Visual Studio does not natively support C++ development on macOS, but you can use Visual Studio for Mac or other alternatives like Xcode or command-line tools to write and compile C++ code.
Here's a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with Visual Studio C++ on Mac
What is Visual Studio for Mac?
Visual Studio for Mac is a powerful integrated development environment (IDE) that allows developers to build, debug, and deploy applications, specifically tailored for macOS. Though primarily known for its capabilities in C#, Visual Studio for Mac also supports C++ development through its project templates and integrated tools. Unlike its Windows counterpart, Visual Studio for Mac provides a streamlined interface and tools that conform to the conventions of macOS, catering to the unique workflow of Mac users.
System Requirements
Before you dive into Visual Studio C++ on Mac, you need to ensure that your machine meets the necessary hardware and software requirements. Here’s what to check:
- macOS Version: You need to have macOS 10.12 (Sierra) or later installed.
- RAM: A minimum of 4GB of RAM is recommended, though 8GB is ideal for larger projects.
- Disk Space: At least 2GB of free disk space is required for installation.
- Processor: Any Intel-based Mac or a newer Apple Silicon Mac is supported.
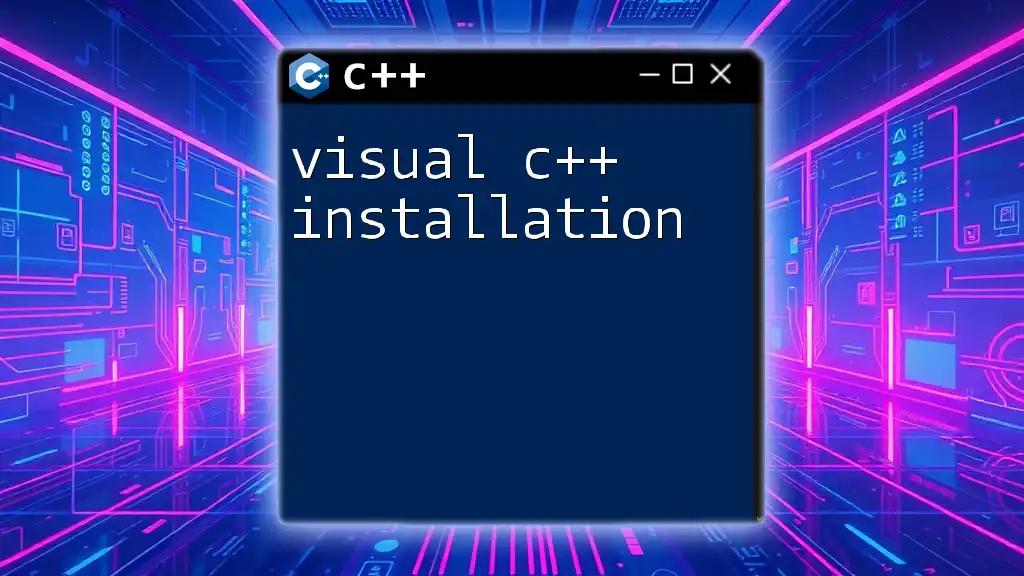
Installing Visual Studio for C++
Step-by-Step Installation Process
To begin your journey with Visual Studio C++ on Mac, follow these steps to install the IDE:
Download Visual Studio for Mac
Visit the official [Visual Studio for Mac download page](https://visualstudio.microsoft.com/vs/mac/) and download the latest version.
Install the Application
Open the downloaded .dmg file and drag the Visual Studio app into your Applications folder. Launch Visual Studio from your Applications.
Selecting C++ Workloads
Upon first launch, you’ll be guided through a setup wizard. Choose the appropriate workloads and make sure to select the C++ workload. This ensures that you have all necessary components to start writing C++ applications.
Configuration After Installation
Once Visual Studio for Mac is installed, it’s time to create your first C++ project.
Creating a First C++ Project
Open Visual Studio and select File > New Solution. Navigate to the C++ section and choose the Console Application template. Enter a project name, select a location, and click Create.
Here's a simple code snippet to get started with a Hello World application:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Just copy this into your main program file and run your project.
Overview of the Visual Studio Interface
The interface of Visual Studio for Mac is intuitive and user-friendly. Key components to familiarize yourself with include:
- Solution Explorer: This panel shows your projects and files, allowing you to manage your project structure easily.
- Code Editor: The heart of your coding environment, where you will write and edit your C++ code.
- Output Window: Displays build activities, errors, and diagnostics output to help manage your workflow.
Customizing the Environment
You can tailor your Visual Studio environment according to your preferences by navigating to Preferences under the Visual Studio menu. Here, you’ll find options for adjusting themes, fonts, and other IDE settings.
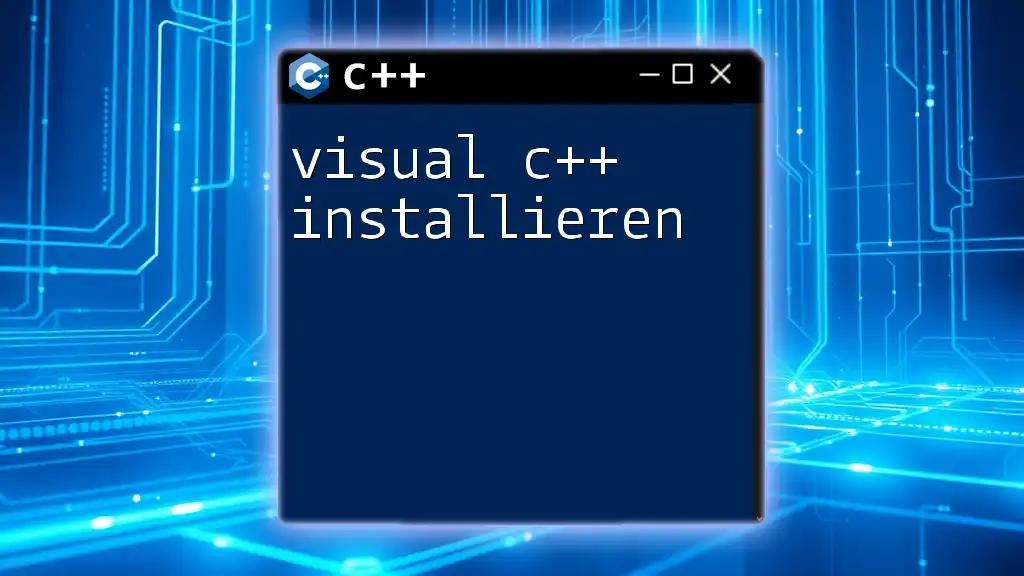
Developing C++ Applications
Writing Your First C++ Program
Once you're set up, it’s time to write a real application. The Hello World program from above is a great way to start, but as you progress, you may want to incorporate user input or even create a small game.
Utilizing the Built-in Debugger
One of the strongest features of Visual Studio C++ on Mac is the integrated debugger. Debugging is essential for identifying and correcting issues in your code.
To make use of it, you can set breakpoints in your code by clicking in the margin next to a line number. When you start your application in debugging mode, execution will pause at your breakpoints, allowing you to inspect variables and control execution flow.
Example of Debugging
Consider the following program that requests user input:
#include <iostream>
#include <string>
int main() {
std::string name;
std::cout << "Enter your name: ";
std::getline(std::cin, name);
std::cout << "Hello, " << name << "!" << std::endl;
return 0;
}
You can set a breakpoint at `std::getline(std::cin, name);` to inspect the `name` variable before it is used.
Using Libraries and Dependencies
As your project evolves, you may want to include external libraries to extend functionality. Visual Studio for Mac allows for easy integration of libraries.
Code Snippet: Including a Library
Here’s how to link an external library:
- Add the Library: Go to your project’s settings by right-clicking on your project in the Solution Explorer.
- Navigate to Build > Compiler, and in the Additional Arguments, input `-l<library_name>` (for example, `-lmylibrary`).
- Example of C++ code using a library:
#include <mylibrary.h> // Assuming mylibrary.h is your library
int main() {
myFunction(); // A function defined in mylibrary
return 0;
}
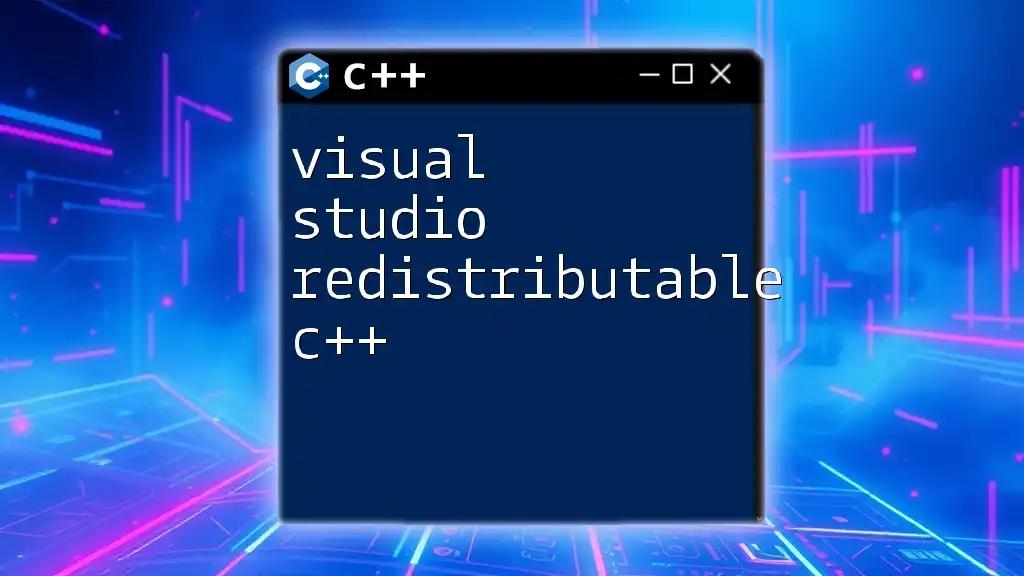
Advanced Features and Tools
Intellisense in Visual Studio C++
Intellisense is a powerful tool that helps you write code faster by providing suggestions, auto-completion, and parameter info as you type. For instance, if you start typing `std::cout`, Intellisense will automatically suggest completing it, along with a list of relevant functions and types.
Version Control Integration
Integrating Git into Visual Studio for Mac allows you to manage your project’s version control seamlessly. To initialize a Git repository:
- Open the Terminal or use the integrated Git tools in Visual Studio.
- Navigate to your project directory and run `git init` to create a new repository.
- After making changes to your code, you can commit these with `git commit -m "Your message here"`.
Unit Testing in Visual Studio C++
Unit testing is a vital aspect of software development that ensures code quality. Visual Studio for Mac supports creating unit tests easily.
Example: Creating a Simple Test Case
You can create a unit test within the same solution:
#include <gtest/gtest.h>
// Function to be tested
int add(int a, int b) {
return a + b;
}
// Test case
TEST(MathTest, Add) {
EXPECT_EQ(add(1, 2), 3);
}
To run the tests, build your solution and run the test explorer.
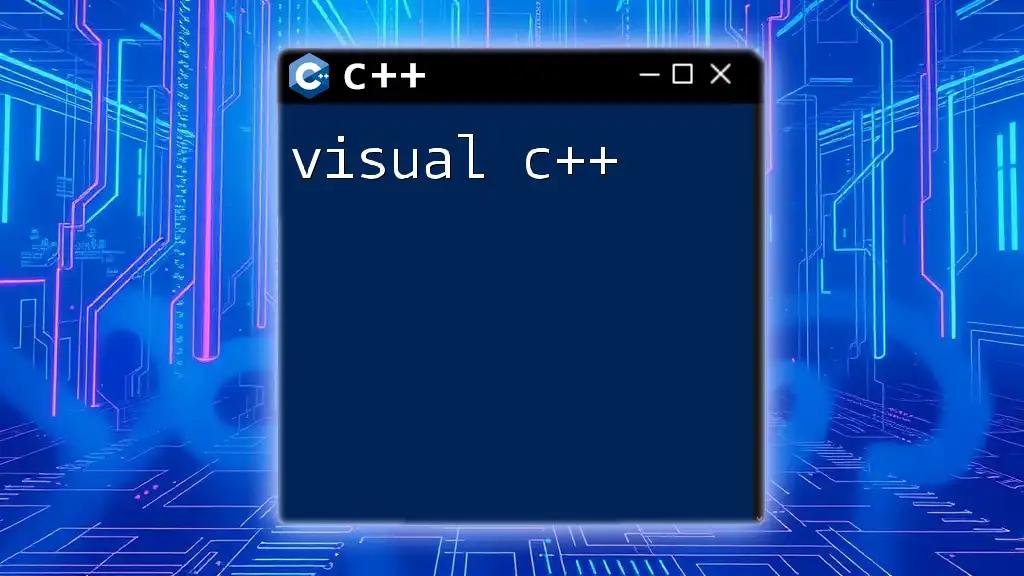
Best Practices for C++ Development on Mac
Code Organization and Structure
Understanding how to best organize your code is crucial for scalability and maintainability. Group related functions and classes into separate files. Adopt the use of namespaces to avoid naming collisions and improve code readability.
Cross-Platform Development Considerations
When developing applications in Visual Studio C++ on Mac, keep in mind that your code should be portable. To ensure this:
- Avoid using platform-specific APIs as much as possible.
- Use tools like CMake to handle different build environments.
Performance Optimization Tips
For optimal performance of your C++ applications:
- Use efficient algorithms and data structures.
- Profile your code to identify bottlenecks and optimize them. Tools are available within Visual Studio for this purpose.
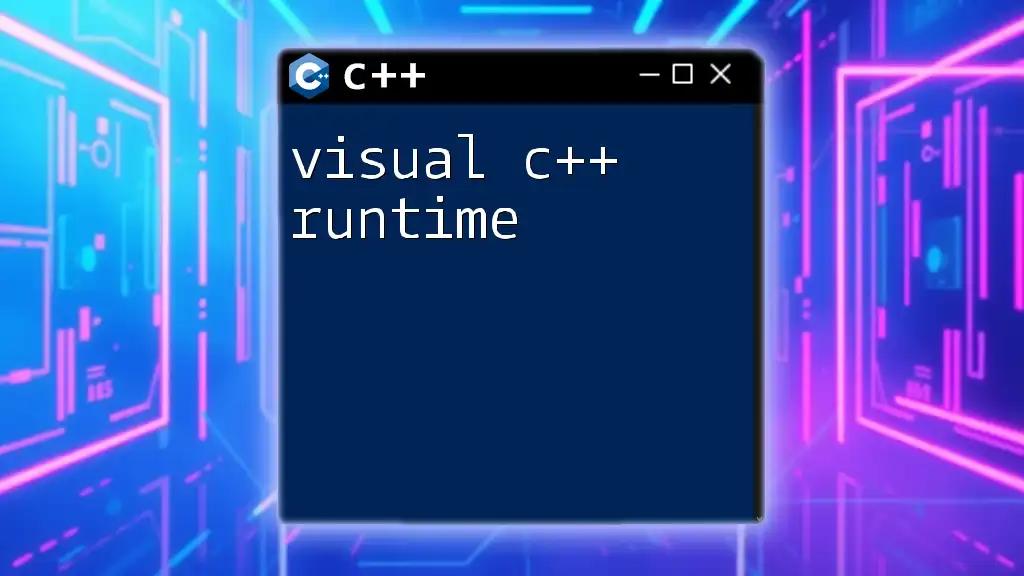
Resources for Further Learning
Recommended Online Courses and Tutorials
To deepen your understanding and skills, consider enrolling in online courses that focus on C++ programming specifically tailored for Visual Studio on Mac. Websites like Udemy, Coursera, or Codecademy offer courses to help you progress rapidly.
Community Forums and Support
Connect with fellow developers in forums such as Stack Overflow and the Visual Studio community. These platforms provide an excellent space for getting assistance, sharing knowledge, and discussing best practices.
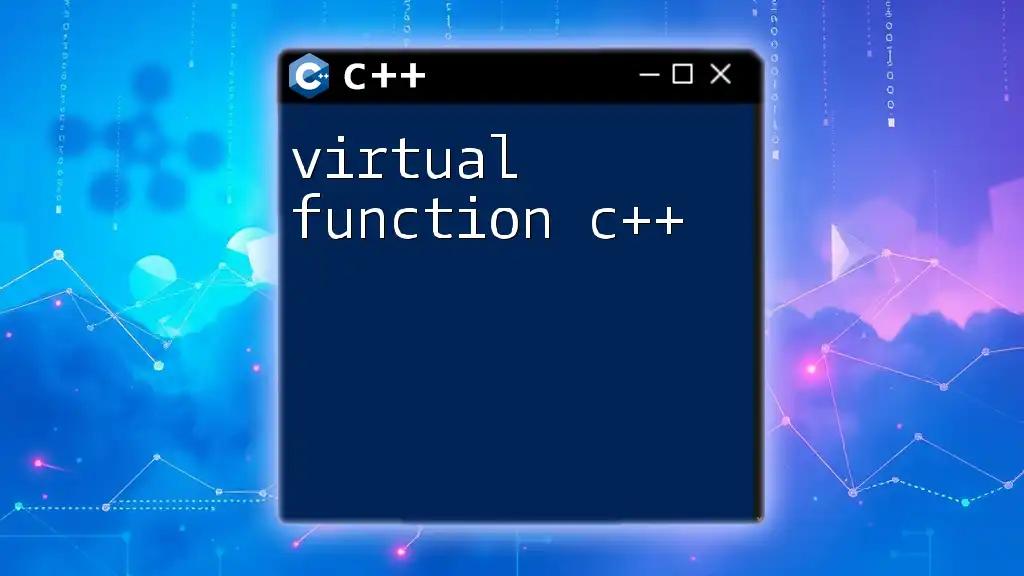
Conclusion
In conclusion, Visual Studio C++ on Mac offers a robust development environment conducive to building high-quality applications. From installation to debugging and optimization, this IDE provides tools that enhance your programming experience. By exploring the features and capabilities outlined here, you can become proficient in C++ development, making your projects not only efficient but also enjoyable to create.
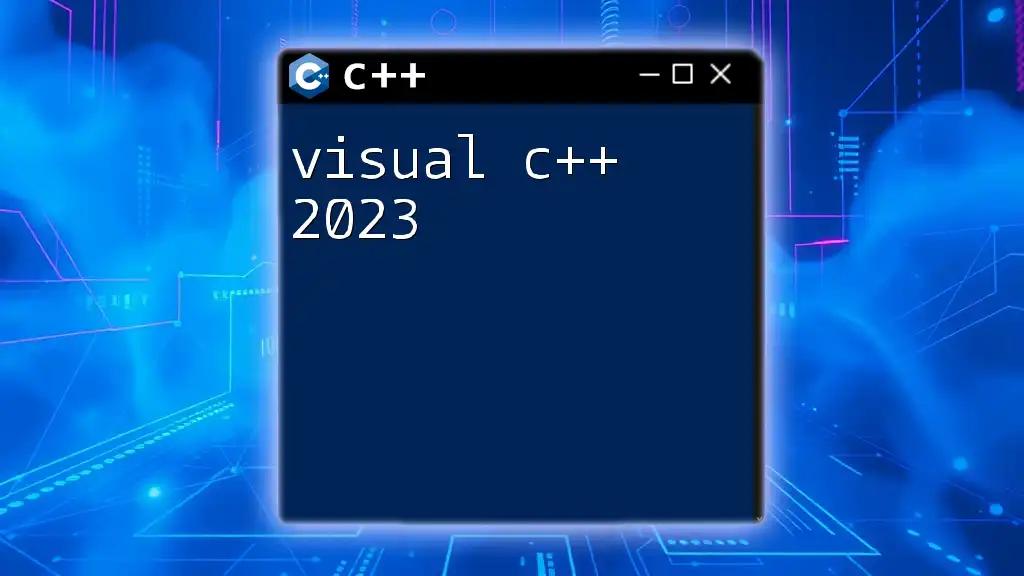
Call to Action
We encourage you to share your experiences with Visual Studio C++ on Mac in the comments below. If you're looking for a structured approach to learning C++, join our training programs for further enrichment and practical knowledge. Stay tuned for more insightful posts and tips!