To read a text file in C++, you can use the `ifstream` class from the `<fstream>` library to open the file and read its contents line by line. Here's a simple example:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
if (file.is_open()) {
while (getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
} else {
std::cout << "Unable to open file" << std::endl;
}
return 0;
}
Understanding File I/O in C++
File Input/Output (I/O) is a critical concept in C++ programming, allowing developers to interact with external data through files. Reading from a text file is an essential skill for handling data, logging information, or simply processing configurations. In C++, file operations are performed through streams. The standard library provides different classes to facilitate these operations, with the primary ones being `ifstream`, `ofstream`, and `fstream`.
What is `ifstream`?
The `ifstream` (input file stream) class is specifically designed for reading files. It allows you to open a file and retrieve its content easily. Unlike `ofstream`, which is used for writing files, `ifstream` is your gate to accessing data that already exists.
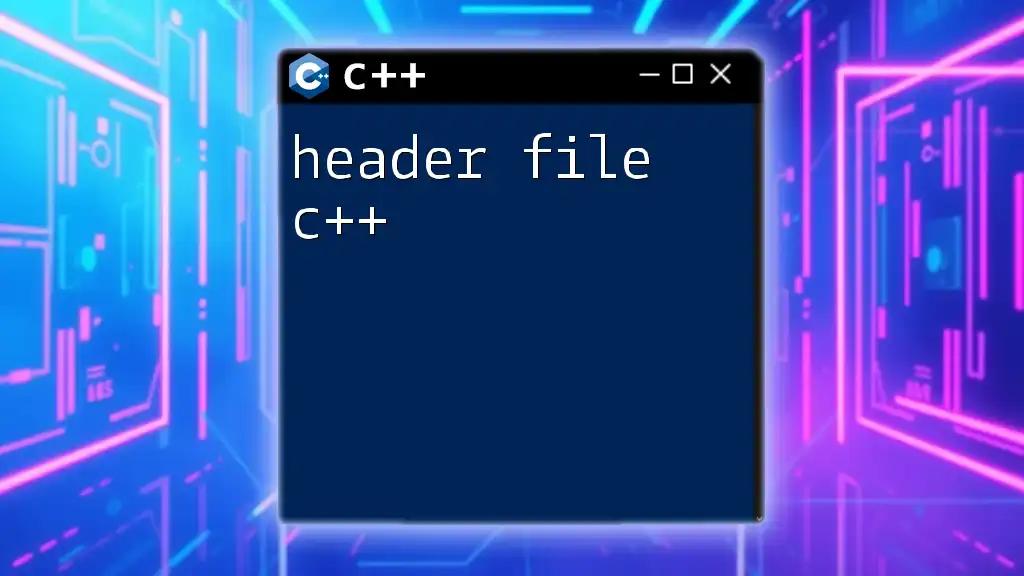
Setting Up Your Environment
Before diving into code, confirm that your development environment is prepared for file operations. Ensure that you have a compiler (like GCC or Clang) or an Integrated Development Environment (IDE) such as Visual Studio or Code::Blocks. Most modern IDEs come with built-in support for file handling, making the process simple and efficient.

How to Open a Text File in C++
Opening a text file requires a straightforward syntax. Here’s how you can do it with `ifstream`:
#include <fstream>
#include <iostream>
int main() {
std::ifstream inFile("example.txt"); // Opening the file
// Continue with file reading...
}
Error Handling
A crucial aspect of reading files is ensuring that the file opens without issues. It’s good practice to check whether the file was successfully opened before proceeding with reading. Here's how you can implement basic error handling:
if (!inFile) {
std::cerr << "Unable to open file";
return 1; // Exit program
}
This code checks whether `inFile` could open the specified file; if not, it will display an error message and terminate the program.

Reading a Text File in C++
Reading Line by Line
One of the most common methods to read a text file is by reading it line by line using `getline()`. This approach is particularly useful for processing structured data.
std::string line;
while (std::getline(inFile, line)) {
std::cout << line << std::endl;
}
This loop continues to retrieve each line from the `inFile` until it reaches the end of the file. The benefit of this method is that it preserves the format of the text and allows you to manipulate or analyze each line individually.
Reading Word by Word
Another approach is to read the file word by word. This can be achieved by using the extraction operator (`>>`).
std::string word;
while (inFile >> word) {
std::cout << word << std::endl;
}
This method treats whitespace as delimiters and is often useful when you need to process individual words, such as counting occurrences or filtering specific terms.
Reading Character by Character
For specific applications where every character matters, you might want to read the file character by character. The `get()` method allows this functionality.
char ch;
while (inFile.get(ch)) {
std::cout << ch;
}
This approach can be useful for tasks such as text analysis or editing, where you need complete control over the input stream.

Examples of Reading from a Text File in C++
Full Program Example
Here is a simple yet comprehensive program that demonstrates how to read from a text file using the methods outlined above:
#include <fstream>
#include <iostream>
int main() {
std::ifstream inFile("example.txt"); // Opening the file
if (!inFile) { // Error handling
std::cerr << "Unable to open file";
return 1; // Exit program
}
// Reading and displaying the file line by line
std::string line;
while (std::getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close(); // Always close the file
return 0;
}
This program showcases the fundamental steps for reading a text file in C++. It begins by opening a file, checks for any errors, and then proceeds to read line by line, printing each line to the console before closing the file—a crucial final step to free up system resources.
Common Pitfalls and How to Avoid Them
When dealing with file operations, several common mistakes can occur. A few pitfalls include:
- Forgetting to close the file: Always remember to call `close()` on your file stream to ensure that resources are released properly.
- Not checking if the file opened correctly: Failing to include error handling can lead to difficult-to-diagnose problems later in your code.
- Assuming that the file exists: Make sure that the file path is correct and that the file exists at the specified location.
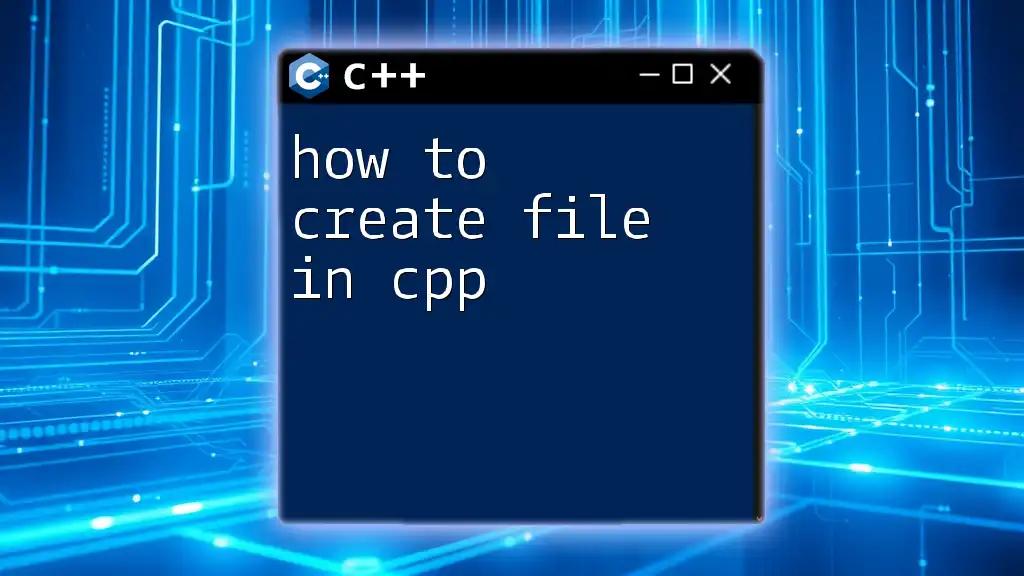
Best Practices for Reading Files in C++
Here are some best practices to keep in mind when reading files in C++:
- Use descriptive variable names: This practice helps make your code more readable and maintainable.
- Extract reading logic into functions: By modularizing your code, you enhance reusability and simplify debugging.
- Handle exceptions gracefully: Use try-catch blocks to manage exceptions so that your program can exit gracefully or retry reading from the file.
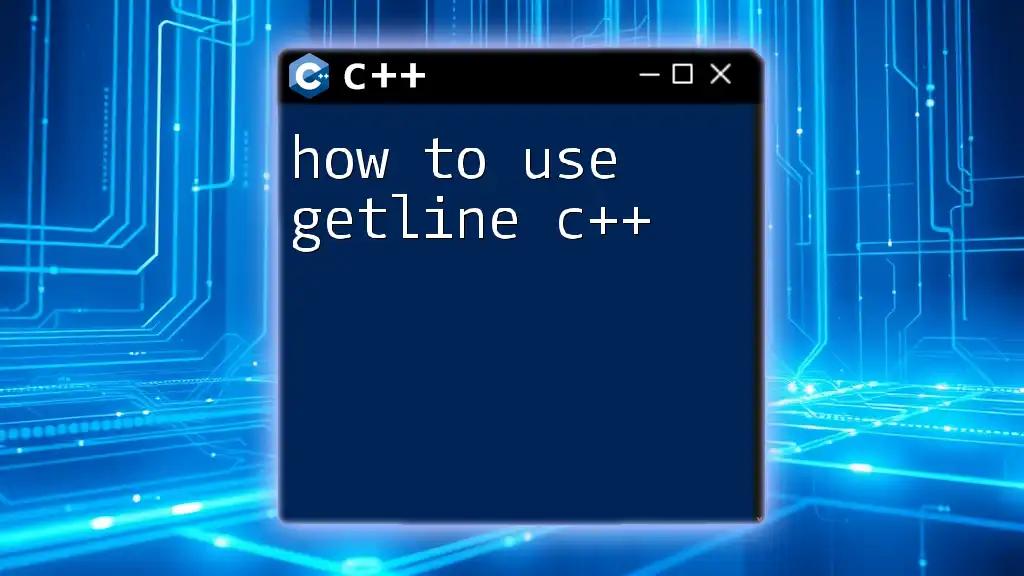
Conclusion
In summary, understanding how to read a text file in C++ is an essential skill for any programmer. The methods covered—reading line by line, word by word, or character by character—provide versatile options for various applications. By following best practices and ensuring proper error handling, you can effectively manage file I/O in your C++ projects. Practice with your own text files to solidify these concepts and enhance your programming capabilities.