In C++, the `find` function is used to locate an element in a map by its key, returning an iterator to the element if found or to the end of the map if not found.
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "one"}, {2, "two"}, {3, "three"}};
auto it = myMap.find(2);
if (it != myMap.end()) {
std::cout << "Found: " << it->second << std::endl;
} else {
std::cout << "Not found." << std::endl;
}
return 0;
}
Understanding `map::find`
What is `map::find`?
The `map::find` function is an essential member function that allows programmers to search for a specific key within a C++ map. This function is vital for efficiently accessing values associated with specific keys and plays a crucial role in any operation that requires searching through key-value pairs.
Syntax of `map::find`:
iterator find(const Key& k);
const_iterator find(const Key& k) const;
The `find` method returns an iterator to the element with the specified key if it exists. If the key does not exist, it returns `map::end()`, which is a special iterator indicating the end of the map.
How `map::find` Works
The `find` function operates with a time complexity of O(log n) due to the underlying balanced tree structure of a standard C++ map. This efficiency is crucial for handling data in larger collections, where searching through unordered elements would be significantly slower.
Internally, when `find` is called, it utilizes a binary search mechanism to locate the corresponding node for the specified key. If the key is present, the iterator points to that element; if not, the iterator indicates the position past the last element.

Using `map::find` in C++
Basic Example of `map::find`
Let's start with a simple example that demonstrates the use of `find` in a C++ map. The following code initializes a map with integer keys and string values, searches for a specific key, and outputs the corresponding value if found:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {
{1, "Apple"},
{2, "Banana"},
{3, "Cherry"}
};
auto it = myMap.find(2);
if (it != myMap.end()) {
std::cout << "Key Found: " << it->second << std::endl;
} else {
std::cout << "Key Not Found" << std::endl;
}
return 0;
}
In this code:
- We create a `map` called `myMap`, which contains three integer keys associated with fruit names.
- The `find` method searches for the key `2`.
- If the iterator `it` does not equal `myMap.end()`, we confirm that the key exists and print the corresponding value. If it does equal `myMap.end()`, it means the key wasn't found.
Handling Cases When the Key is Not Found
When using `map::find`, it’s essential to handle cases where the key might not exist. The method returns an iterator to `end` if the key is not found. To avoid attempting to access an element that doesn't exist, always check if the returned iterator is equal to `end`.
Another best practice is to use the returned iterator to avoid redundant lookups:
auto it = myMap.find(4); // Searching for a key that doesn't exist
if (it == myMap.end()) {
std::cout << "Key Not Found. Please check the key." << std::endl;
} else {
// Safe access
std::cout << "Value: " << it->second << std::endl;
}

Advanced Usage of `map::find`
Finding Values with Different Types of Keys
The power of `map::find` is not limited to integer keys. Let's explore how it can be utilized with string keys:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap = {
{"Alice", 30},
{"Bob", 25},
{"Charlie", 35}
};
auto it = ageMap.find("Bob");
if (it != ageMap.end()) {
std::cout << "Age of Bob: " << it->second << std::endl;
} else {
std::cout << "Key Not Found" << std::endl;
}
return 0;
}
In this example:
- We establish a map `ageMap` with string keys and integer values.
- Searching for "Bob" demonstrates that `map::find` is versatile, allowing for efficient lookups across various types of data.
Using Iterators with `map::find`
When using `find`, you can also leverage iterators to traverse the map. Here's how you can combine `find` with iterators:
std::map<int, std::string>::iterator it = myMap.find(1);
if (it != myMap.end()) {
for (auto current = it; current != myMap.end(); ++current) {
std::cout << current->first << ": " << current->second << std::endl;
}
}
This segment not only finds the specified key but also iterates from that position to the end of the map, printing all remaining key-value pairs.

Common Pitfalls with `map::find`
Case Sensitivity Issues with Strings
When using strings as keys, be aware that string comparisons in C++ are case-sensitive. This can lead to scenarios where "Bob" and "bob" are treated as different keys. To mitigate this, ensure consistency in how keys are defined and searched. Implement converting keys to lowercase prior to insertion or searching.
Performance Considerations
Choosing the right key type for your map can significantly impact performance. For instance, using complex object types as keys without proper hash or comparison functions can lead to poor performance and inefficient searches. Always opt for simple types (like integers and strings) when possible, or implement efficient custom comparison functions if necessary.
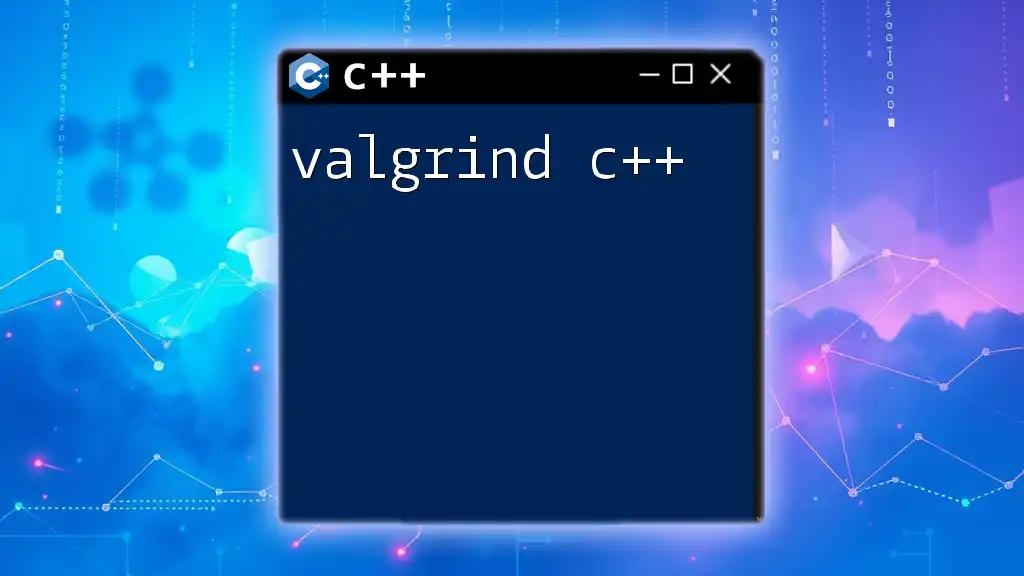
Best Practices for Using `map::find`
Choosing the Right Key Type
Selecting the appropriate key type affects both efficiency and performance. If your application frequently accesses elements based on computed keys, consider leveraging hash functions. C++ allows users to define their comparison functions for custom key types, thereby enhancing the flexibility of `find`.
Avoiding Redundant Operations
When searching for keys in a map, it’s crucial to avoid performing multiple lookups for the same key. Cache the result of `find` and use the retrieved iterator for subsequent operations; this will optimize performance significantly.

Conclusion
The `map find c++` function is an invaluable tool for developers working with associative containers. Understanding its workings, handling edge cases, and implementing advanced and best practices will help you maximize its efficiency. As you gain confidence with `map::find`, practice using it in various scenarios to deepen your understanding and proficiency in C++.
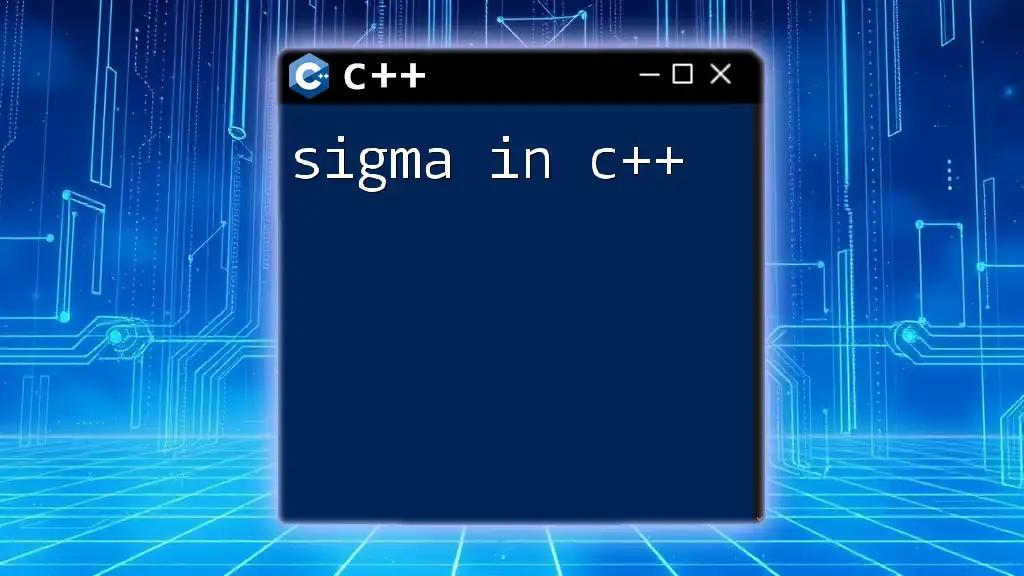
Additional Resources
For those eager to learn more, numerous online courses and C++ documentation can enhance your knowledge of maps and STL containers. Books like "The C++ Programming Language" by Bjarne Stroustrup also provide in-depth coverage of key topics in C++.

Frequently Asked Questions
What does `map::find` return if the key is not found?
If the specified key does not exist in the map, `map::find` returns an iterator that points to `map::end()`, indicating there are no elements with that key.
Can I use `map::find` with a custom comparison function?
Yes, C++ allows for custom comparison functions in map creation, enabling `find` to function appropriately with user-defined key types.
How does `find` compare to other map searching methods?
Compared to other methods like `count` or indexing operators, `find` is more efficient for searching since it can immediately locate an element and provide an iterator directly, whereas `count` simply checks for existence and indexing may throw exceptions if the key isn't found.