Heapify in C++ is a process that rearranges a binary tree to satisfy the heap property, transforming it into a max-heap or min-heap.
Here's a simple example of a max-heapify function in C++:
#include <iostream>
using namespace std;
void maxHeapify(int arr[], int n, int i) {
int largest = i; // Initialize largest as root
int left = 2 * i + 1; // left child
int right = 2 * i + 2; // right child
if (left < n && arr[left] > arr[largest])
largest = left;
if (right < n && arr[right] > arr[largest])
largest = right;
if (largest != i) {
swap(arr[i], arr[largest]);
maxHeapify(arr, n, largest);
}
}
int main() {
int arr[] = {3, 9, 2, 1, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
maxHeapify(arr, n, 0);
cout << "After heapifying: ";
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
return 0;
}
What is Heapify?
Heapify is a crucial operation in computer science and programming that transforms an unordered array into a heap structure. A heap is a special tree-based data structure that satisfies the heap property: in a max-heap, every parent node is greater than or equal to its child nodes, whereas in a min-heap, every parent node is less than or equal to its child nodes. Understanding how to implement heapify is pivotal because it lays the foundation for advanced algorithms, particularly heap sort and priority queues.
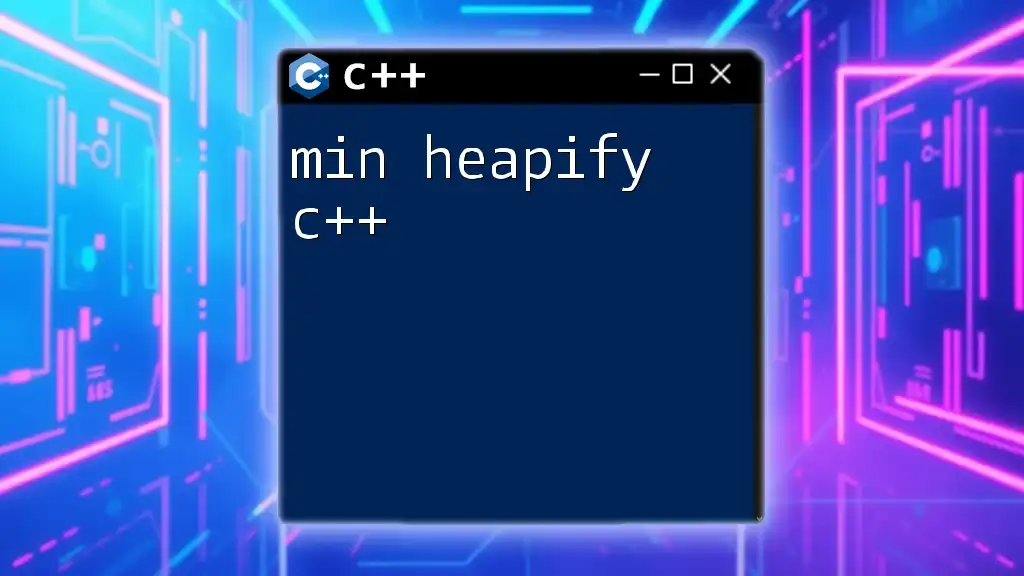
Why Use Heapify in C++?
Utilizing heapify in C++ comes with several advantages:
- Efficiency: Heap operations run in logarithmic time, making them ideal for applications needing quick access and modification.
- Dynamic Data Handling: Heaps can efficiently manage dynamic datasets where elements need frequent insertion and deletion.
- Widely Applicable: Used in algorithms like heap sort and in data structures like priority queues, making it versatile in various applications.
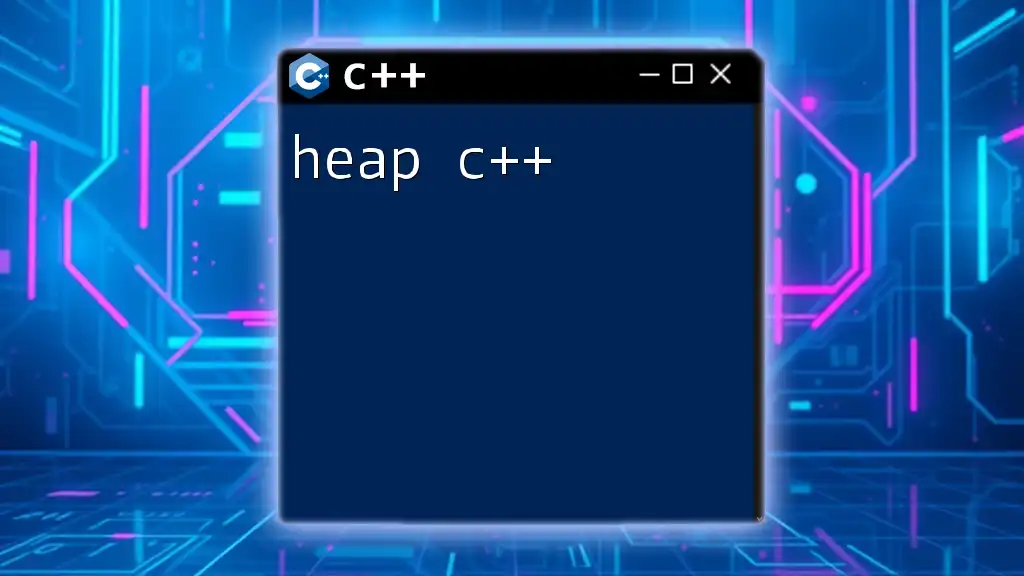
Understanding Heaps in C++
Types of Heaps
Heaps can be categorized into two main types:
-
Min-Heap: The smallest element is at the root, and every parent node is less than its children. This structure is useful when you want to extract the minimum element efficiently.
-
Max-Heap: Conversely, the largest element is at the root, with every parent node being greater than or equal to its children. This is useful for algorithms that need the largest values efficiently.
Heap Structure in C++
In C++, heaps are commonly represented as arrays. The relationships between the elements are defined by their indices:
- Parent node: For any node at index `i`, its parent can be found at index `(i - 1) / 2`.
- Left child: The left child of the node at index `i` is located at index `2 * i + 1`.
- Right child: The right child can be found at index `2 * i + 2`.
This hierarchical representation allows us to utilize array indexing to manage the heap structure effectively.
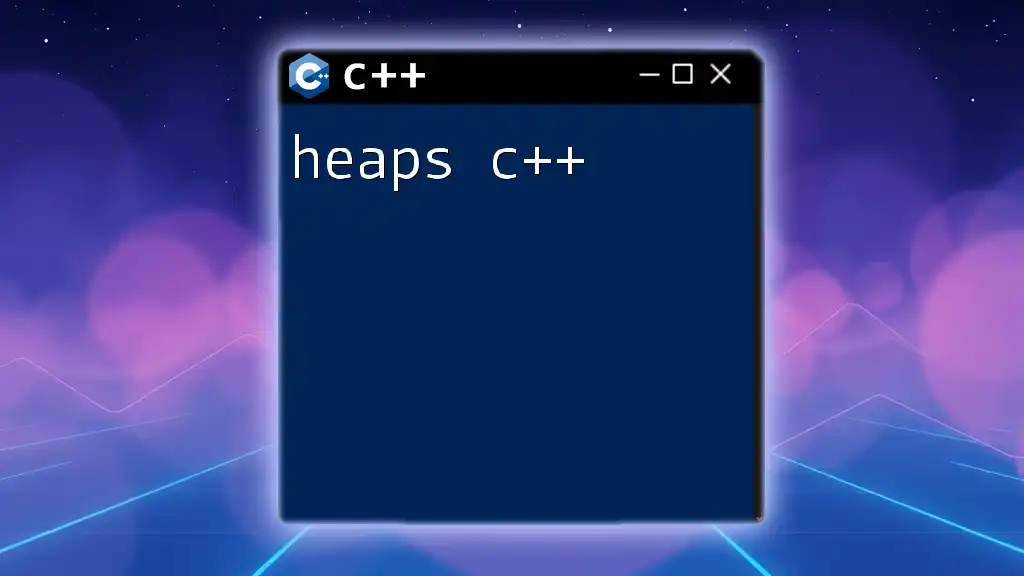
How Heapify Works
Heapify Process Explained
The heapify process involves arranging an array into the proper heap order. The method typically involves recursive calls to ensure that each subtree adheres to heap properties. The process can begin from any node and adjust as necessary.
Key Operations
Heapify typically encompasses two key operations:
-
Insert: When a new element is added, it must be compared with its parent and potentially swapped to maintain the heap property.
-
Remove: Removing an element, particularly the root, also requires restructuring the heap to maintain its properties.
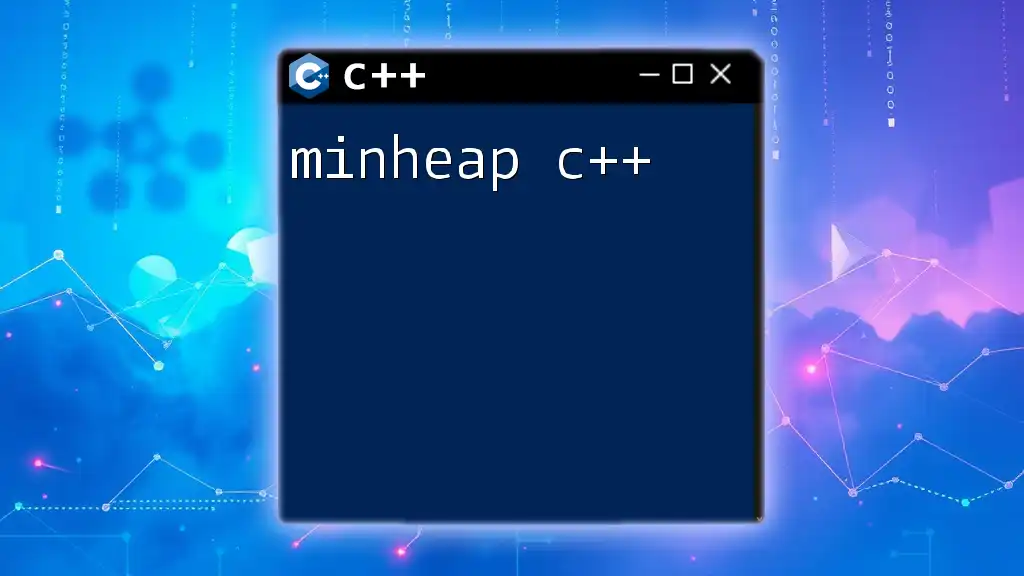
Implementing Heapify in C++
Basic Code Structure for Heapify Function
To demonstrate how the heapify operation is implemented, consider the following C++ function for max-heap:
void heapify(int arr[], int n, int i) {
int largest = i; // Initialize largest as root
int left = 2 * i + 1; // left = 2*i + 1
int right = 2 * i + 2; // right = 2*i + 2
// Check if left child exists and is greater than root
if (left < n && arr[left] > arr[largest])
largest = left;
// Check if right child exists and is greater than largest so far
if (right < n && arr[right] > arr[largest])
largest = right;
// If largest is not root
if (largest != i) {
swap(arr[i], arr[largest]);
// Recursively heapify the affected sub-tree
heapify(arr, n, largest);
}
}
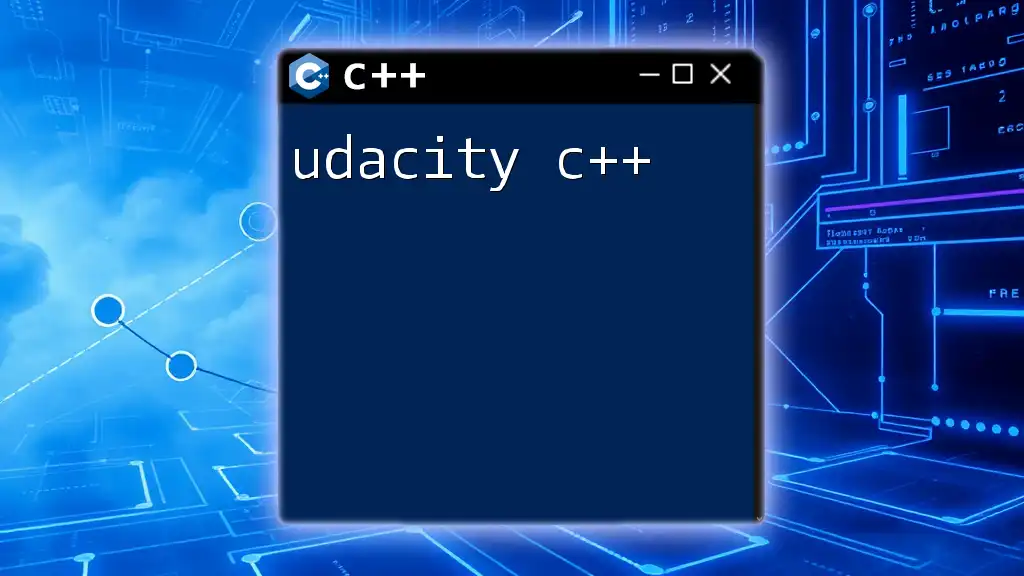
Performing Heapify on an Array
Step-by-Step Guide to Heapifying an Array
To heapify an entire array, we typically start from the last non-leaf node and apply the heapify function to each node from bottom to top, left to right.
Example of Heapifying an Unordered Array
Here is a complete example of how to heapify an entire array in C++:
void buildMaxHeap(int arr[], int n) {
// Start from the last node that is not a leaf
for (int i = n / 2 - 1; i >= 0; i--)
heapify(arr, n, i);
}
// Example of using `buildMaxHeap`
int main() {
int arr[] = {3, 9, 2, 1, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
buildMaxHeap(arr, n);
// Output the heap
}
Building a max heap ensures that the largest value is accessible at the root, paving the way for efficient sorting and retrieval operations.
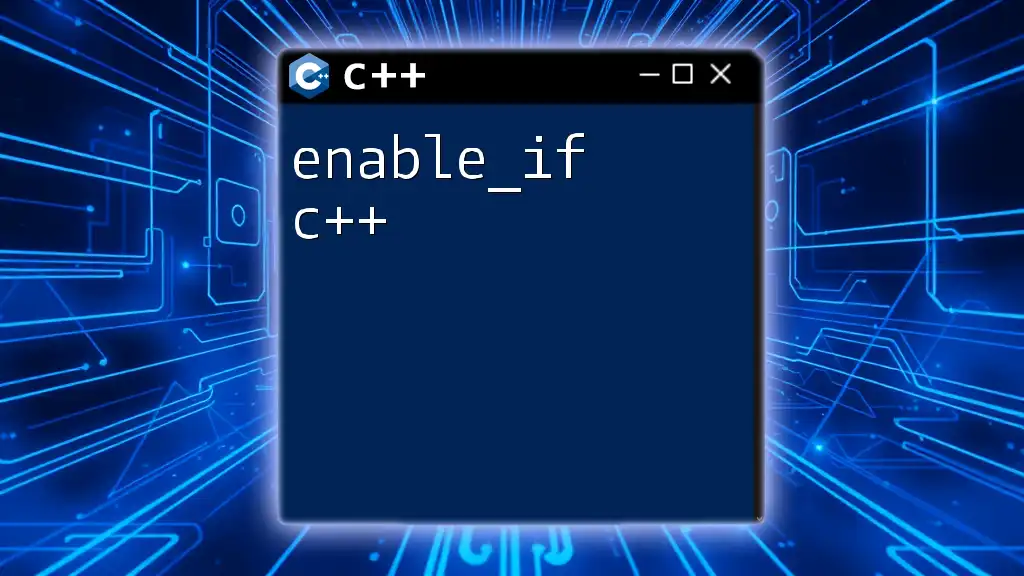
Heapify as a Recursive Function
Understanding Recursion in Heapify
Heapify commonly employs a recursive approach to adjust the tree. Once a node's children have been heapified, it checks whether the current node meets the heap conditions. If not, it swaps the elements and recursively calls itself on the affected child. This method provides clarity and minimizes code complexity, although it may lead to deeper call stacks in large heaps.
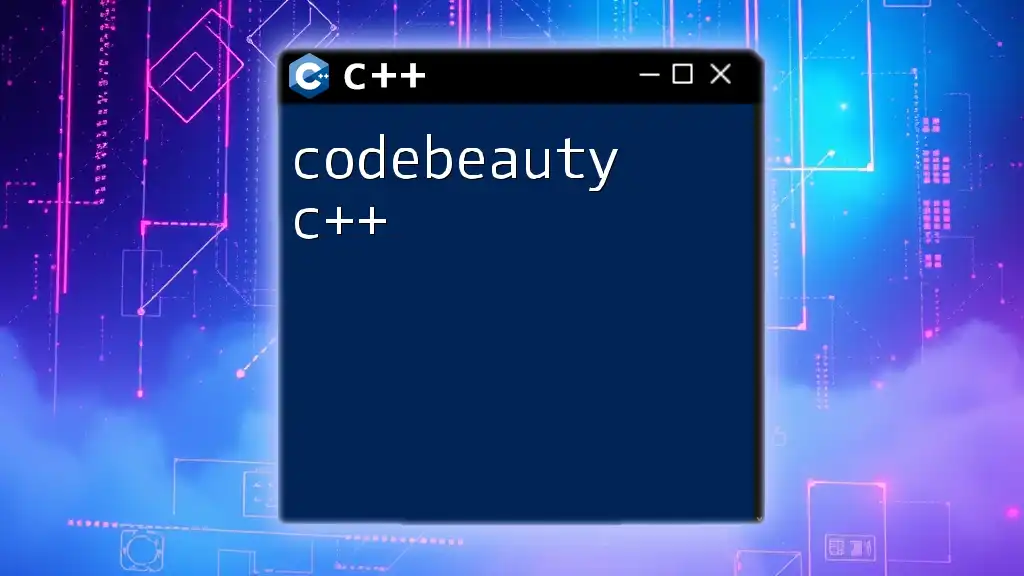
Practical Applications of Heapify
Sorting Algorithms
Heapify serves as a fundamental aspect of heap sort. Heap sort uses the heap structure to sort an array efficiently by repeatedly removing the largest elements from the heap and placing them into their correct position. After the heap has been built, the sort operation can be conducted by repeatedly extracting the maximum element.
Priority Queues
Another significant application of heapify is in the implementation of priority queues. Priority queues use heaps to allow fast retrieval, insertion, and deletion based on priority. This is particularly useful in applications such as job scheduling and real-time system management, where tasks must be processed based on their urgency.
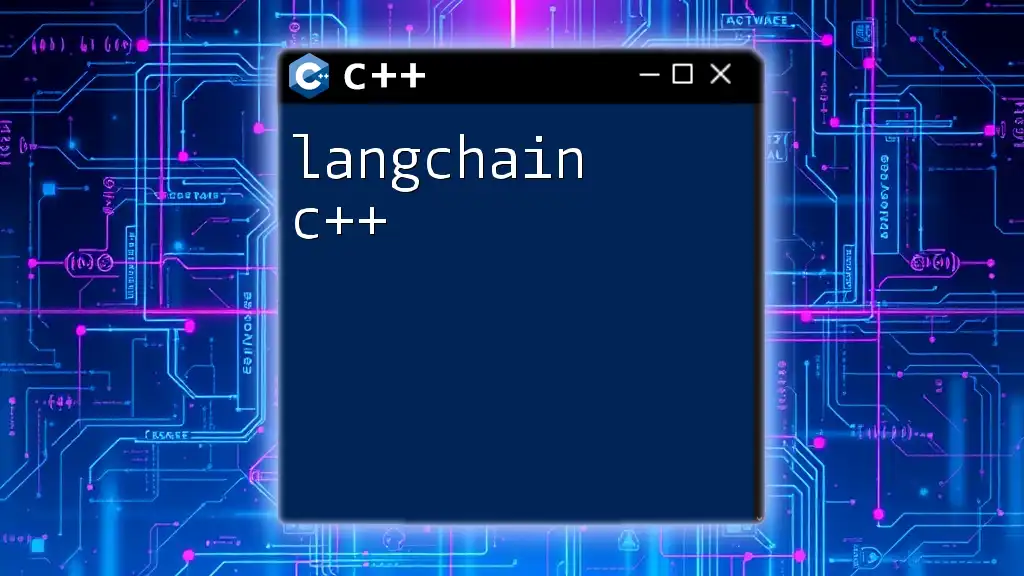
Common Errors and Troubleshooting
Frequently Encountered Issues
Implementing heapify can sometimes lead to common pitfalls:
-
Index Errors: Inadequate checks for leaf nodes can lead to accessing out-of-bounds array indices.
-
Infinite Loops: Failure to update the largest index during the comparisons can lead to the recursive function not terminating.
To troubleshoot, carefully verify the array indices and ensure proper condition checks. Utilizing debug logs can help trace the state of the heap during operations.
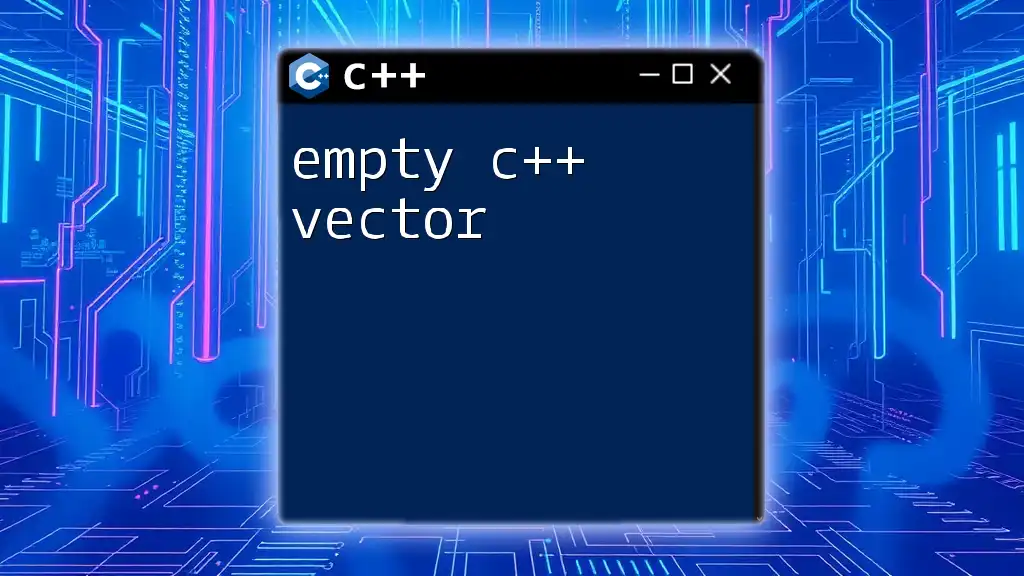
Conclusion
In summary, heapify is an essential concept in C++ programming, providing key functionality for managing heaps, performing efficient sorting, and implementing priority queues. Mastering this operation equips developers with tools for highly effective data management and manipulation. For more advanced techniques, consider diving into specialized resources and practical projects that focus on heaps and heap operations.
Take the next step in your C++ journey; practice implementing heapify functions and explore its various applications!