The `arc` command in C++ is used to create a rounded arc shape, commonly utilized in graphical applications to draw curves.
Here's a simple example of how to use the arc command in C++ with the SFML library:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Arc Example");
sf::CircleShape arc(100, 100); // Radius and point count
arc.setPointCount(30);
arc.setFillColor(sf::Color::Transparent);
arc.setOutlineColor(sf::Color::Red);
arc.setOutlineThickness(5);
arc.setPosition(300, 200); // Set position of arc
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(arc); // Draw the arc
window.display();
}
return 0;
}
Overview of `std::shared_ptr`
What is `std::shared_ptr`?
`std::shared_ptr` is a smart pointer in C++ that manages shared ownership of a dynamically allocated object. Contrary to raw pointers, which can result in memory leaks and dangling pointers, `shared_ptr` automatically handles memory deallocation when its last owner is destroyed or reset.
In comparison to other smart pointers, like `std::unique_ptr`, which has sole ownership, `std::shared_ptr` allows multiple pointers to point to the same object. This makes it an ideal choice in scenarios involving shared resource setups, such as in data structures or singletons.
Understanding Ownership
The concept of ownership in `std::shared_ptr` hinges on reference counting. Every `shared_ptr` maintains a reference count to track how many pointers are sharing ownership of the object it points to. When a new `shared_ptr` is created from an existing one, the reference count increases. Conversely, when a `shared_ptr` is destroyed, the count decreases. Once the count reaches zero, the managed object is automatically deleted.
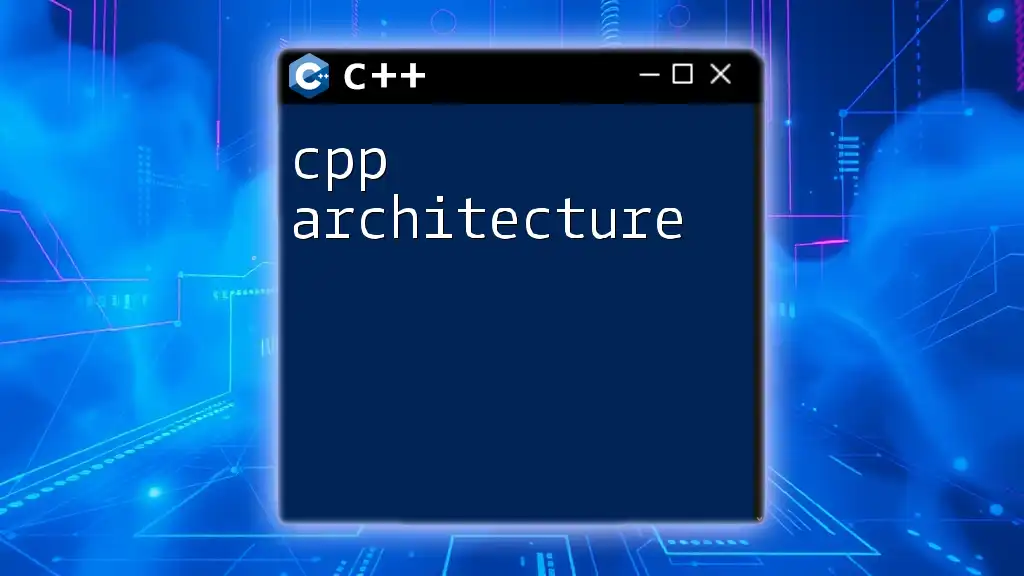
Key Features of `std::shared_ptr`
Automatic Memory Management
One of the major advantages of `std::shared_ptr` is automatic memory management. When the last reference to the managed object goes out of scope or is reset, the destructor is automatically called, freeing up memory and preventing memory leaks.
For instance, you can create a `shared_ptr` like this:
#include <memory>
std::shared_ptr<int> ptr1 = std::make_shared<int>(10);
This line not only allocates memory for an integer but also wraps it in a `shared_ptr`, ensuring garbage collection once it is no longer needed.
Standard Operations on `std::shared_ptr`
Common operations with `std::shared_ptr` include:
-
Creating a `shared_ptr`: As shown above, the recommended way to create a `shared_ptr` is using `std::make_shared`.
-
Copying and Assigning `shared_ptr`: When you copy a `shared_ptr`, both pointers will point to the same object, and the reference count will increment:
std::shared_ptr<int> ptr2 = ptr1; // Both ptr1 and ptr2 point to the same int
This seamless sharing is one of the key power features of `std::shared_ptr`.
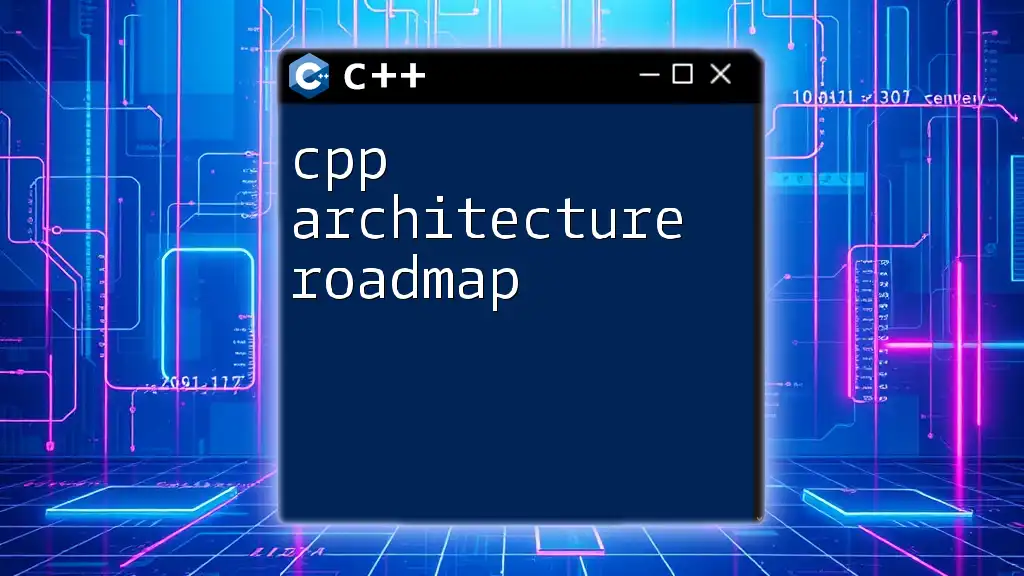
When to Use `std::shared_ptr`
Use Cases for `shared_ptr`
Due to its shared ownership model, `shared_ptr` excels in situations like:
- Shared data structures: Such as trees or linked lists where multiple nodes might point to the same child or parent nodes.
- Resource management in APIs: Managing resources whose lifespan may exceed that of the calling context.
Performance Considerations
While the use of `std::shared_ptr` provides many advantages, it does come with a performance cost. The overhead of reference counting can result in slower performance compared to raw pointers or `std::unique_ptr`. Therefore, it is essential to assess the use case and select the most efficient smart pointer based on the ownership model of your application.
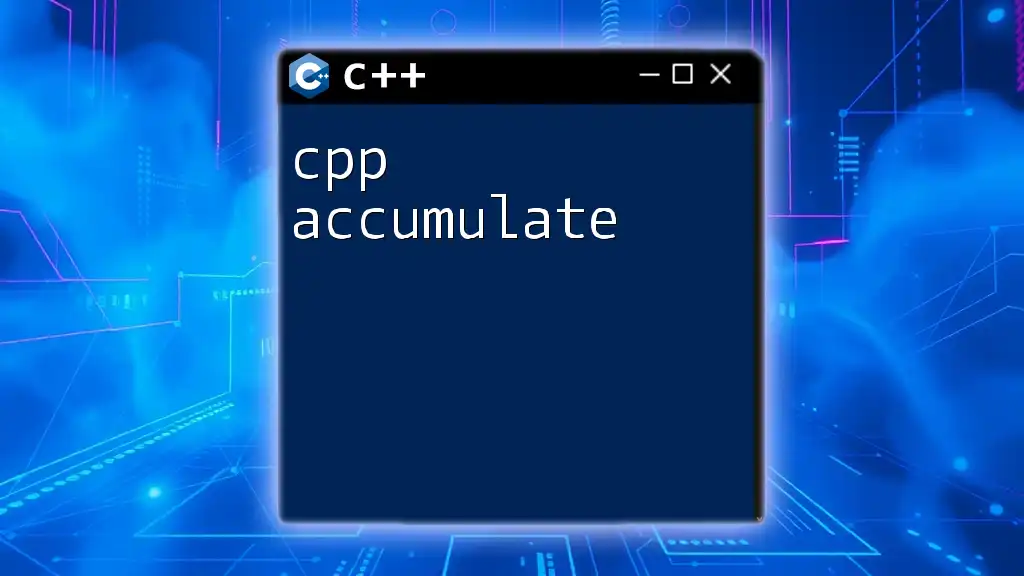
Best Practices for Using `std::shared_ptr`
Avoiding Circular References
One common pitfall of `std::shared_ptr` usage is creating circular references, where two `shared_ptr` instances point to each other, preventing memory from being freed.
For example, consider the following structure:
struct Node {
std::shared_ptr<Node> next; // Circular reference
};
In such cases, the memory will not be released because each `Node` holds a reference to another. It's best to utilize `std::weak_ptr` to break this cycle.
Using `std::make_shared`
Using `std::make_shared` is highly recommended over manually allocating a `shared_ptr` using `new`. It performs the allocation in a single operation, which is more efficient:
std::shared_ptr<int> ptr = std::make_shared<int>(10);
This function call optimally combines the memory allocation of both the control block (which holds the reference count) and the managed object.
Combining `std::shared_ptr` with Containers
`shared_ptr` can be effectively stored in standard library containers like `std::vector` or `std::map`. Utilizing smart pointers in this fashion allows you to manage collections of dynamically allocated objects without worrying about manual memory management, as shown below:
std::vector<std::shared_ptr<int>> vec;
vec.push_back(std::make_shared<int>(5));
This ensures that all pointers are automatically cleaned up when they go out of the vector's scope.
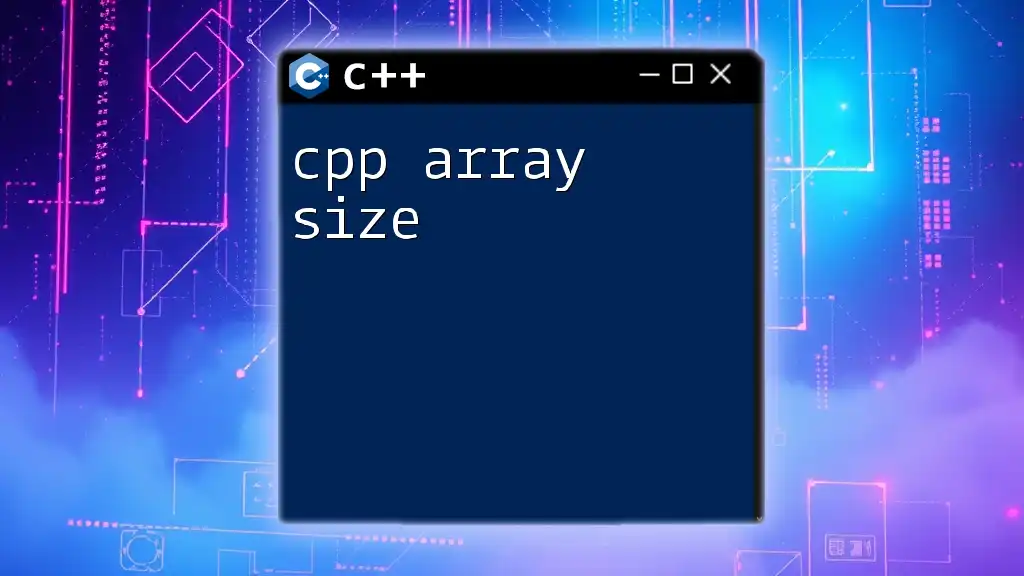
Common Mistakes with `std::shared_ptr`
Misusing `shared_ptr` and Raw Pointers
A frequent mistake is mixing `shared_ptr` and raw pointers improperly. For instance, directly assigning a raw pointer to a `shared_ptr` without ensuring ownership can lead to confusion and potential memory management issues.
Copying `shared_ptr` Incorrectly
While it may seem trivial, incorrectly copying a `shared_ptr` can lead to unintended behavior. Always ensure you understand sharing semantics; for instance, avoid copying a `shared_ptr` that should remain unique.
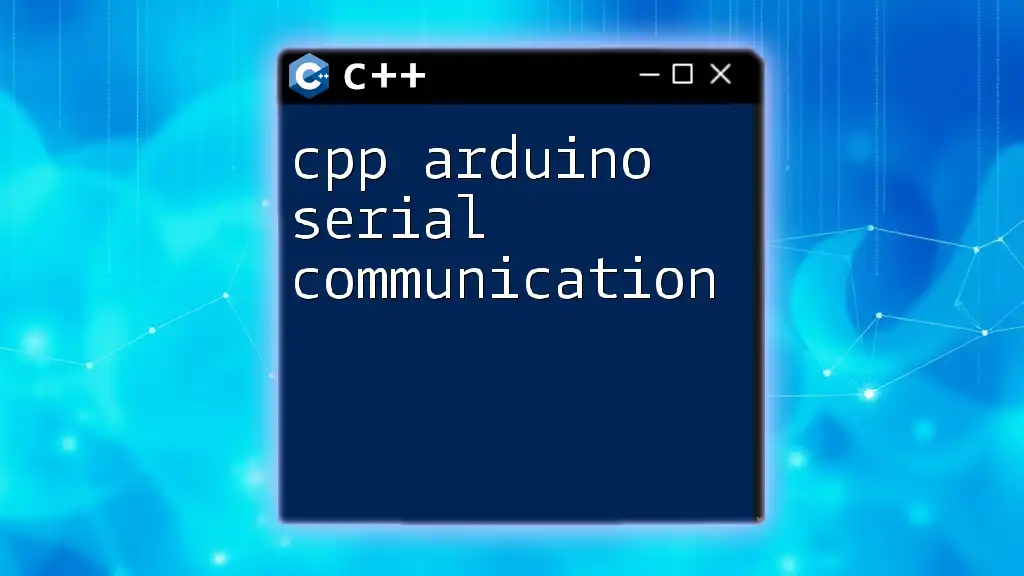
Advanced Features of `std::shared_ptr`
Using Custom Deleters
`shared_ptr` gives you the flexibility of using custom deleters. This is particularly useful when managing resources that require specific cleanup procedures. Here’s an example:
auto customDeleter = [](int* p) {
std::cout << "Deleting pointer\n";
delete p;
};
std::shared_ptr<int> ptr(new int(10), customDeleter);
This allows you to define a specific cleanup action when the last reference is destroyed.
Interoperability with Other Smart Pointers
`std::shared_ptr` can be converted to and managed alongside other smart pointers, providing additional flexibility in managing resources. For example, you might need to share a resource that is primarily owned by a `unique_ptr`.
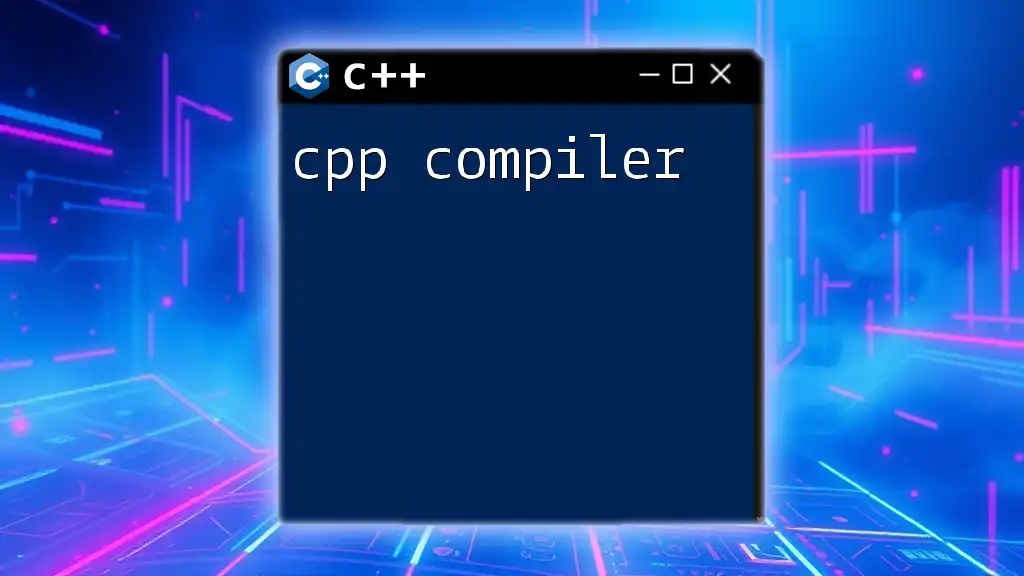
Conclusion
The introduction of smart pointers like `std::shared_ptr` has revolutionized memory management in C++. By promoting safer practices, preventing memory leaks, and offering automatic management capabilities, `shared_ptr` can greatly simplify C++ programming for both beginners and seasoned developers.
To truly master the nuances of `std::shared_ptr`, experimentation in a variety of scenarios is encouraged. Familiarizing yourself with these concepts can significantly improve both your coding efficiency and your applications' robustness in managing dynamic memory.
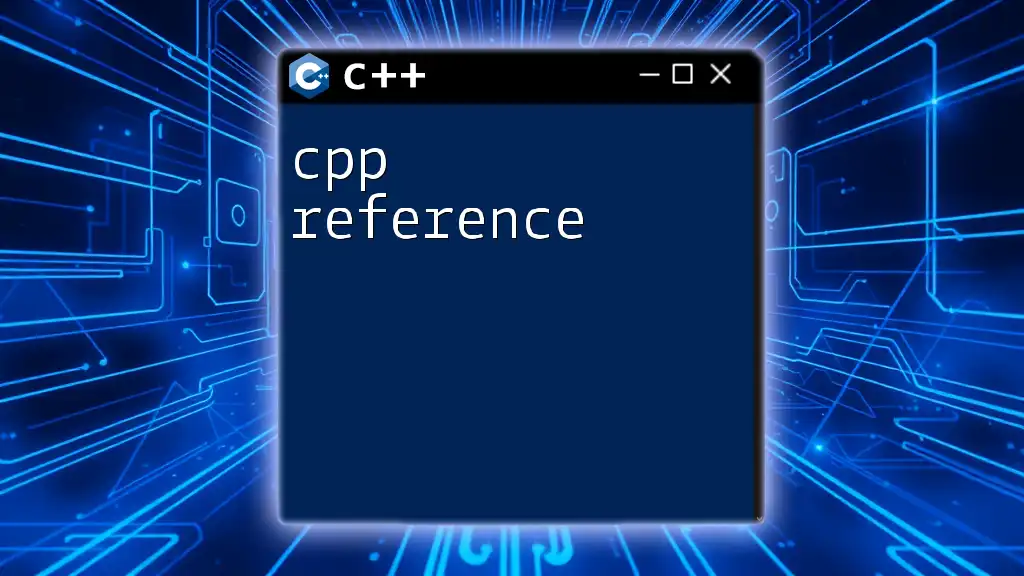
Additional Resources
For further learning, consider exploring the official C++ documentation, recommended literature on modern C++ practices, and engaging with online communities where developers share their experiences and solutions regarding memory management in C++.