The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, often starting with 0 and 1; here's a simple C++ code snippet to compute it:
#include <iostream>
using namespace std;
int fibonacci(int n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
int main() {
int n = 10; // Example: Get the 10th Fibonacci number
cout << "Fibonacci(" << n << ") = " << fibonacci(n) << endl;
return 0;
}
Understanding the Fibonacci Sequence
Fibonacci numbers form a sequence where each number is the sum of the two preceding ones. The sequence typically starts with 0 and 1, resulting in the sequence: 0, 1, 1, 2, 3, 5, 8, 13, ... The mathematical formula that defines the Fibonacci numbers is provided as follows:
F(n) = F(n-1) + F(n-2)
Thus, F(0) = 0, F(1) = 1, F(2) = 1, F(3) = 2, and so on. This simple recursive relationship lays the groundwork for programming Fibonacci calculations.
The Fibonacci sequence exhibits intriguing properties and patterns, including the golden ratio. As the sequence progresses, the ratio of consecutive Fibonacci numbers approaches approximately 1.61803398875 (the golden ratio, φ). This observation connects mathematics, art, nature, and even markets, leading to practical applications.
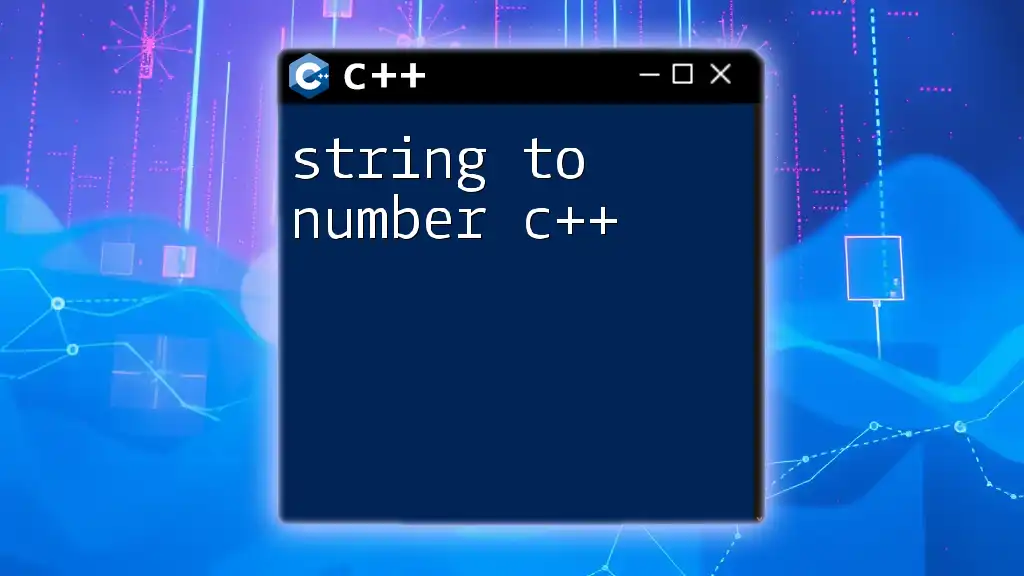
Fibonacci in C++: An Overview
Using C++ for implementing Fibonacci computations offers several benefits primarily related to performance and efficiency. C++ is a statically typed language that is known for its speed, which becomes apparent when performance matters, especially for larger sequences.
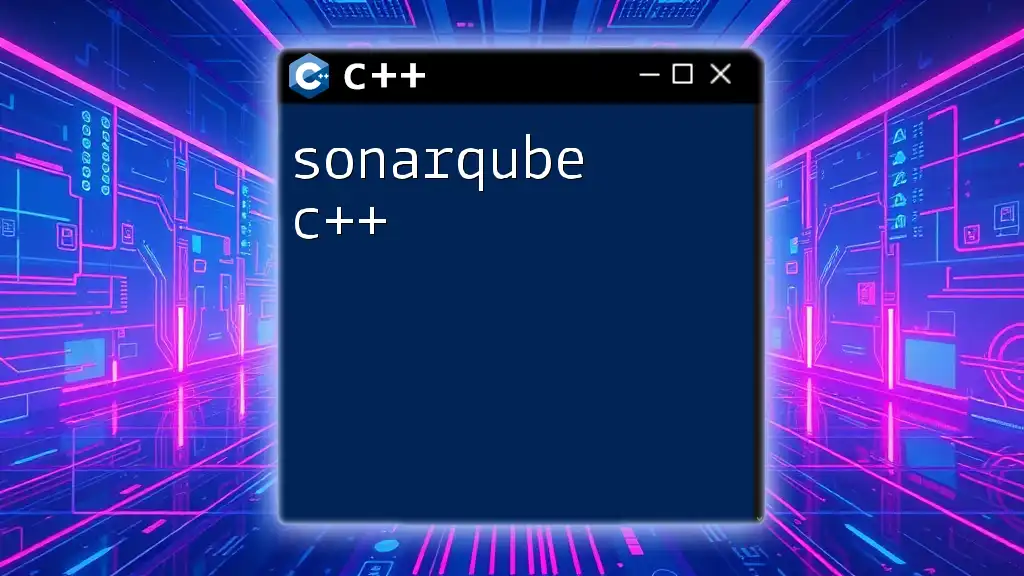
Implementing the Fibonacci Sequence in C++
Basic Iterative Fibonacci Code
One of the simplest methods for generating Fibonacci numbers is the iterative approach. Below is a C++ implementation:
#include <iostream>
using namespace std;
void fibonacciIterative(int n) {
int a = 0, b = 1, c;
cout << "Fibonacci Series: ";
for (int i = 0; i < n; ++i) {
cout << a << " ";
c = a + b; // Sum of the last two numbers
a = b;
b = c; // Update the last two numbers for the next iteration
}
cout << endl;
}
In this code, we initialize the first two Fibonacci numbers (0 and 1) and use a loop to calculate the subsequent numbers by summing the last two. This iterative implementation is straightforward and efficient, particularly for generating a fixed number of Fibonacci numbers.
While iteration is usually more performant than its recursive counterpart, it's essential to understand the recursive process, too.
Recursive Fibonacci Code
A classic recursive approach to calculating Fibonacci numbers is implemented as follows:
#include <iostream>
using namespace std;
int fibonacciRecursive(int n) {
if (n <= 1) return n; // Base case
return fibonacciRecursive(n - 1) + fibonacciRecursive(n - 2); // Recursive call
}
void printFibonacciRecursive(int n) {
cout << "Fibonacci Series: ";
for (int i = 0; i < n; ++i) {
cout << fibonacciRecursive(i) << " "; // Generate Fibonacci numbers recursively
}
cout << endl;
}
In this example, if the input is less than or equal to 1, we return that value. Otherwise, we recursively call the function, adding the results of the previous two Fibonacci numbers.
While elegant, this recursive method's performance can diminish for larger input sizes due to repeated calculations of the same Fibonacci numbers—a major downside compared to iteration.
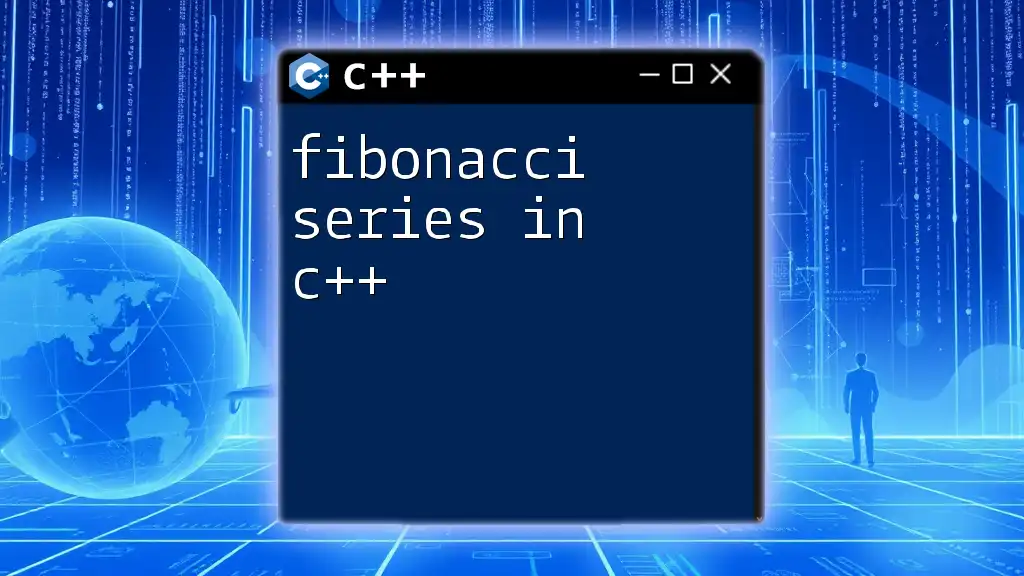
Optimizing Fibonacci Code in C++
To leverage better performance, especially for larger values of `n`, we can employ advanced techniques like dynamic programming.
Dynamic Programming Approach
Dynamic programming saves previously computed values for quick results, minimizing repeated calculations. Below is how this can be implemented in C++:
#include <iostream>
using namespace std;
int fibonacciDynamic(int n) {
int f[n+2]; // 1 extra to handle case when n is 0
f[0] = 0;
f[1] = 1;
for (int i = 2; i <= n; i++) {
f[i] = f[i-1] + f[i-2]; // Building from previously computed values
}
return f[n];
}
This implementation maintains an array `f` that fills using previous Fibonacci numbers. This saves time significantly, especially for large inputs, as each number is calculated just once.
Using Matrix Exponentiation for Fibonacci Numbers
A more sophisticated approach leverages matrix exponentiation. The nth Fibonacci number can be derived from a transformation matrix, leading to logarithmic time complexity.
#include <iostream>
using namespace std;
void multiply(int F[2][2], int M[2][2]) {
int x = F[0][0] * M[0][0] + F[0][1] * M[1][0];
int y = F[0][0] * M[0][1] + F[0][1] * M[1][1];
int z = F[1][0] * M[0][0] + F[1][1] * M[1][0];
int w = F[1][0] * M[0][1] + F[1][1] * M[1][1];
F[0][0] = x;
F[0][1] = y;
F[1][0] = z;
F[1][1] = w;
}
void power(int F[2][2], int n) {
int M[2][2] = {{1, 1}, {1, 0}};
if (n == 0 || n == 1) return;
power(F, n / 2); // Recursively square the matrix
multiply(F, F);
if (n % 2 != 0) multiply(F, M); // If n is odd
}
int fibonacciMatrix(int n) {
int F[2][2] = {{1, 1}, {1, 0}};
if (n == 0) return 0;
power(F, n - 1); // Raise matrix to the power of (n-1)
return F[0][0]; // Top left cell contains the nth Fibonacci number
}
In this method, the transformation matrix is raised to the power of `n-1`, allowing for the efficient calculation of Fibonacci numbers without direct iteration or recursion.
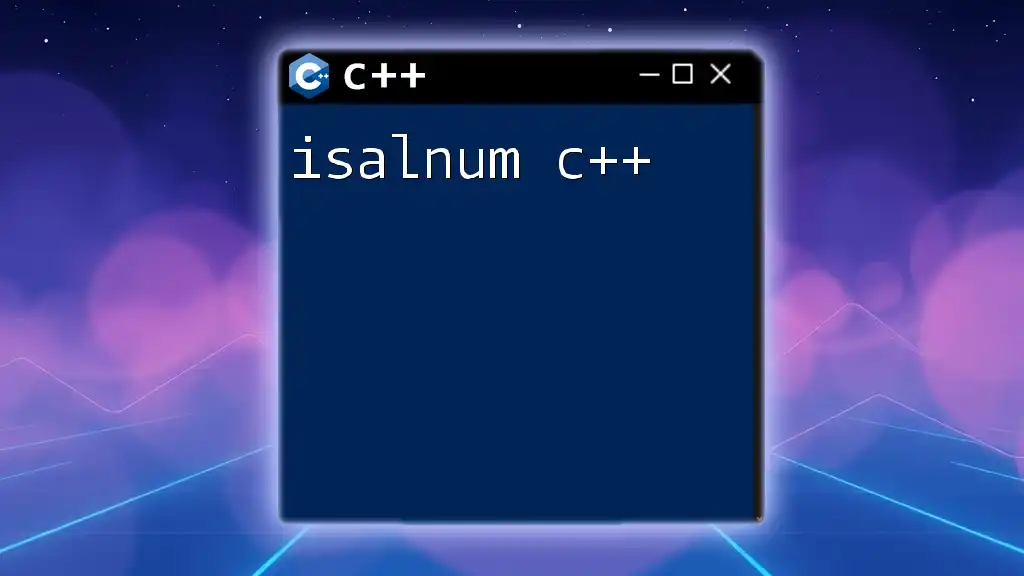
Real-World Applications of Fibonacci Numbers in C++
Fibonacci numbers find applications beyond just theoretical interest. For instance:
- In algorithm design, Fibonacci numbers are pivotal in constructing data structures like Fibonacci heaps, which optimize priority queue functionalities.
- Fibonacci numbers manifest in various natural phenomena, such as population growth models, computer algorithms, and financial markets, providing essential insights into more profound patterns in nature and humanity.
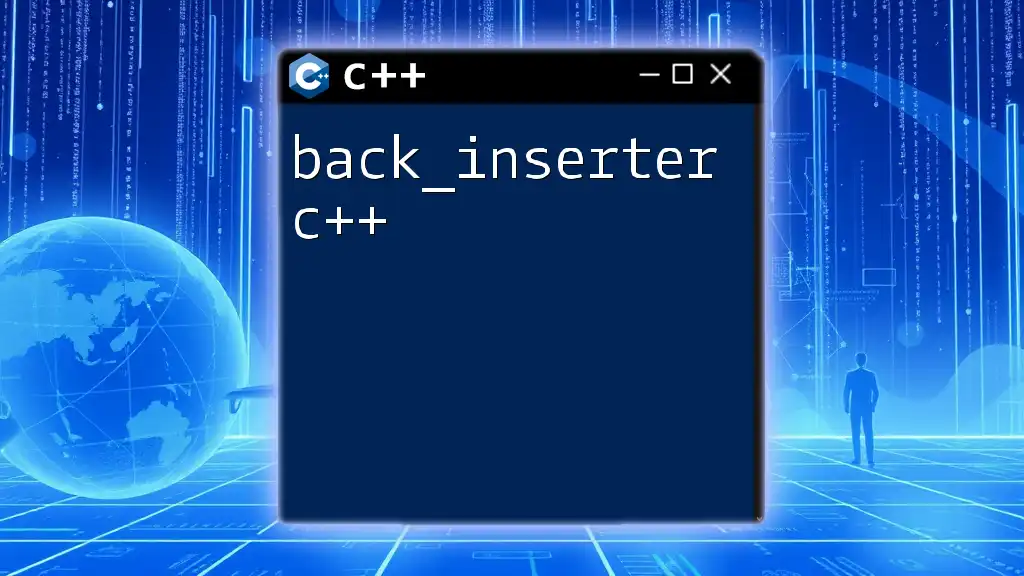
Conclusion
Fibonacci numbers represent a significant concept in C++ programming, showcasing various approaches from iterative and recursive methods to advanced matrix exponentiation techniques. By mastering these techniques, programmers can efficiently compute Fibonacci numbers, leading to improved algorithm efficiency and real-world applications. The invitation stands for you to explore these methods further and share your Fibonacci implementations, contributing to the vibrant coding community!
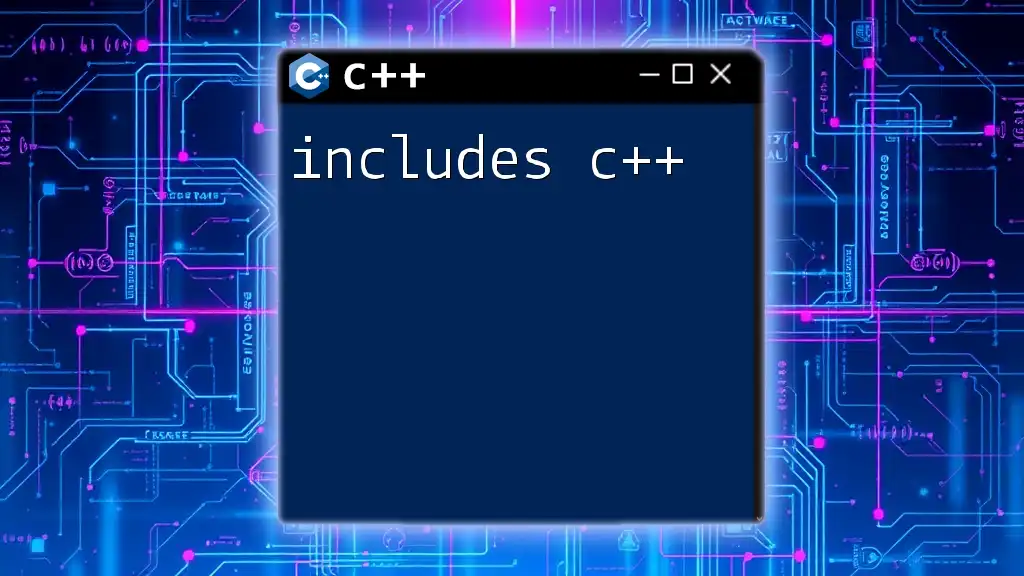
Additional Resources
For further reading, consider diving into books on C++ programming, online courses related to algorithm design, and tutorials focusing on recursion or dynamic programming as these will solidify your understanding and skills related to Fibonacci numbers in C++.