The `var` keyword is not a part of standard C++, but if you're referring to creating variables, you can declare them using the syntax below.
Here’s a simple example of variable declaration in C++:
int x = 5; // Declare an integer variable x and assign it the value of 5
double y = 3.14; // Declare a double variable y and assign it the value of 3.14
What is var in C++?
In the context of programming, the term "var" typically refers to a variable, serving as a symbolic name for data, meaning it holds a reference to a value in memory. In C++, the word "var" itself is not an official keyword or command; C++ requires explicit type declaration for variables. This means instead of using a simplistic keyword like "var," C++ programmers specify the type of variable being declared.
The concept may draw parallels from languages like JavaScript or Python, where "var" is used for variable declarations. However, in C++, the emphasis is on strong typing, meaning every variable must have a defined data type when it is declared.
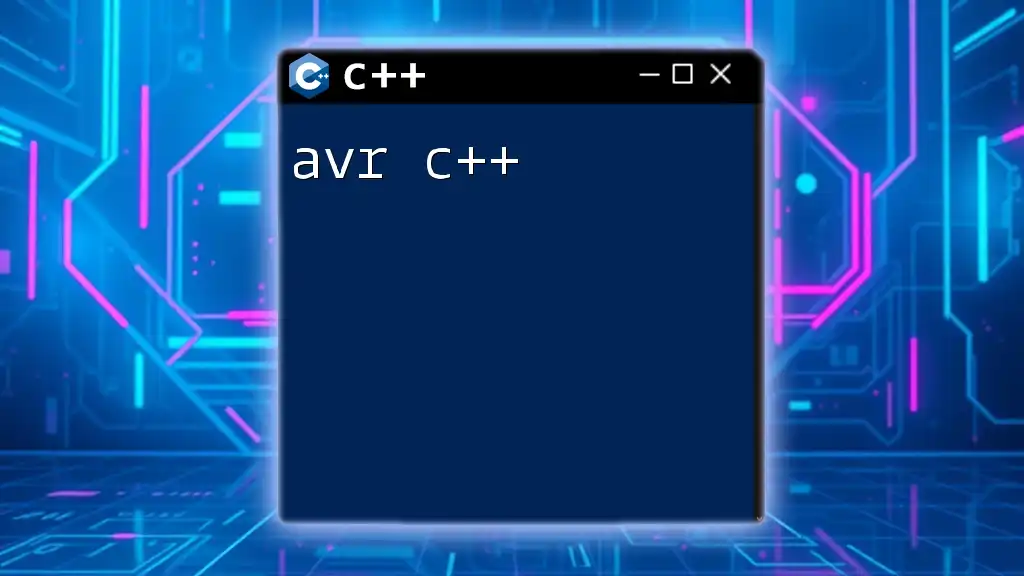
Understanding C++ Defining Variables
What is a Variable in C++?
A variable in C++ is a container that holds data that can change during the execution of a program. It allows for data manipulation and is fundamental to storing information.
Types of Variables in C++
Built-in Data Types
C++ provides several built-in data types to support different kinds of data.
- Integer (int): Used for whole numbers.
- Floating-point (float/double): Used for decimal numbers.
- Character (char): Used for single characters.
- Boolean (bool): Represents true or false.
Here's a simple code example demonstrating different data types:
int age = 25;
float salary = 50000.50;
char initial = 'A';
bool isEmployed = true;
These types allow programmers to choose the appropriate kind of data for their specific needs.
User-Defined Data Types
In addition to built-in types, C++ allows for user-defined types, enhancing flexibility.
- Structs: Used to group different types of variables together.
- Classes: Fundamental for Object-Oriented Programming.
- Enums: Useful for defining a variable that can hold a set of predefined constants.
Here’s an example of a custom structure:
struct Person {
int age;
float height;
};
This example shows how user-defined types can enhance data organization and management.
Declaring and Initializing Variables
Syntax for Declaring Variables
Declaring a variable in C++ is straightforward. The syntax requires first specifying the data type followed by the variable name:
int number; // Declaration
This line creates an integer variable `number` but does not initialize it with a value yet.
Initializing Variables
It’s beneficial to initialize variables at the time of declaration to prevent unexpected behavior.
int number = 10; // Declaration and initialization
In this example, `number` is created and simultaneously assigned a value. It’s crucial to ensure variables are initialized to avoid undefined behavior.
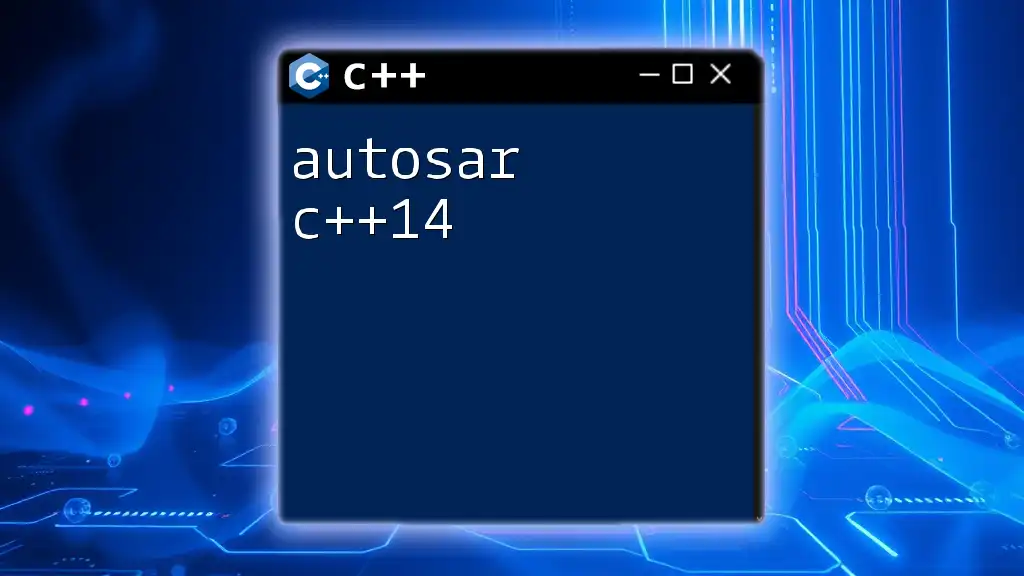
Variable Scoping in C++
Understanding Variable Scope
Variable scope determines the visibility and lifetime of a variable within different parts of a program. Understanding the types of variable scope is essential for effective C++ programming.
- Local Variables: Declared within a function and can only be accessed inside it.
- Global Variables: Declared outside any function and can be accessed from any function within the same file.
- Static Variables: These retain their value even after the function has exited.
Here's an example of different scopes:
int globalVar = 20; // Global variable
void function() {
int localVar = 10; // Local variable
// Accessing globalVar inside function is allowed
}
Accessing Variables in Different Scopes
Accessing variables in C++ depends significantly on the scope where they are declared. For instance, a local variable is not accessible outside its function.
If you need to access a global variable inside functions, you can do so directly:
void display() {
std::cout << globalVar; // Global variable can be accessed
}
C++ also provides the `extern` keyword if you want to declare a variable in one file but define it in another, providing a way to share variables across multiple files.
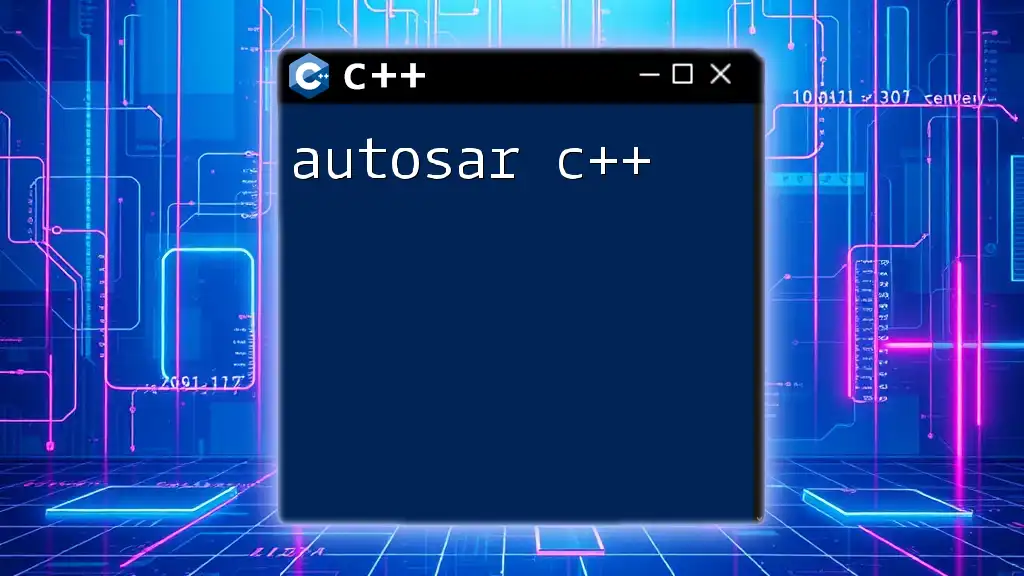
Best Practices for Defining Variables in C++
Naming Conventions
Using clear and descriptive naming conventions for variables plays a vital role in writing maintainable code. Common approaches include:
- Camel Case: `totalValue`
- Snake Case: `total_value`
It is crucial that variable names clearly convey their purpose, which enhances code readability.
Commenting and Documentation
Lines of code are easier to understand with appropriate comments. Documenting the purpose of variables or complex logic ensures clarity for anyone reading the code, including future you.
For example:
// Age of the user in years
int age = 30;
Optimizing Memory Usage
Choosing the right variable types impacts the program’s memory usage. For example, using `int` when `short` would suffice can waste space. Here’s how to choose the right type:
- Use `int` for numeric values without decimal.
- Use `float` or `double` for decimal numbers depending on precision needed.
- Prefer `char` arrays or strings for text manipulation over an `int`.
Choosing the appropriate types leads to better memory management, which is crucial in performance-sensitive applications.
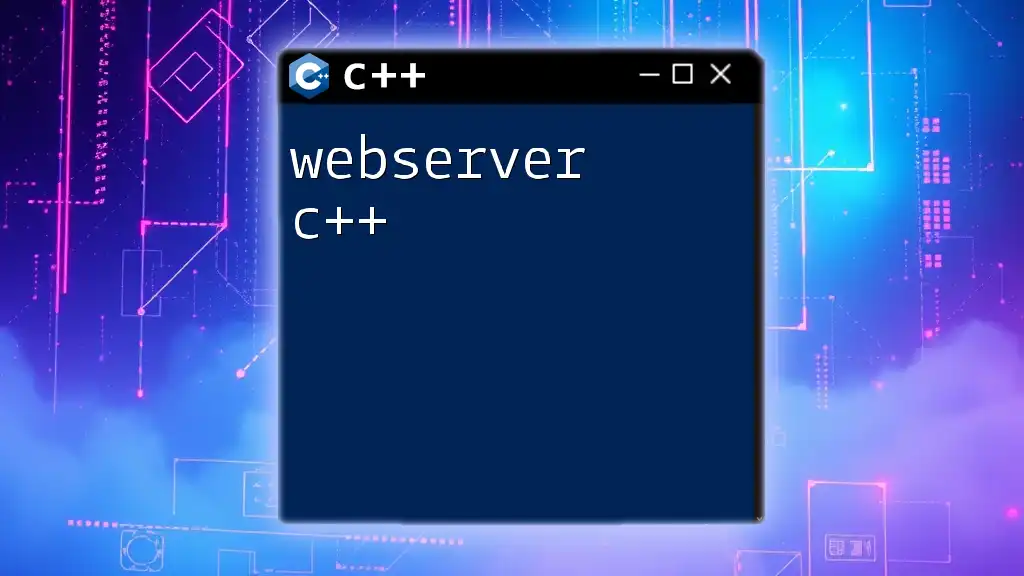
Conclusion
Understanding the concept of variables and their manipulation in C++ is foundational for any programmer looking to utilize C++ effectively. While the term "var" may not exist in C++, the importance of defining and utilizing variables resonates throughout the language.
As you familiarize yourself with these principles, practice is vital. Start coding with different types of variables and explore their scope, initialization, and memory management to enhance your C++ skills!
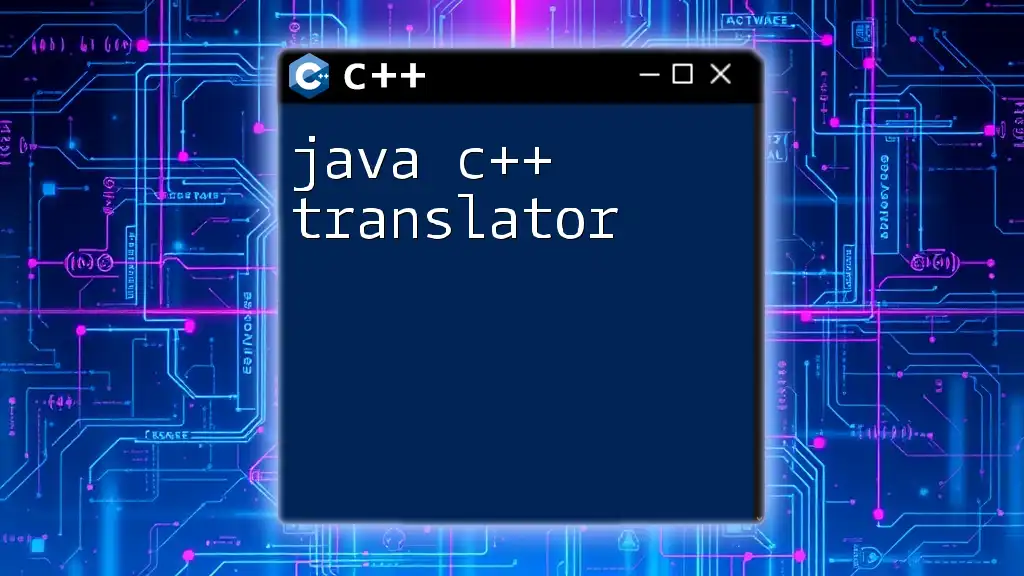
Additional Resources
- C++ programming tutorials on websites like Codecademy or Coursera.
- The official C++ documentation for deeper understanding of variable types and scoping.
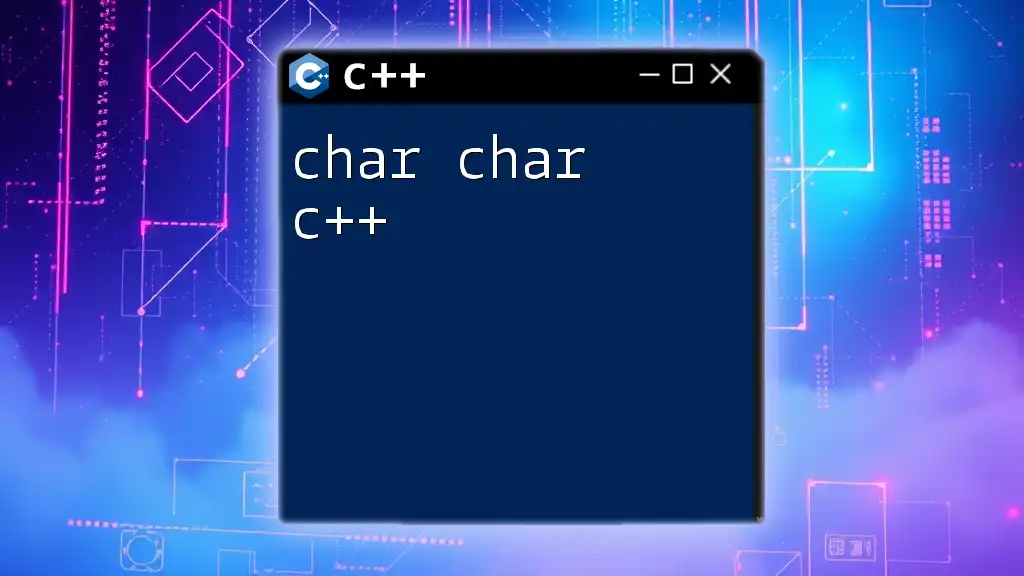
Frequently Asked Questions
-
What is a variable? A variable is a storage location paired with an associated symbolic name that contains a value.
-
How do I declare a variable in C++? You declare a variable by specifying its data type followed by the variable name.
-
Are there shortcuts in variable definition? C++ requires explicit type declarations; there are no shortcuts like 'var' as seen in other languages.
-
What happens if I don’t initialize my variable? If not initialized, the variable may start off with an undefined value, leading to unpredictable behavior.
By mastering the use of variables, you will be well-equipped to take on more complex C++ programming challenges!