AVR C++ is a programming approach that utilizes C++ to develop applications for AVR microcontrollers, enabling efficient hardware control with object-oriented programming features.
Here’s a simple code snippet demonstrating how to blink an LED connected to pin PB0 of an AVR microcontroller:
#include <avr/io.h>
#include <util/delay.h>
int main() {
DDRB |= (1 << PB0); // Set PB0 as an output
while (1) {
PORTB ^= (1 << PB0); // Toggle the LED
_delay_ms(1000); // Wait for 1 second
}
}
What is AVR?
AVR refers to a family of microcontrollers developed by Atmel (now part of Microchip Technology). These microcontrollers are based on RISC (Reduced Instruction Set Computing) architecture, which allows for efficient processing and improved performance in comparison to other architectures. AVR microcontrollers are widely used in various applications, from simple hobby projects to complex industrial systems. Some popular models include the ATmega series and ATtiny series, which come with built-in flash memory, EEPROM, and various I/O functionalities.
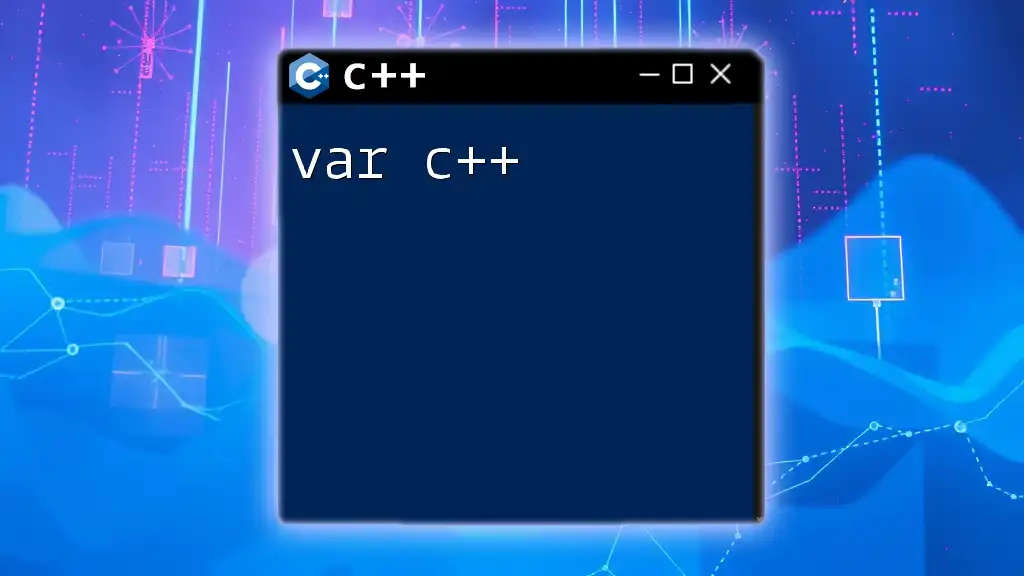
Why Use C++ with AVR?
Choosing C++ for AVR programming provides several advantages over traditional C programming. While C is effective for low-level hardware control, C++ offers advanced programming features such as classes and exception handling, which can lead to cleaner code and better encapsulation. Using C++, developers can design more modular and reusable code, making project maintenance easier and scalable. The object-oriented nature of C++ allows for more intuitive interactions with hardware components, crucial in creating complex applications.
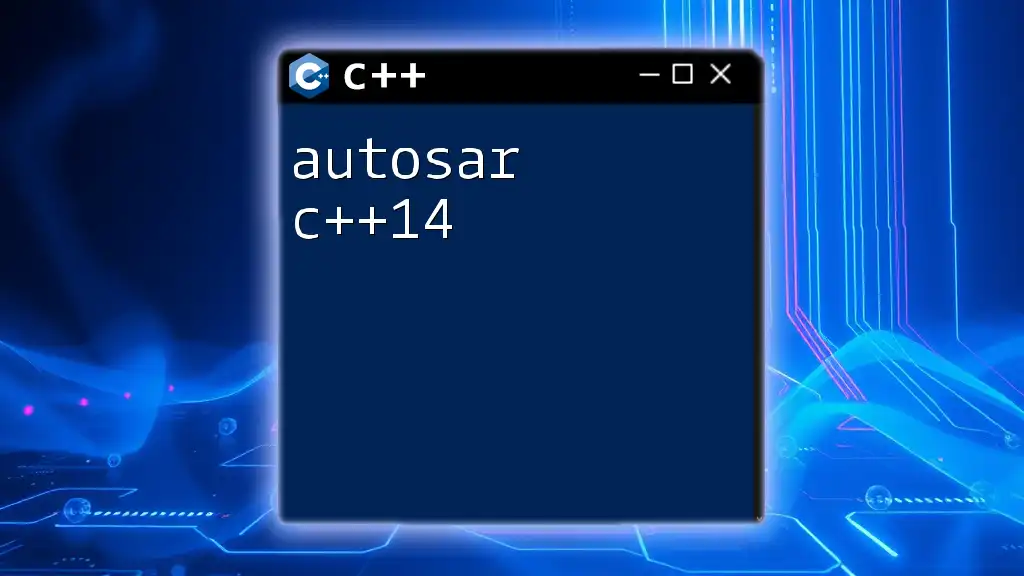
Setting Up Your AVR Development Environment
Required Software and Tools
Before diving into AVR C++ programming, you need to prepare your development environment. Select an Integrated Development Environment (IDE) that suits your needs. Popular choices include:
- Atmel Studio: A powerful IDE specifically designed for AVR development.
- PlatformIO: An open-source ecosystem for IoT development that supports multiple platforms.
Installing AVR Toolchain
To compile C++ code for AVR microcontrollers, you need to install the AVR Toolchain. Here’s a quick guide for different operating systems:
-
Windows:
- Download the installer for WinAVR.
- Follow the installation steps and ensure that the installation path is added to the system PATH.
-
Mac:
- Use Homebrew to install the toolchain with the command: `brew install avr-gcc`.
-
Linux:
- Use the package manager, e.g., for Ubuntu, run: `sudo apt install avr-gcc`.
After installation, configure your IDE to recognize the AVR Toolchain and set up the appropriate board settings.
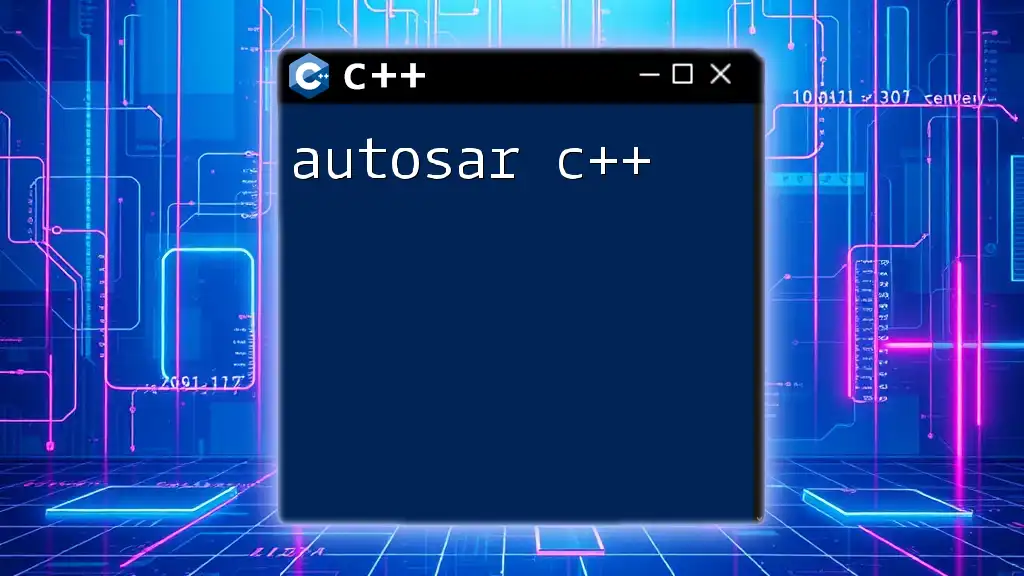
Understanding the AVR Architecture
Overview of AVR Microcontroller Architecture
To write effective AVR C++ code, it is essential to understand the architecture of AVR microcontrollers. Each AVR microcontroller includes a CPU, various I/O ports, RAM, ROM, and timers. The RISC architecture is key to its efficiency, allowing for many instructions to execute in a single clock cycle.
Reading the AVR Datasheet
To utilize any specific features of an AVR microcontroller, familiarize yourself with its datasheet. Datasheets provide critical information about:
- Pin configuration
- Electrical characteristics
- Memory layout
A thorough reading of the datasheet will enable you to harness the full potential of your selected AVR model.
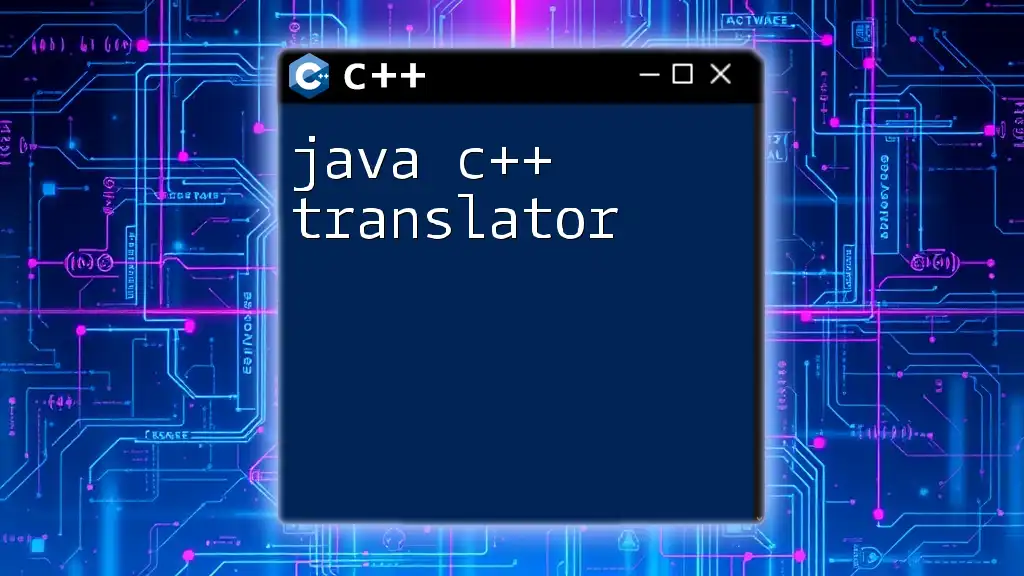
Getting Started with C++ on AVR
Basic C++ Syntax Recap
Before diving into AVR C++ implementation, ensure you are familiar with key C++ syntax. Understanding variables, functions, loops, and control structures is crucial for effective programming.
Setting Up a Simple LED Blink Program
One of the most common introductory projects is creating a LED blink application. This project will help you grasp the basics of programming AVR microcontrollers using C++. Here’s a simple code snippet to accomplish this:
#include <avr/io.h>
#include <util/delay.h>
int main(void) {
DDRB |= (1 << DDB5); // Set pin 5 of PORTB as output
while (1) {
PORTB |= (1 << PORTB5); // Turn LED on
_delay_ms(1000); // Wait for a second
PORTB &= ~(1 << PORTB5); // Turn LED off
_delay_ms(1000); // Wait for a second
}
return 0;
}
In the above program, we configure pin 5 of PORTB as an output to control an LED. The program repeatedly turns the LED on and off with a one-second delay.
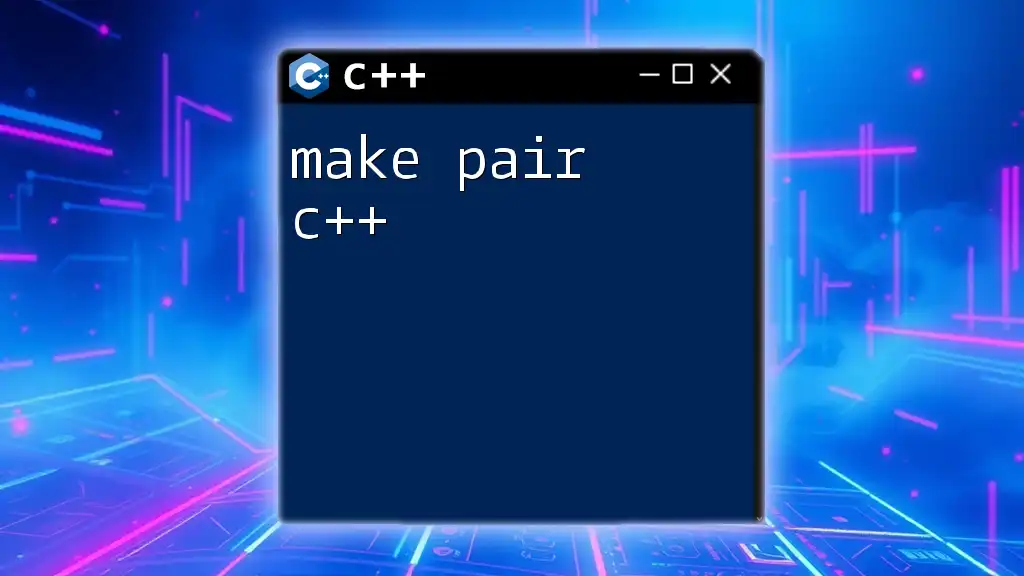
C++ Features Utilized in AVR Programming
Classes and Objects
C++ allows you to create a systematic approach to device interaction through classes. For example, creating a class for managing a timer can make your code cleaner and reusable. Here’s an example class:
class Timer {
public:
Timer() {
// Timer initialization
}
void delayMs(int milliseconds) {
for(int i = 0; i < milliseconds; i++) {
_delay_ms(1); // Delay for 1 millisecond
}
}
};
Inheritance and Polymorphism
Building on the class example, inheritance allows you to extend functionality. You can create different time, sensor, or communication classes that inherit from a base class. This structure can improve your code's modularity and make it easier to implement changes.
Namespaces
Namespaces help organize your code and avoid name clashes. You can encapsulate your I/O operations within a namespace like this:
namespace IOPort {
void setOutput(uint8_t pin) {
DDRB |= (1 << pin); // Set the pin as output
}
}
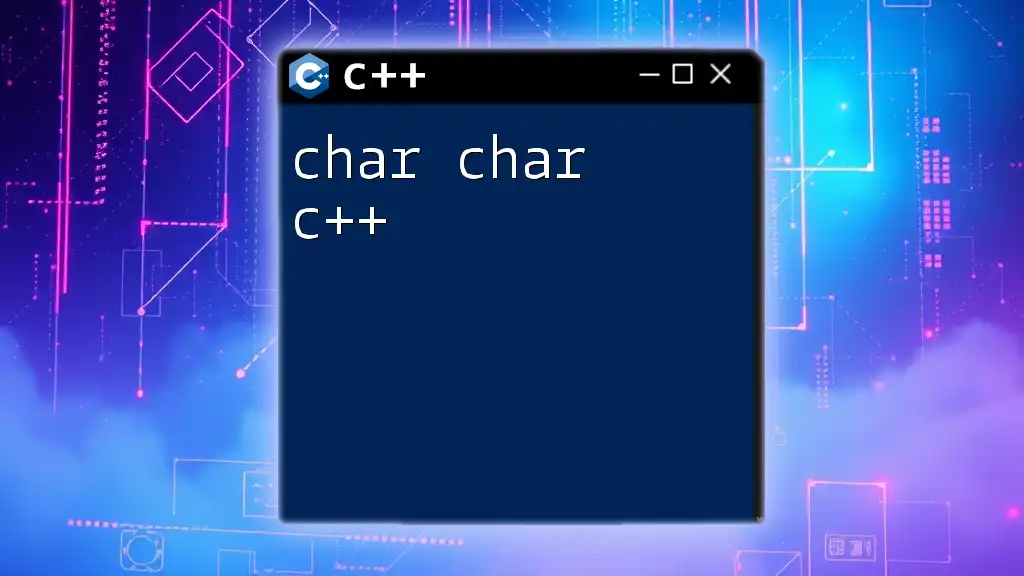
AVR Libraries for C++
Using AVR-specific Libraries
Make use of popular libraries designed for AVR development, such as the standard Arduino core, which provides high-level APIs for handling numerous onboard peripherals, making your development tasks easier.
Creating Your Own Libraries
Creating custom libraries can streamline code reuse. Start by grouping related functions in a single header and implementation file. For example, if you frequently work with a specific sensor, wrap its functionalities in a library to minimize code duplication.
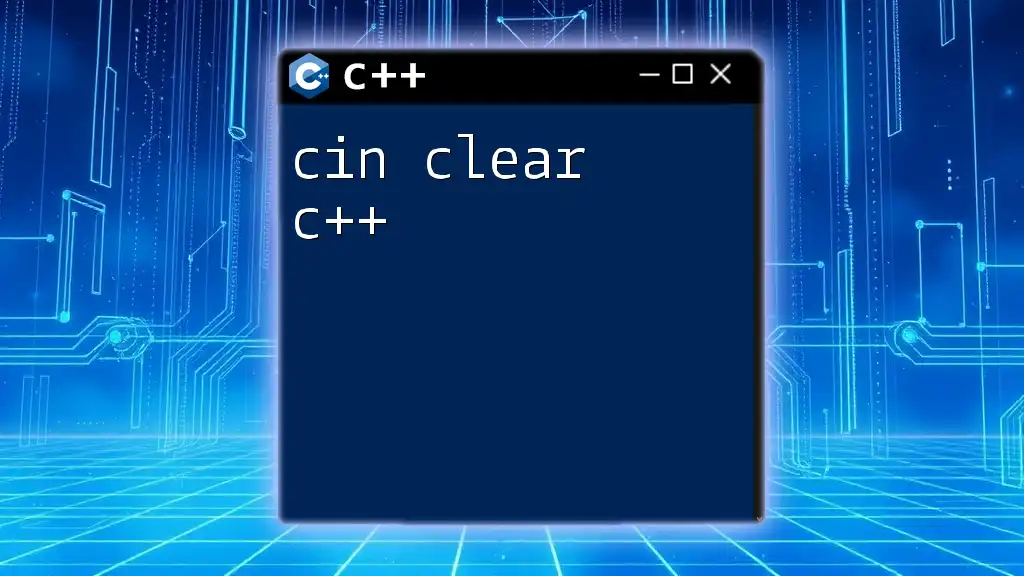
Advanced C++ Concepts in AVR
Templates and Generic Programming
C++ templates enable you to write generic functions or classes, saving you time and boosting code reusability. Here’s a brief example of a generic function for swapping two variables:
template <typename T>
void swap(T& a, T& b) {
T temp = a;
a = b;
b = temp;
}
Exception Handling in Embedded C++
Although supported, using exceptions in embedded systems should be done with care. Exceptions can increase the code size and may lead to performance penalties. It’s best to handle errors through simpler methods, like return codes or status flags, ensuring your resource-constrained system remains efficient.
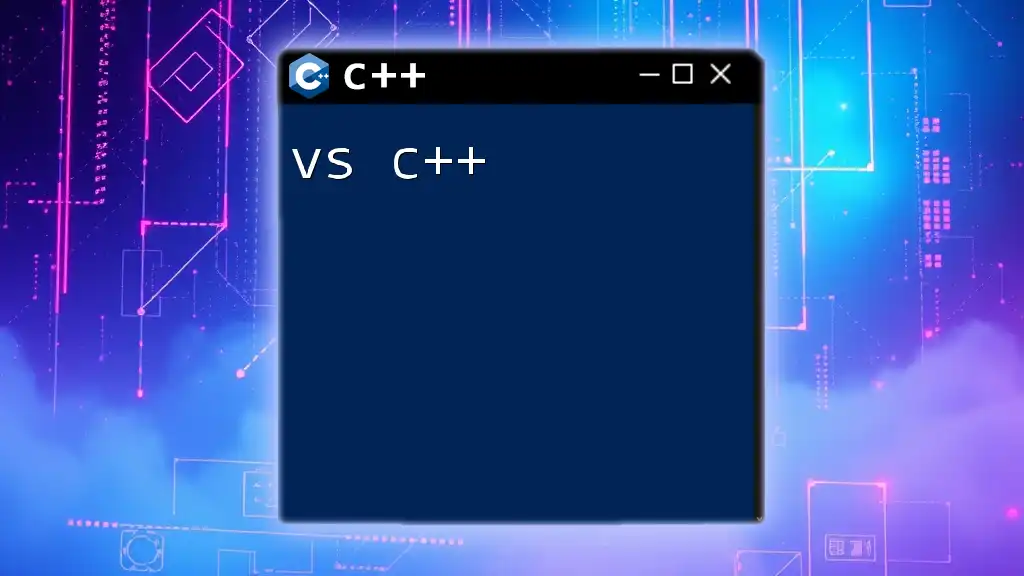
Debugging and Optimization in AVR C++
Common Debugging Techniques
Debugging AVR C++ programs can be challenging, especially without advanced debugging tools. Utilize serial communication for logging outputs or use LEDs to signal status changes during development.
Optimizing C++ Code for AVRs
Optimization is critical in embedded programming. Constant variables can save memory, and the use of `inline` functions can speed up function calls. Avoiding dynamic memory allocation during runtime is also a best practice to prevent fragmentation and ensure reliability.
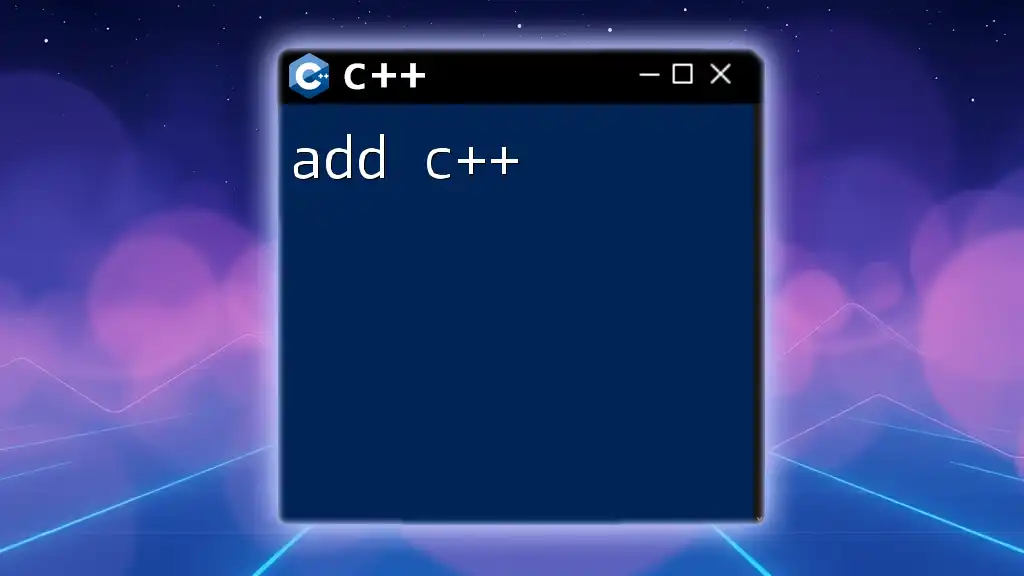
Best Practices for AVR C++ Development
Writing Clean and Maintainable Code
To ensure your code remains easy to read and maintain, focus on the following best practices:
- Use meaningful variable and function names.
- Keep code consistent with indentation and styling.
- Add comments to clarify complex logic or explain hardware interactions.
Testing and Simulation
Utilize tools like Proteus or SimulIDE for testing and simulating your projects before hardware implementation. This can uncover issues early in the development process and save time during the debugging phase.
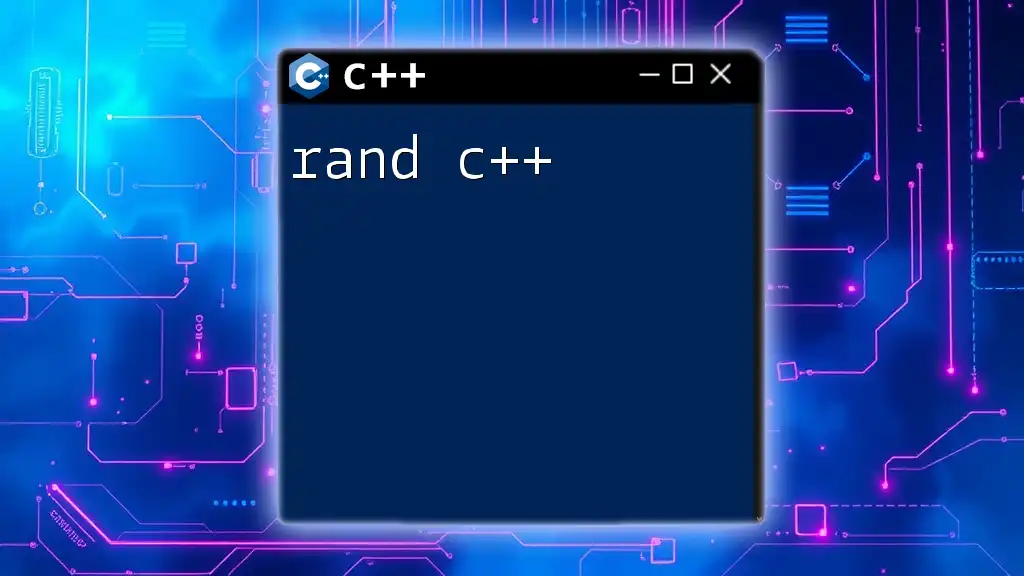
Conclusion
AVR C++ programming offers powerful features that can significantly enhance how you interact with embedded systems. By setting up a robust development environment, utilizing advanced C++ features, and following best practices, you can create efficient and maintainable applications. As you continue your journey in AVR C++, don’t forget to explore further resources to deepen your knowledge and refine your skills.