In C++, `cin.fail()` is a member function that checks if the last input operation failed, which can occur due to invalid input types or stream errors.
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
if (cin.fail()) {
cout << "Invalid input! Please enter a valid number." << endl;
cin.clear(); // Clear the error flag
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Remove invalid input
} else {
cout << "You entered: " << number << endl;
}
return 0;
}
Understanding cin in C++
What is cin?
In C++, `cin` is an object from the `iostream` library that stands for "character input." It is primarily used for reading data from the standard input, typically the keyboard. As part of C++’s input/output model, `cin` is integral for interacting with users and receiving their input in various formats, including integers, floating-point numbers, and strings.
The Role of cin.fail()
The `cin.fail()` function is crucial in the realm of input validation and error handling. It checks the state of the `cin` input stream when reading data. If the last input operation encountered an error—such as a type mismatch—`cin.fail()` returns `true`. This allows developers to catch input errors gracefully, ensuring that the program does not proceed with invalid data.
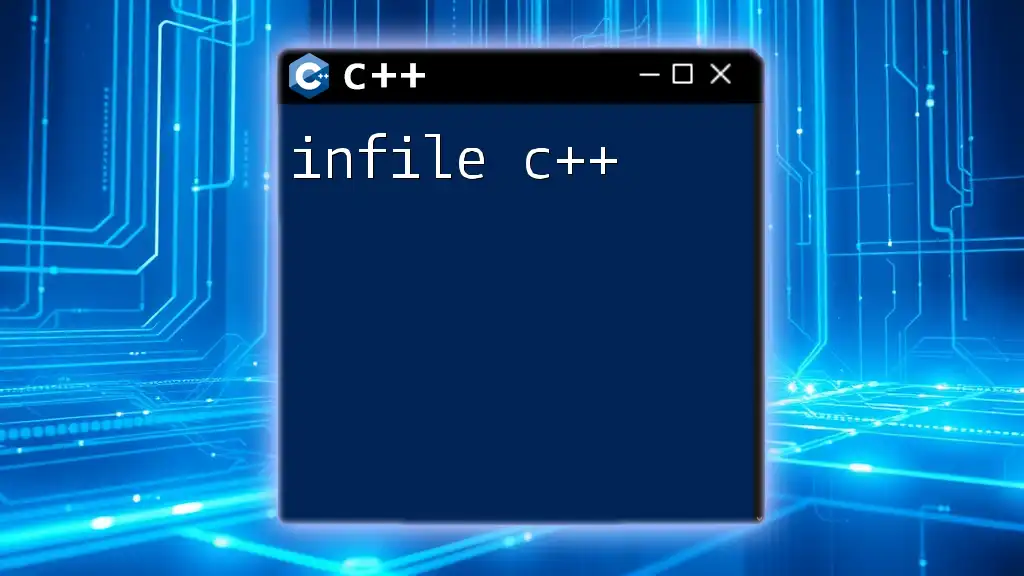
When Does cin.fail() Occur?
Types of Input Errors
Errors can occur for several reasons when using `cin`:
- Data Type Mismatches: For instance, if a user tries to enter a string when an integer is expected, the input operation will fail.
- Stream State Errors: These may occur when unexpected end-of-file conditions happen, or if the input stream is in an invalid state due to a previous failure.
Example of cin.fail() Triggering
Consider the following code snippet, where we prompt the user for an integer:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Please enter an integer: ";
cin >> number;
if (cin.fail()) {
cout << "Error: input was not an integer." << endl;
}
return 0;
}
Explanation: In this example, if a user types a letter instead of a number, the `cin.fail()` method will trigger, as the input does not conform to the expected integer type. This illustrates how `cin.fail()` is on the front lines of input validation.
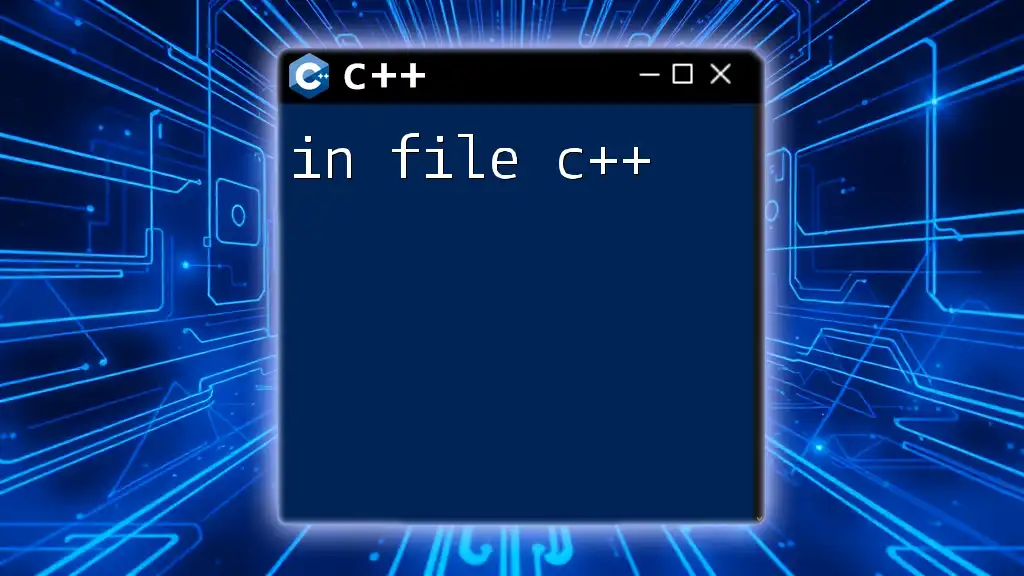
How to Use cin.fail() Effectively
Basic Usage
To effectively use `cin.fail()`, you should always check it immediately after an input operation. The syntax is straightforward:
if (cin.fail()) {
// Handle failure
}
Handling Input Errors
When `cin` fails due to invalid input, you must reset the error state to continue processing input successfully. This is done using `cin.clear()` to clear the failure flag. Additionally, it's critical to clear the input buffer to remove any extraneous characters using `cin.ignore()`.
Example of Error Handling
Here’s a complete example that incorporates robust error handling:
#include <iostream>
#include <limits>
using namespace std;
int main() {
int number;
while (true) {
cout << "Please enter an integer: ";
cin >> number;
if (cin.fail()) {
cin.clear(); // Clear the error state
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Discard invalid input
cout << "Invalid input. Please try again." << endl;
} else {
cout << "You entered: " << number << endl;
break; // Exit the loop if input is valid
}
}
return 0;
}
Explanation: In this snippet, the program prompts the user for an integer, checks for input validity, and utilizes both `cin.clear()` to reset the input state and `cin.ignore()` to remove invalid input. This structure allows users to repeatedly attempt input until successful, enhancing the program's resilience.
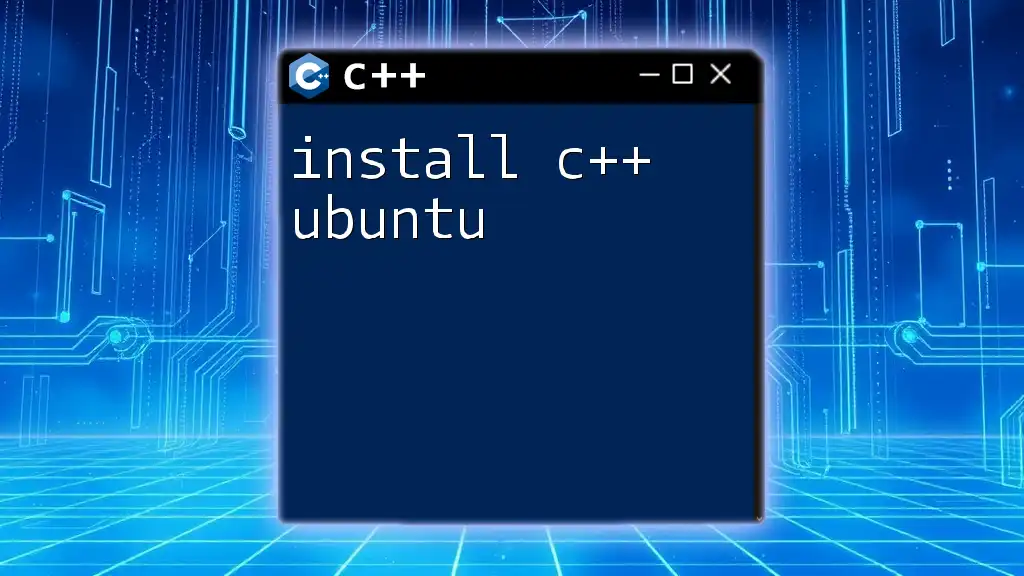
Common Mistakes When Using cin.fail()
Failing to Check for Fail State
One common oversight is neglecting to check for the fail state after input operations. This can lead to programs that operate with invalid data, potentially causing significant bugs or crashes during execution.
Misusing cin.clear() and cin.ignore()
Another frequent mistake is mismanaging `cin.clear()` and `cin.ignore()`. It is essential to clear the error state before attempting further input and to correctly ignore the buffer to avoid infinite loops or processing stale input. Being diligent in these practices is key to effective input handling.
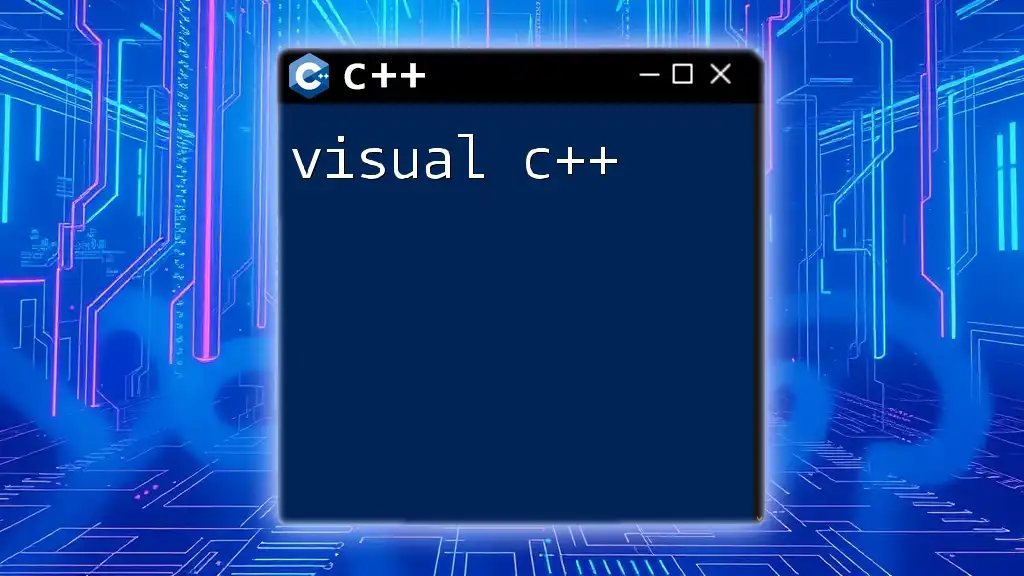
Best Practices for Using cin and cin.fail()
Input Validation Techniques
It is vital to validate user input consistently to ensure your application can handle unexpected data without failure. One effective strategy is utilizing loops that continue to prompt the user until a valid input is received. This not only improves program robustness but also enhances user experience.
Encouraging User-Friendly Errors
Customizing error messages can promote a better user experience. Informative messages can guide users about what went wrong and how they can correct their input. Interactive prompts encourage users to engage with the program and refine their input for clearer communication.
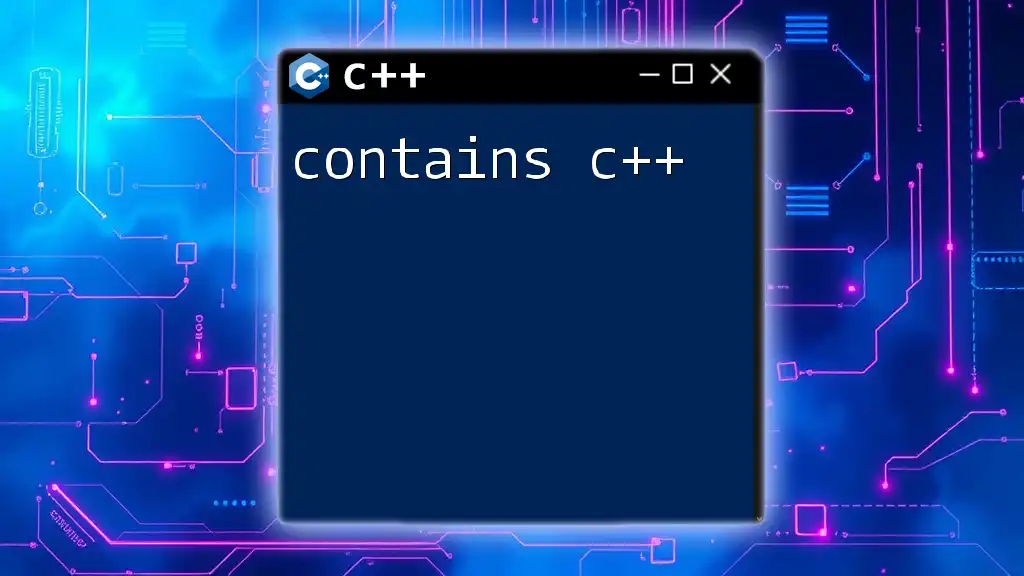
Conclusion
In summary, understanding and effectively utilizing `cin.fail()` is imperative for managing input errors in C++. With diligent error handling practices—such as checking the state of `cin`, clearing the error flag, and providing user-friendly messages—you can significantly improve the reliability and robustness of your C++ applications. Practice using these techniques to build familiarity and confidence in handling various input scenarios.