`uint32_t` in C++ is a fixed-width unsigned integer type that guarantees a size of 32 bits, making it ideal for representing non-negative integral values within a specific range.
Here's a simple code snippet demonstrating the declaration and initialization of a `uint32_t` variable:
#include <iostream>
#include <cstdint>
int main() {
uint32_t myNumber = 3000000000; // A large unsigned number
std::cout << "The value of myNumber is: " << myNumber << std::endl;
return 0;
}
What is `uint32_t`?
The `uint32_t` type is part of the fixed-width integer types introduced in C++11. It is defined in the `<cstdint>` header and represents an unsigned 32-bit integer. This means it can store whole numbers ranging from 0 to 4,294,967,295 (2^32 - 1). Using `uint32_t` provides clarity and ensures that your code behaves consistently across different platforms, as its size is precisely defined.
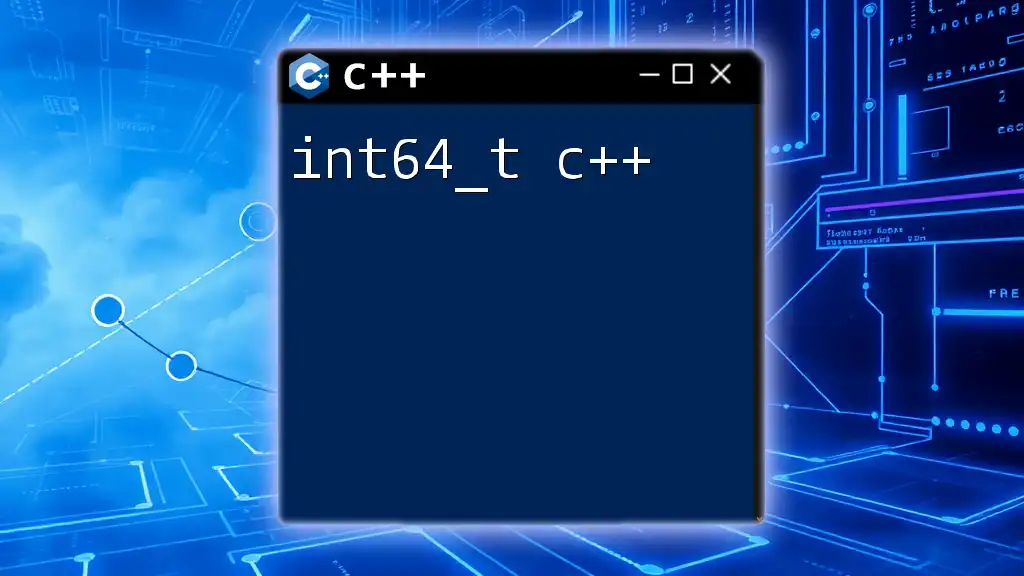
Why Use `uint32_t`?
Using `uint32_t` is beneficial for several reasons:
- Memory Efficiency: By explicitly defining the size of the integer, you can optimize memory usage in your applications, especially when handling large datasets.
- Portability: Different platforms may implement standard integer types (like `int`) with varying sizes. By using `uint32_t`, you ensure that your code will work the same way everywhere.
- Predictability: Since `uint32_t` guarantees a specific range, you avoid unexpected behaviors due to integer size discrepancies and overflow issues.
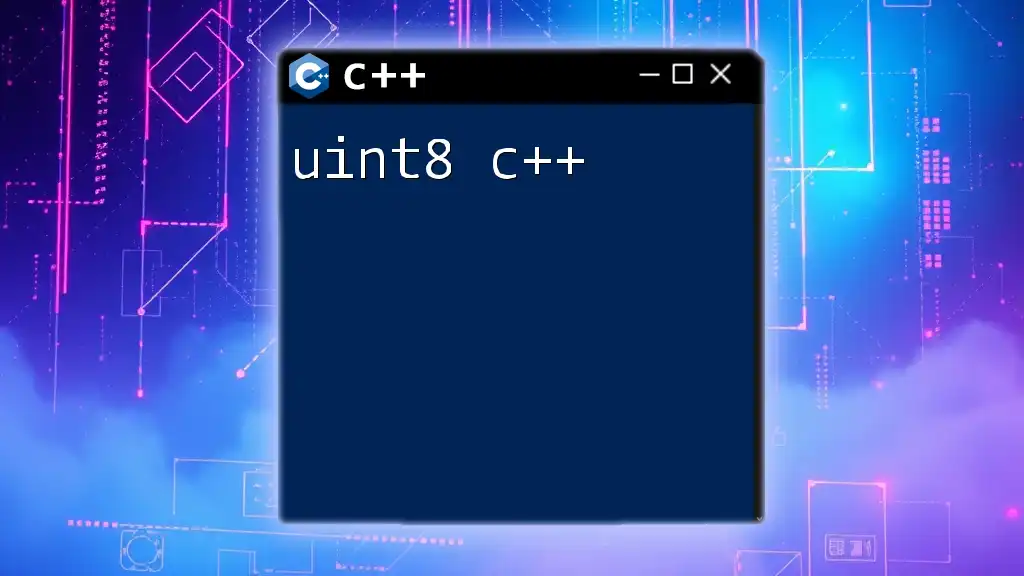
The Basics of `uint32_t`
Definition and Characteristics
The definition of `uint32_t` is straightforward; it's an unsigned integer type with a guaranteed width of exactly 32 bits. Its characteristics include:
- Range: `uint32_t` can represent values from 0 to 4,294,967,295. This is particularly useful when you only need non-negative numbers.
- Comparison with other types: Unlike `int`, which may vary in size from 16 to 64 bits depending on the compiler and platform, `uint32_t` remains constant and predictable.
Including the Required Headers
To use `uint32_t`, you need to include the following header in your program:
#include <cstdint>
This ensures that your code has access to all fixed-width integer types provided by C++.
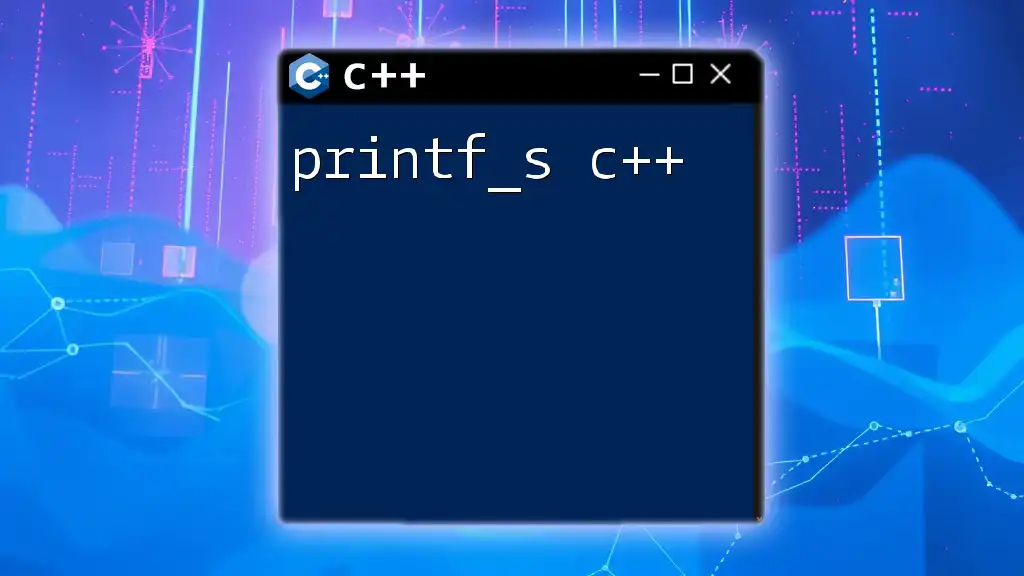
Working with `uint32_t`
Declaring `uint32_t` Variables
Declaring a `uint32_t` variable is simple and follows the syntax used for other variable types:
uint32_t myVar = 42;
This example initializes `myVar` with the value of 42. The syntax is straightforward and similar to declaring other integer types.
Basic Operations with `uint32_t`
Arithmetic Operations
`uint32_t` supports the standard arithmetic operations, including addition, subtraction, multiplication, and division. Here’s how you can perform basic arithmetic:
uint32_t a = 10;
uint32_t b = 5;
uint32_t sum = a + b; // sum = 15
uint32_t difference = a - b; // difference = 5
One crucial point to remember is that if the result of an arithmetic operation exceeds the maximum value of `uint32_t` (4,294,967,295), it will wrap around due to overflow, potentially leading to unexpected results.
Bit Manipulation
`uint32_t` is also commonly used for bit manipulation operations. Here’s a brief overview of bitwise operations:
uint32_t x = 0b00001111; // Initialize x with binary literal
uint32_t y = 0b11110000; // Initialize y with binary literal
uint32_t result = x & y; // result = 0b00000000 (AND operation)
Bitwise operators such as AND (`&`), OR (`|`), XOR (`^`), and NOT (`~`) are fundamental when interacting with hardware or performing low-level programming.
Comparison and Logical Operations
You can also perform comparisons using `uint32_t` with the usual comparison operators:
uint32_t x = 10;
uint32_t y = 20;
if (x < y) {
// Code block to execute when x is less than y
}
Logical operators (like `&&` for logical AND) can also be utilized when working with `uint32_t` in conditional statements.
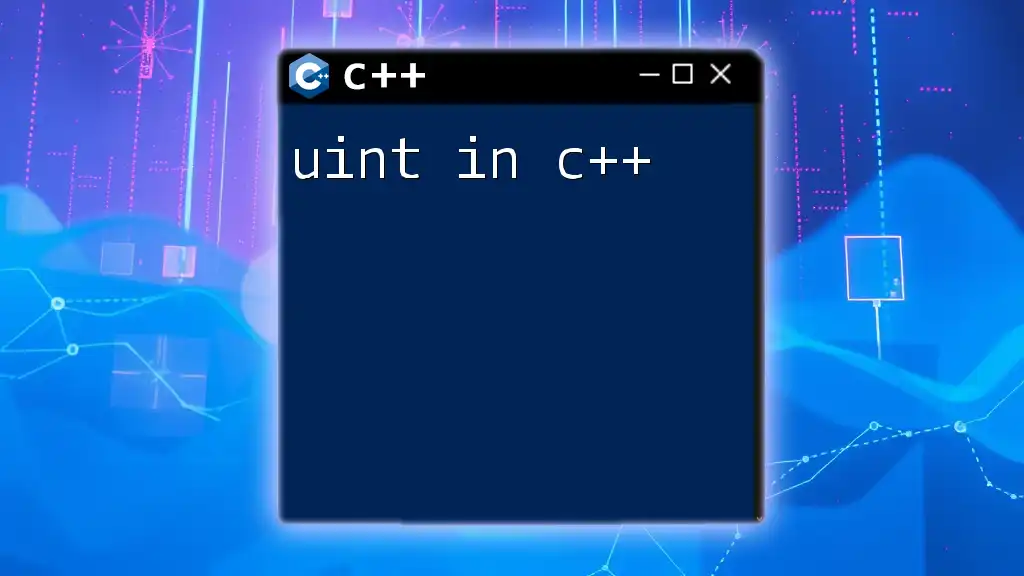
Advanced Uses of `uint32_t`
`uint32_t` in Functions
Defining functions that take `uint32_t` arguments is straightforward:
void add(uint32_t a, uint32_t b) {
uint32_t result = a + b;
// Function implementation
}
This allows you to leverage the benefits of fixed-width integers even within function parameters, ensuring consistency in calculations.
Using `std::vector<uint32_t>`
You can also use `uint32_t` within the Standard Template Library (STL). For instance, you can create a vector of `uint32_t` for dynamic arrays:
#include <vector>
std::vector<uint32_t> myVector;
This usage is common in applications where you need to store a collection of unsigned integers.
Combining `uint32_t` with Other Data Types
`uint32_t` can be combined with other C++ data types like structs or classes for more complex data modeling. Here’s an example:
struct MyData {
uint32_t id;
std::string name;
};
This struct can be useful for representing entities with an identifier and a name, all while maintaining efficiency and clarity.
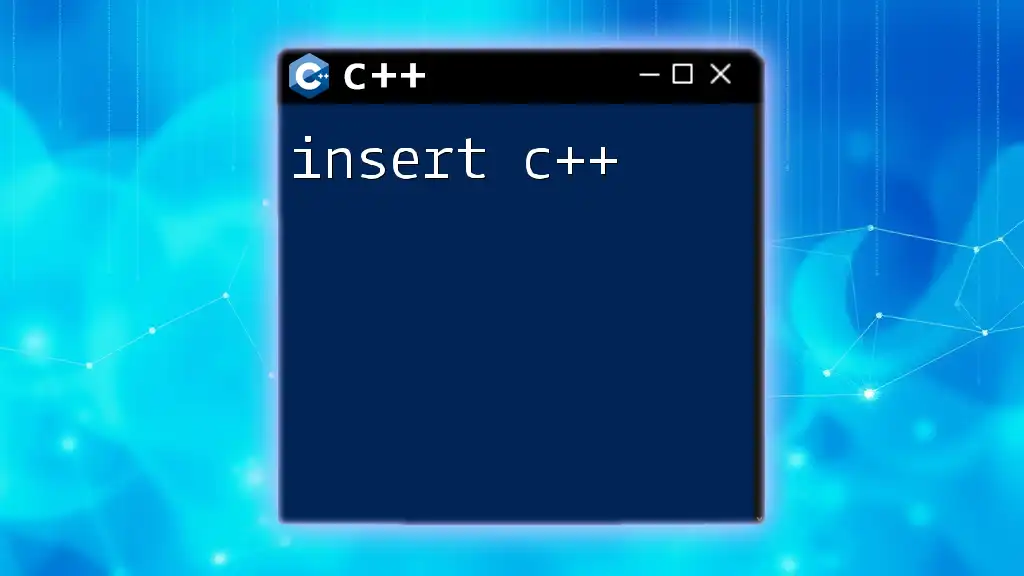
Best Practices and Common Pitfalls
When to Use `uint32_t` Over Other Types
Opt for `uint32_t` when you need:
- A guaranteed width for portability.
- An unsigned value, particularly in cases where negative values are logically impossible (like sizes and counts).
- Predictable behavior concerning overflow and range.
Potential Pitfalls
Be cautious of common errors while using `uint32_t`:
- Overflow/Underflow: If you're unsure of the value range expected, always check before performing operations to prevent wrap-around errors.
- Assuming Size Equality: Don't assume `uint32_t` is the same as `unsigned int`; it may vary by platform. Always rely on fixed-width types for critical applications requiring consistent behavior.
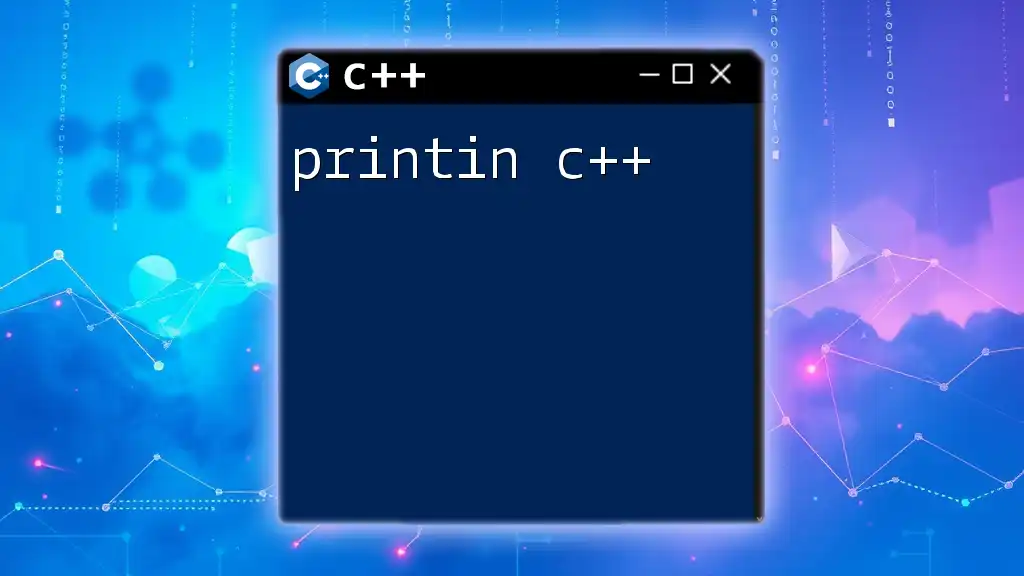
Conclusion
In summary, `uint32_t` is a powerful type in C++ that offers numerous benefits, especially in applications requiring strict control over integer sizes. By using `uint32_t`, you enhance the portability and predictability of your code. Explore its features and consider implementing `uint32_t` in your next C++ project to experience its full potential.
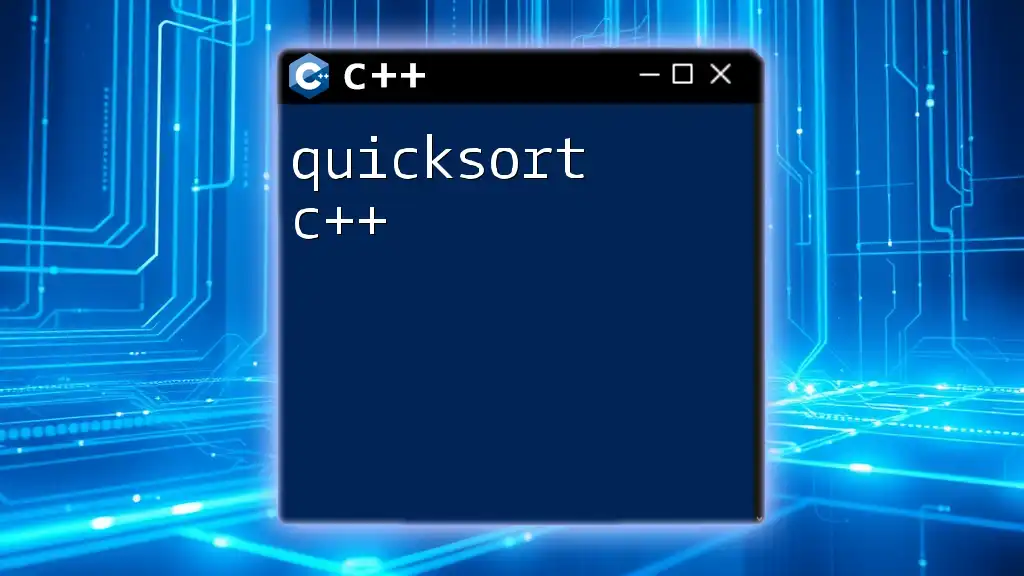
Call to Action
Dive into your own experiments with `uint32_t`! Write code snippets, test operations, and discover how this fixed-width integer can elevate your C++ programming experience. Share your findings and queries in the comments section; we’d love to hear what you’ve learned!