To use GDB to debug a C++ program, you can compile your C++ code with debugging information and then run GDB to set breakpoints and inspect the program's state during execution.
Here’s how you can do it:
-
Compile your C++ code with the `-g` option:
g++ -g your_program.cpp -o your_program
-
Start GDB with your program:
gdb ./your_program
-
Set a breakpoint at the `main` function:
break main
-
Run your program:
run
-
Inspect variables or step through your code:
print variable_name step
Here's a simple example of C++ code to debug:
#include <iostream>
using namespace std;
int main() {
int a = 5;
int b = 10;
cout << "Sum: " << a + b << endl;
return 0;
}
In GDB, you can follow the steps above to set breakpoints and inspect variables like `a` and `b`.
What is GDB?
GDB, short for GNU Debugger, is a powerful debugging tool designed to help developers analyze and troubleshoot their C++ programs. Its ability to halt program execution and inspect the current state allows developers to identify issues that might lead to bugs or crashes. Understanding the fundamentals of GDB is critical for effective C++ programming, as it provides insights into your code's execution flow and variable states.
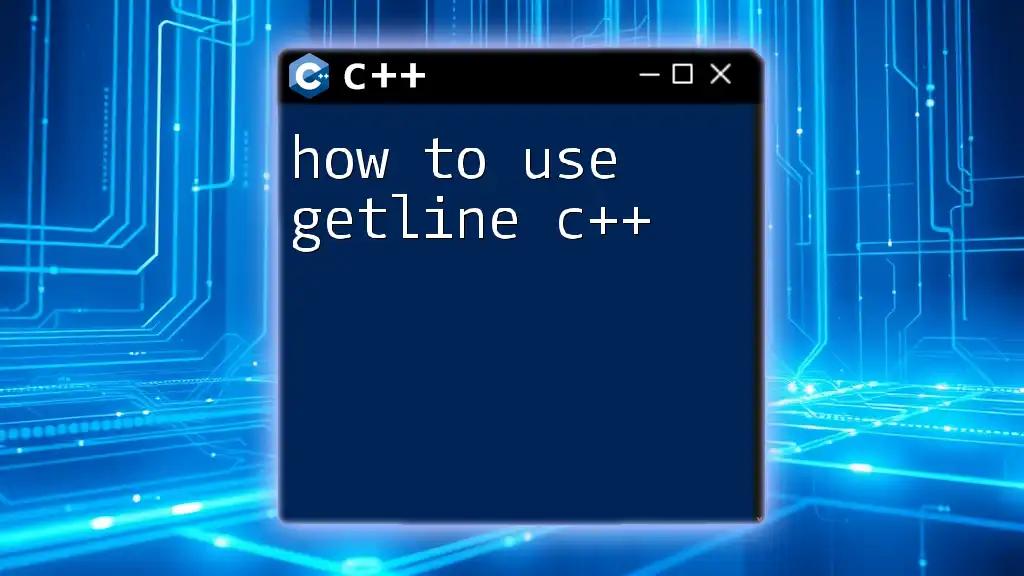
Getting Started with GDB
Installing GDB
Installation of GDB varies across operating systems. Here are a few common methods:
-
Linux: You can easily install GDB using your package manager. For example, on Ubuntu, use:
sudo apt install gdb
-
Mac: If you use Homebrew, you can install it with:
brew install gdb
-
Windows: You can install GDB as part of MinGW or Cygwin. Alternatively, you can use the Visual Studio environment which has built-in support for debugging.
Compiling C++ Code for Debugging
Before debugging, it's essential to compile your C++ code with debugging symbols. This is done using the `-g` flag when invoking the compiler. Debugging symbols provide GDB with the necessary information about the variables, functions, and structures within your code.
Here's how you compile your program for debugging:
g++ -g -o my_program my_program.cpp
By including the `-g` flag, you're ensuring that GDB has the detailed enough information to make debugging more straightforward.
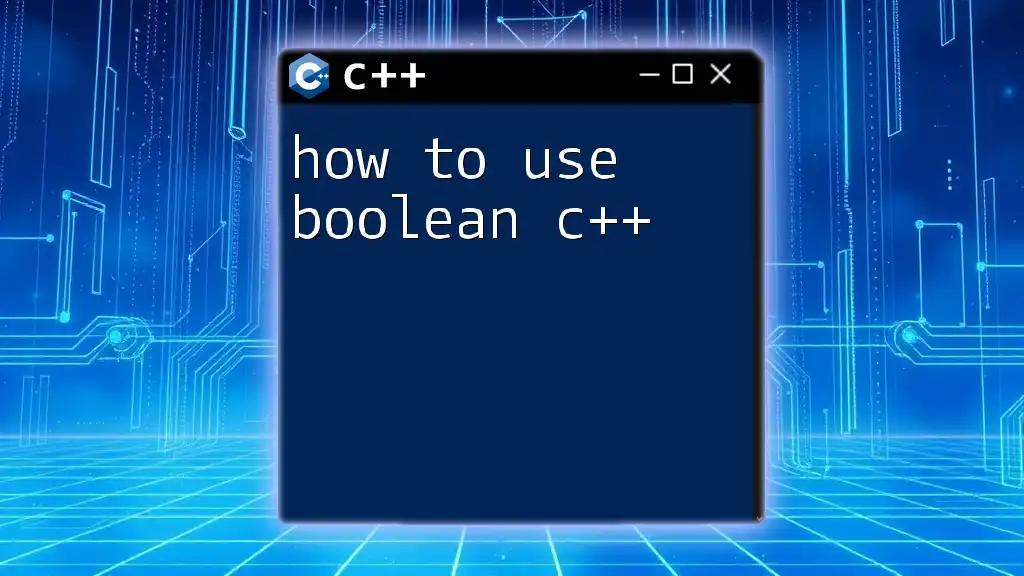
Basic GDB Commands
Launching GDB
To start using GDB, launch it from the command line with the program's executable as an argument. For instance, if your executable is named `my_program`, you would write:
gdb my_program
This opens GDB with your specified program ready to be debugged.
Running the Program
Once inside GDB, you can start your program using the `run` command. This is how you invoke that command:
(gdb) run
Executing this command begins your program and allows you to interact with it as it runs.
Stopping a Program
If you need to stop execution before it reaches the end, you can simply interrupt it by pressing Ctrl + C. This command sends a signal to GDB to halt program execution, allowing you to investigate the program’s current state.
Examining Code and Variables
Displaying Source Code
To visualize your code while debugging, use the `list` command. This tells GDB to show you the relevant portion of your source code:
(gdb) list
This will display lines of the source code around the current execution point, helping you understand what part of the code is running.
Printing Variables
When you want to inspect the values stored in variables, you can utilize the `print` command. For example, if you have a variable called `my_variable`, you can check its value with:
(gdb) print my_variable
This displays the value of `my_variable` in the GDB console, providing instant feedback on how your code executes.
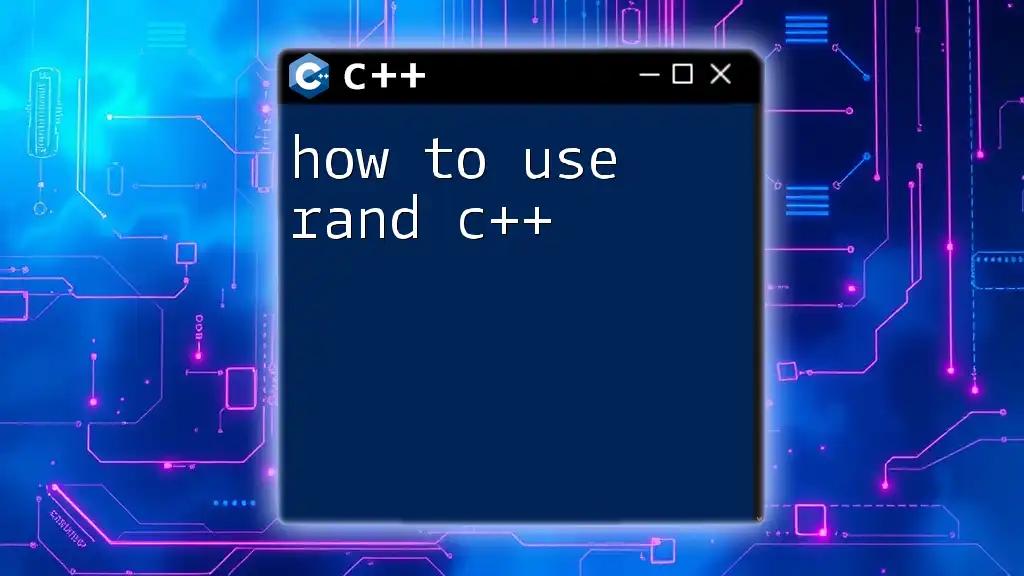
Setting Breakpoints
What is a Breakpoint?
A breakpoint allows you to pause program execution at a specific point in your code. This lets you inspect the current state of the program, including variable values and control flow. Breakpoints are crucial when trying to diagnose where a bug might occur.
Setting and Clearing Breakpoints
To set a breakpoint at a particular function or line, use the `break` command. If you want to set a breakpoint at the `main` function, the command would look like this:
(gdb) break main
If you decide to remove a breakpoint, you can use the `clear` command:
(gdb) clear main
This keeps your debugging session flexible and allows focused analysis only where needed.
Conditional Breakpoints
You can also set conditional breakpoints that trigger only when certain conditions are met. For instance, if you want to break inside `my_function` only when `my_variable` exceeds 10, you can set it this way:
(gdb) break my_function if my_variable > 10
This feature is invaluable for debugging scenarios where you want to avoid unnecessary stops when conditions do not match.
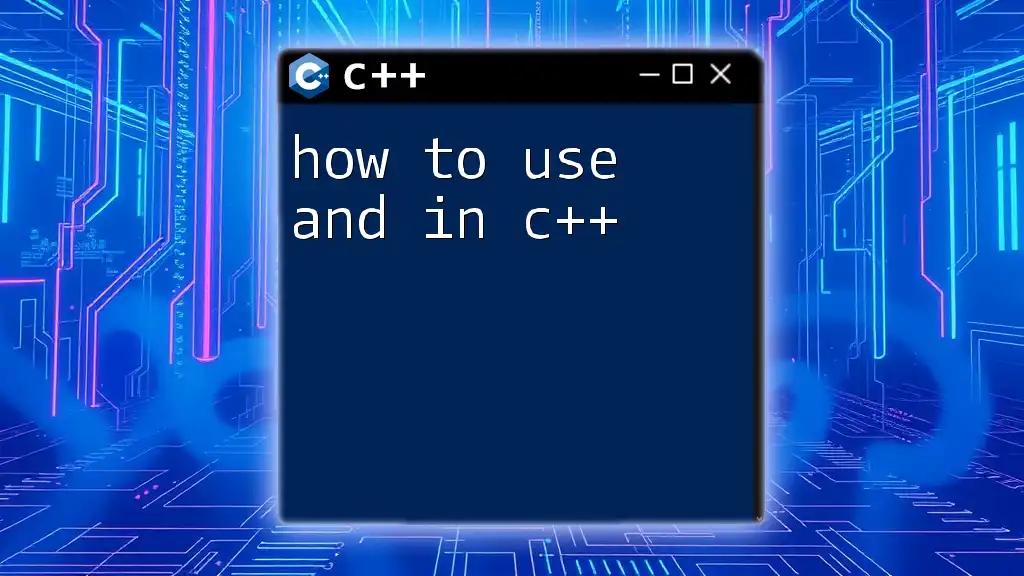
Navigating Through Code
Stepping Through Code
One of the most important aspects of debugging is navigating through your code. GDB provides different commands to allow you to step through your code line-by-line or function-by-function.
Next vs. Step Commands
-
The `next` command will step over function calls, executing them but not diving inside:
(gdb) next
-
In contrast, the `step` command will execute your program line by line, stepping into function calls if they are present:
(gdb) step
Continuing Execution
Once you have paused your program at a breakpoint, you can resume execution using the `continue` command. This tells GDB to proceed until the next breakpoint or until the program terminates:
(gdb) continue
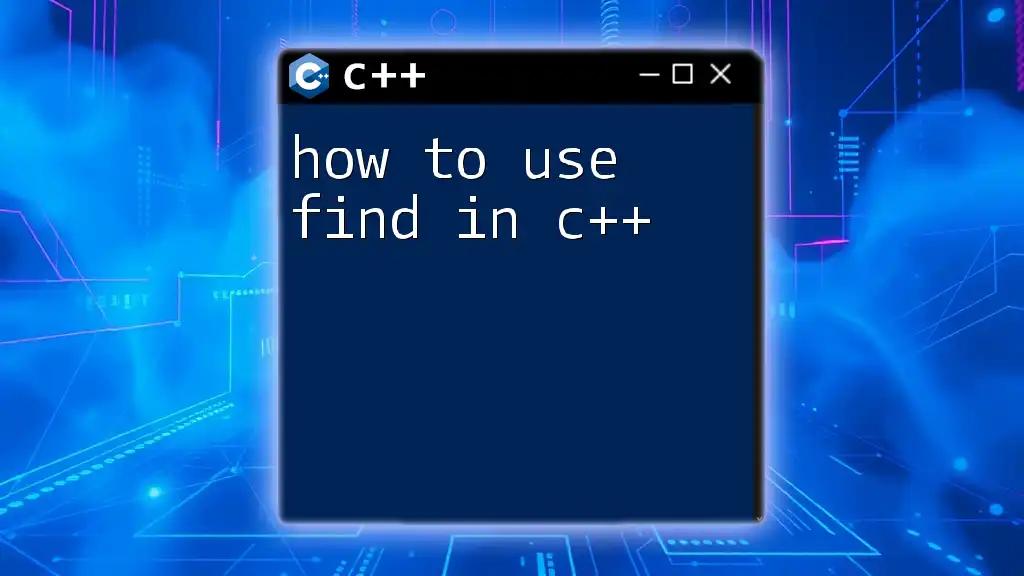
Inspecting Memory
Viewing Memory Contents
GDB allows you to inspect raw memory using the `x` command. This is useful for checking memory layout, especially for arrays and structures. For example, to examine an array of integers named `my_array`, you might write:
(gdb) x/10xw &my_array
Here, `10xw` indicates that you want to view 10 words in hexadecimal format, starting from the memory address of `my_array`.
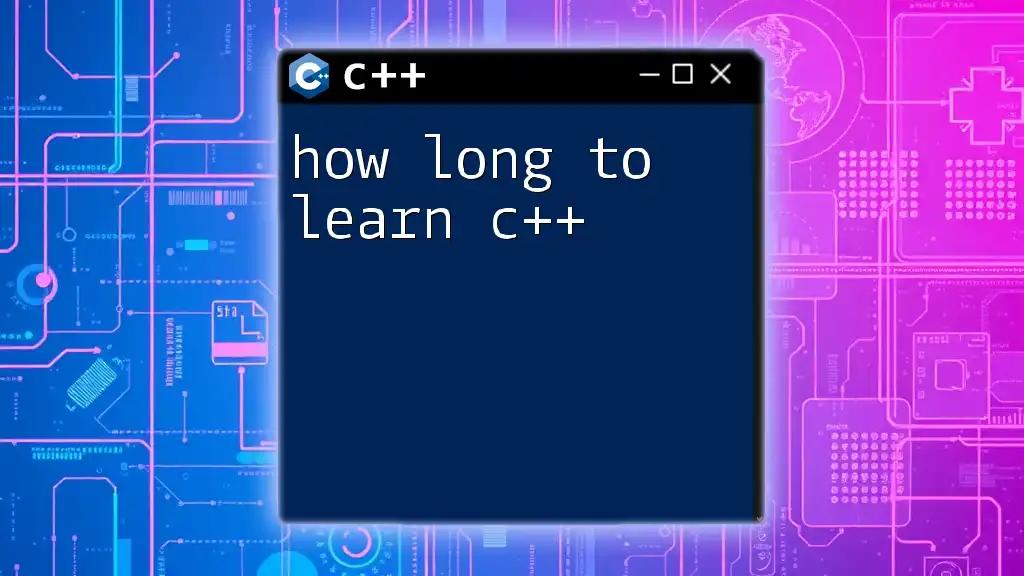
Using Backtrace for Call Stack
Understanding Call Stack
The call stack in programming keeps track of the sequence of function calls that led to the current state of your application. When your program crashes or hits a breakpoint, the call stack reveals this sequence.
To view the call stack in GDB, utilize the `backtrace` command:
(gdb) backtrace
This will provide a stack trace output that shows you the functions currently active in memory, along with their respective line numbers in your source code.

Common Debugging Scenarios
Memory Issues
Debugging memory issues like leaks and segmentation faults is crucial when working with C++ due to its manual memory management. GDB helps diagnose these problems effectively. For example, after a segmentation fault occurs, you can analyze it using:
(gdb) run
(gdb) backtrace
This sequence allows you to see which function caused the fault and aids in identifying logic errors in your code.
Infinite Loops
If your program appears to be stuck in an infinite loop, you can use Ctrl + C to interrupt the execution. Then, you can check where it is stuck by using the `backtrace` command to identify the current function and the last executed statement.
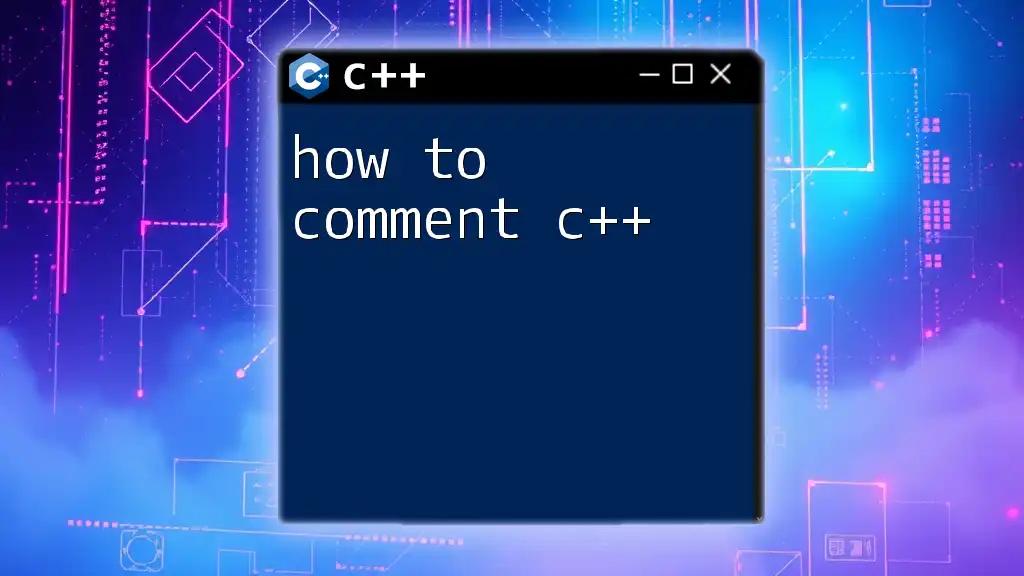
Advanced GDB Features
Scripting and Automation
GDB supports scripting, allowing you to automate your debugging tasks. You can write scripts in GDB commands or automate processes using Python scripts in GDB, which can significantly speed up your debugging workflow.
GDB with Other Tools
GDB integrates well with several other tools and IDEs, enhancing the debugging process. For instance, both Visual Studio Code and Eclipse support debugging through GDB, making it easier for developers who prefer working within a full-fledged development environment.
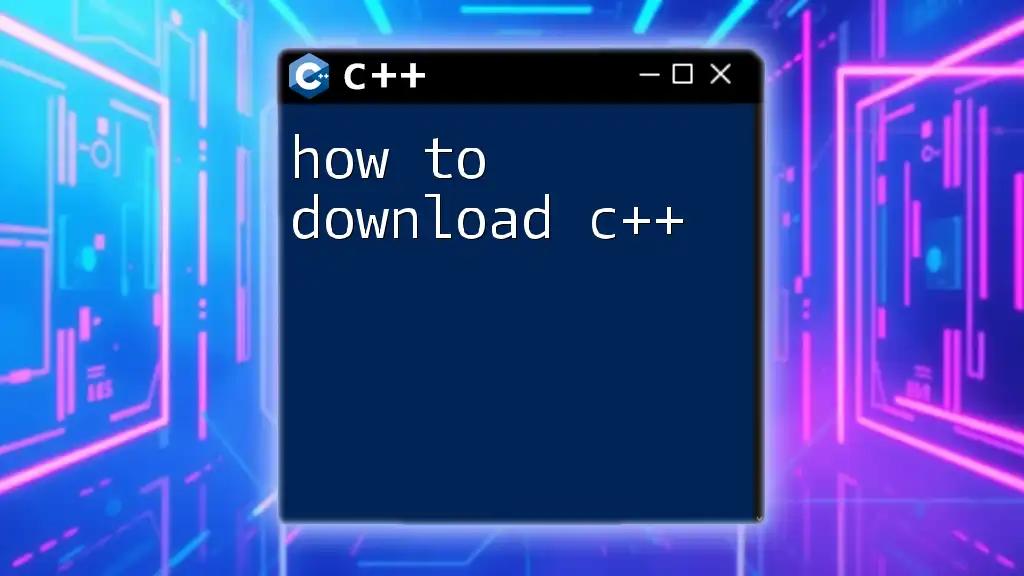
Best Practices for Debugging with GDB
To get the most out of GDB, consider the following best practices:
- Regularly use version control to help in easily identifying when bugs were introduced.
- Practice setting breakpoints and stepping through code to familiarize yourself with the GDB interface and commands.
- Keep your code clean and organized to prevent confusion while debugging.
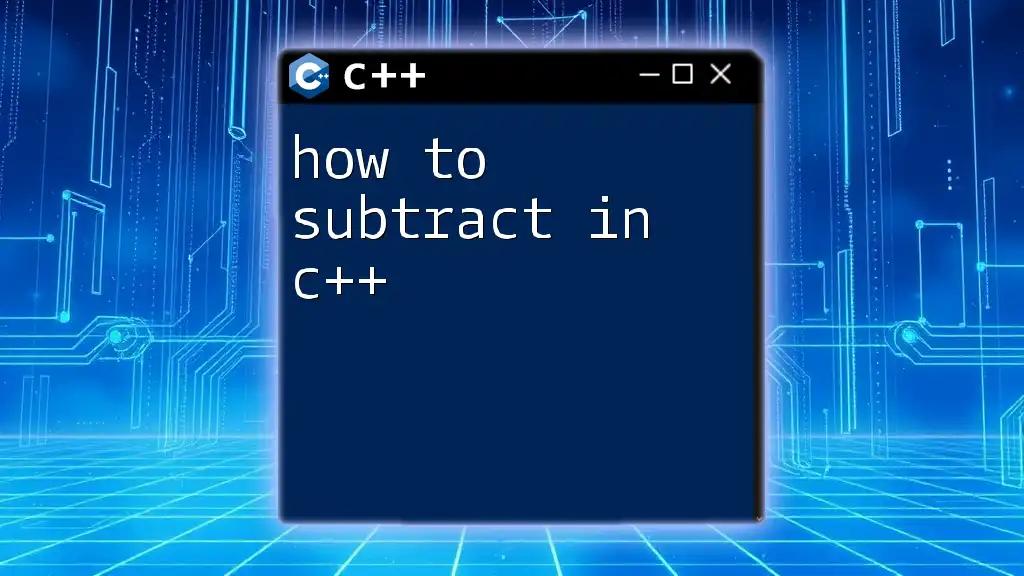
Conclusion
In summary, knowing how to use GDB to debug C++ is an invaluable skill for any developer. Mastering its commands, features, and best practices not only enhances your debugging capabilities but also improves your overall coding proficiency. Embrace the power of GDB to transform your debugging experience and elevate your coding skills.

Further Resources
For additional learning, consider the following:
- Official GDB documentation for detailed command usage and features.
- Recommended books on advanced C++ programming and debugging techniques.
- Online forums and communities where developers share tips, ask questions, and discuss their debugging challenges.