To check CPP contributions, you can use the `cppcheck` command-line tool to analyze your code for potential issues and contributions in coding quality. Here's a simple snippet to run the tool:
cppcheck --enable=all --inconclusive path/to/your/code.cpp
Understanding C++ Contributions
What Are C++ Contributions?
C++ contributions refer to any code changes, enhancements, or fixes made to C++ projects that improve functionality, fix bugs, or add new features. These contributions are essential in collaborative environments, enabling developers to work together effectively toward enhancing software. Recognizing contributions, whether by individuals or teams, is crucial for promoting accountability and fostering growth.
Why Check C++ Contributions?
Understanding how to check C++ contributions offers several benefits. For individual developers, it allows for self-assessment and identifies areas for improvement. Within a team, analyzing contributions can indicate how effectively team members are collaborating and identify potential skill gaps or resource needs. Organizations can leverage contribution data to evaluate overall productivity and project health.

Getting Started with Contribution Checking
Setting Up Your Environment
To effectively check C++ contributions, you need to set up a conducive development environment. This includes:
- Required Tools and Software: Essential items like Git for version control, a C++ compiler (such as GCC or Clang), and an integrated development environment (IDE) like Visual Studio, Code::Blocks, or CLion.
- Installing Necessary Compilers and IDEs: Follow the installation guidelines specific to your operating system, ensuring that your code can compile correctly.
Acquiring Contribution Data
C++ contributions are often tracked within version control systems, with Git being the most widely used. To get started, you need to clone the repository that contains the C++ project you're interested in. This is done using the command:
git clone https://github.com/username/repository.git
By cloning the repository, you'll have local access to the entire project history, allowing you to analyze contributions conveniently.
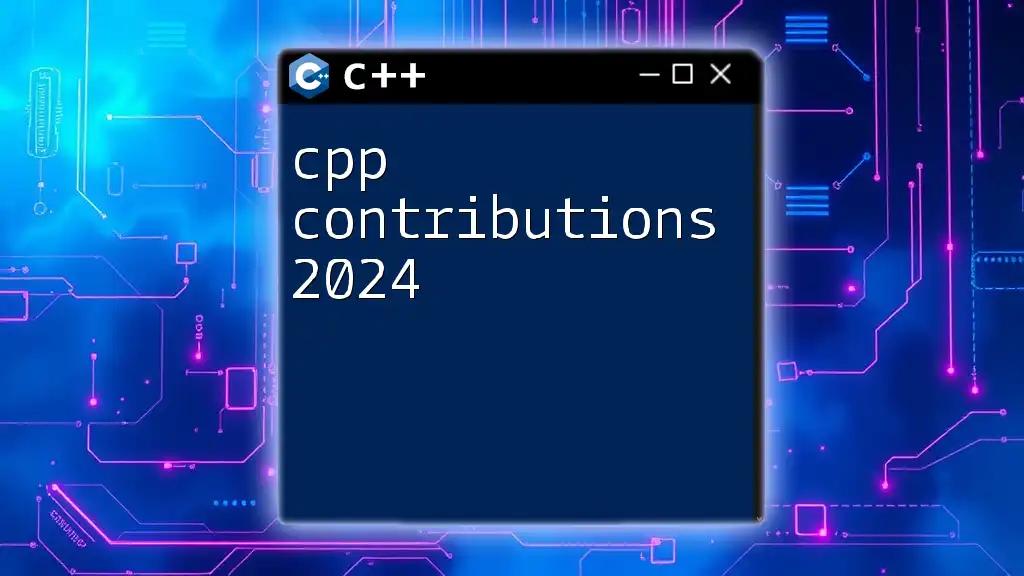
Methods to Check C++ Contributions
Utilizing Git Commands
Introduction to Git for Contribution Checking
Git is a powerful tool for tracking changes to your codebase. It keeps a detailed history of contributions made by all collaborators, allowing you to analyze this data precisely. By utilizing various Git commands, you can gather valuable insights into contribution patterns.
Key Git Commands for Contribution Checking
-
`git log`: This command lets you explore the commit history, showing a list of all changes made, along with their authors. To focus on contributions by a specific author, you can use:
git log --author="Your Name"
This will filter the log to display only commits authored by you. Understanding how to interpret this output is crucial, as it will show timestamps, commit messages, and specific files changed.
-
`git shortlog`: Use this command to get a summarized view of contributions sorted by author. The command looks like this:
git shortlog -sn
The output provides a concise view of how many commits each author has made to the repository. This summary is particularly useful in collaborative projects where multiple contributors are involved, highlighting who has been most active.
Online Platforms and Tools
Using GitHub Insights
Many C++ projects are hosted on GitHub, which provides built-in tools for tracking contributions. By navigating to the “Insights” section of your repository, you can access a wealth of data, including a contribution graph that visually represents commits over time. This graph can be filtered by author, which allows for deeper analysis of individual contributions.
Other Popular Platforms
In addition to GitHub, platforms like Bitbucket and GitLab also offer contribution tracking features. These platforms have tools for analyzing commit frequency, open issues, and pull requests. Moreover, utilizing third-party analytics tools like CodeClimate can provide even more granular insights into code quality and contributions.
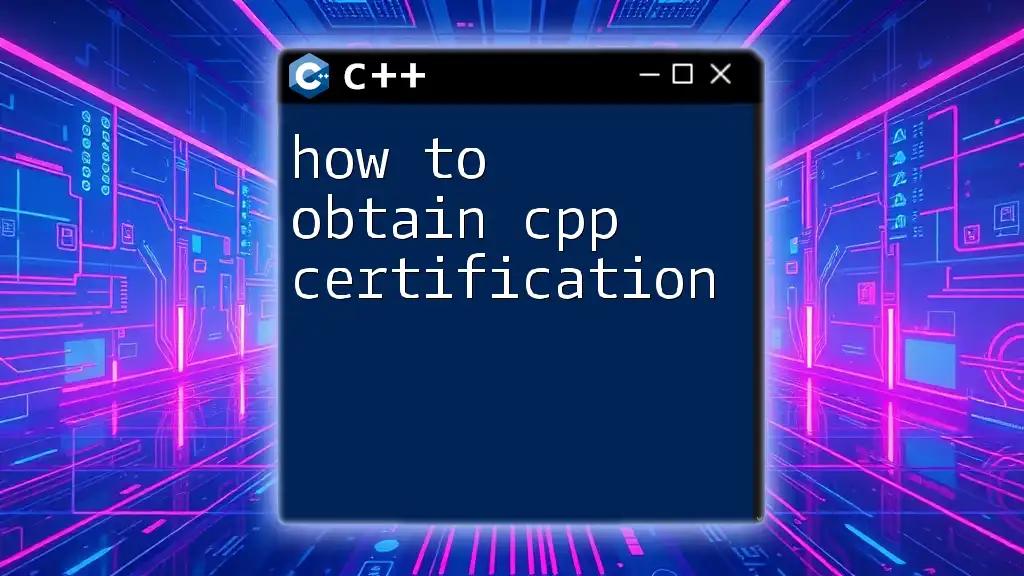
Analyzing Contribution Data
Understanding Contribution Metrics
Commit Frequency
Analyzing commit frequency can reveal insights into a developer's productivity and engagement level. Regular commits suggest that a developer actively participates in the project, enabling incremental improvements and quicker problem resolution.
Lines of Code (LOC) Added/Deleted
Lines of code (LOC) metrics can also indicate the scope of a developer's contributions. While the number of lines alone isn't a perfect measure of productivity—since quality over quantity often holds true—it does provide context. Tools like cloc can accurately calculate lines of code added or deleted, helping to quantify contributions effectively.
Visualizing Contributions
Creating Contribution Graphs
Visualizing contributions can amplify understanding and engagement within teams. Tools such as Gource can generate animated visual representations of contributions over time, showcasing how the codebase has evolved and how each developer contributes. This visualization can foster discussions about project trends and team dynamics.

Best Practices for Tracking and Improving Contributions
Regularly Review Contributions
Establishing a routine for reviewing contributions is vital for continuous improvement. Regular assessment can help identify patterns, recognize standout contributors, and promote accountability within the development team. Setting up weekly or monthly review sessions fosters an environment of openness and motivation.
Encouraging Collaboration
Fostering a culture of collaboration can significantly enhance contributions. Encourage team members to participate in open-source projects or contribute to shared codebases. Tools like GitHub and GitLab provide collaborative features such as pull requests and code reviews which can help cultivate teamwork and improve the overall quality of contributions.
Continuing Education
Promoting continuous education among team members is essential in the fast-evolving tech landscape. Provide resources, such as online courses, relevant literature, and access to community forums, to help developers improve their C++ skills. Participation in local meetups or tech conferences can also enhance technical knowledge and networking opportunities.
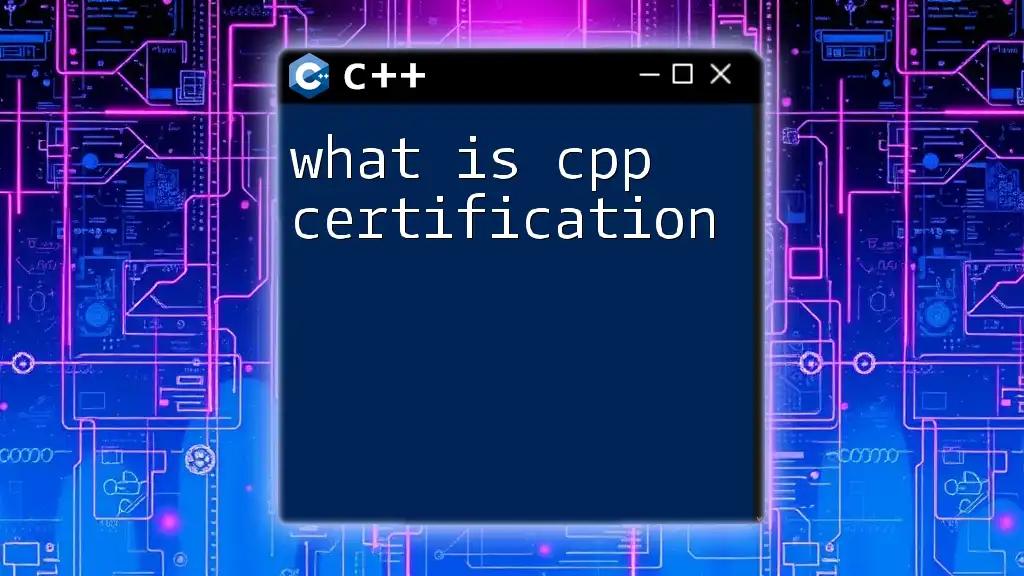
Conclusion
Summary of Key Takeaways
Understanding how to check C++ contributions is vital for both individual developers and teams. Key practices such as utilizing Git commands, leveraging online tools, regularly reviewing contributions, and fostering collaboration can enhance both individual and team performance. Continuous education is essential for keeping skills sharp and adapting to industry changes.
Call to Action
Stay tuned for more insights and tips on improving your C++ contributions. Your journey in mastering C++ development doesn’t end here—engage with the community and explore how you can further enhance your skills. Share your experiences with contribution tracking and let us know what you’ve found most helpful on your path to becoming a more effective contributor!

Appendix
Helpful Resources and Links
- [Git Documentation](https://git-scm.com/doc)
- [C++ Programming Community Forums](https://wwwcplusplus.com/forum/)
- [Recommended C++ Books and Online Courses](https://www.coursera.org/courses?query=c%2B%2B%20programming)