In C++, methods are functions defined within a class that operate on the class's data members and can be called on objects of that class to perform specific actions or calculations.
Here’s a simple example of a class with a method:
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
};
int main() {
Calculator calc;
int sum = calc.add(5, 3);
}
Understanding Classes and Objects
What is a Class?
A class in C++ is essentially a blueprint for creating objects. It encapsulates data and functions that manipulate that data. Each class can have attributes (variables) and methods (functions) that define its behavior and state. Thus, the class serves as a template from which you can create multiple objects with similar properties.
Here's a simple example of a class definition:
class Dog {
public:
std::string name;
int age;
void bark() {
std::cout << "Woof! My name is " << name << " and I am " << age << " years old." << std::endl;
}
};
What is an Object?
An object is an instance of a class. When you create an object, you allocate memory for it, and it can store its own data. You can think of an object as a specific example of a class.
Here's how you can create an object based on the Dog class above:
Dog myDog;
myDog.name = "Buddy";
myDog.age = 3;
myDog.bark();
In this snippet, `myDog` is an object of the class Dog, and calling `bark()` will execute the method associated with that object.
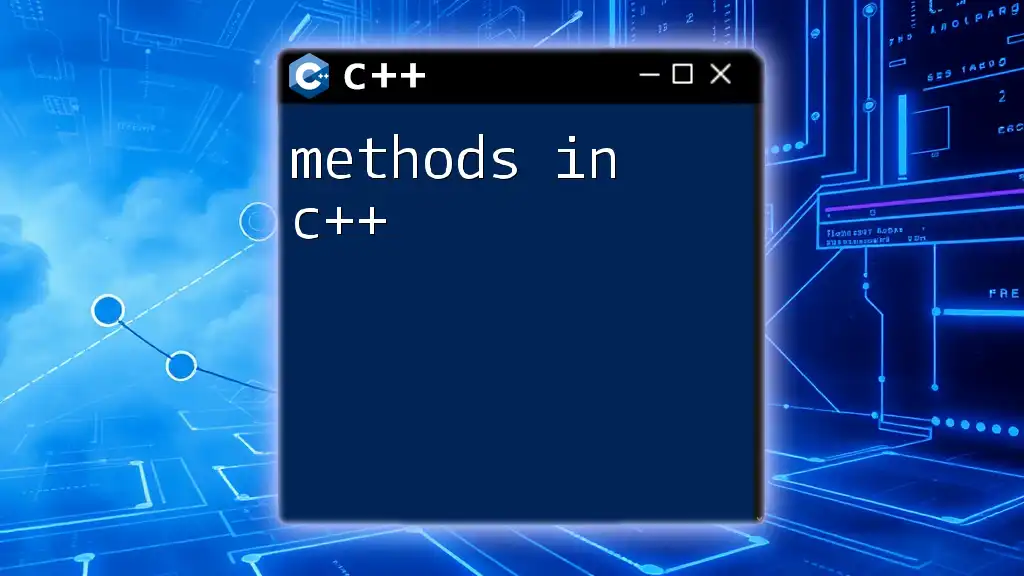
Types of Methods in C++ Class
Member Functions
Member functions are functions defined within a class that operate on objects of that class. They have direct access to the attributes of the class, allowing them to manipulate or retrieve the state of the object.
The syntax for defining a member function includes the return type, function name, parameters, and the function body. Here's an example:
class Rectangle {
public:
int width, height;
int area() {
return width * height;
}
};
In this example, `area()` is a member function that computes and returns the area of the rectangle.
Static Methods
Static methods belong to the class itself rather than any specific object. They can be called without creating an instance of the class and are often used for utility functions that do not need access to object-specific data.
To declare a static method, use the `static` keyword:
class MathUtil {
public:
static int add(int a, int b) {
return a + b;
}
};
You can call a static method like this:
int sum = MathUtil::add(5, 7);
This method does not require an object of MathUtil to be created, demonstrating its independence from the instance's data.
Const Methods
A const method is a member function that guarantees not to modify any member variables of the class. This is important for maintaining data integrity, especially when passing objects around in your application.
To define a const method, add the `const` qualifier after the parameter list:
class Circle {
public:
int radius;
double area() const {
return 3.14 * radius * radius;
}
};
In this example, `area()` is a const method and assures the caller that the method will not modify the state of the Circle object.
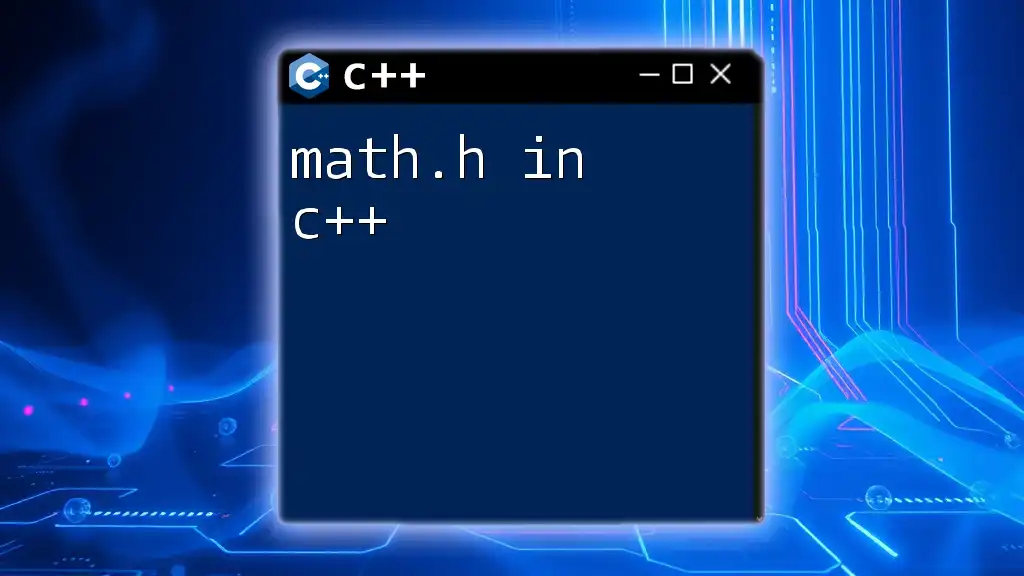
Method Overloading
What is Method Overloading?
Method overloading allows multiple methods in the same class to have the same name but different parameter lists. This is a feature that enables a more intuitive interface by allowing the same method name to be used for different contexts.
How to Overload Methods
When overloading methods, the compiler differentiates them based on the number or type of parameters. Here's an example:
class Print {
public:
void display(int i) {
std::cout << "Integer: " << i << std::endl;
}
void display(double d) {
std::cout << "Double: " << d << std::endl;
}
void display(std::string s) {
std::cout << "String: " << s << std::endl;
}
};
In this example, the `display()` method is overloaded three times, accommodating different types of arguments.
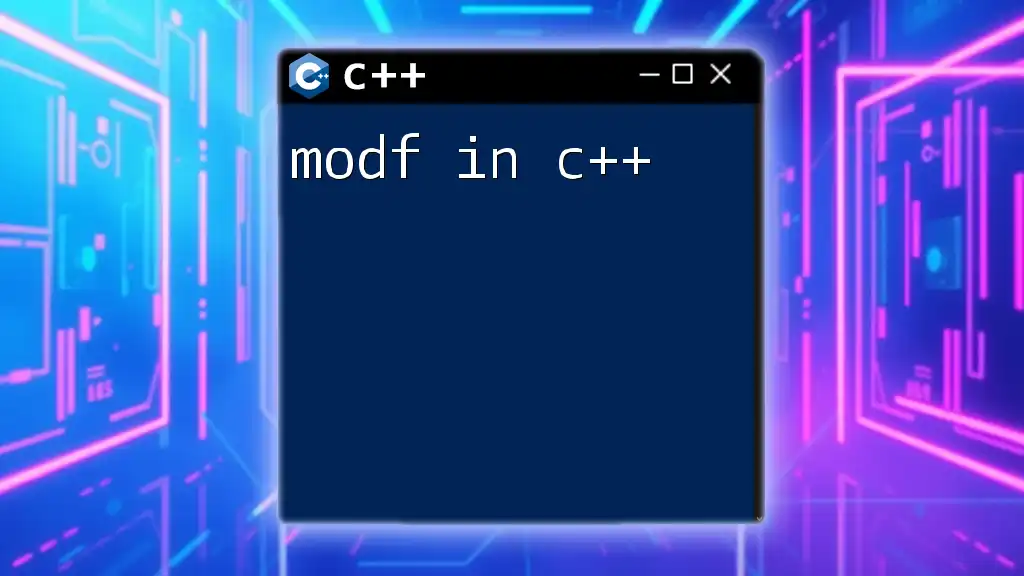
Method Overriding
What is Method Overriding?
Method overriding allows a derived class to provide a specific implementation of a method that is already defined in its base class. This is essential for achieving polymorphism in C++.
How to Override Methods
To override a method, you need to declare the base class method as `virtual`, allowing derived classes to provide their own implementation:
class Animal {
public:
virtual void sound() {
std::cout << "Some generic animal sound." << std::endl;
}
};
class Dog : public Animal {
public:
void sound() override {
std::cout << "Woof!" << std::endl;
}
};
Here, the `sound()` method in the `Dog` class overrides the same method in the `Animal` class, allowing for specific behavior according to the object's type.
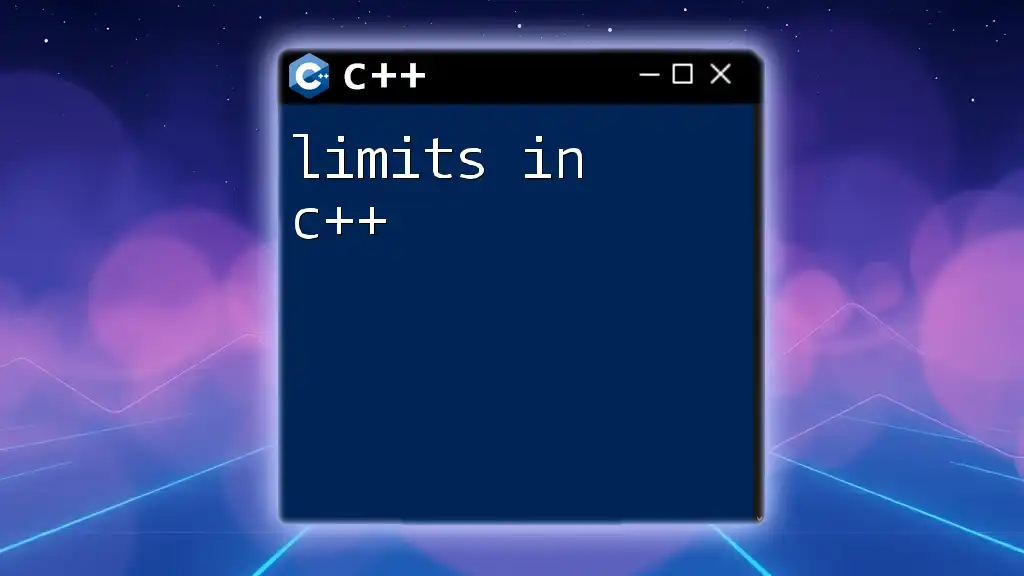
Access Modifiers and Their Impact on Methods
Public Methods
Public methods are accessible from any other part of the program. They define the interface through which other classes, functions, or code can interact with the object's data.
class Car {
public:
void drive() {
std::cout << "Car is driving." << std::endl;
}
};
Private Methods
Private methods are not accessible from outside the class. They are intended for internal use only and help implement the class's functionality without exposing unnecessary details.
class Engine {
private:
void start() {
std::cout << "Engine starting." << std::endl;
}
};
Protected Methods
Protected methods can be accessed in derived classes but are not available outside the class hierarchy. This access modifier strikes a balance between encapsulation and inheritance.
class Vehicle {
protected:
void honk() {
std::cout << "Honking!" << std::endl;
}
};
class Truck : public Vehicle {
public:
void operation() {
honk(); // Accessing protected method
}
};
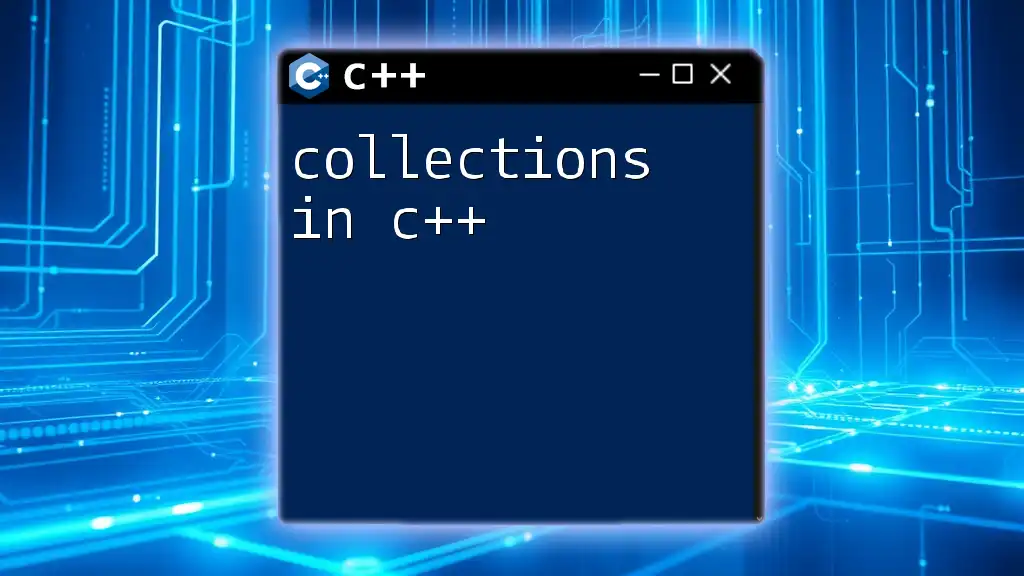
Best Practices for Using Methods in C++
Code Readability and Maintenance
Writing descriptive method names greatly enhances the readability and maintainability of your code. A method name should reflect its functionality clearly, preventing confusion for anyone who reads the code later.
Avoiding Code Duplication
Employ methods to adhere to the DRY principle (Don’t Repeat Yourself). By creating reusable methods, you can abstract repetitive logic into a single place, making your code easier to manage and less error-prone.
Documentation and Comments
Clear documentation and comments around methods explain their purpose and behavior. This practice improves the understanding of the code both for yourself and for others who may work on it in the future. Make sure to comment on complex logic or important details in the implementation.
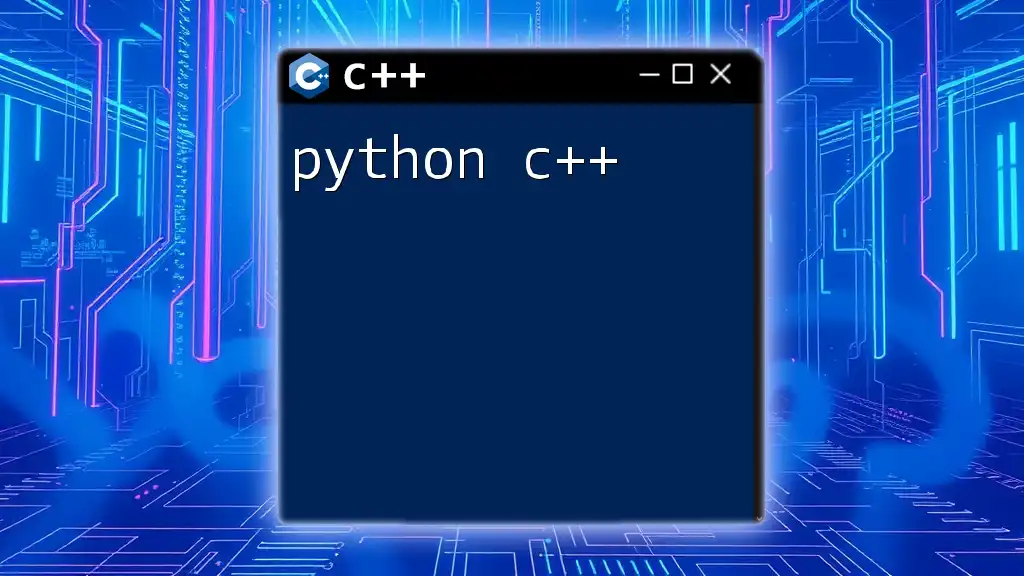
Conclusion
In this comprehensive guide, we explored various aspects of methods in C++ class. We defined classes and objects, delved into different types of methods, and examined important concepts like method overloading and overriding. We also discussed access modifiers and best practices to enhance code quality and maintainability.
By understanding and effectively utilizing methods in C++, you can write more organized, reusable, and efficient code. We encourage you to practice creating your own classes and methods while exploring further resources to deepen your knowledge of C++ programming.