To install C++ on Windows, you can use the MinGW installer to set up the environment quickly by downloading it from the official site and using the command line to set the PATH variable.
Here's how to add MinGW to your system's PATH:
setx PATH "%PATH%;C:\MinGW\bin"
Understanding C++ Compilers
What is a C++ Compiler?
A C++ compiler is a special program that converts C++ source code into machine code, which can be executed by your computer's CPU. This process is crucial because without a compiler, your code, though well-written, remains in a format that the machine cannot understand. The compiler processes the high-level language, optimizing the commands and translating them into binary format, thus enabling execution.
Popular C++ Compilers for Windows
Several C++ compilers are widely used among developers on Windows. Understanding the differences can help you make an informed decision about which one to install:
-
GCC (GNU Compiler Collection): A powerful and versatile compiler suite that works not just with C++, but with several other programming languages. It's highly regarded for its performance and optimization capabilities.
-
Visual Studio Compiler (MSVC): Developed by Microsoft, MSVC is integrated into the Visual Studio IDE. It’s a popular choice for professionals because of its seamless integration with code-editing tools and extensive debugging features.
-
MinGW (Minimalist GNU for Windows): This is a native Windows port of the GCC, providing a more lightweight option. It’s particularly popular among developers who need a quick setup without too much overhead.
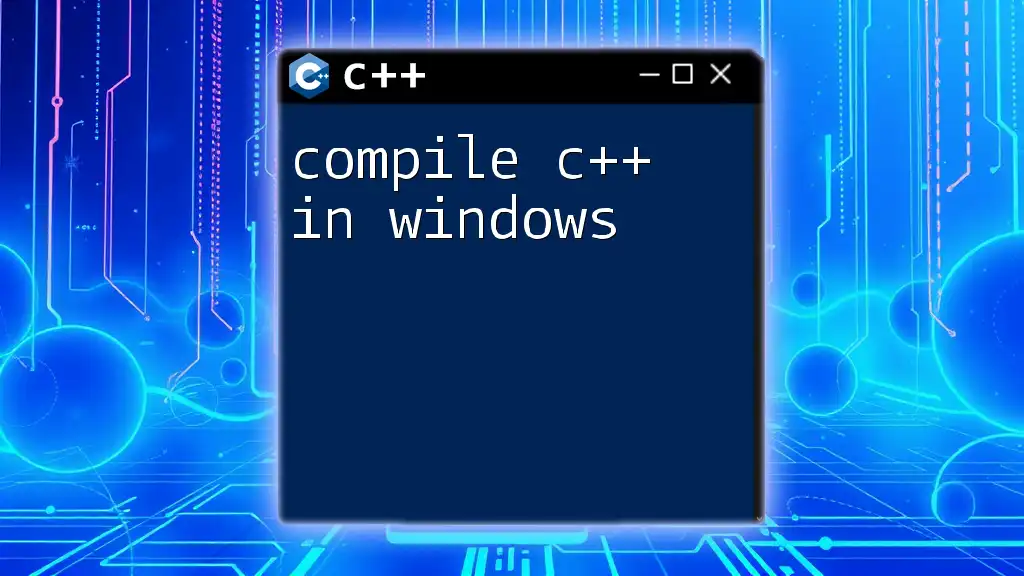
Prerequisites
System Requirements
Before you proceed to install C++ on Windows, ensure that your system meets some basic requirements:
-
Operating System: Windows 10 or higher is recommended for the best compatibility with modern compilers.
-
RAM & CPU: A minimum of 4GB RAM is advisable, although more is recommended for larger projects. A multi-core processor will provide better performance.
-
Storage: Prepare at least 1GB of free disk space for installation, as some compilers and IDEs can require additional space for libraries and dependencies.
Checking Your System
To verify your Windows version, follow these steps:
- Press the `Windows key + R` to open the Run dialog.
- Type `winver` and press `Enter`. This will display your current version of Windows.
If you already have Visual Studio installed, you can check its version by opening the application and navigating to Help > About Microsoft Visual Studio.
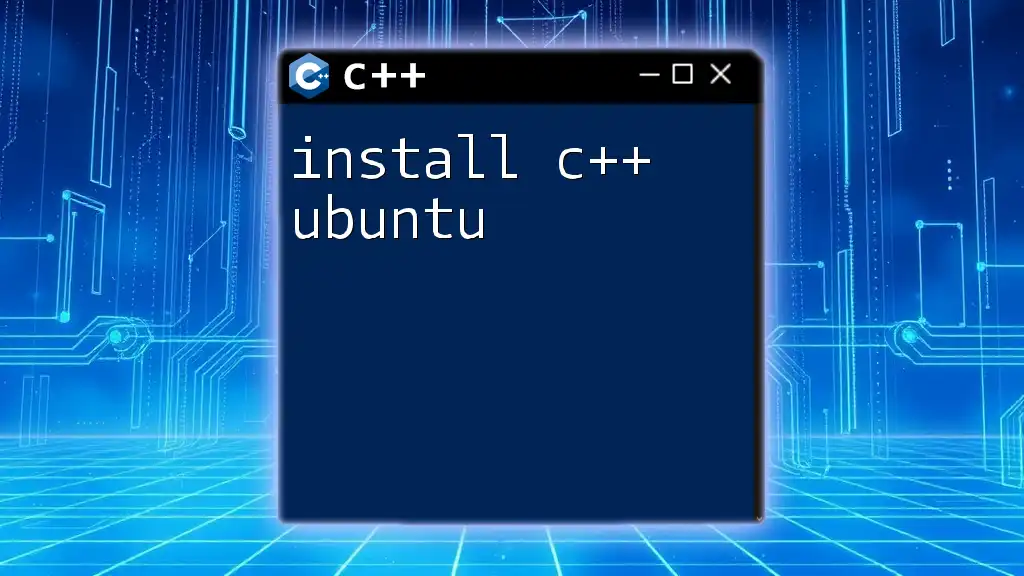
Installing a C++ Development Environment
Using Microsoft Visual Studio
Downloading Visual Studio
To start with Microsoft Visual Studio, visit the official [Visual Studio website](https://visualstudio.microsoft.com/) for downloading the IDE. Here, you'll see various editions. For beginners, the Community Edition is free and provides all the essential features needed for C++ development.
Installation Steps
Once you’ve downloaded the installer:
- Launch the Installer: Run the downloaded executable file.
- Choose Your Workload: You'll be prompted to select a workload. For C++, select the Desktop development with C++ workload. This ensures all necessary components for C++ development are included in the installation.
- Modify Installation: If you need specific tools or libraries, you can modify the installation to include those.
- Click Install: Proceed with the installation, and wait for the process to complete.
After installation, Visual Studio will allow you to create C++ projects easily, with numerous templates available for quick access.
Installing MinGW
Downloading MinGW
To install MinGW, visit the [MinGW website](http://mingw.org/) and select the appropriate installer. The most common option is the mingw-get-setup.exe.
Setting up MinGW
- Run the Installer: After downloading, run the installer.
- Select Packages: It’s essential to choose the packages for C++. Select `mingw32-base` and `mingw32-gcc-g++`. Ensure these packages are checked.
- Installation Directory: During the installation, it’s best to install MinGW in a directory without spaces in its path, like `C:\MinGW`.
Once installation is complete, you'll need to add MinGW to the system PATH:
Add the following line to your PATH environment variable:
setx PATH "%PATH%;C:\MinGW\bin"
This allows you to access the MinGW compiler from any command prompt window.
Installing Cygwin (Optional)
What is Cygwin?
Cygwin is a significant tool that provides a Unix-like environment on Windows. It's particularly beneficial for developers familiar with Unix commands and tools.
Installation Steps for Cygwin
- Download Cygwin: Go to the [Cygwin website](https://www.cygwin.com/) and download the setup executable.
- Run the Installer: Launch the installer and select a mirror to download packages.
- Select Packages: Choose the Devel category and make sure to select `gcc-g++` to install the C++ compiler along with the required tools.
- Finish Installation: Proceed through the rest of the installation steps.
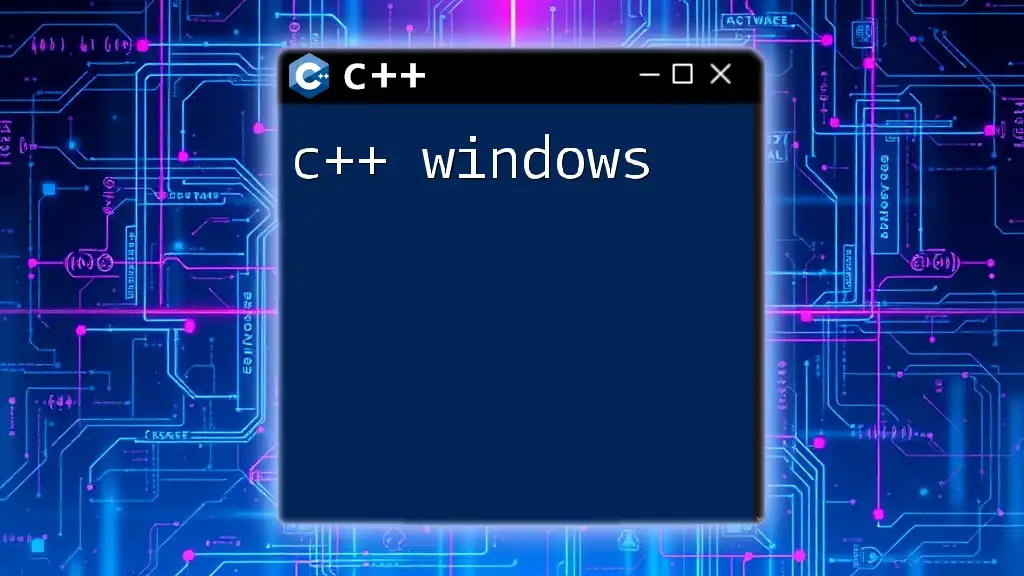
Writing Your First C++ Program
Setting Up Your IDE/Editor
Once you have installed a compiler, you need to choose an IDE or text editor to write your C++ code. Some popular choices are:
- Code::Blocks: An open-source IDE that provides a simple and user-friendly interface for C++ programming.
- Eclipse: Known for its extensibility, Eclipse can be configured for C++ programming with the appropriate plugins.
- Visual Studio Code: A lightweight code editor that supports numerous extensions, including powerful C++ tools.
Creating a Sample Program
Let’s write a simple Hello World program to confirm that your installation works correctly. Open your IDE or text editor and create a new file named `hello.cpp`. Enter the following code:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Compiling and Running Your Program
To compile and run your program, follow these steps based on your setup:
-
If you are using MinGW:
- Open a command prompt.
- Navigate to the directory that contains `hello.cpp`.
- Compile your program using:
g++ hello.cpp -o hello
- Run the executable:
./hello
-
If you are using Visual Studio, you can simply press `Ctrl + F5` to run your program directly.
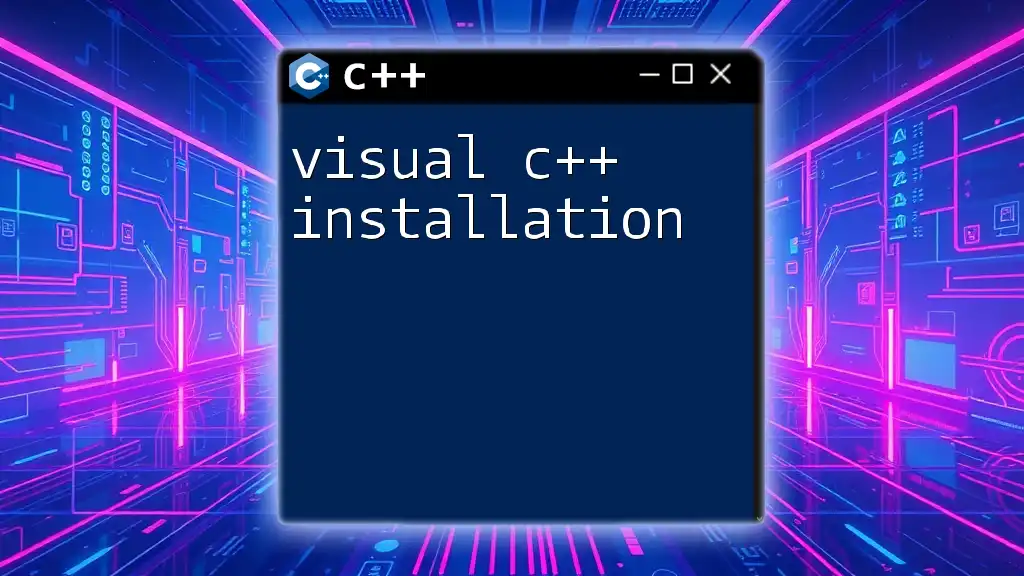
Troubleshooting Common Installation Issues
Compiler Not Found
If you encounter a message stating that the compiler was not found, ensure that you have added the compiler’s bin directory to your PATH environment variable correctly. Restart the command prompt or IDE to apply changes.
Setting Environment Variables
Correct environment variables are vital for the compiler tools to function properly. Verify your system's PATH variable includes the path to your compiler's bin directory (like `C:\MinGW\bin` for MinGW).
Incompatibility Issues
Incompatibility issues may arise due to different versions of Windows or installed components. Always ensure you download versions compatible with your Windows environment.
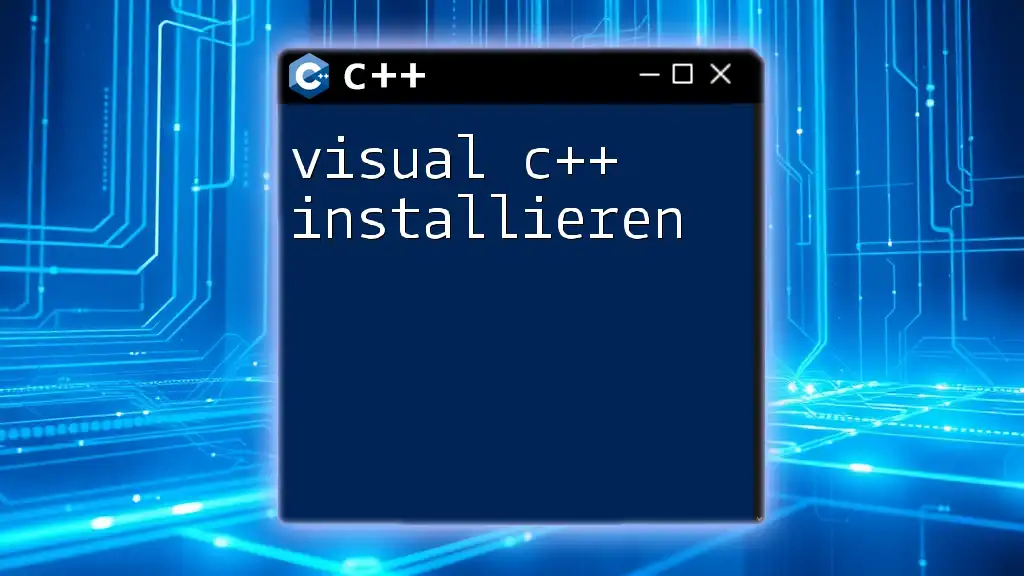
Conclusion
Having successfully completed the steps to install C++ on Windows, you are well on your way to begin coding. A proper installation sets the foundation for writing efficient, powerful C++ programs.
As you explore more C++ concepts and libraries, this environment will be essential in your development journey. Embrace the challenges and dive into the resources available; the world of C++ awaits you!
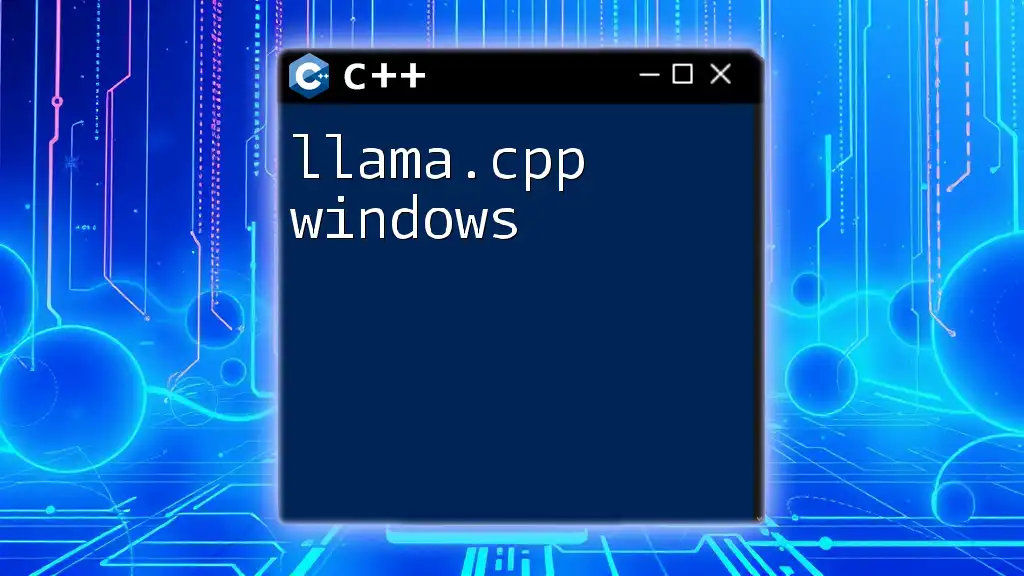
FAQs
Most Common Questions
-
What version of C++ should I install on Windows? Generally, installing the latest stable versions of compilers like GCC or MSVC is recommended to have access to the most up-to-date features.
-
Can I install multiple compilers? Yes, you can install multiple compilers, but make sure they don’t conflict in your PATH settings.
-
Are there free alternatives to Visual Studio? Absolutely! Options like Code::Blocks and Eclipse provide excellent free solutions for C++ development.
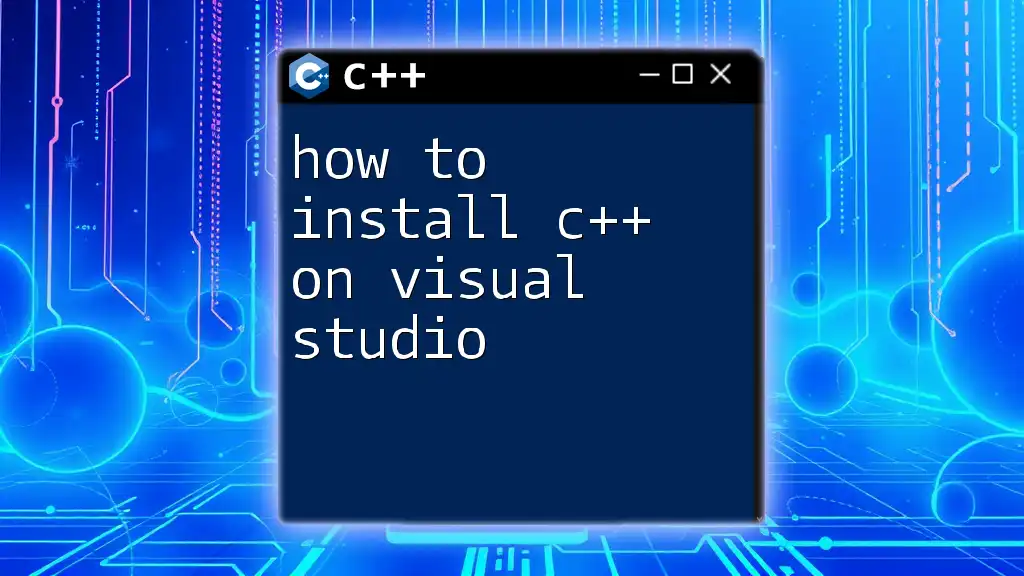
Additional Resources
For further exploration, consider visiting the official documentation for each compiler or enrolling in online courses dedicated to mastering C++. Your journey into C++ programming is just beginning!