The "Hello, World!" program in C++ serves as a simple introduction to the language, demonstrating how to display text output to the console.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a Hello World Program?
A Hello World program is often the first step in learning any programming language. It is a simple program designed to demonstrate the basic syntax and structure of that language. Historically, it serves as a rite of passage for new programmers, introducing them to the essentials of coding, compiling, and running a program.
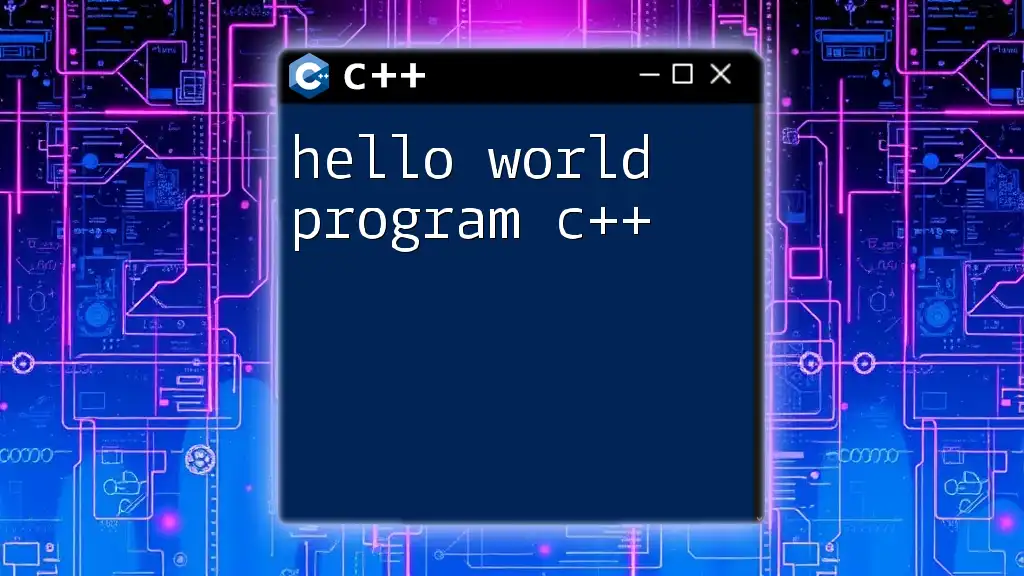
Setting Up Your C++ Development Environment
Choosing a Compiler
To effectively run a C++ Hello World program, you need a C++ compiler. Some popular options include:
- GCC (GNU Compiler Collection): A widely used compiler that works on various operating systems.
- Clang: Known for its modern features and performance.
- Microsoft Visual C++: A robust choice for Windows users.
Downloading and installing a compiler is straightforward. For instance, if you are on a Linux system, you can use the terminal:
sudo apt-get install g++
Setting Up an IDE or Text Editor
Choosing an Integrated Development Environment (IDE) or a text editor is crucial for writing your C++ code. Popular IDEs include:
- Code::Blocks: An open-source IDE that's user-friendly for beginners.
- Visual Studio: Comprehensive tools for Windows development.
- CLion: A commercial IDE with advanced features.
If you prefer a lightweight approach, consider text editors such as Sublime Text or Visual Studio Code. To set up Visual Studio Code, for instance, you can install the C++ extension from the Marketplace for enhanced functionality.
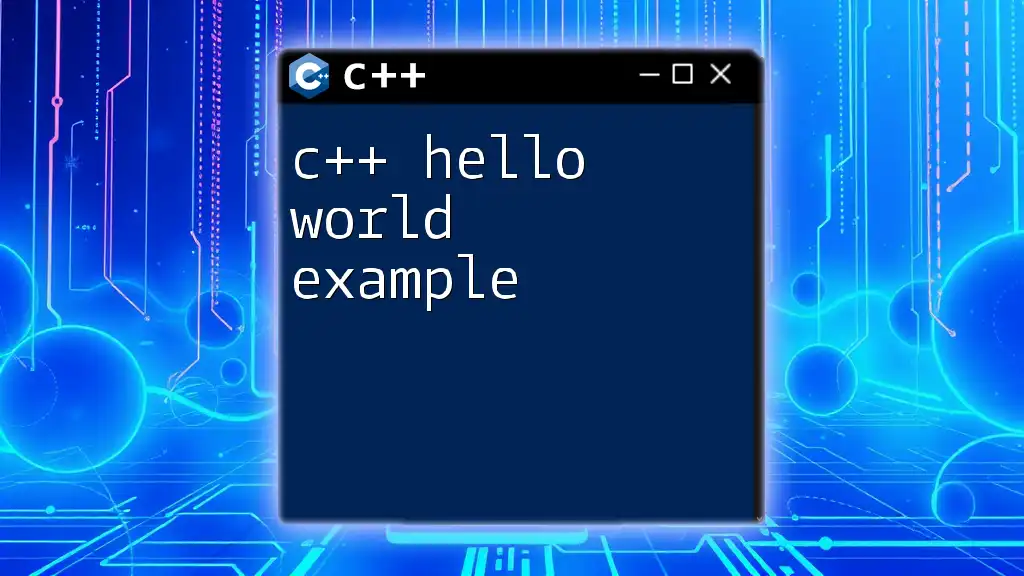
Writing a C++ Hello World Program
Basic Structure of a C++ Program
Every C++ program consists of several important components, including preprocessor directives, namespaces, and functions. Here’s the fundamental structure you'll encounter:
#include <iostream> // Preprocessor directive for input/output
using namespace std; // Using standard namespace
int main() { // Main function
cout << "Hello, World!"; // Output statement
return 0; // Exit status
}
Code Breakdown and Explanation
Let's examine each part of our first C++ Hello World program:
-
#include <iostream>: This directive tells the preprocessor to include the standard input-output stream library, essential for using the `cout` object, which handles output.
-
using namespace std;: This line allows us to use standard library features without prefixing them with `std::`, simplifying our code.
-
int main(): All C++ programs must have a `main` function, which acts as the entry point of execution. Everything within this block will run when the program starts.
-
cout << "Hello, World!";: The `cout` object is used to output data to the console. The `<<` operator directs the string "Hello, World!" to be displayed on the screen.
-
return 0;: This statement signifies successful completion of the program. By convention, returning a zero value indicates no errors occurred.
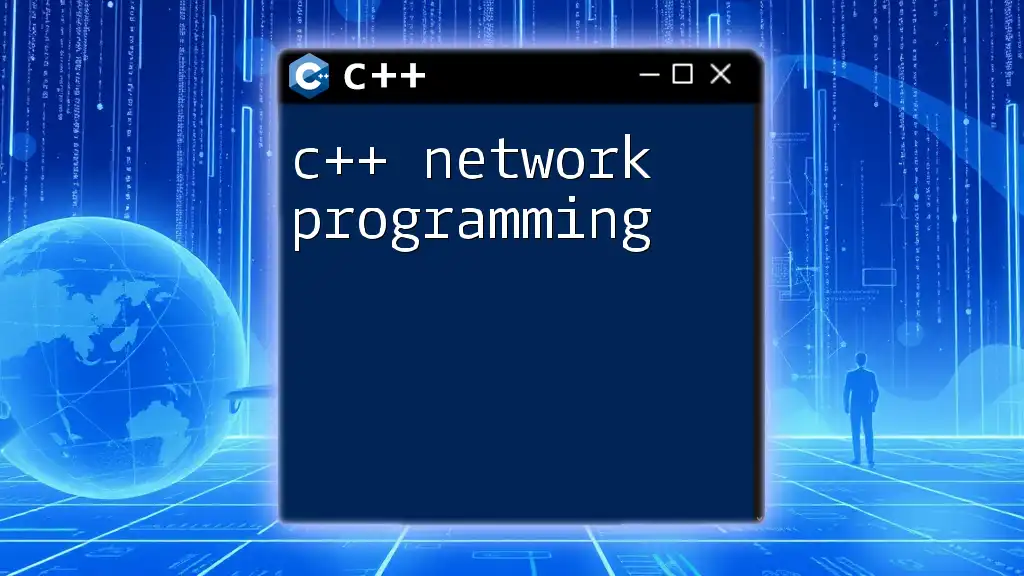
Compiling and Running Your C++ Hello World Program
Compiling the Program
Once your code is written, it must be compiled to convert it into an executable format. If you’re using the GCC compiler, you can do this from the command line:
g++ hello.cpp -o hello
In this command, `hello.cpp` is your source file, and `-o hello` specifies the output filename for the compiled program.
Running the Program
After compiling successfully, executing the program is straightforward. You can run it from the command line like this:
./hello
This command triggers the executable, and you should see "Hello, World!" printed on your screen.
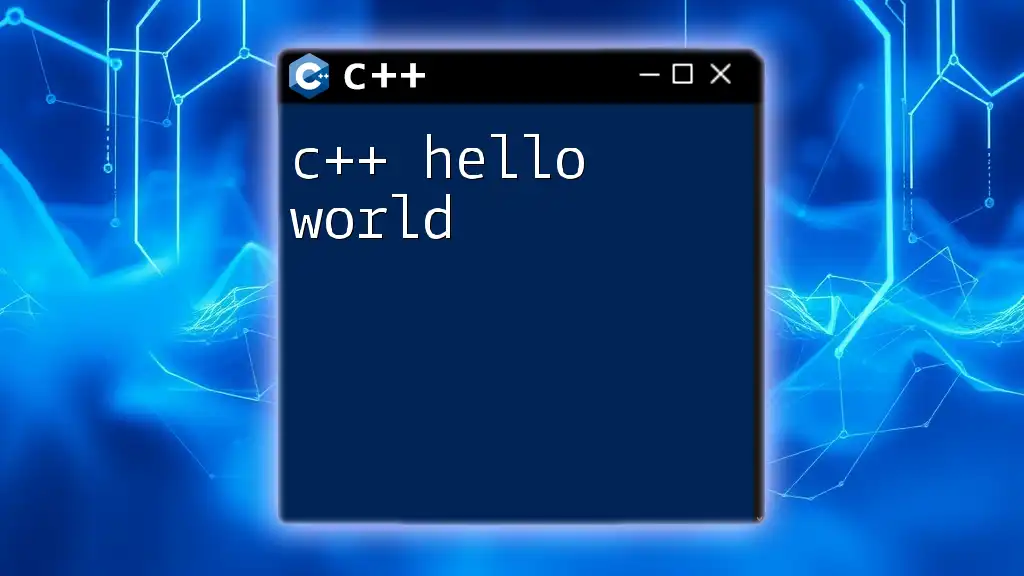
Common Errors and Troubleshooting
Compilation Errors
When compiling your C++ Hello World program, you may encounter various compilation errors. Common issues include:
- Syntax errors: Make sure every statement ends with a semicolon.
- Misspelled keywords: C++ is case-sensitive, so be careful with naming.
Resolving these issues often involves checking your code against the error messages provided by the compiler, which usually indicate the line number of the issue.
Runtime Errors
Runtime errors can occur if your program attempts illegal operations, such as dividing by zero. To troubleshoot these errors:
- Use debugging tools available in your IDE.
- Print variable values to the console to trace where the error arises.
Through careful debugging, most issues can be resolved.
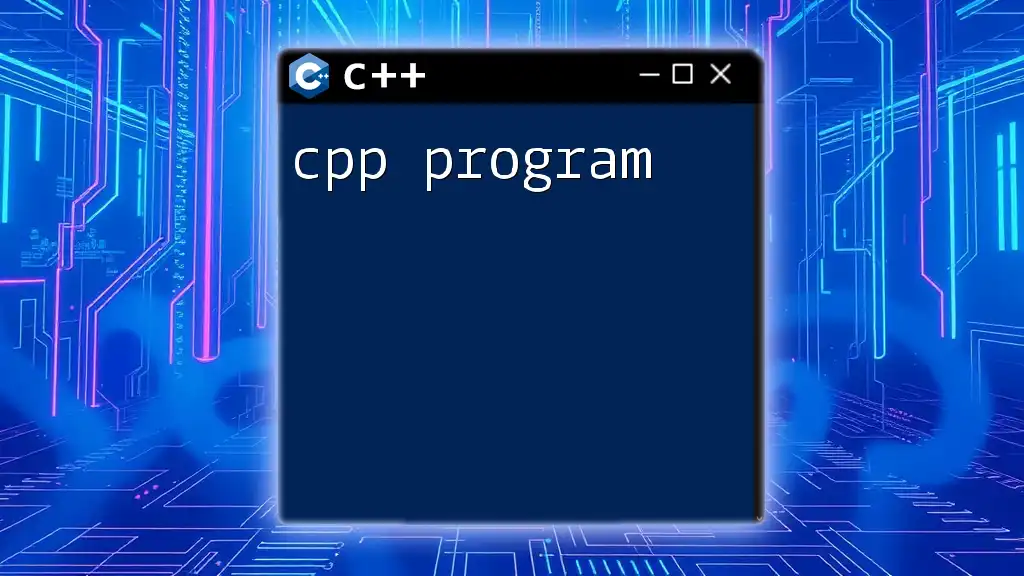
Conclusion
The C++ Hello World program serves as an essential introduction to the world of programming in C++. By writing and executing this simple program, you learn the fundamental concepts of syntax, compiling, and running code. From here, you can expand your knowledge and begin exploring more complex C++ programming constructs.
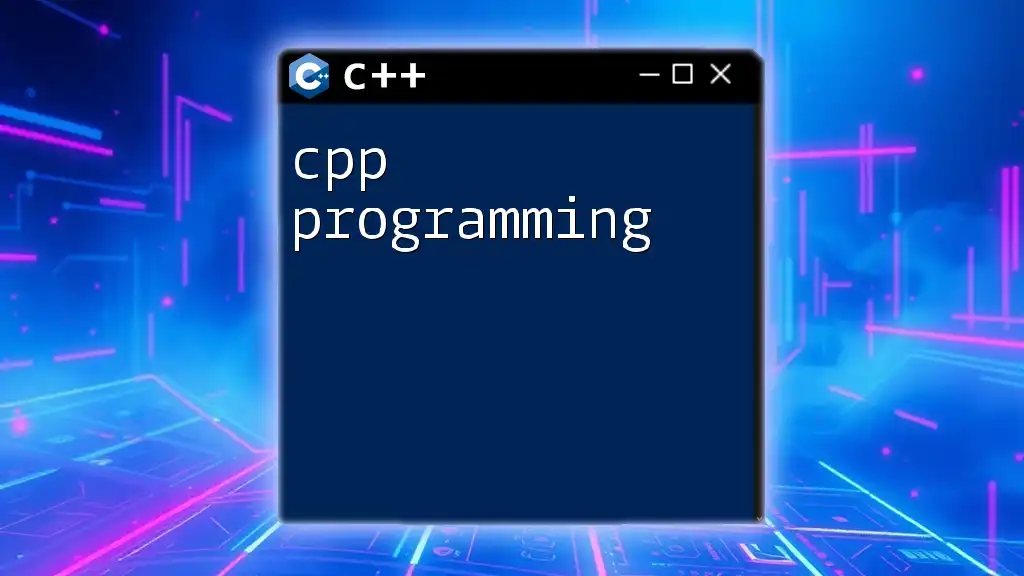
Frequently Asked Questions (FAQs)
-
What does the Hello World program teach?
- It introduces you to C++ syntax, flow of execution, and basic input/output operations.
-
Can I modify the Hello World program?
- Absolutely! Experimenting with modifications can teach you about syntax variations, formatting, and error handling.
-
Why is the Hello World program considered a rite of passage for programmers?
- It symbolizes the transition from theory to practice, demonstrating the essentials of creating, compiling, and running code.
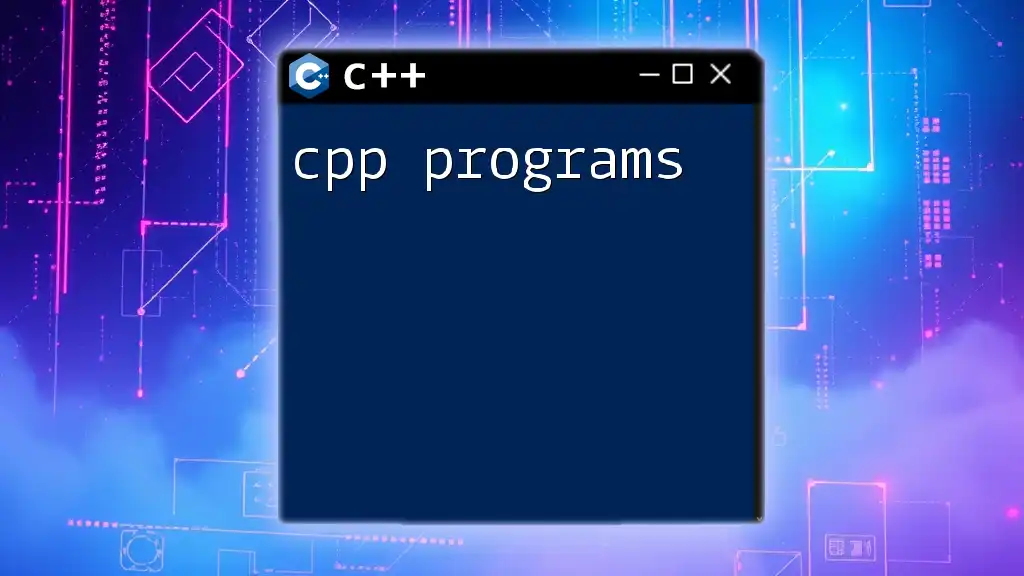
Additional Resources
To further enhance your learning, consider exploring tutorials on online platforms, joining community forums, and seeking books that delve deeper into C++. Engaging with these resources will help you build a solid foundation in C++ programming and prepare you for more advanced concepts.