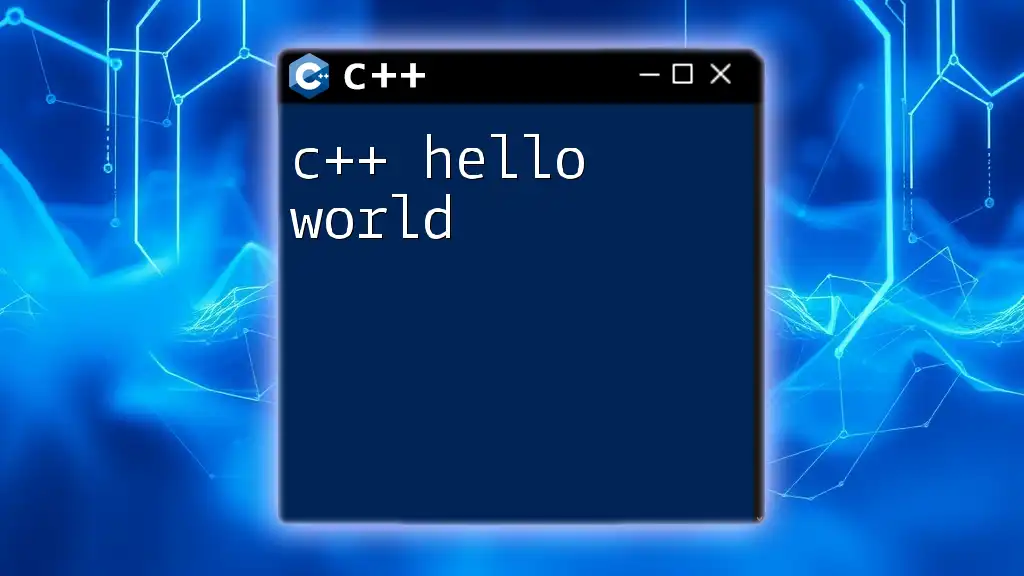
Why Learn C++?
Learning C++ can provide a solid foundation in programming concepts that are applicable in many other languages. C++ is utilized in a myriad of applications, including:
- Game Development: Many game engines, like Unreal Engine, are built using C++.
- Systems Programming: C++ is often chosen for operating systems due to its performance efficiency.
- Embedded Systems: C++ is used in applications that require direct interaction with hardware.
- Real-time Systems: C++ is optimal for applications that require real-time performance, thanks to its speed and control.
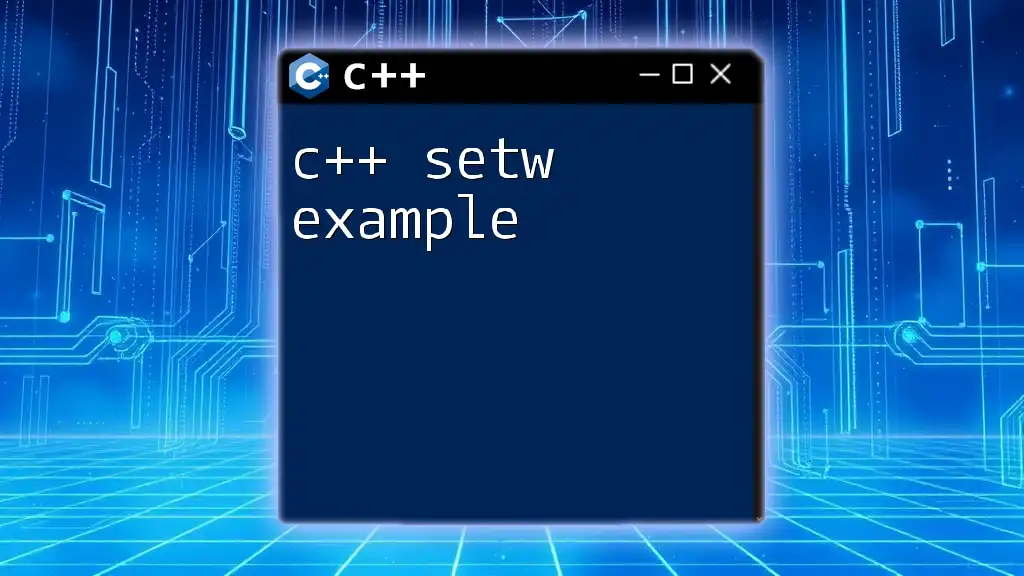
The Hello World Program: An Introduction
What is a "Hello World" Program?
The "Hello World" program is a simple program that outputs the text "Hello, World!" to the screen. Its simplicity makes it an essential first step for learning any programming language. It acts as a gateway into understanding how to write, compile, and run a program.
Why Use C++ for Hello World?
Using C++ for a Hello World example helps beginners understand the syntax and structure of C++ programs. The experience gained from writing this simple program lays down the groundwork for more complex programming constructs.
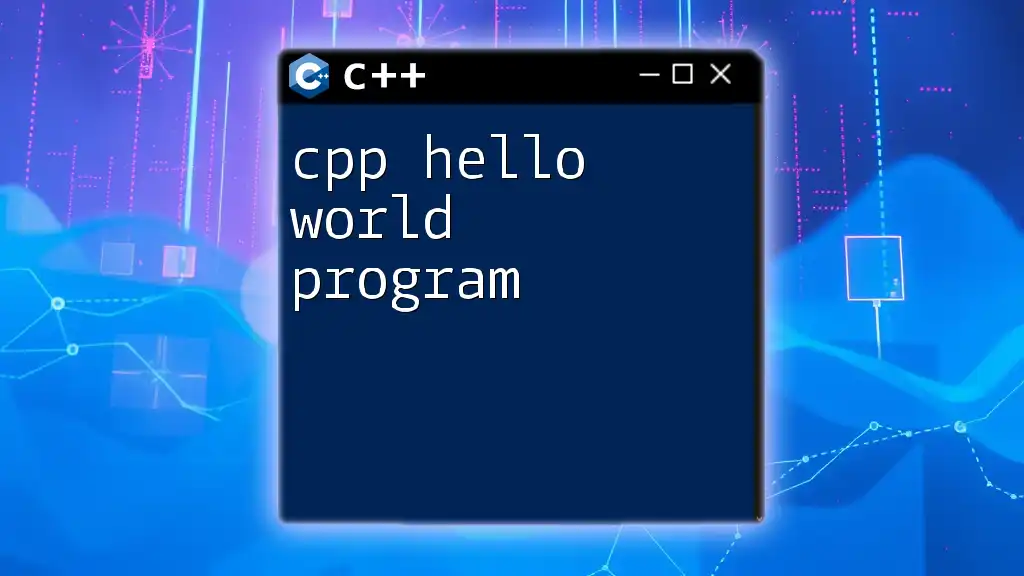
Getting Started with C++: Setting Up Your Environment
Choosing a Development Environment
To write C++ programs efficiently, it's essential to have the right development environment. Here are a few popular choices:
- Integrated Development Environments (IDE): Visual Studio, Code::Blocks, and Eclipse provide comprehensive tools and features that simplify writing and debugging code.
- Code Editors: Lightweight alternatives like Visual Studio Code or Sublime Text can be customized with extensions for C++ development.
Installing C++ Compiler
To compile and run C++ code, you need a C++ compiler. Here’s a simple guide for different platforms:
- Windows: You can install MinGW or use Microsoft Visual Studio to get the compiler included.
- Linux: GCC is a popular choice. Install it via your package manager (e.g., `sudo apt install g++`).
- macOS: You can install the Xcode Command Line Tools, which includes the Clang compiler, by running `xcode-select --install` in the terminal.
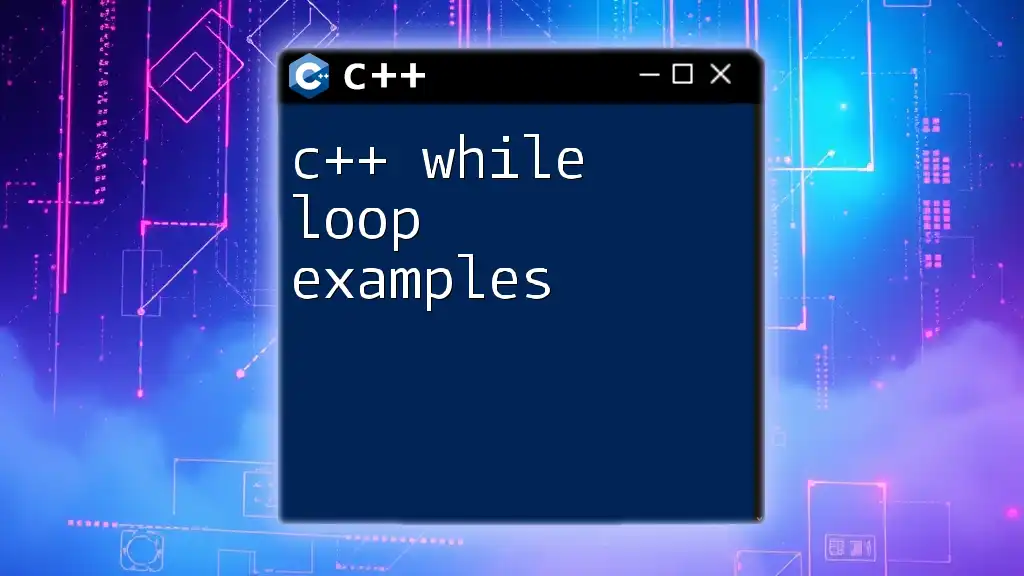
Writing Your First C++ Hello World Program
Structure of a C++ Program
Every C++ program has a certain structure, which includes:
- Headers: Libraries that provide access to necessary functionalities.
- Main Function: The entry point of the program, where execution begins.
- Return Statement: Indicates the successful completion of the program.
Hello World Code in C++
Here's a simple representation of the Hello World program written in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Breaking Down the Code:
- `#include <iostream>`: This line includes the input-output stream library, which allows the program to use `std::cout` for output.
- `int main()`: This defines the main function. Every C++ program must have a main function.
- `std::cout << "Hello, World!" << std::endl;`: This line outputs the string "Hello, World!" to the console. The `std::endl` part ensures that the output is followed by a newline character.
- `return 0;`: This indicates that the program has run successfully.
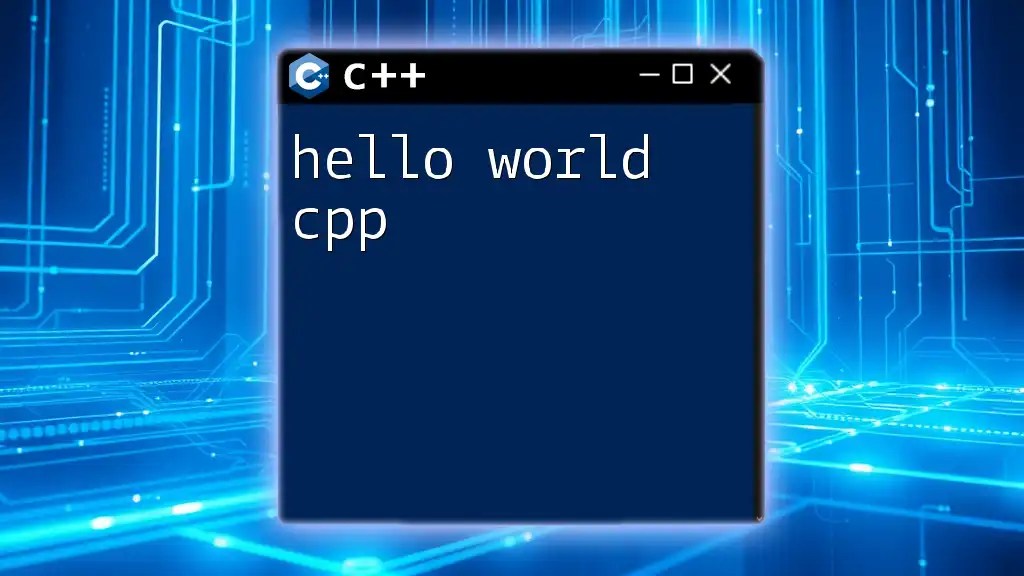
Running Your Hello World Program
Compiling the C++ Code
Once you've written your code, the next step is compiling it. The method can vary depending on your setup. For example:
- Command Line: If you're using GCC, navigate to your code's directory and compile it using:
g++ hello_world.cpp -o hello_world
- IDE: Most IDEs have a "Build" option which automatically compiles your code.
Executing Your Program
After compiling your program, you can run it. In the command line, simply type:
./hello_world
If using an IDE, it typically has a "Run" button to execute the program. Expect to see: "Hello, World!" displayed in the console.
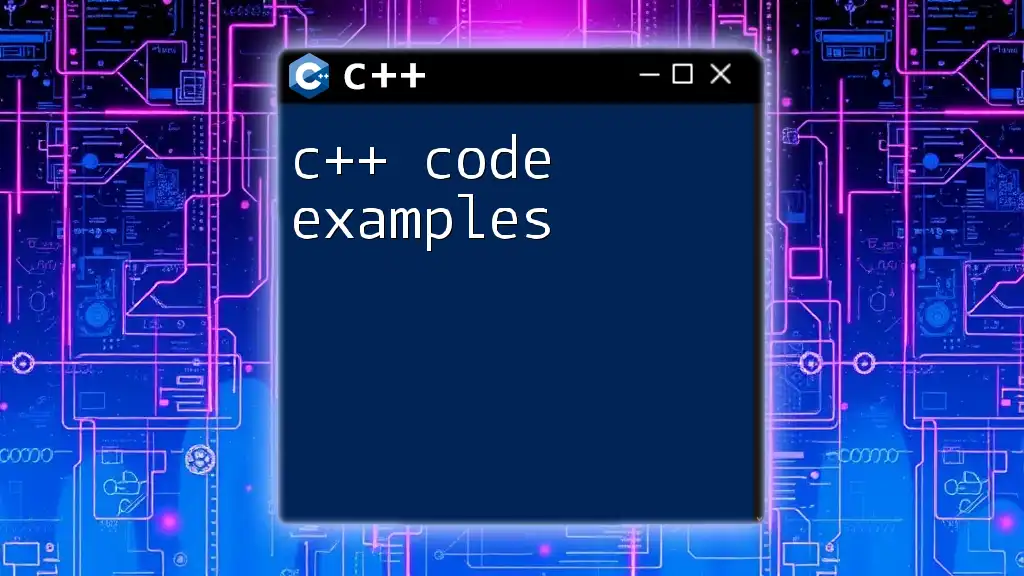
Common Errors and Troubleshooting
Basic Syntax Errors
As beginners, you'll likely face syntax errors while writing your code. Common mistakes include:
- Missing Semicolons: Every statement in C++ must end with a semicolon.
- Mismatched Braces: Make sure every opening brace `{` has a closing brace `}`.
Compiler Errors
Compiler errors can sometimes be more complex. Always pay attention to the error messages as they typically provide information about where the issue lies.
Tip: Search online for solutions to specific error messages you encounter; the developer community is often very helpful.
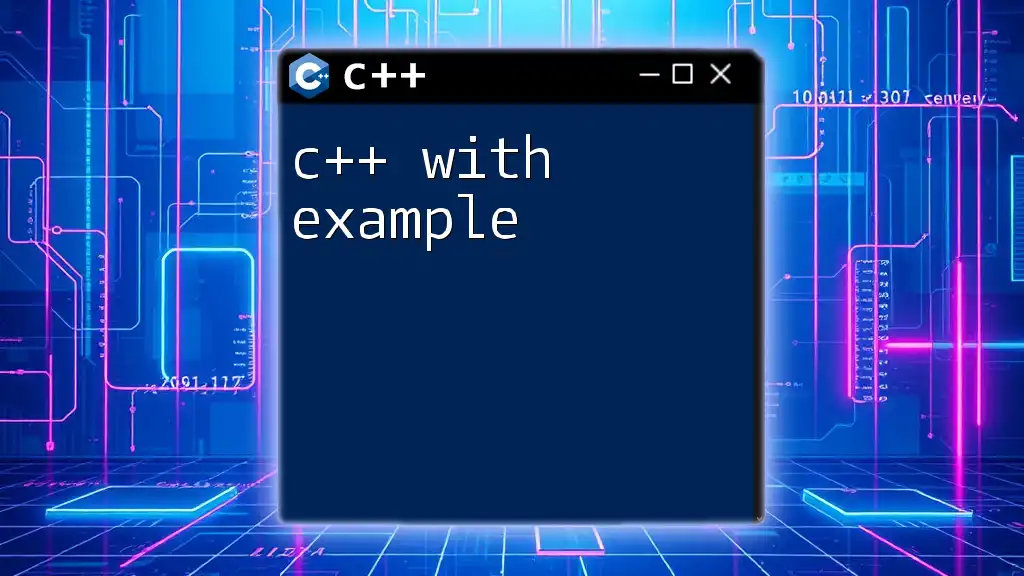
Exploring Variations of the Hello World Program
Using Different Output Methods
C++ allows for multiple methods to display output. Here's how you can use `printf` instead of `cout`:
#include <cstdio>
int main() {
printf("Hello, World!\n");
return 0;
}
In this code, `printf` is part of the C standard library, which can also be used within C++, though `std::cout` is generally preferred for C++ programs.
User-Defined Functions
Creating functions allows for modularity in your programs. Here’s how to define a function that prints "Hello, World!":
#include <iostream>
void greet() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
greet();
return 0;
}
Here, we’ve defined a function named `greet`. This encapsulates the printing logic, showcasing how you can break a program into manageable chunks.
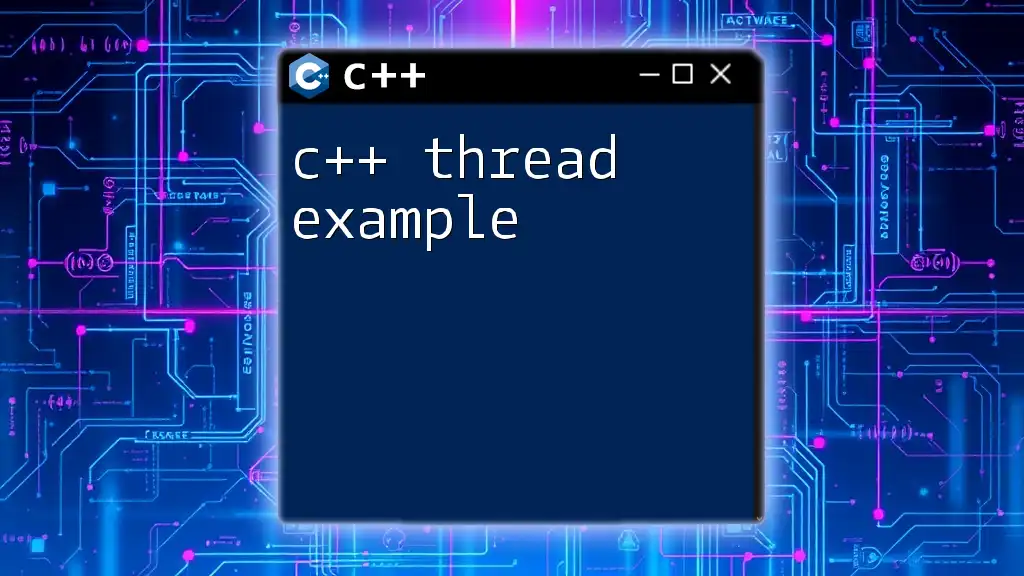
Conclusion
Mastering the basics of C++, like writing a Hello World program, is a paramount step in becoming a proficient programmer. This simple exercise not only introduces you to C++ syntax but also familiarizes you with the process of compiling and running programs.
Next Steps
Once you've written and run your Hello World program with confidence, consider delving into more complex topics such as data types, loops, and conditionals. The foundation you’ve built will prove invaluable as you explore the broader landscape of C++ programming.
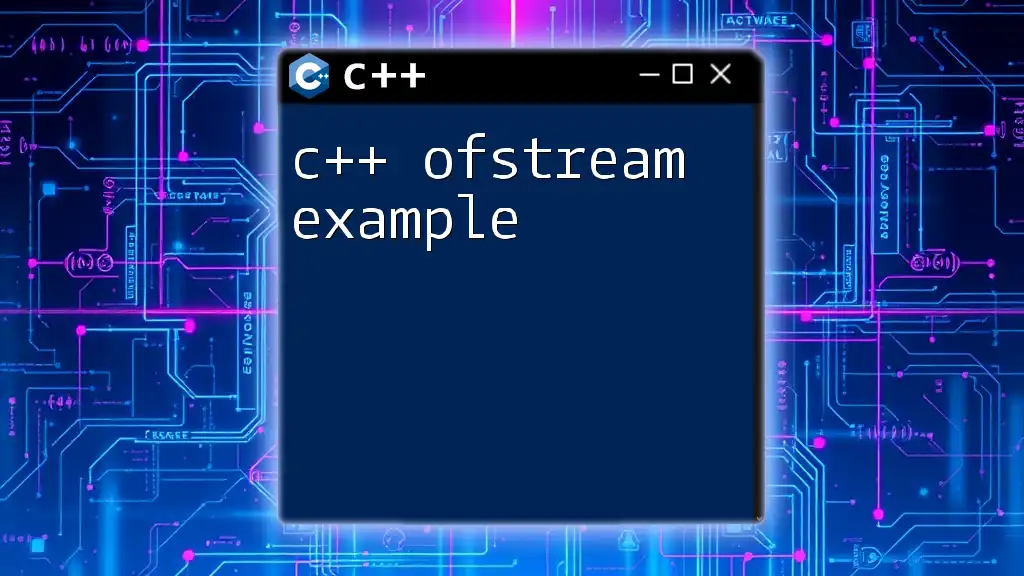
Additional Resources
Helpful Tools and Online Compilers
There are many platforms available for practicing C++ online, such as Replit, JDoodle, and Ideone. They offer instant compilation and execution, allowing you to experiment without setting up a local environment.
Further Reading and Learning Materials
For those eager to dive deeper into C++, consider reading books like "The C++ Programming Language" by Bjarne Stroustrup or taking online courses on platforms like Coursera or Udemy. These resources provide comprehensive education on this versatile programming language.
Final Thoughts
Embark on your C++ programming journey with enthusiasm! The Hello World example is just the beginning. With practice and perseverance, the powerful features of C++ await you, ready to be explored.