To include a header file in C++, use the `#include` preprocessor directive followed by the name of the header file in angled brackets for standard libraries or in double quotes for user-defined files.
#include <iostream> // for standard libraries
#include "myHeader.h" // for user-defined header files
Understanding Header Files in C++
What Are Header Files?
Header files in C++ are files that contain declarations of functions, classes, and variables, which can be shared between multiple source files. They typically have a `.h` or `.hpp` extension and are critical for organizing code effectively. By using header files, developers can promote reusability and keep their code modular.
The Role of Header Files in C++
Header files serve several vital roles in C++ programming:
-
Code Reusability: By placing commonly used functions and definitions in header files, developers can reuse the same code across various projects without duplicating it in every source file.
-
Separation of Interface and Implementation: Header files allow you to separate the interface (what functions do) from the implementation (how they work), making projects easier to manage.
-
Faster Compilation: Only changes made to a header file necessitate recompilation of source code that includes it, rather than recompiling everything.
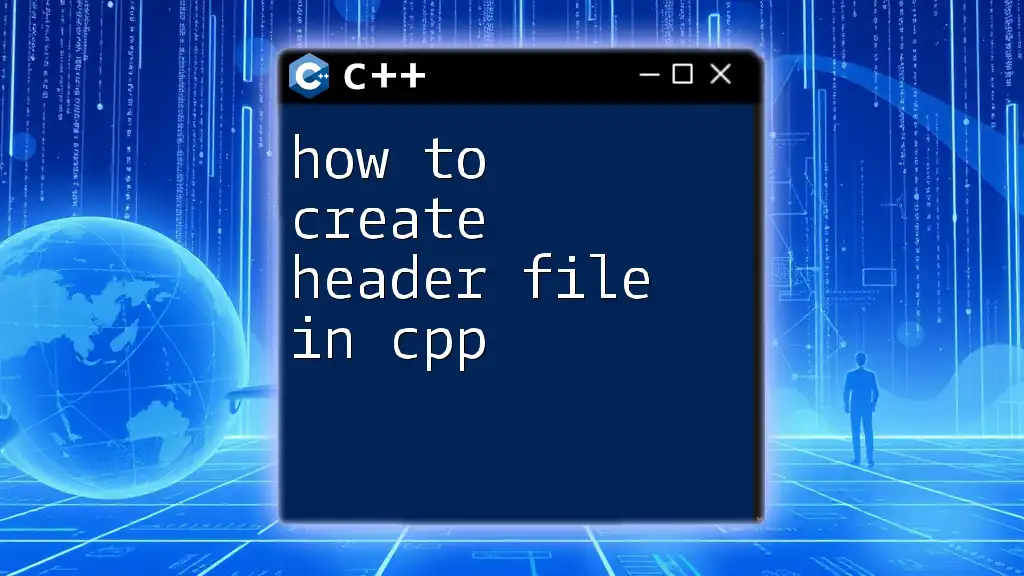
How to Include Header Files in C++
Syntax of the Include Directive
The inclusion of header files in C++ is achieved using the `#include` directive. The syntax can be one of two forms:
-
Standard Library Header Files:
#include <filename>
This form is used for system or library headers. For example, including the standard I/O library:
#include <iostream>
-
User-Defined Header Files:
#include "filename"
This form is used when including your own header files. For instance, if you have a custom header named `myheader.h`, you would include it as follows:
#include "myheader.h"
Including Standard Library Header Files
One of the most common header files to include is `<iostream>`, which provides functions for input and output operations. Here’s a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
In this code, we are including the `<iostream>` standard header, enabling us to use the `std::cout` object to print "Hello, World!" to the console.
Including User-Defined Header Files
Creating and using user-defined header files is straightforward.
-
Create a User-defined Header File: Here’s a simple example of a custom header named `myheader.h`:
// myheader.h #ifndef MYHEADER_H #define MYHEADER_H void myFunction(); #endif
This header file declares a function `myFunction()`. The `#ifndef`, `#define`, and `#endif` preprocessor directives are known as header guards and prevent multiple inclusions of the same header file, which could lead to compilation errors.
-
Include the Header File: To use this header in your source file, you would include it as follows:
// main.cpp #include <iostream> #include "myheader.h" void myFunction() { std::cout << "This is my function!"; } int main() { myFunction(); return 0; }
In this example, we include the user-defined header `myheader.h` and define the `myFunction()` within `main.cpp`, allowing us to use it seamlessly in the `main()` function.
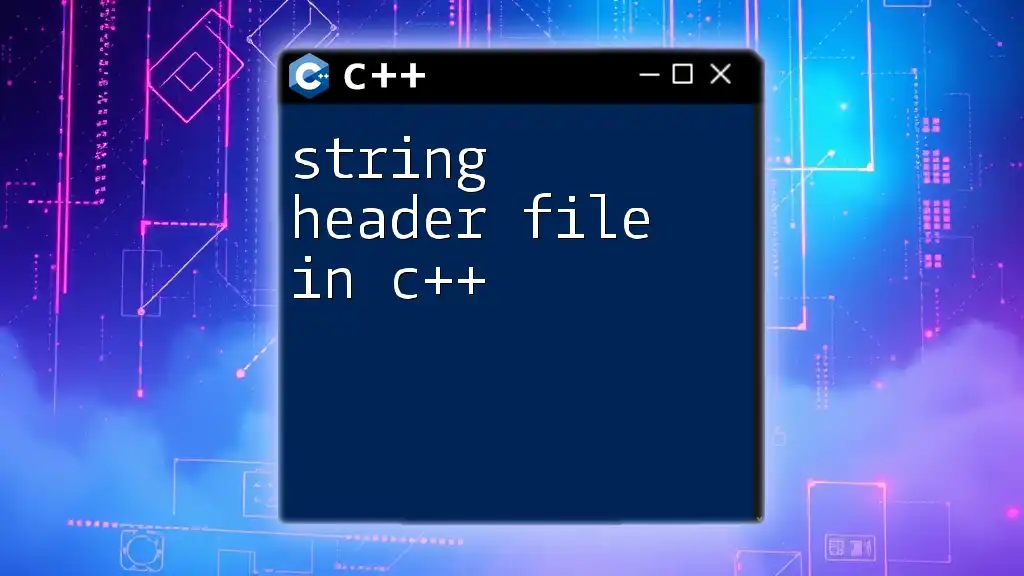
Practical Examples of Including Header Files
Example 1: Simple Program Using iostream
Below is a basic program that demonstrates utilizing the standard library header `<iostream>`:
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
In this program, the header `<iostream>` facilitates basic input and output operations using `std::cout`. Each statement illustrates how straightforward C++ can be while utilizing header files.
Example 2: Using a User-Defined Header File
Let’s take the previous header file example and run it in full:
// main.cpp
#include <iostream>
#include "myheader.h"
void myFunction() {
std::cout << "This is my function!";
}
int main() {
myFunction();
return 0;
}
Here, we import `myheader.h`, and within `main()`, we simply call `myFunction()` to execute its code, which prints "This is my function!". This simplicity showcases the power of organizing your code with header files.
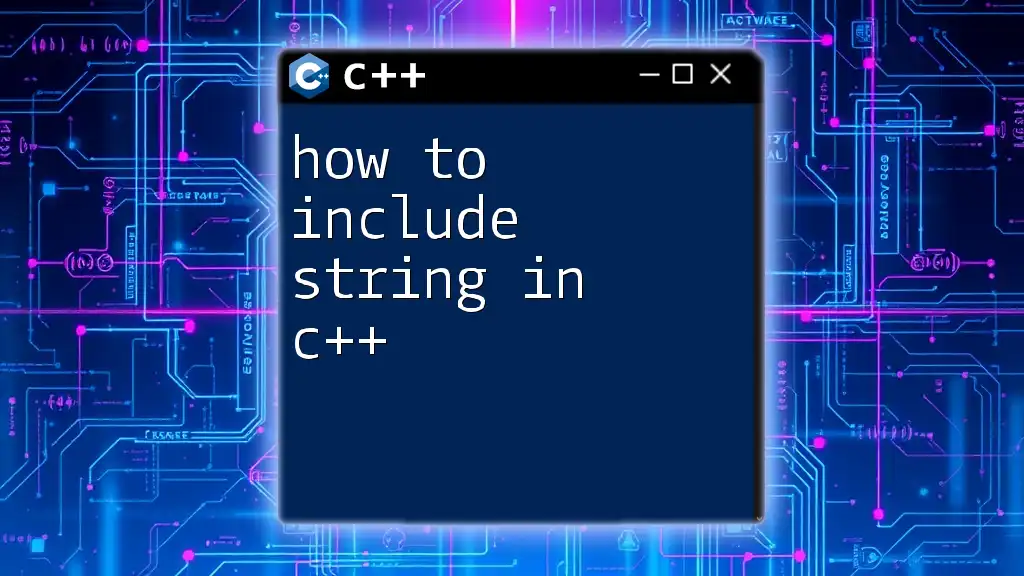
Common Errors When Including Header Files
Misplaced Include Directives
Placing include directives should be done at the top of your source file. If they are misplaced or added after function definitions, it will result in compilation errors as the compiler would not recognize the functions declared in them.
Missing Header Guards
If you forget to add header guards to a user-defined header file, it can create multiplication inclusion problems, leading to redefined identifier errors. Always ensure each header file has proper guards to prevent compilation conflicts.
Compilation Errors
Common compilation errors related to header inclusion can include:
- Undefined References: This occurs when a function is declared in a header but not defined anywhere in your code.
- Multiple Definitions: If a file is included multiple times without guards, the compiler will see multiple copies of the same declaration.
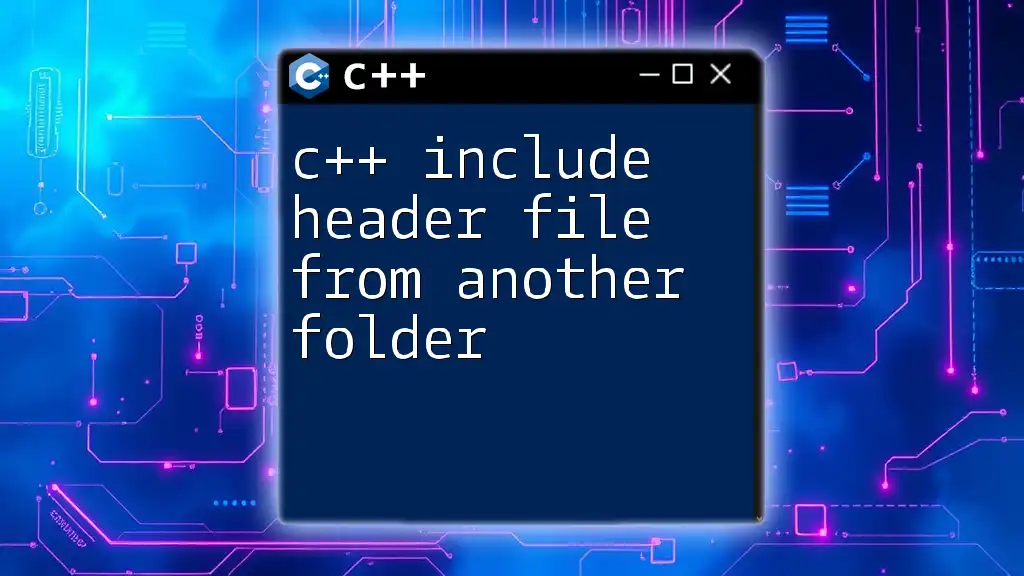
Best Practices for Including Header Files
Organizing Your Header Files
To maintain clarity and efficiency:
- Group related functions into a single header file.
- Keep your header files independent; avoid circular dependencies where two headers include each other.
Avoiding Inclusion Loops
Inclusion loops can lead to long compile times and ambiguous references. To avoid these loops:
- Ensure that each header includes necessary dependencies in a controlled manner.
- Use forward declarations when possible to minimize includes in headers.
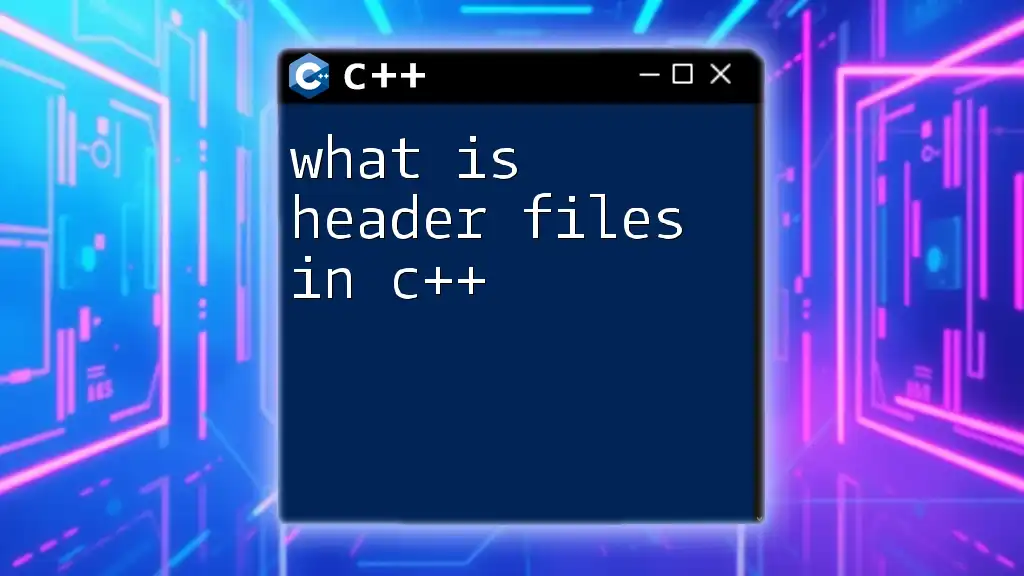
Conclusion
Knowing how to include a header file in C++ is fundamental for any C++ developer. Header files help organize code, extend modularity, and enable code reuse. Utilizing them effectively promotes better coding practices and eases project management. By embracing header files and following best practices, you can enhance your C++ programming skills significantly.
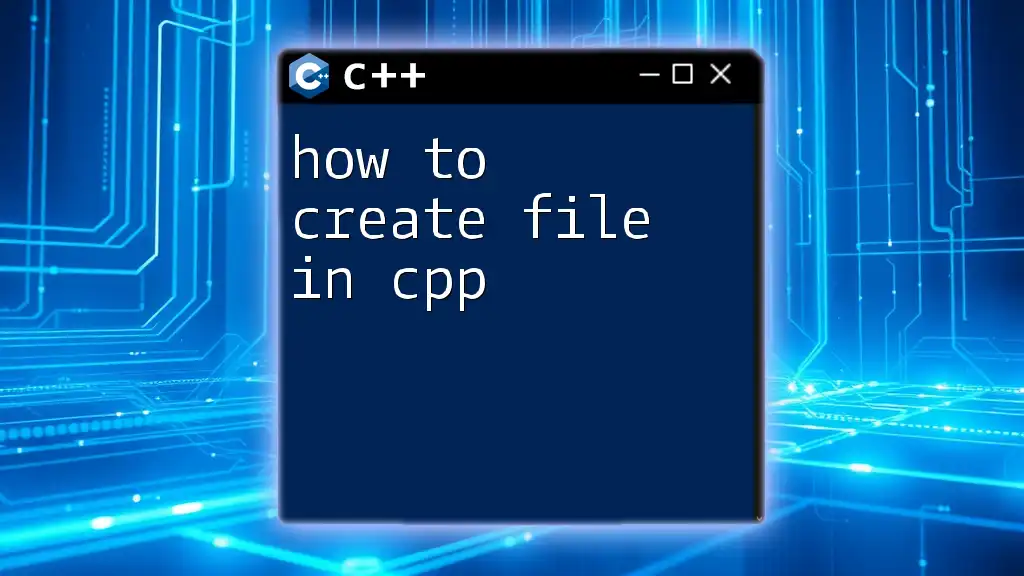
Call to Action
To further improve your C++ proficiency, explore more tutorials on C++ commands and concepts. Practice the inclusion of header files by creating your own projects, and don’t hesitate to dive deeper into advanced topics surrounding the C++ programming language.