"Qt 6 C++ GUI Programming Cookbook" is a practical guide designed to help developers learn efficient and effective coding techniques in C++ for creating graphical user interfaces using the Qt framework.
Here's a simple example of creating a basic Qt window:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, Qt!");
button.resize(200, 100);
button.show();
return app.exec();
}
Getting Started with Qt 6
What is Qt 6?
Qt 6 is a powerful and versatile multi-platform framework designed for developing graphical user interfaces (GUIs) using C++. Building on years of evolution from earlier versions, Qt 6 introduces enhanced features, more flexibility, and improved performance. Key highlights include a more modern C++ API, better support for high-DPI displays, and new modules that simplify the development process.
One of the standout advantages of Qt 6 is its ability to facilitate cross-platform applications. Whether your application is destined for Windows, macOS, or Linux, Qt provides the tools needed to create a seamless user experience across multiple operating environments.
Setting Up Your Environment
Installing Qt 6
To start developing with Qt 6, you first need to install the framework on your system. The installation process is similar across various platforms (Windows, macOS, Linux):
- Download the Qt online installer from the official [Qt website](https://www.qt.io/download).
- Run the installer and follow the prompts to select your desired components, including Qt 6 and Qt Creator IDE.
- Ensure to select the appropriate compiler for your operating system.
Creating Your First Project
Once the setup is complete, you can create your first Qt project. Qt Creator IDE offers an intuitive user interface to help streamline project creation.
- Open Qt Creator and click File > New File or Project.
- Select Application > Qt Widgets Application.
- Name your project, choose a location, and click Finish.
To verify your setup, create a simple "Hello, World!" application:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.resize(200, 100);
button.show();
return app.exec();
}
Running this code creates a window with a button that displays "Hello, World!" This serves as a simple introduction to creating GUI applications with Qt 6.
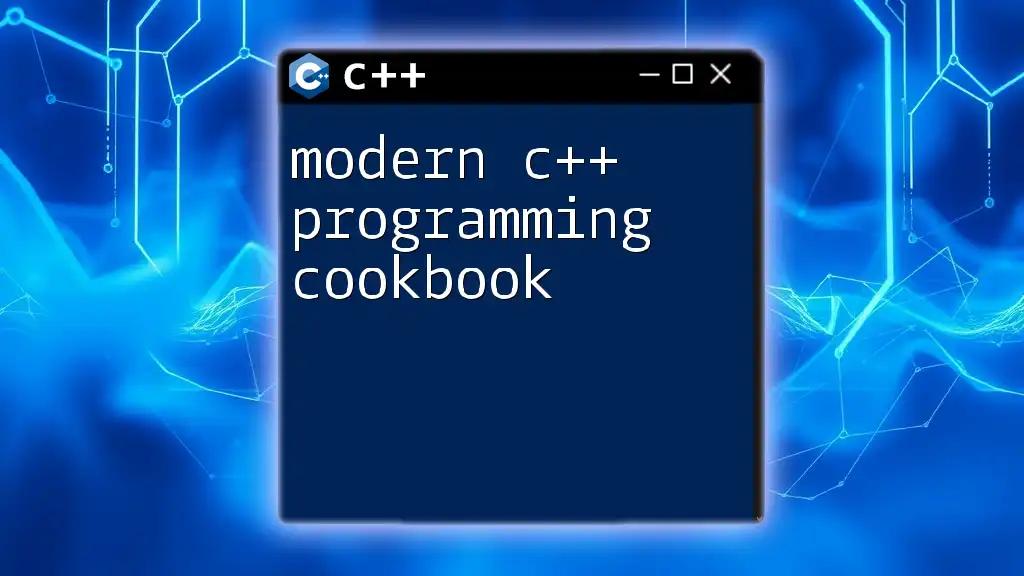
Understanding the Qt Framework
Components of Qt
Qt comprises several essential components that allow developers to create rich interfaces:
- Widgets: Basic elements such as buttons, labels, text boxes, and more. They are the building blocks of any GUI application.
- Layouts: Manage the arrangement of widgets within your application, making it responsive to user interactions and screen sizes.
- Signals & Slots: A powerful mechanism for communication between objects. This ability to link events with reactions is foundational to reactive programming in Qt.
- QML: A language designed for creating fluid user interfaces with an emphasis on declarative design.
Detailed Look at Widgets
Common Widgets in Qt
Widgets are at the heart of any Qt application. Here are a few commonly used widgets along with example code snippets:
- QPushButton: A button that can be clicked to trigger an action.
QPushButton *button = new QPushButton("Click Me");
- QLabel: A simple display widget used for showing text or images.
QLabel *label = new QLabel("This is a label");
- QLineEdit: A single-line text input field.
QLineEdit *lineEdit = new QLineEdit();
These widgets can be easily incorporated into layouts to create a visually appealing interface.
Utilizing Layouts
Types of Layouts (QHBoxLayout, QVBoxLayout, QGridLayout)
Layouts are vital for arranging widgets in your application window. Here’s a brief overview:
- QHBoxLayout: Arranges widgets horizontally.
- QVBoxLayout: Arranges widgets vertically.
- QGridLayout: Places widgets in a 2D grid.
Using layouts avoids hard-coding widget positions, which enhances responsiveness. An example using QHBoxLayout is shown below:
QHBoxLayout *layout = new QHBoxLayout();
layout->addWidget(label);
layout->addWidget(lineEdit);
layout->addWidget(button);
Applying layouts helps ensure your application adapts well to various window sizes, enhancing its usability.
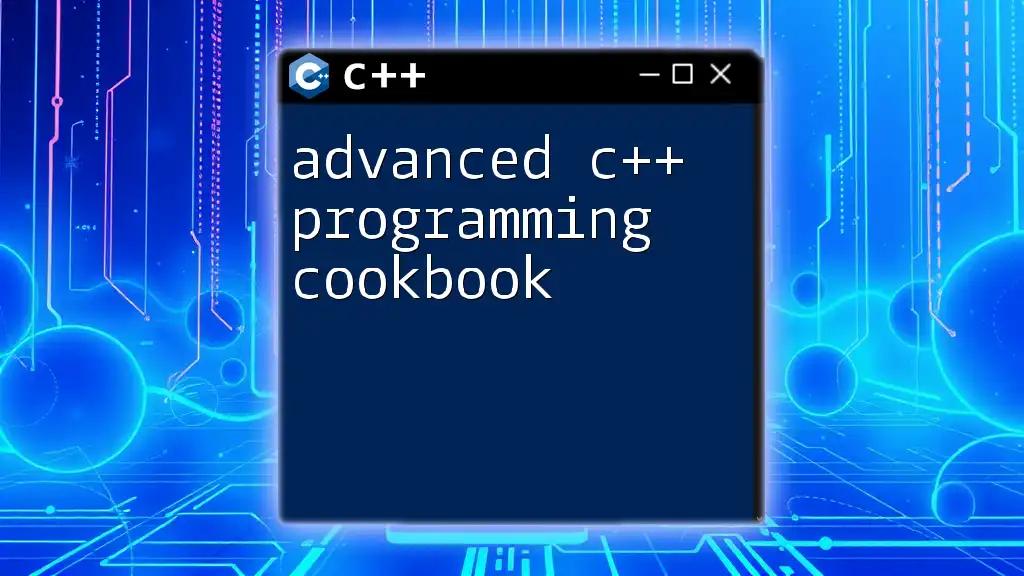
Advanced GUI Programming Concepts
Custom Widgets and Painting
Creating custom widgets allows you to tailor your application’s interface to your needs. To create a custom widget, you need to derive a class from `QWidget` and override its `paintEvent` method for custom drawing.
class MyCustomWidget : public QWidget {
protected:
void paintEvent(QPaintEvent *event) override {
QPainter painter(this);
painter.setBrush(Qt::blue);
painter.drawRect(rect());
}
};
This example creates a simple blue rectangle in the custom widget. You can expand this further by adding more intricate drawing logic or integrating with existing widgets.
Managing Events
Event management is crucial for responsive applications. Qt allows you to handle events effectively through event handlers.
For instance, to interact with key presses, you can override the `keyPressEvent` method:
void MyWidget::keyPressEvent(QKeyEvent *event) {
if (event->key() == Qt::Key_Space) {
// Your code here for space key action
}
}
This code snippet allows you to perform specific actions whenever the space key is pressed, demonstrating how to handle user input elegantly.
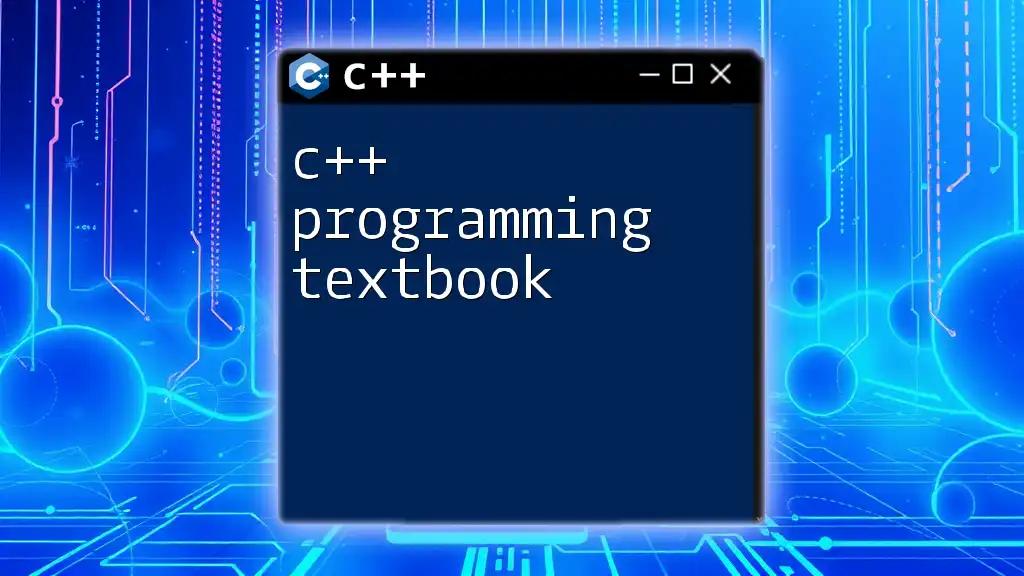
Application Architecture
Model-View-Controller (MVC) Pattern
Qt employs the Model-View-Controller (MVC) pattern, which separates application logic into three interconnected components.
- Model: Manages the data and business logic.
- View: Responsible for the graphical representation of the model.
- Controller: Interacts with the model to update its state based on user actions.
This separation enhances maintainability and facilitates unit testing, making it a preferred approach for large applications.
Multithreading in Qt
Efficient applications often require concurrent processing. Qt offers a simple way to implement multithreading using QThread.
class WorkerThread : public QThread {
void run() override {
// Perform time-consuming task
}
};
By using QThread, you can offload intensive operations to separate threads, ensuring that your GUI remains responsive even during long-running tasks.
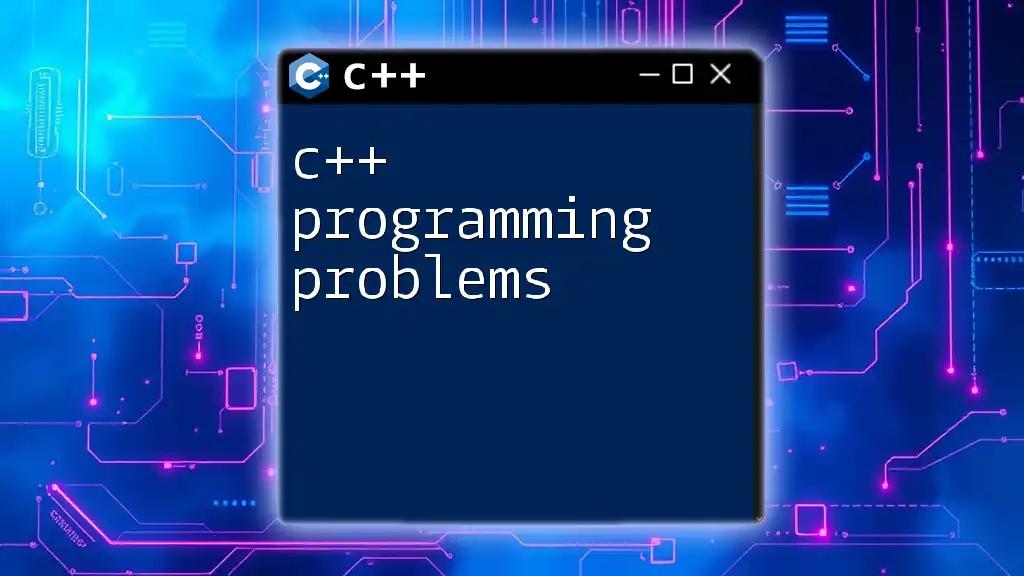
Finalizing Your Application
Debugging and Testing
Debugging is a critical step in software development. Qt Creator includes robust debugging tools that you can utilize by setting breakpoints and step-through debugging to isolate issues.
Additionally, you can employ the QTest framework to write unit tests for your application:
#include <QTest>
class MyTest : public QObject {
Q_OBJECT
private slots:
void testExample() {
QVERIFY(true); // Replace with actual test conditions
}
};
Testing helps ensure the reliability and stability of your application before deployment.
Packaging Your Application
Once development and testing are complete, packaging is the final step. Qt provides tools such as `windeployqt`, `macdeployqt`, and `linuxdeployqt` to streamline the process of creating deployable versions of your application.
Creating installers enhances user experience and allows for easy distribution. Remember to test your application on different platforms to verify compatibility before distribution.
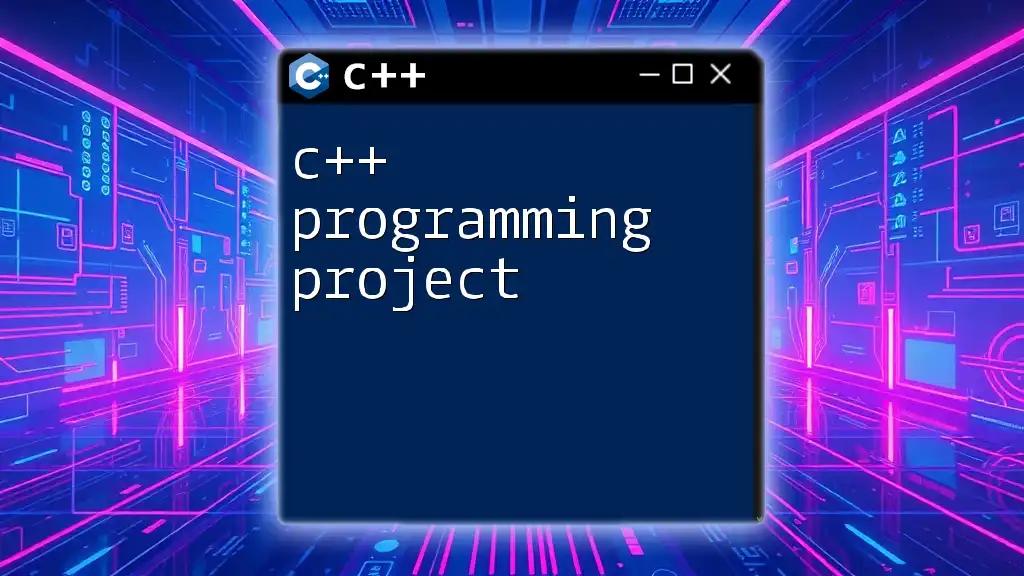
Additional Resources
Recommended Books and Online Courses
Aside from the Qt 6 C++ GUI Programming Cookbook ePub, there are several resources for expanding your knowledge:
- C++ GUI Programming with Qt 6 - A comprehensive guide that provides practical examples and detailed explanations.
- Udemy and Coursera - Online courses featuring both free and paid tutorials from industry experts.
Community and Support
Joining the Qt community can provide invaluable support. You can engage with others through:
- Official documentation available on the [Qt website](https://doc.qt.io).
- Forums and mailing lists like Qt Centre or Stack Overflow.
- Local meetups and virtual conferences that bring developers together.
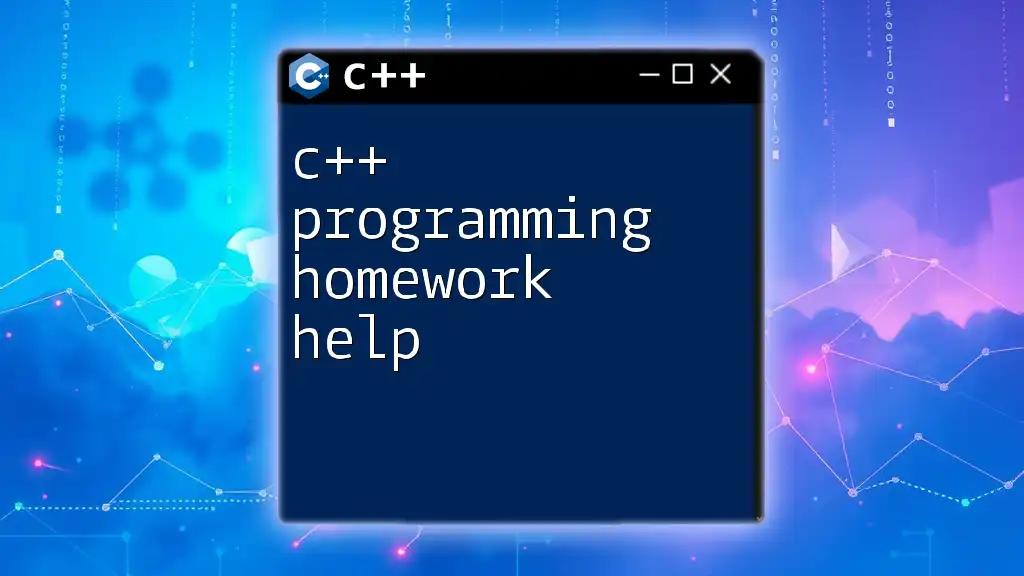
Conclusion
In summary, mastering Qt 6 for C++ GUI programming opens a world of possibilities for developing powerful and engaging applications. By leveraging its extensive libraries and framework capabilities, you can create user interfaces that are not only functional but also visually appealing.
Explore the potential of your coding skills, dive into the Qt 6 C++ GUI Programming Cookbook ePub, and start building your next great application today!