Visual Studio Code provides a powerful and flexible environment for debugging C++ applications, allowing developers to set breakpoints, inspect variables, and control program execution seamlessly.
Here's a simple code snippet to demonstrate how to set up and use a breakpoint in a C++ program within Visual Studio Code:
#include <iostream>
int main() {
int a = 5;
int b = 10;
int sum = a + b; // Set a breakpoint here to inspect variables
std::cout << "Sum: " << sum << std::endl;
return 0;
}
Setting Up Visual Studio Code for C++ Debugging
Installing Visual Studio Code
To get started with debugging in C++, you'll first need to download and install Visual Studio Code. You can find the installer on the official [Visual Studio Code website](https://code.visualstudio.com/). Before installing, ensure that your system meets the necessary requirements to run VS Code effectively.
After downloading, follow these steps for installation:
- Run the installer and follow the prompts to complete the setup.
- Upon successful installation, launch Visual Studio Code.
Initially, it's crucial to configure your workspace for your C++ projects. This can be done by creating a dedicated folder for your C++ development.
Installing C++ Extensions
To enhance your development experience in Visual Studio Code, install the recommended extensions for C++. The two essential extensions you should include are:
- C/C++ (by Microsoft): This extension provides features such as IntelliSense, debugging support, and code browsing.
- CMake Tools: If you plan to use CMake for managing your project builds, this extension is invaluable.
How to Install Extensions
To install these extensions:
- Go to the Extensions view by clicking on the Extensions icon in the Activity Bar on the side of the window or pressing `Ctrl + Shift + X`.
- Search for "C/C++" and "CMake Tools" and click on the Install button for each.
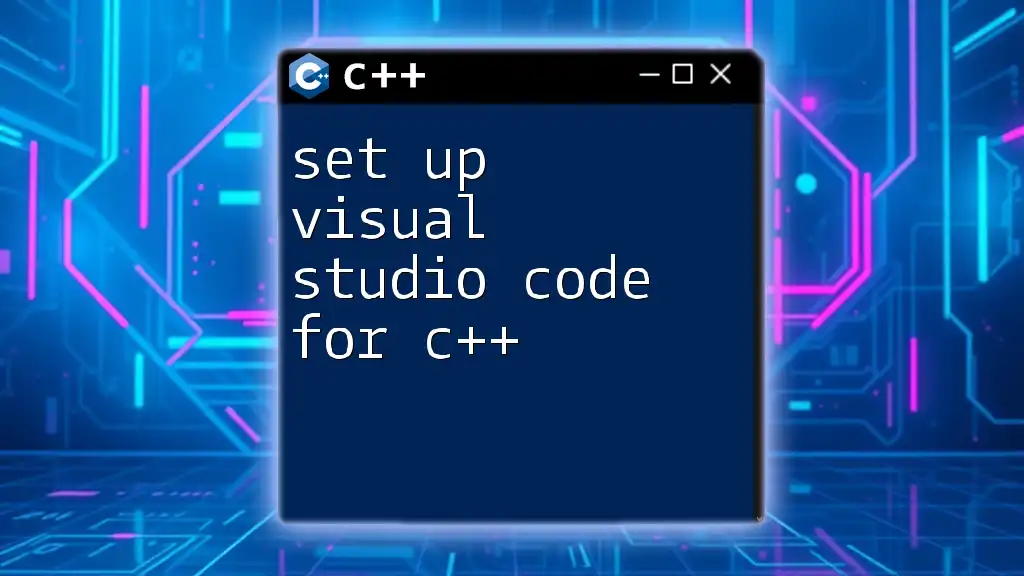
Configuring the C++ Environment for Debugging
Creating a C++ Project
Once your extensions are installed, it's time to create a new C++ project. Begin by creating a new folder where your project files will reside.
With your project folder ready, write your first simple C++ program to ensure everything is set up correctly. Here’s a basic example:
#include <iostream>
int main() {
std::cout << "Hello, Debugger!" << std::endl;
return 0;
}
Setting Up Build Tasks
To build your C++ application within Visual Studio Code, you'll need to create a `tasks.json` file where you can define your build tasks.
Creating a Tasks.json File
Within your project folder, press `Ctrl + Shift + P` to open the Command Palette and type "Tasks: Configure Default Build Task." Select it, and a `tasks.json` file will be generated.
Example Configuration for Building C++
Here is an example of what your `tasks.json` might look like:
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "shell",
"command": "g++",
"args": [
"-g", // Include debug information
"main.cpp",
"-o",
"main"
],
"group": {
"kind": "build",
"isDefault": true
}
}
]
}
In this configuration, we specify that the `g++` compiler should be used to compile `main.cpp`, and the `-g` flag adds debugging information to the executable.
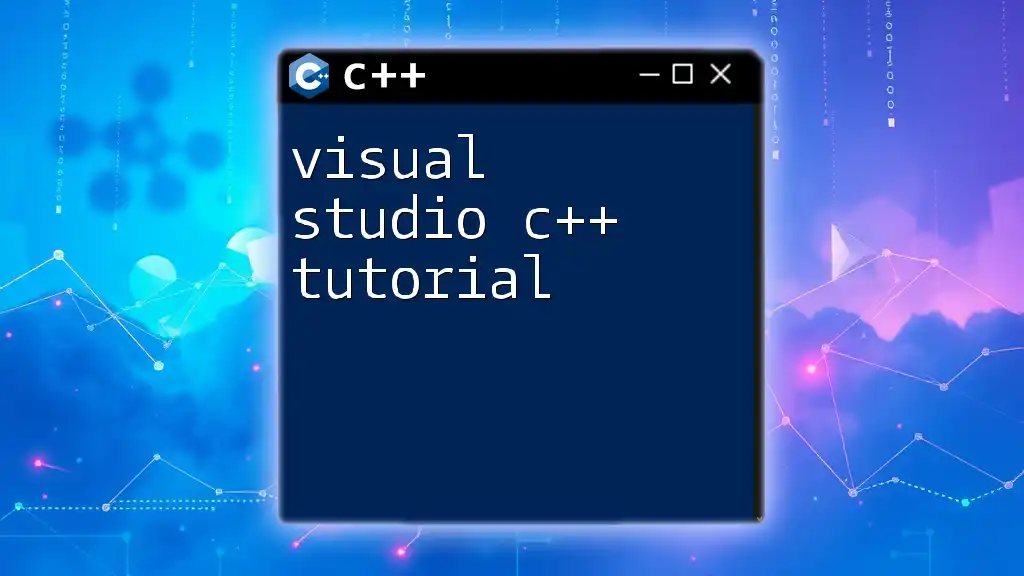
Launching the Debugger in Visual Studio Code
Setting Up Launch Configuration
In order to run the debugger, you need to create a `launch.json` file, which defines the configurations for the debugging session.
Creating the launch.json File
Follow these steps to create your `launch.json` file:
- Open the Command Palette with `Ctrl + Shift + P`.
- Type "Debug: Open launch.json" and select it. Choose `C++ (GDB/LLDB)` if prompted.
Example Configuration for Debugging C++
This is a basic example of what your `launch.json` could look like:
{
"version": "0.2.0",
"configurations": [
{
"name": "Debug C++",
"type": "cppdbg",
"request": "launch",
"program": "${workspaceFolder}/main",
"args": [],
"stopAtEntry": false,
"cwd": "${workspaceFolder}",
"environment": [],
"externalConsole": false,
"MIMode": "gdb",
"setupCommands": [
{
"description": "Enable pretty-printing for gdb",
"text": "-enable-pretty-printing",
"ignoreFailures": true
}
],
"preLaunchTask": "build",
"setupCommands": [
{
"text": "-enable-pretty-printing",
"description": "Enable gdb pretty printing"
}
]
}
]
}
This configuration tells Visual Studio Code how to execute your compiled program, where to find it, and whether to launch an external console for input/output.
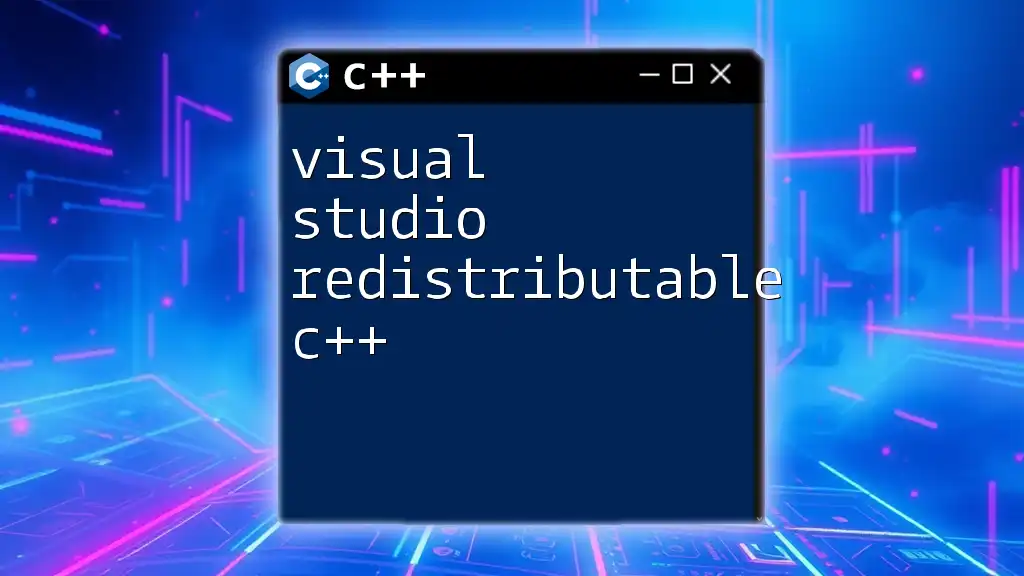
Using the Debugger Features
Starting the Debugging Session
With everything set up, you're ready to start debugging. You can launch the debugger by clicking on the green arrow in the Run and Debug view or simply press `F5`. This will start the session based on the configuration you've just set up.
Understand the Debug Console
The Debug Console is a vital part of the debugging experience. It allows you to evaluate expressions, execute commands, and view output during your debugging session. Familiarize yourself with this panel to enhance your debugging efficiency.
Setting Breakpoints
Breakpoints are essential debugging tools that let you pause the program execution at specified points, allowing you to inspect the application's state.
What are Breakpoints?
A breakpoint is a marker that you set on a line of code to indicate that execution should pause when the program reaches that line.
How to Set and Remove Breakpoints
In Visual Studio Code, you can set a breakpoint by clicking in the gutter (the area to the left of your line numbers) next to the line of code you’re interested in. A red dot will appear, indicating the breakpoint.
To remove a breakpoint, simply click on the red dot again.
Inspecting Variables
While debugging, understanding the state of your variables is crucial.
Using the Variables Pane
In the Debug view, you'll find the Variables pane. This pane displays all current variables and their values while your program is stopped at a breakpoint. You can hover over variables in your code to see their current values without looking away from the code.
Example: Inspecting Variables
Let’s return to our earlier program. If you set a breakpoint on the line just before you output `Hello, Debugger!`, you can examine the variables. Although the example is simple, in more complex programs, this can help you track down unexpected behavior.
Stepping Through Code
Effective debugging requires stepping through your code to see how it executes line by line.
Understanding Step Over, Step Into, and Step Out
- Step Over (F10): Executes the next line of code but does not step into functions.
- Step Into (F11): Moves the debugger into the function call, allowing inspection of its inner workings.
- Step Out (Shift + F11): Exits the current function and returns to the location in the code where it was called.
Practical Example of Stepping Through Code
If you have a function that calculates the factorial of a number and you wish to observe how it operates step-by-step, you'll place your breakpoints on the function call and each line within the function to see how values change.
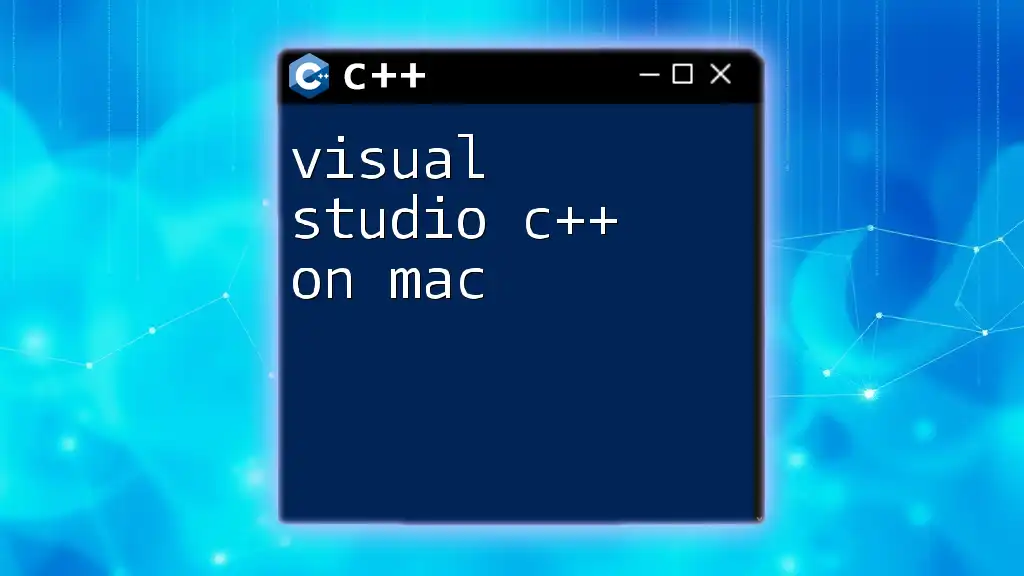
Advanced Debugging Techniques
Conditional Breakpoints
What are Conditional Breakpoints?
Conditional breakpoints are a powerful feature that allows you to halt execution only when specific conditions are met. This is especially useful when debugging loops or frequently called functions.
How to Set Conditional Breakpoints in VS Code
To set a conditional breakpoint, right-click the breakpoint dot in the gutter and select "Edit Breakpoint." A prompt will appear; enter your condition (e.g., `i == 5`), and the program will only stop when that condition is true.
Data Visualizations
Visualizing Data Structures
Visual Studio Code allows for the inspection and visualization of complex data structures, making it easier to understand their state during debugging.
Practical Example: Visualizing a Vector
Consider the following code snippet that utilizes a `std::vector`:
#include <vector>
#include <iostream>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
for (auto num : nums) {
std::cout << num << " ";
}
return 0;
}
If you set a breakpoint inside the loop, you can examine the contents of `nums` through the Variables pane, allowing you to see how it looks at each iteration.
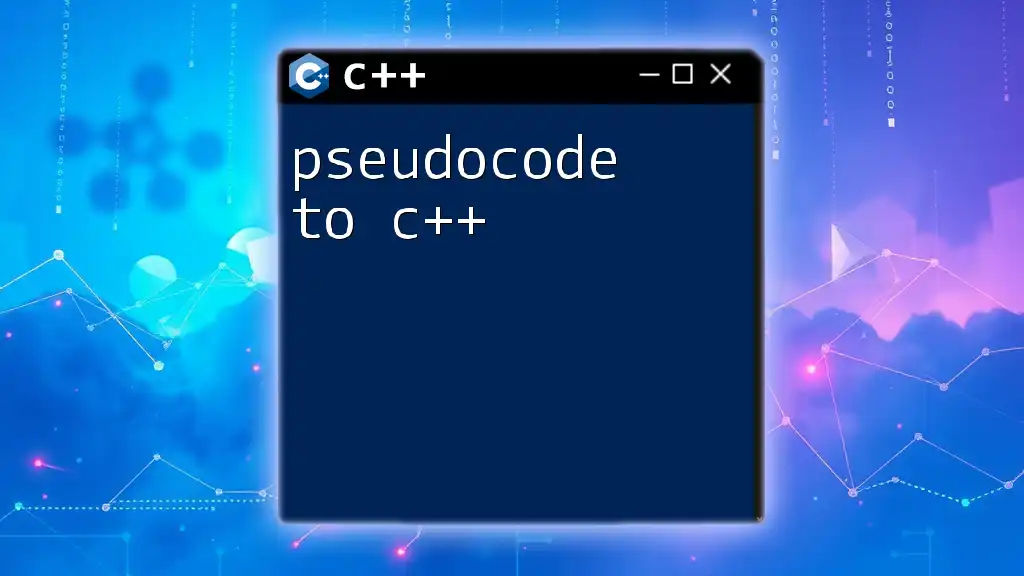
Common Debugging Issues and Solutions
Troubleshooting Debugging Problems
Despite the straightforward setup, you may encounter issues when debugging in Visual Studio Code:
- Debugging Not Starting: Verify that your `launch.json` configuration is correct, especially the path to the executable.
- Compiler or Linker Errors: Ensure your build task is correctly configured and that you have all required libraries linked.
Resource Links for Further Help
Should you face challenges that this guide doesn’t address, numerous online communities and resources can assist you, including Stack Overflow, the Visual Studio Code documentation, and various C++ programming forums.
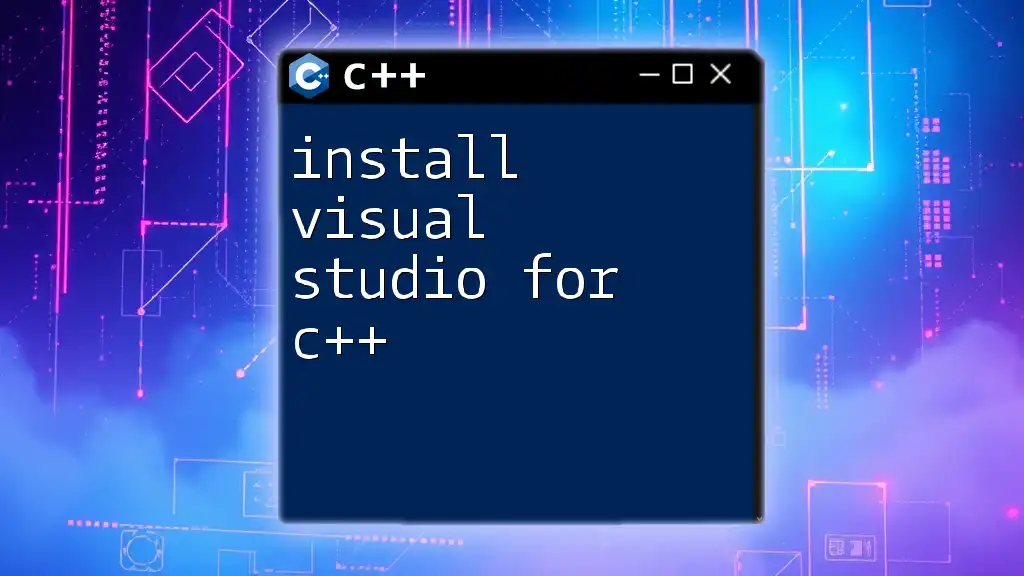
Conclusion
Debugging in Visual Studio Code can significantly streamline your C++ development process. By following the steps outlined in this article, you can efficiently set up your environment, employ debugging features, and troubleshoot issues with minimum hassle. Remember that practice is essential—take the time to experiment with the debugger to fully harness its capabilities and incorporate best practices into your debugging routine.
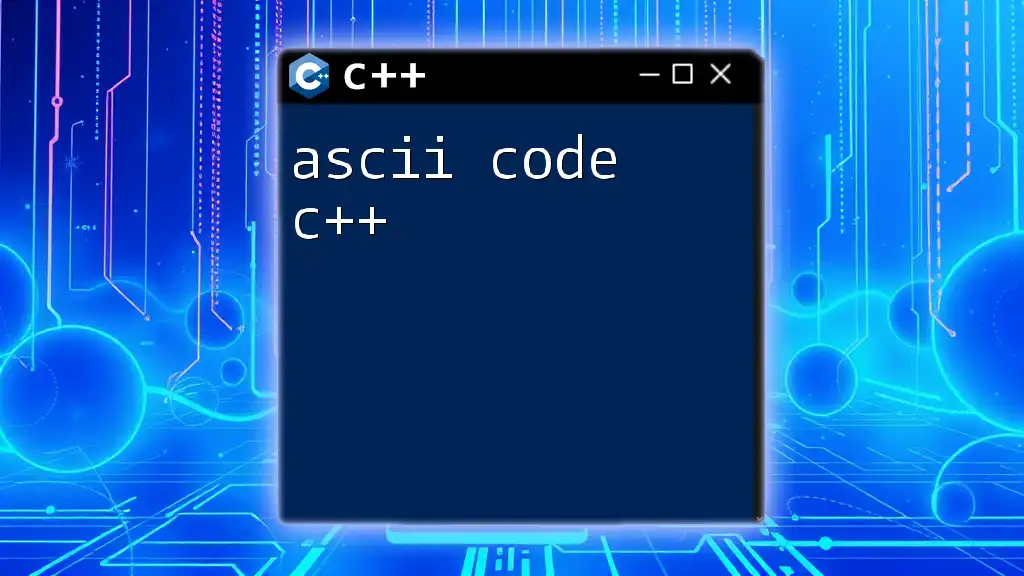
Call to Action
We encourage you to engage with us! Share your experiences, ask questions, or request additional topics of interest. Your feedback is invaluable as we strive to provide practical knowledge in working with C++.
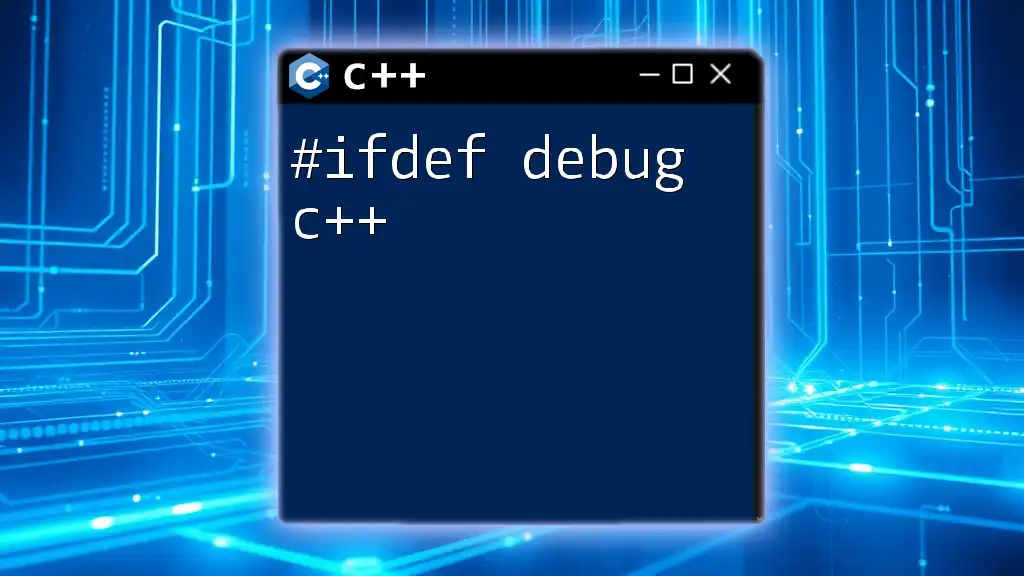
Additional Resources
To further enhance your skills and knowledge in C++, feel free to explore online communities, forums, and additional reading material, which can serve as great supplements to this guide.