The Kalman Filter in C++ is an algorithm that uses a series of measurements over time, containing noise and other inaccuracies, to produce estimates of unknown variables that tend to be more precise than those based on a single measurement alone.
Here's a simple implementation of the Kalman Filter in C++:
#include <iostream>
class KalmanFilter {
public:
KalmanFilter() : Q(1e-5), R(1e-2), x(0), P(1) {}
double update(double measurement) {
// Prediction step
P = P + Q;
// Measurement update step
K = P / (P + R);
x = x + K * (measurement - x);
P = (1 - K) * P;
return x;
}
private:
double Q; // Process noise covariance
double R; // Measurement noise covariance
double x; // Estimated state
double P; // Estimate error covariance
double K; // Kalman gain
};
int main() {
KalmanFilter kf;
for (double measurement : {1, 2, 3}) {
std::cout << "Updated estimate: " << kf.update(measurement) << std::endl;
}
return 0;
}
What is a Kalman Filter?
The Kalman Filter is an advanced mathematical tool used for estimating the state of a dynamic system from a series of incomplete and noisy measurements. Developed by Hungarian mathematician Rudolf Kalman in the 1960s, it has become a fundamental algorithm in various fields, including robotics, navigation, and financial modeling.
This filter efficiently estimates the state of a linear dynamic system over time, providing a means to predict future states and correct estimates based on new observations. Its approach combines predictions from the system’s model and measurements, which allows for real-time applications that handle noise effectively.
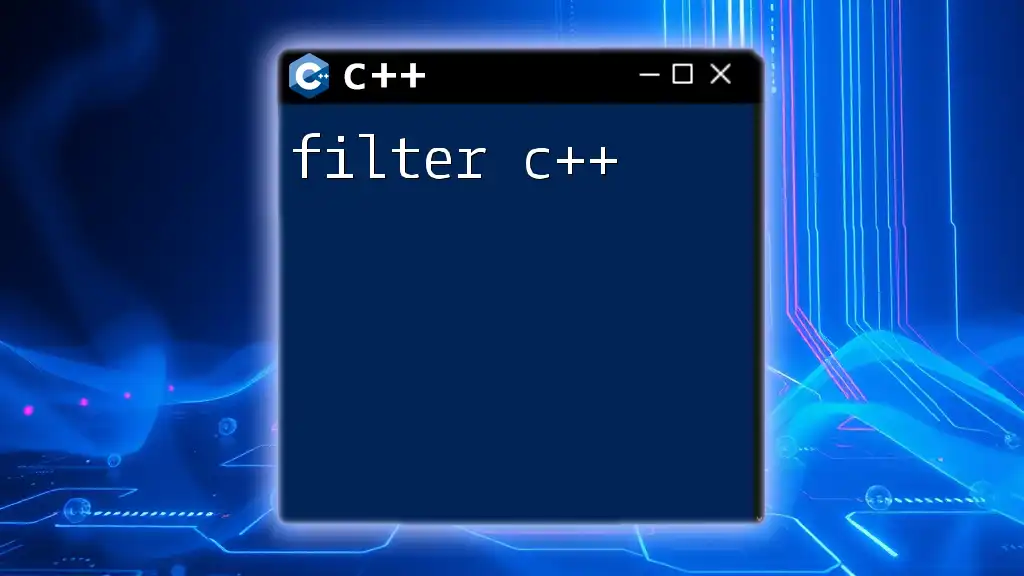
Applications of Kalman Filters
The versatility of the Kalman Filter in C++ makes it suitable for several applications:
- Robotics: Used for navigation and sensor fusion, helping robots understand their location and surroundings.
- Finance: Analyzes market trends and aids in predicting stock prices by filtering out noise from historical data.
- Aerospace: Critical for estimating the positions and movements of aircraft and satellites using sensor data.
- Computer Vision: Assists in tracking moving objects in video feeds, providing better accuracy in identifying positions.
The Kalman Filter plays a crucial role in estimation problems, where accurate measurement and forecasting are essential.
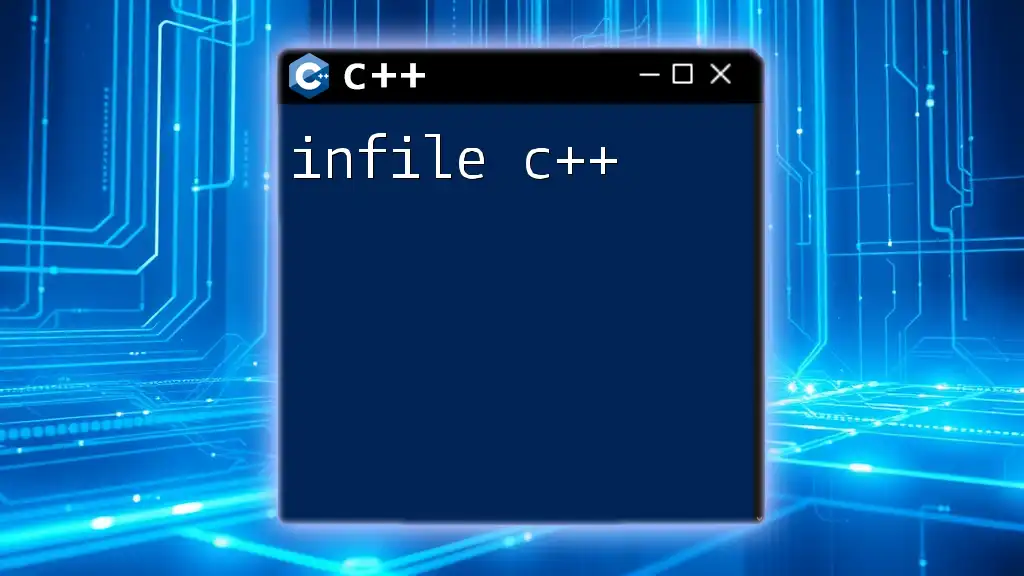
Understanding the Mathematical Foundations
State Estimation
At the core of the Kalman Filter is the concept of a state vector. The state vector encapsulates all relevant information about the system at any given time. The filter also maintains a covariance matrix that represents the uncertainty associated with the state estimation.
Process and Measurement Models
The filter relies on two primary models:
-
Process Model: This model describes how the system evolves over time, represented by the state transition equations in the Kalman Filter. It predicts the future state based on the current state.
-
Measurement Model: This model relates the observations to the state vector. It provides the framework to update the prediction based on new measurements.
Importantly, the Kalman Filter traditionally operates under the assumption of linear systems. However, modifications for nonlinear systems exist through Extended Kalman Filters (EKF).
Kalman Filter Equations
The Kalman Filter operates through two main phases: prediction and update.
Predict Step
During this phase, the filter predicts the current state and uncertainty based on the previous state:
-
Predicting the State: \[ x_{k} = F \cdot x_{k-1} \]
-
Predicting the Covariance: \[ P_{k} = F \cdot P_{k-1} \cdot F^T + Q \]
Where \( F \) is the state transition matrix, \( Q \) is the process noise covariance, \( x \) is the state vector, and \( P \) is the covariance matrix.
Update Step
In this phase, the filter updates the state based on a new measurement:
-
Measurement Update: \[ y_{k} = z_{k} - H \cdot x_{k} \]
-
Kalman Gain Calculation: \[ K_{k} = P_{k} \cdot H^T \cdot (H \cdot P_{k} \cdot H^T + R)^{-1} \]
-
Correcting the State: \[ x_{k} = x_{k} + K_{k} \cdot y_{k} \]
-
Updating Covariance: \[ P_{k} = (I - K_{k} \cdot H) \cdot P_{k} \]
Where \( H \) is the observation model and \( R \) is the measurement noise covariance.
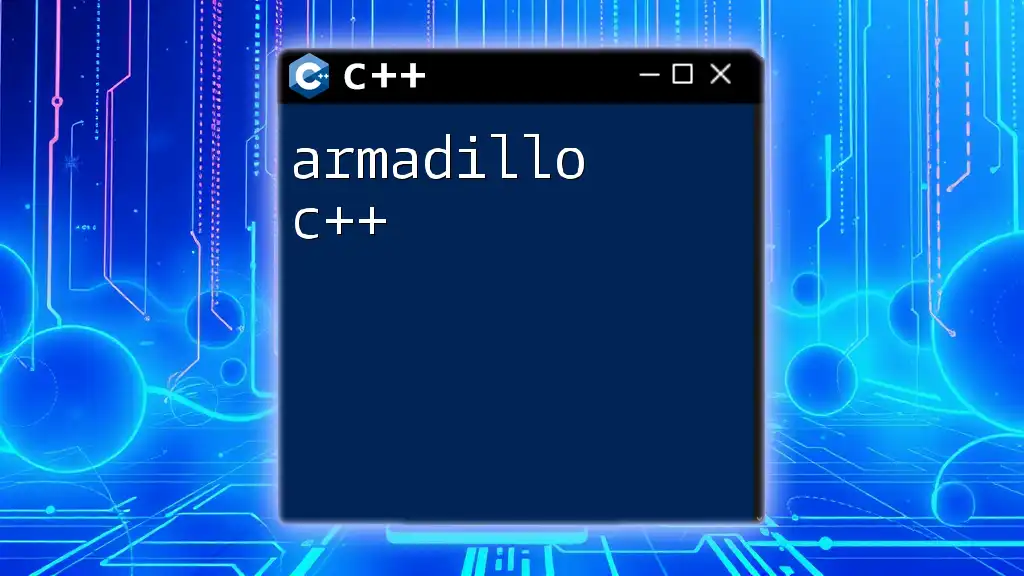
Implementing Kalman Filter in C++
Setting Up the Project
To begin implementing the Kalman Filter in C++, you’ll need to set up a project that supports the necessary libraries. A popular choice is the Eigen library, which simplifies matrix and vector operations essential for Kalman filtering.
Coding the Kalman Filter
Step 1: Defining the Kalman Filter Class
A well-structured class will encapsulate all aspects of the Kalman Filter. Here’s a basic outline of a `KalmanFilter` class:
class KalmanFilter {
public:
Eigen::VectorXd x; // state
Eigen::MatrixXd P; // covariance
Eigen::MatrixXd F; // state transition matrix
Eigen::MatrixXd H; // observation model
Eigen::MatrixXd R; // observation noise covariance
Eigen::MatrixXd Q; // process noise covariance
};
In this class, the matrices are defined to store the current state, covariance, state transition model, measurement model, and noise covariance matrices.
Step 2: Implementing the Predict Function
The predict function updates the state and covariance matrices based on the process model:
void KalmanFilter::Predict() {
x = F * x; // Update the state estimate
P = F * P * F.transpose() + Q; // Update the covariance
}
This function is crucial as it forms the basis for all subsequent state estimations.
Step 3: Implementing the Update Function
The update function incorporates the latest measurement into the state estimation:
void KalmanFilter::Update(const Eigen::VectorXd &z) {
Eigen::VectorXd y = z - H * x; // Measurement residual
Eigen::MatrixXd K = P * H.transpose() * (H * P * H.transpose() + R).inverse(); // Kalman gain
x = x + K * y; // Updated state estimate
P = (Eigen::MatrixXd::Identity(x.size(), x.size()) - K * H) * P; // Updated covariance estimate
}
This segment effectively improves the state estimate by leveraging observations to refine predictions.
Example Application
To illustrate the application of the Kalman Filter in C++, let's consider a simple 1D object tracking scenario where we use a Kalman filter to track the position of a moving object. Below is a basic outline of the implementation:
- Define the initial state and matrices.
- Create a loop to simulate measurements.
- In each iteration, call the `Predict()` and `Update()` functions.
Here’s a sample code that showcases the entire process:
int main() {
KalmanFilter kf;
// Initial state
kf.x = Eigen::VectorXd(2);
kf.x << 0, 0; // [position, velocity]
// Initial covariance
kf.P = Eigen::MatrixXd::Identity(2, 2);
// State transition matrix
kf.F = (Eigen::MatrixXd(2, 2) << 1, 1, 0, 1).finished();
// Observation model
kf.H = Eigen::MatrixXd(1, 2);
kf.H << 1, 0;
// Process noise covariance
kf.Q = 0.1 * Eigen::MatrixXd::Identity(2, 2);
// Measurement noise covariance
kf.R = 0.1 * Eigen::MatrixXd::Identity(1, 1);
for (int i = 0; i < 100; ++i) {
// Simulated measurement
double measurement = /*... obtain measurement ...*/;
kf.Predict(); // Perform prediction
kf.Update(measurement); // Update with new measurement
}
// Output final estimated state
std::cout << "Estimated State: " << kf.x.transpose() << std::endl;
}
This snippet sets up the filter and runs through a loop simulating measurements. The predictive and corrective stages are executed, updating the state each time a new measurement is available.
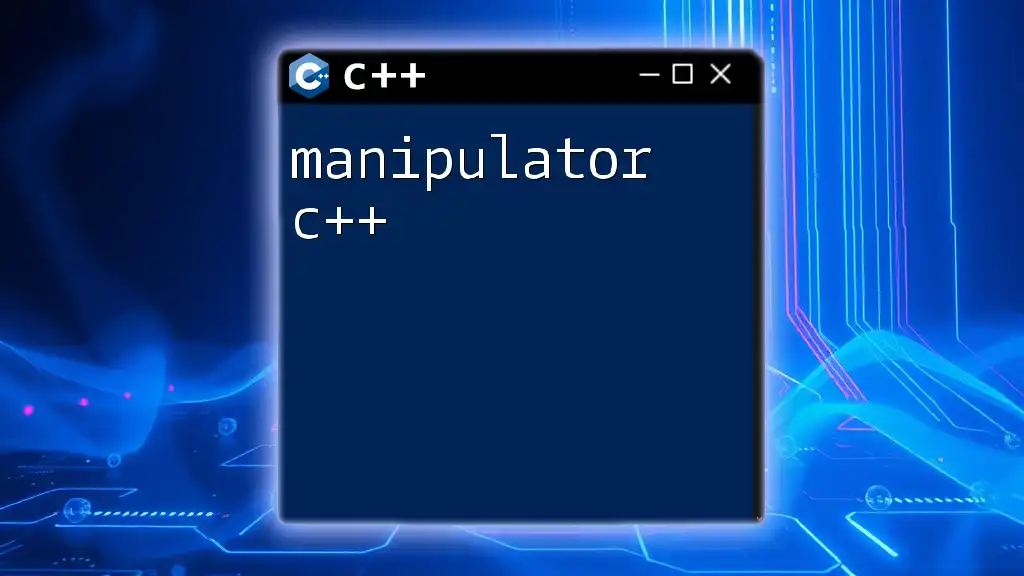
Troubleshooting Common Issues
Tuning the Kalman Filter
Tuning is an essential part of getting the Kalman Filter in C++ to perform optimally. The most common parameters to adjust are the process noise covariance \(Q\) and the measurement noise covariance \(R\). Improper tuning can lead to unstable or biased estimates, resulting in poor performance.
Debugging Techniques
When working with the Kalman Filter, you may encounter several issues:
- Convergence: If the filter fails to converge, revisit your selection of \(Q\) and \(R\). Often, adjusting these values helps the filter to respond adequately to noise.
- Behavior: Check the dimensions of your matrices to prevent mismatched operations, which can lead to runtime errors.
- Verification: Compare the filtered output against known inputs or other estimation techniques to ensure correctness.
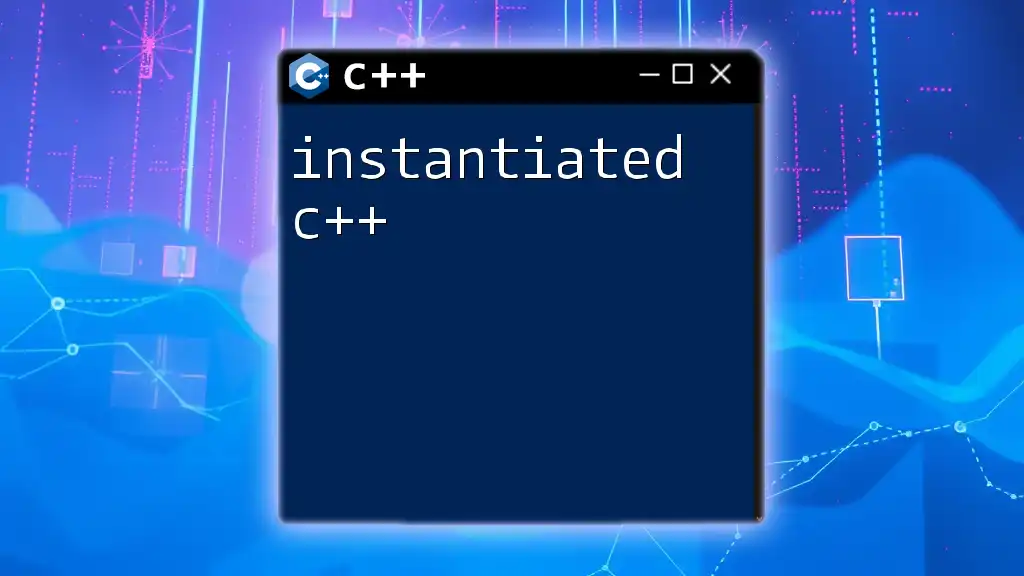
Conclusion
In summary, the Kalman Filter in C++ provides a powerful, versatile tool for state estimation in dynamic systems, effectively handling noise and uncertainty in measurements. This guide has provided a comprehensive look into the filter’s theoretical underpinnings, implementation details, and best practices for troubleshooting.
As you continue to develop your skills with Kalman filters, consider exploring nonlinear adaptations and real-world implementations that highlight the filter's capabilities in various sectors. With persistent practice, you will be well-equipped to leverage Kalman filters in your projects and applications.
Further Reading and Resources
For those looking to dive deeper into the subject, consider exploring recommended resources including textbooks, online tutorials, and communities that focus on Kalman filtering, control theory, and the application of these concepts in C++.