To exit a program in C++, you can use the `exit()` function from the `<cstdlib>` header, or return a value from the `main()` function.
Here's a code snippet demonstrating both methods:
#include <cstdlib> // Required for exit()
int main() {
// Method 1: Using exit()
exit(0); // Exits the program with a status code of 0
// Method 2: Using return
return 0; // Exits the program with a status code of 0
}
Understanding Exiting in C++
What Does it Mean to Exit a C++ Program?
Exiting a C++ program refers to the process of terminating the execution of the program and returning control to the operating system. Properly exiting a program is crucial as it ensures that all resources are released and that the program signals its completion status.

How to Exit a C++ Program
Using the `exit()` Function
The `exit()` function is a built-in C++ function, defined in the `<cstdlib>` header. It provides a way to terminate a program from any point in the code. The syntax is:
void exit(int status);
In this case, the parameter status is an integer that indicates the exit status of the program. Conventionally, a status code of 0 indicates success, while any non-zero value indicates an error.
Here is an example demonstrating its use:
#include <iostream>
#include <cstdlib> // For exit()
int main() {
std::cout << "Program is exiting with status 0." << std::endl;
exit(0);
}
Understanding Exit Status Codes
Exit status codes are valuable for debugging and scripting. When you use a non-zero exit code, it signals an error condition to the operating system or calling program. For example:
- 0: Successful completion
- 1: Generic error
- 2: Misuse of shell builtins (according to Bash documentation)
The `return` Statement in `main()`
Another common method of exiting a C++ program is using the `return` statement. When you return a value from `main()`, it serves as the exit status for the program. For example:
#include <iostream>
int main() {
std::cout << "Program is ending with status 0." << std::endl;
return 0;
}
In this case, returning 0 explicitly states that the program completed successfully. It’s important to note that if you forget to include a return statement, many compilers will assume an implicit return of 0, but this behavior can vary.
Using `abort()` for Immediate Termination
If immediate termination of the program is required—typically due to an unrecoverable error—you can use the `abort()` function from the `<cstdlib>` header. Unlike `exit()`, which performs cleanup routines, `abort()` stops the program unexpectedly and does not execute destructors for static objects.
Here's how you might use `abort()`:
#include <iostream>
#include <cstdlib> // For abort()
int main() {
std::cout << "Program is aborting!" << std::endl;
abort();
}
Calling `abort()` typically results in a core dump, which is useful for debugging.

Exiting from Non-Main Functions
Exiting from non-main functions can be done using `exit()`, which allows you to terminate the program regardless of the current function. This can be useful when specific conditions in subordinate functions necessitate stopping the entire application.
Consider this example:
#include <iostream>
#include <cstdlib>
void riskyFunction() {
std::cout << "Exiting from riskyFunction due to an error." << std::endl;
exit(1);
}
int main() {
riskyFunction();
std::cout << "This line will not execute." << std::endl;
return 0;
}
In the above scenario, the call to `exit(1)` in `riskyFunction()` effectively terminates the entire program, and thus the message in `main()` will not be printed.
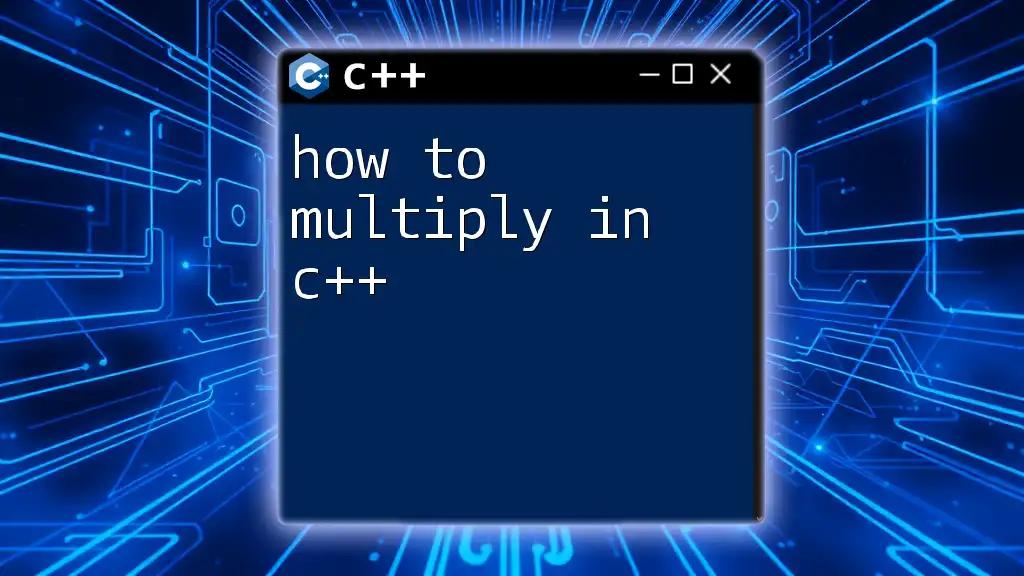
Best Practices for Exiting a C++ Program
When to Use Each Method
Choosing the right exit method is vital for writing clear and maintainable code. Use:
- `exit()`: When you want to terminate the program from anywhere in your code and need to specify an exit status.
- `return` in `main()`: When you finish executing `main()` and want to inform the operating system about the program's success or failure.
- `abort()`: When an unrecoverable error occurs, and you need to terminate the program immediately without cleanup.
Graceful Shutdown
It is essential to perform a graceful shutdown by cleaning up resources before exiting. This can be especially important for applications that manage dynamic memory, file handles, or network connections. You can use the `atexit()` function to register cleanup functions that will run upon normal program termination.
Here's an example demonstrating cleanup:
#include <iostream>
#include <cstdlib>
void cleanup() {
std::cout << "Cleaning up resources..." << std::endl;
}
int main() {
atexit(cleanup); // Register cleanup function
std::cout << "Exiting the program." << std::endl;
exit(0);
}
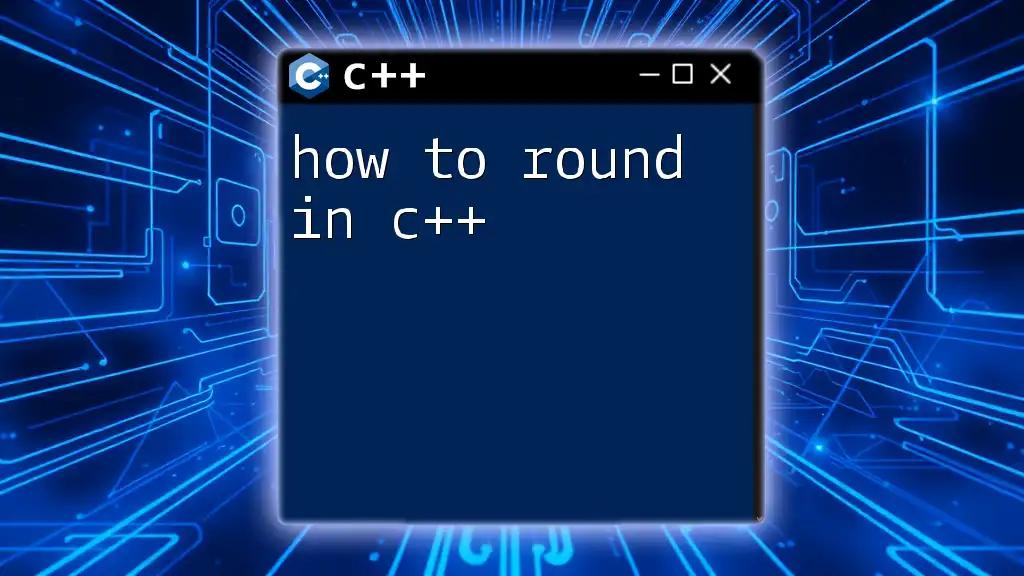
Common Mistakes When Exiting a C++ Program
Forgetting to Return a Value from `main()`
One common mistake is failing to include a return statement in `main()`. While some compilers may automatically assume a return of 0, it is better to explicitly state your intended exit code for clarity and compatibility with different environments.
Using `exit()` without Cleanup
Another frequent error is invoking `exit()` without prior cleanup, which could lead to resource leaks or inconsistent states. Always consider whether your program needs to release resources or handle certain tasks before termination.

Conclusion
Understanding how to exit the program in C++ is essential for writing effective programs. This knowledge enables you to control the flow of your applications while ensuring they terminate smoothly and return the appropriate statuses to the operating system. By employing proper exit strategies, you can prevent resource leaks, improve error handling, and enhance the overall maintainability of your code.
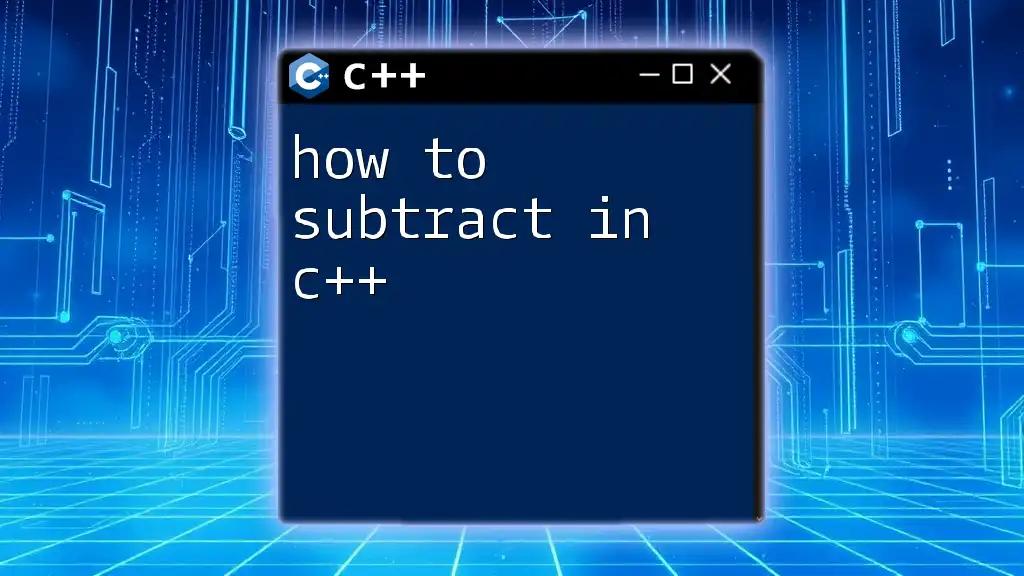
Further Reading
For more information, it’s recommended to consult the official C++ documentation on exit functions and to explore additional resources for mastering C++ programming fundamentals.