The C++ compiler in Visual Studio allows developers to write, compile, and debug C++ code efficiently, leveraging the powerful features of the IDE to streamline the development process.
Here's a simple code snippet demonstrating the "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Visual Studio?
Overview of Visual Studio
Visual Studio is a comprehensive Integrated Development Environment (IDE) developed by Microsoft that supports various programming languages, including C++. It provides developers with a flexible workspace, sophisticated tools for coding, debugging, and compiling applications. With its rich feature set and user-friendly interface, Visual Studio stands out as a preferred choice for C++ developers.
Visual Studio Editions
Visual Studio is available in several editions, each tailored to different needs:
- Community Edition: Ideal for individual developers and small teams. It offers a robust feature set without any associated costs.
- Professional Edition: This edition targets professional developers and teams needing advanced collaboration features and support.
- Enterprise Edition: Designed for large organizations, it includes additional tools for monitoring application performance and advanced testing solutions.
Understanding the differences helps you choose the best version for your needs, ensuring you have the right tools to succeed in C++ development.
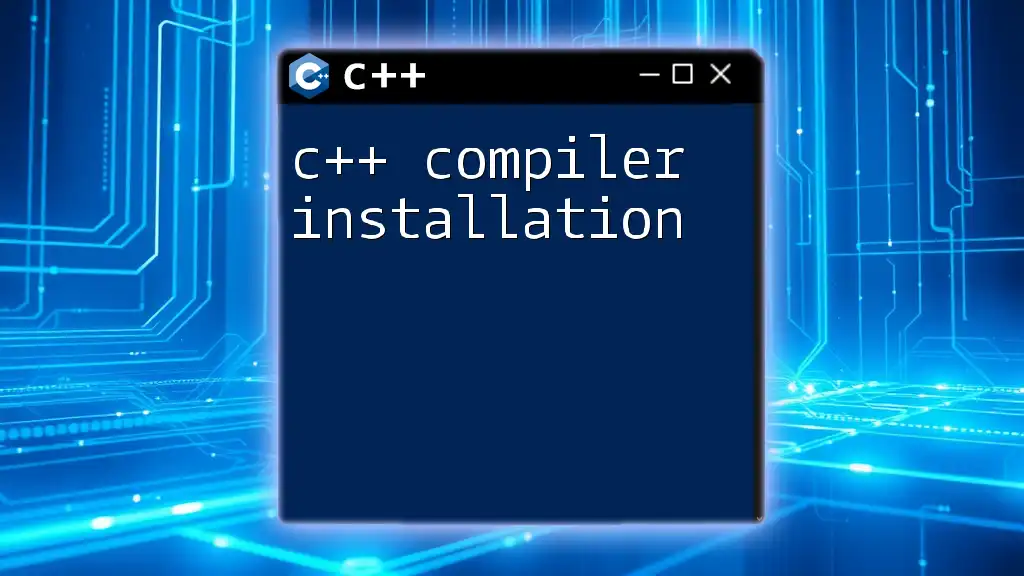
Getting Started with Visual Studio
Installing Visual Studio
To begin your journey with Visual Studio, you need to install it first. Here’s how to do that:
- Download the installer from the official Visual Studio website.
- Run the installer and select the Desktop development with C++ workload. This option ensures that all necessary components for C++ development are included.
- Follow the prompts to finalize the installation. Make sure to choose the versions of tools and features that match your development needs.
Setting Up C++ Development Environment
During installation, Visual Studio will present different components. Make sure you select:
- MSVC (Microsoft Visual C++): The compiler for building C++ applications.
- Windows SDK: For building Windows applications.
- C++ profiling tools: For checking and improving code performance.
This configuration sets up a robust environment tailored for C++ programming.
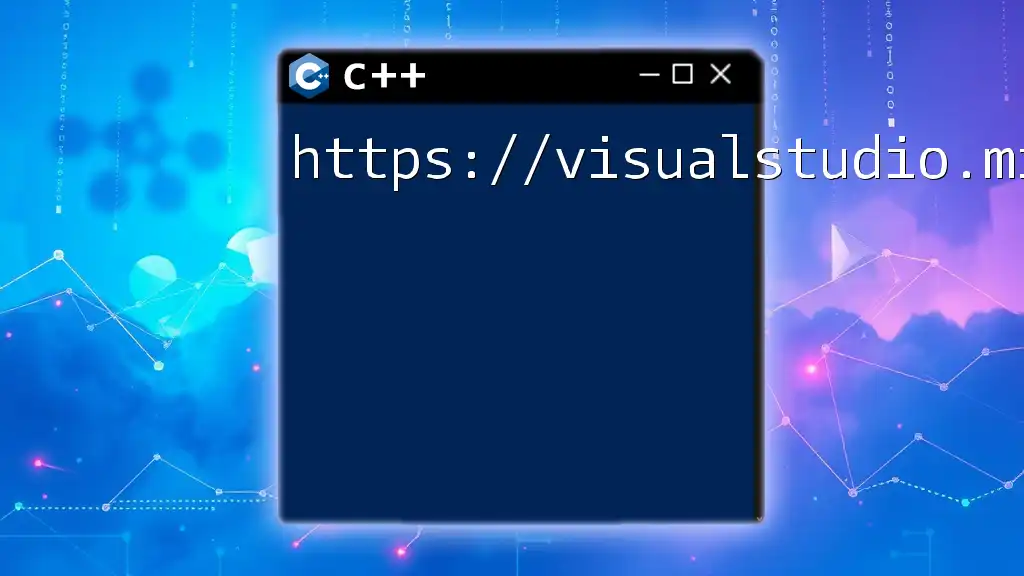
Creating Your First C++ Project
Starting a New Project
Once Visual Studio is installed, opening it up and starting your first project is straightforward:
- Launch Visual Studio.
- Choose Create a new project.
- In the project template selection, search for Console App under C++.
- Name your project, set its location, and click Create.
Understanding the Project Structure
When you create a new project, Visual Studio sets up various directories. Key components include:
- Source Files: This folder contains your main C++ source files.
- Header Files: For declarations and definitions used across multiple files.
The structure helps keep your code organized and manageable as you expand your project.
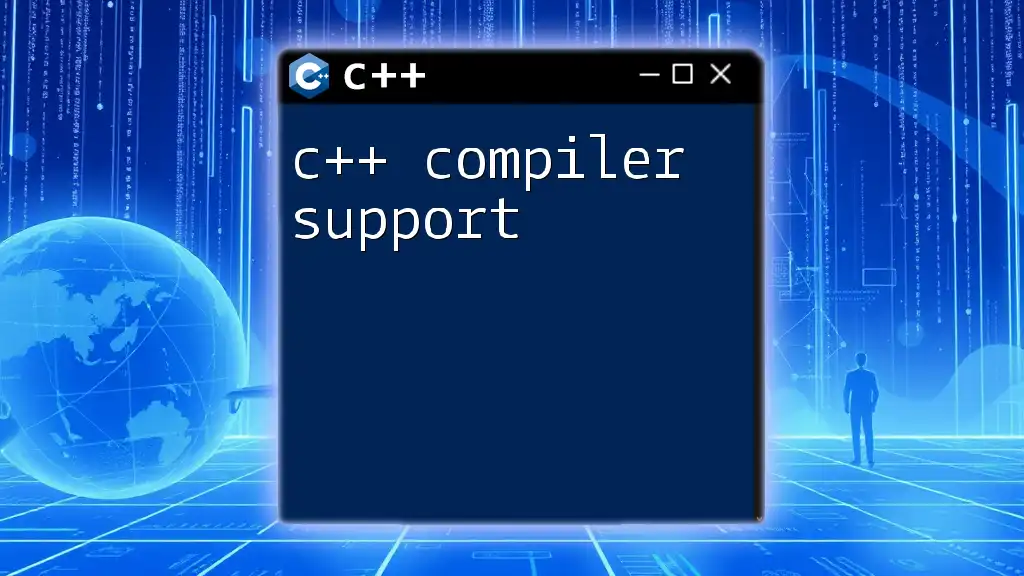
Writing Your First C++ Program
Code Snippet: Hello World Program
Now it's time to write your first C++ program. Write the following code in the main.cpp file:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code, `#include <iostream>` is essential for using standard input and output streams. The `main` function serves as the entry point to your program, while `std::cout` is responsible for displaying output on the screen.
Compiling and Running Your Code
To compile and run your project in Visual Studio:
- Click on the Local Windows Debugger button or press F5. This action builds the project and runs it.
- Upon successful execution, the output window should display "Hello, World!".
If you encounter errors, Visual Studio will clearly indicate the issues, allowing you to troubleshoot effectively.
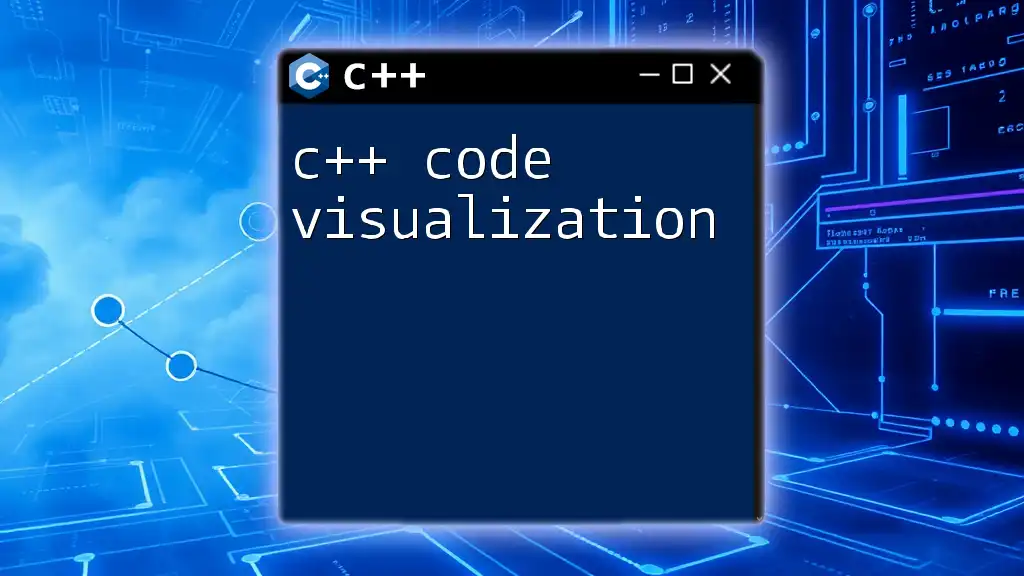
Debugging in Visual Studio
Importance of Debugging
Debugging is a crucial part of programming, allowing you to identify and fix errors, thereby ensuring your code runs smoothly. Visual Studio offers excellent tools to facilitate this process.
Debugging Tools in Visual Studio
Among the key features for debugging are:
- Breakpoints: Setting breakpoints in your code allows you to pause execution at specific lines. This is useful for examining variable states.
- Watches: This tool lets you monitor variables as your application runs.
- Call Stack: Displays the active function calls, aiding in tracking program flow.
To set a breakpoint, click on the left margin next to the code line where you want execution to pause. Starting the debugger will reveal how your program behaves at that point.
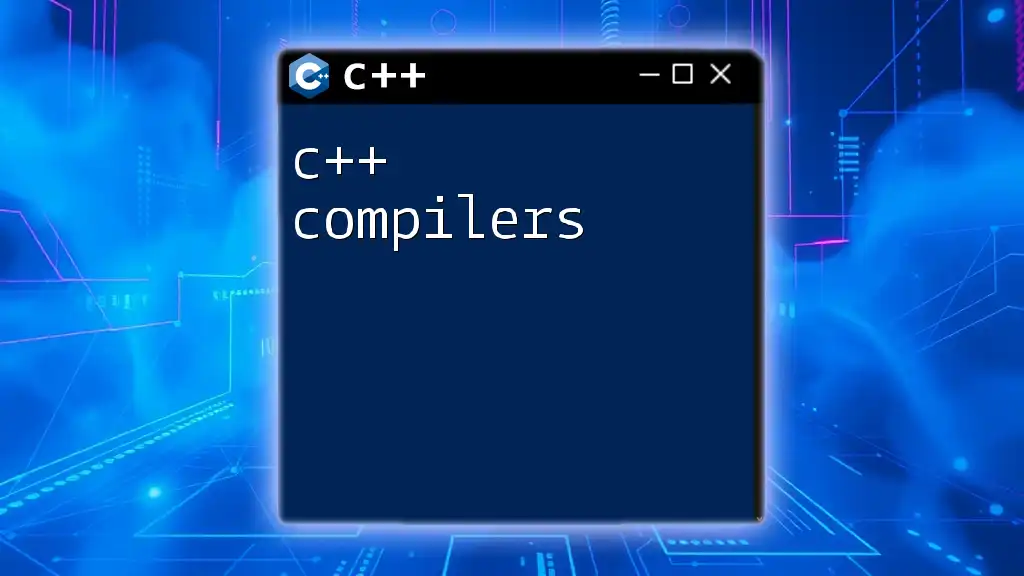
Additional Features of Visual Studio for C++
Code IntelliSense and Analyzers
Visual Studio’s IntelliSense feature enhances productivity by providing code suggestions and completions as you type. This not only speeds up coding but also reduces syntax errors. Error squiggles appear in real-time, guiding you to fix issues proactively as you write the code.
Project Configuration and Management
Managing project configurations is a breeze with Visual Studio. By default, projects are configured in two modes: Debug and Release.
- Debug Mode: Ideal for development, allowing you to step through code and inspect variables.
- Release Mode: Used for final builds, optimizing the code for better performance.
You can easily switch configurations through the toolbar, allowing for seamless transitions between development and production environments.
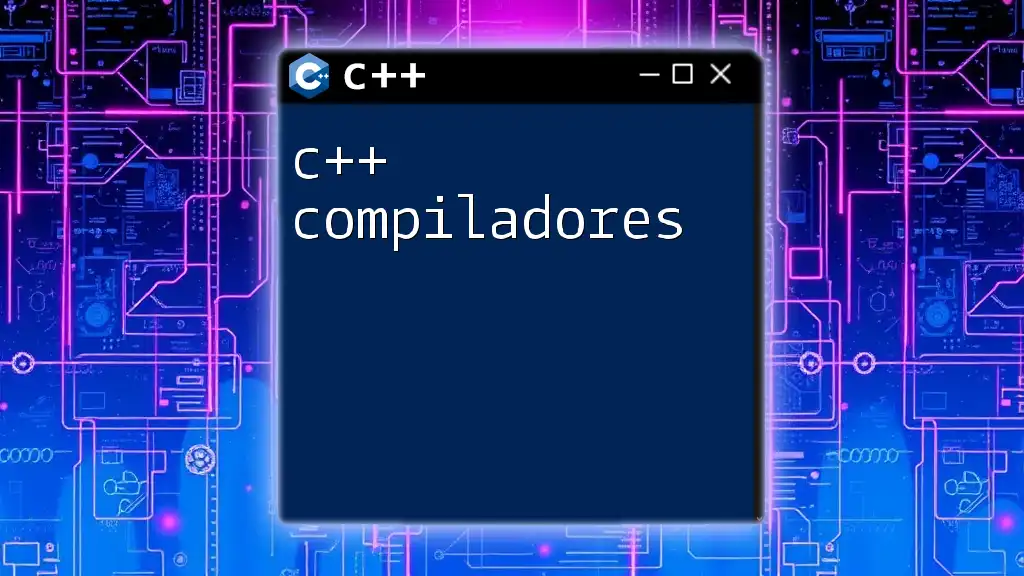
Troubleshooting Common Issues
Installation Problems
Sometimes installation can present challenges. Common errors include:
- Missing components: Ensure you selected the required workloads during installation.
- System requirements: Verify your system meets the access requirements for running Visual Studio.
Compilation Errors
Compilation errors can stem from various issues, often indicating problems in your code. Common examples include:
- Syntax mistakes: Review error messages for guidance on fixing code lines.
- Undefined references: Ensure all necessary headers are included and check your project settings.
Runtime Errors
Runtime errors occur when the program is executed. Debugging tools like breakpoints and variable watches are indispensable for addressing these issues.
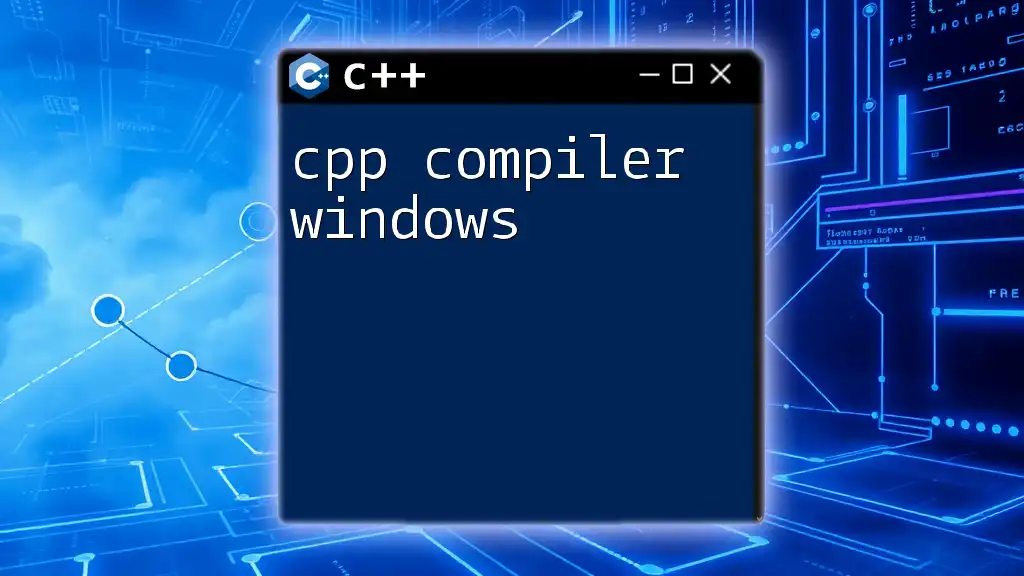
Conclusion
Using the C++ compiler in Visual Studio enhances your development experience and provides comprehensive tools for coding, debugging, and optimizing your applications. As you explore its many features, you’ll find that Visual Studio is not only an IDE but also a powerful ally in your C++ programming journey.
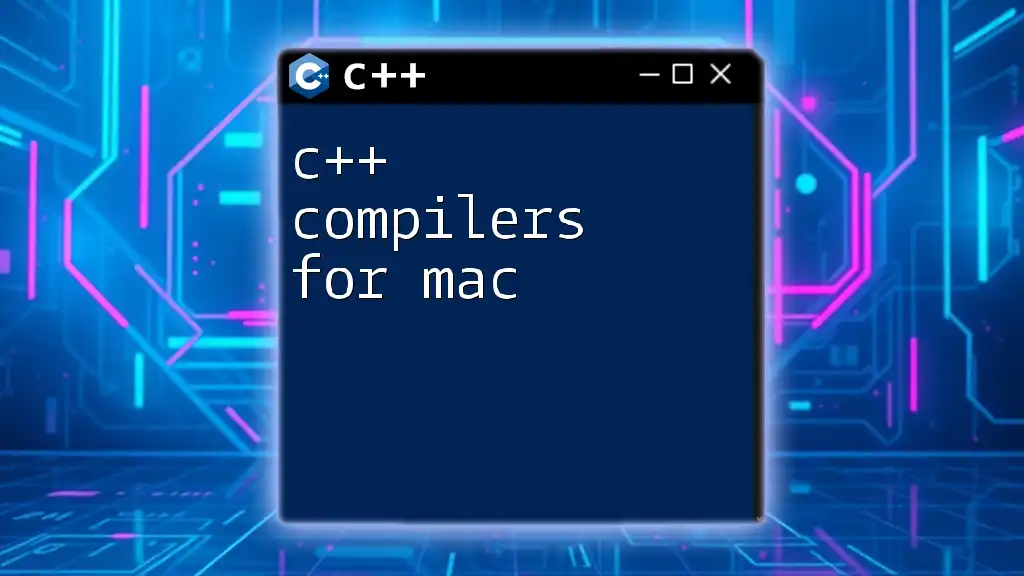
Call to Action
We invite you to share your experiences with Visual Studio or ask questions in the comments below. To stay updated with more tips, tutorials, and insights on mastering C++, subscribe to our newsletters and follow our community!