In C++, you can check if a key exists in a `std::map` using the `find` method, which returns an iterator to the element if found or `map::end()` if not.
#include <map>
#include <iostream>
int main() {
std::map<int, std::string> myMap = { {1, "one"}, {2, "two"}, {3, "three"} };
int keyToCheck = 2;
if (myMap.find(keyToCheck) != myMap.end()) {
std::cout << "Key found: " << keyToCheck << " -> " << myMap[keyToCheck] << std::endl;
} else {
std::cout << "Key not found: " << keyToCheck << std::endl;
}
return 0;
}
What is a Map in C++?
Definition and Purpose
In C++, a map is an associative container that stores elements in key-value pairs, allowing for the efficient retrieval of values based on their associated keys. The primary purpose of a map is to associate unique keys with specific values, enabling quick lookups, inserts, and deletions.
Characteristics of C++ Maps
Maps in C++ offer several important characteristics:
- Automatic Sorting: Keys are stored in a sorted order based on the key comparison function, making them easy to traverse in order.
- Uniqueness of Keys: Each key in a C++ map must be unique. If you try to insert a duplicate key, it will overwrite the existing value.
- Performance: Maps typically offer logarithmic time complexities for lookups, inserts, and deletions, making them quite efficient for many applications.
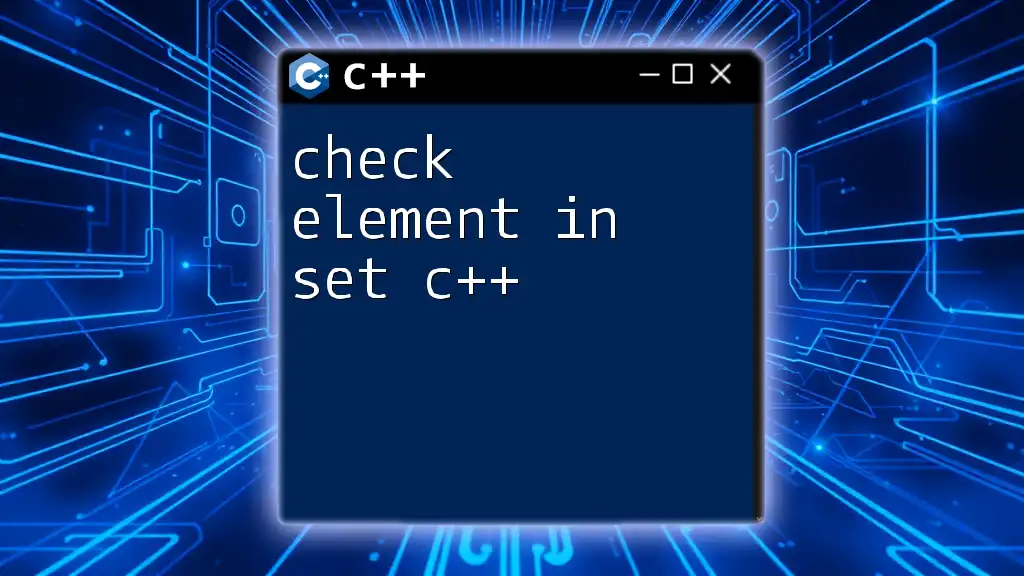
How to Create a Map in C++
Basic Map Syntax
To declare a map in C++, you typically use the `std::map` template from the Standard Template Library (STL). The basic syntax is as follows:
#include <map>
std::map<KeyType, ValueType> mapName;
In this syntax, `KeyType` represents the data type of the keys and `ValueType` represents the data type of the values.
Initializing a Map with Values
You can initialize a map with values at the time of declaration. Here's how:
std::map<std::string, int> myMap = {{"apple", 1}, {"banana", 2}, {"cherry", 3}};
This creates a map where "apple", "banana", and "cherry" are keys mapped to the respective integer values of 1, 2, and 3.
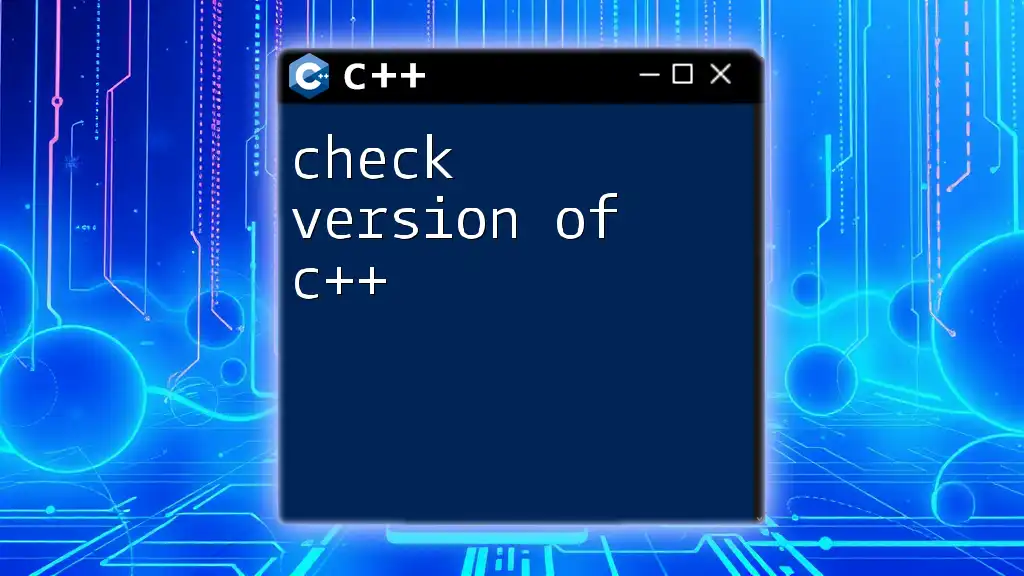
Checking if a Key Exists in a C++ Map
Using the `find()` Method
The `find()` method is a common way to check if a key exists in a C++ map. It returns an iterator to the element if found, or `map::end()` if not found.
Understanding the `find()` Method: This method allows you to perform a check without modifying the map, providing a way to identify if the key exists.
Example:
std::map<std::string, int> myMap = {{"apple", 1}, {"banana", 2}};
auto it = myMap.find("banana");
if (it != myMap.end()) {
// Key exists
std::cout << "Key found: " << it->first << " with value: " << it->second << std::endl;
} else {
// Key does not exist
std::cout << "Key not found." << std::endl;
}
In this example, the program checks if "banana" exists in `myMap` and prints its value if it does.
Using the `count()` Method
Another way to check if a key exists in a map is by using the `count()` method. This method returns the number of elements with a specified key.
Understanding the `count()` Method: Because a map does not allow duplicate keys, the count can only be 0 or 1.
Example:
if (myMap.count("kiwi") > 0) {
// Key exists
std::cout << "Key found: kiwi." << std::endl;
} else {
// Key does not exist
std::cout << "Key not found: kiwi." << std::endl;
}
This example shows how to check for the existence of the key "kiwi" using `count()`. Since "kiwi" is not present, the output will indicate that the key is not found.
Using the Subscript Operator
You can also use the subscript operator `[]` to check for a key’s existence, but be cautious—accessing a non-existent key will insert it with a default value.
How the Subscript Operator Works: When trying to access a key that does not exist, the subscript operator will create a new entry in the map with the default value for the value type.
Example:
int value = myMap["key"]; // This will create an entry for "key" with a default value of 0.
if (myMap["key"] == 0) {
std::cout << "Key added with a default value." << std::endl;
}
In this situation, if "key" was not previously in `myMap`, it gets added with a default integer value of 0.
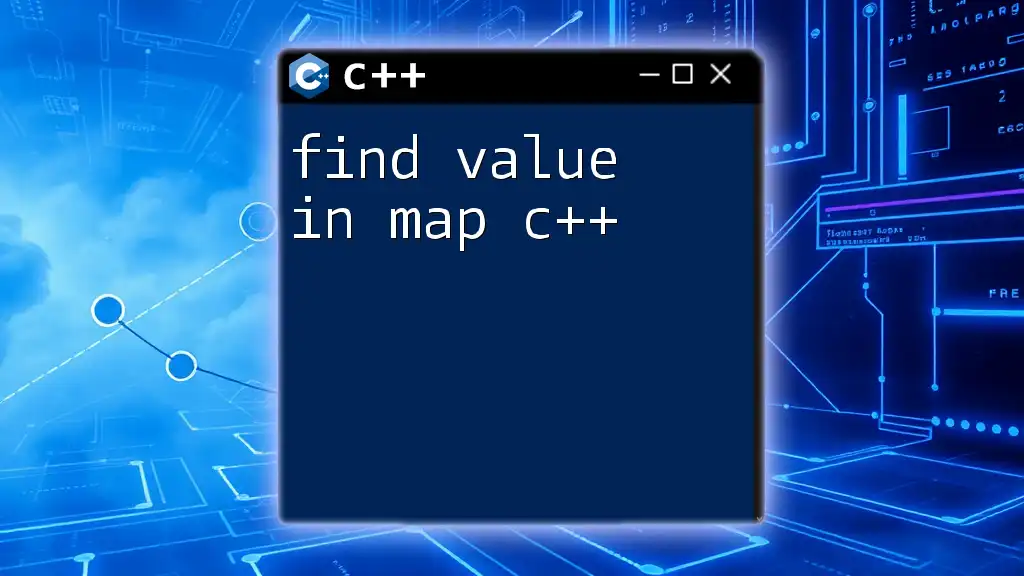
Best Practices for Key Existence Checks
Choosing the Right Method
When deciding how to check for the existence of a key, consider:
- `find()`: Use when you need to retrieve the corresponding value or perform additional operations.
- `count()`: A straightforward and quick option for checking if a key is present.
- Subscript: Use with caution, primarily if you want to also insert a default value if the key isn’t found.
Handling Non-Existent Keys Gracefully
In real-world applications, it’s often important to manage cases where keys are not present. Using C++17 and later, consider using `std::optional` for a more graceful approach that avoids unnecessary insertion when keys are absent.
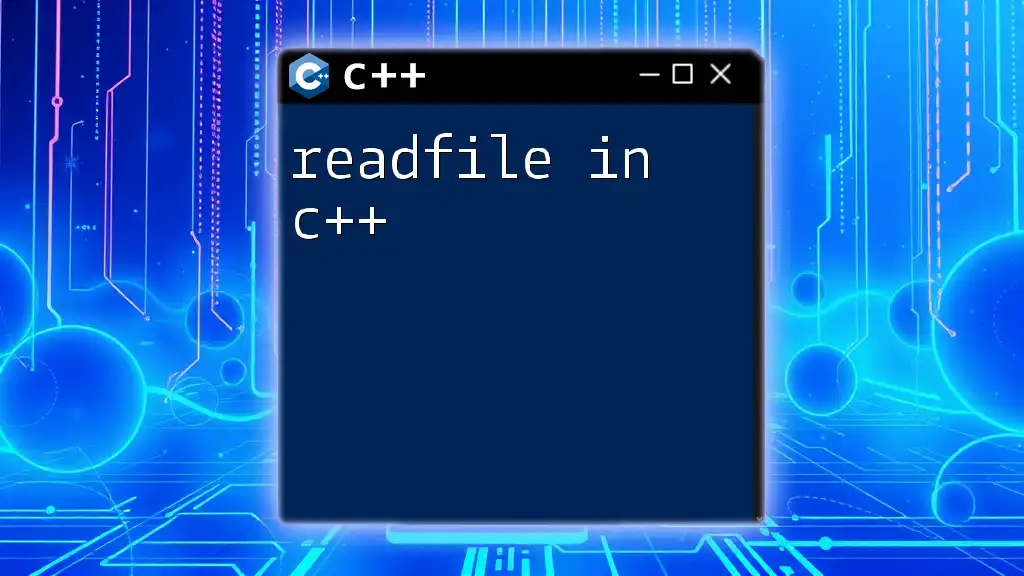
Real-World Examples
Example 1: User Authentication
In a simple authentication system, you might check if a user ID exists in the user ID map.
std::map<std::string, std::string> userMap = {{"user1", "password1"}, {"user2", "password2"}};
std::string inputUsername = "user1"; // Example input
if (userMap.find(inputUsername) != userMap.end()) {
std::cout << "User found, proceed to password verification." << std::endl;
} else {
std::cout << "User not found." << std::endl;
}
This demonstrates checking whether a user exists before proceeding with password verification.
Example 2: Inventory Management
In an inventory system, you might want to check whether a product is available before attempting to process an order.
std::map<std::string, int> inventoryMap = {{"itemA", 10}, {"itemB", 0}};
std::string productToCheck = "itemB";
if (inventoryMap.find(productToCheck) != inventoryMap.end() && inventoryMap[productToCheck] > 0) {
std::cout << "Product available for order." << std::endl;
} else {
std::cout << "Product out of stock." << std::endl;
}
This example checks both the existence of the key and its associated stock level, ensuring orders are only processed for available items.
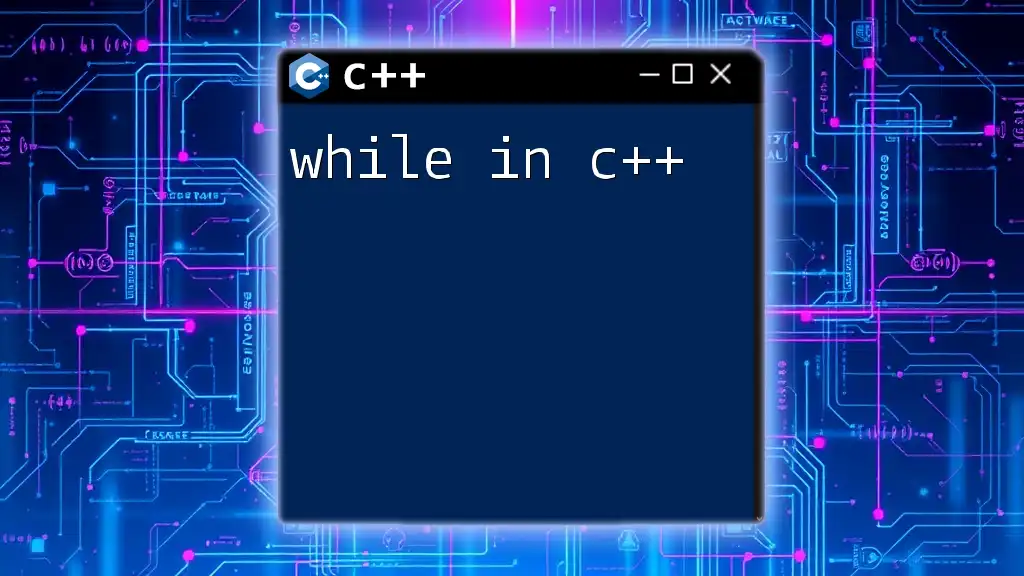
Conclusion
In this article, we covered various methods to check if a key exists in a map in C++. We discussed the different techniques such as using the `find()`, `count()`, and subscript operator, alongside practical examples to illustrate their use in real-world scenarios. Understanding how to efficiently check for key existence can enhance your programming skills and help you make the most of C++ maps. As you continue to explore C++, keep practicing these methods for effective key management in your applications.
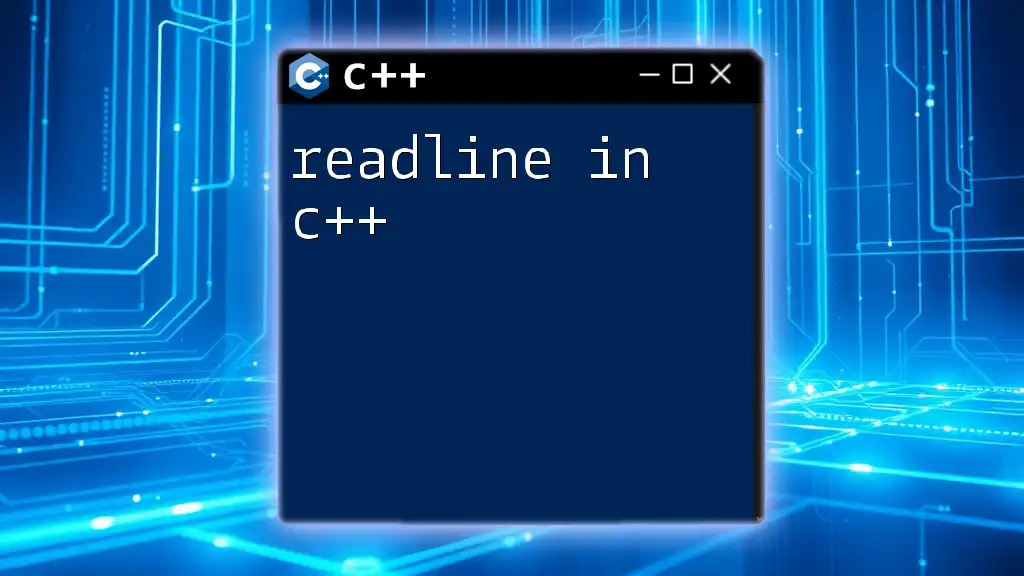
Additional Resources
For further learning, consider exploring the official C++ documentation, as well as reputable books and online courses that delve into C++ standard libraries and advanced data structures.