In C++, a class within a class is known as a nested class, which allows you to encapsulate functionality and organize code more effectively.
Here’s a simple example of a nested class in C++:
class OuterClass {
public:
class InnerClass {
public:
void display() {
std::cout << "Hello from InnerClass!" << std::endl;
}
};
void callInner() {
InnerClass inner;
inner.display();
}
};
What Are Classes in C++?
In C++, a class serves as a blueprint for creating objects, encapsulating data for the object in a single entity along with the methods that operate on that data.
A class typically includes:
- Attributes: These are the data members of the class that hold information about the object.
- Methods: Functions defined within a class that provide functionality or responses to actions.
Basic syntax for defining a class looks like this:
class ClassName {
// Attributes
public:
int attribute;
// Methods
public:
void method() {
// Implementation
}
};
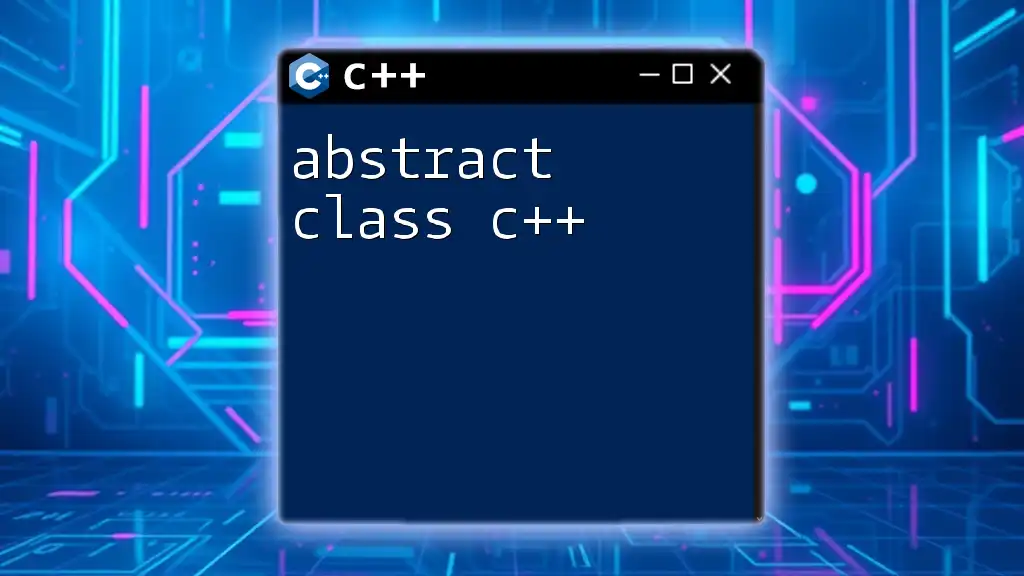
Understanding Nested Classes
Definition of Nested Class
A nested class is a class defined within the scope of another class. This structuring allows the nested class to have access to the members (attributes and methods) of the enclosing (outer) class.
Why Use Nested Classes?
Nested classes can:
- Promote encapsulation, keeping related classes together in a logical part of the code.
- Enhance the readability and organization of code, especially when the inner class is only relevant to the outer class.
- Be advantageous in real-world applications, like implementing bulk data or operations that logically form a cohesive unit.
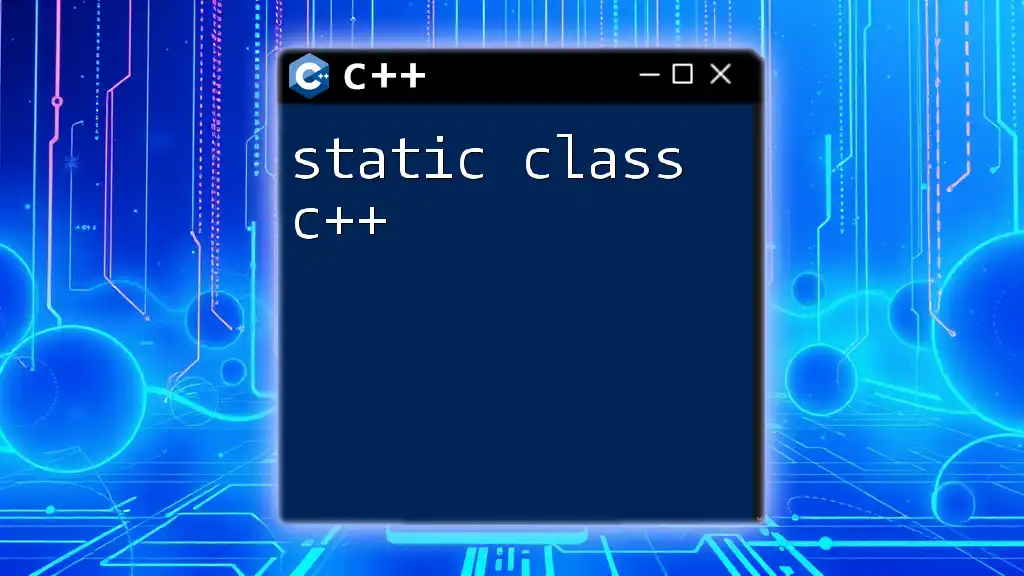
Syntax and Structure of Nested Classes
Basic Syntax for Creating a Nested Class
Here's how to define a nested class:
class OuterClass {
class InnerClass {
// Inner class attributes and methods
};
};
Access Modifiers in Nested Classes
Understanding access modifiers is crucial when working with nested classes.
- Public: Items declared as public can be accessed from outside the class and will also be accessible to the outer class.
- Private: Only accessible within the nested class or outer class.
- Protected: Accessible within the nested class and any derived classes.
This unique structuring allows for better data hiding and provides flexibility to the code.
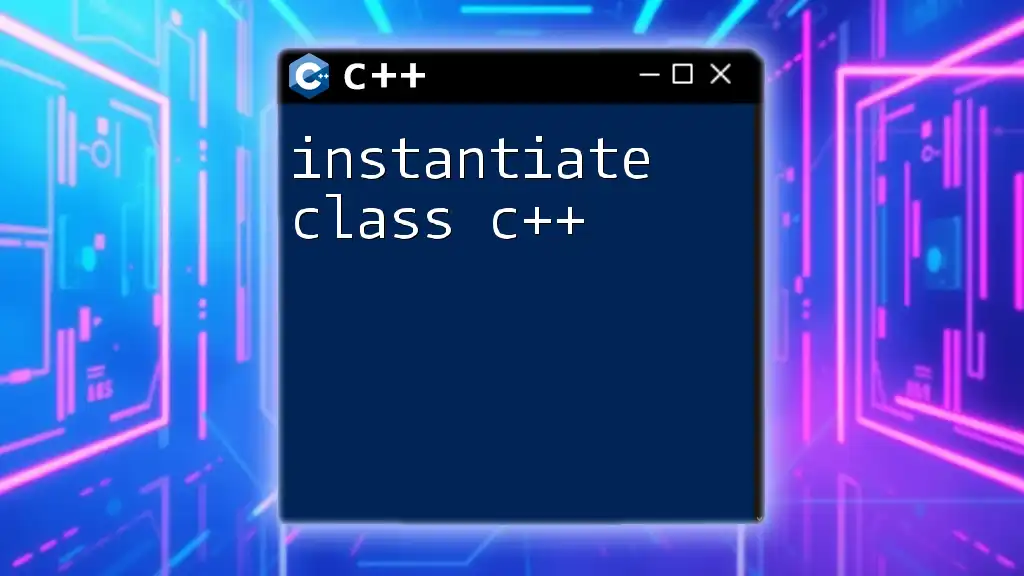
Example of a Class in a Class
Creating an Example: Library System
Let’s go through an example of a library system using a nested class structure.
First, we will define the outer class, `Library`, which holds details specific to a library.
class Library {
private:
string name;
public:
Library(string n) : name(n) {}
// Inner class for Books
class Book {
private:
string title;
public:
Book(string t) : title(t) {}
void display() {
cout << "Book Title: " << title << endl;
}
};
void showLibrary() {
cout << "Library Name: " << name << endl;
}
};
Creating Instances of Nested Classes
Now that we’ve structured our classes, let’s see how we can create instances of these nested classes.
int main() {
Library myLibrary("City Library");
Library::Book myBook("C++ Programming");
myLibrary.showLibrary();
myBook.display();
return 0;
}
Explanation of the Example
In this example, the outer class, `Library`, holds a name, while the inner class, `Book`, holds the title of the book. The inner class is related to the outer class, encapsulating functionality that is specific to libraries and their books. By calling `showLibrary()` and `display()`, we carefully demonstrate the interactions between the classes.
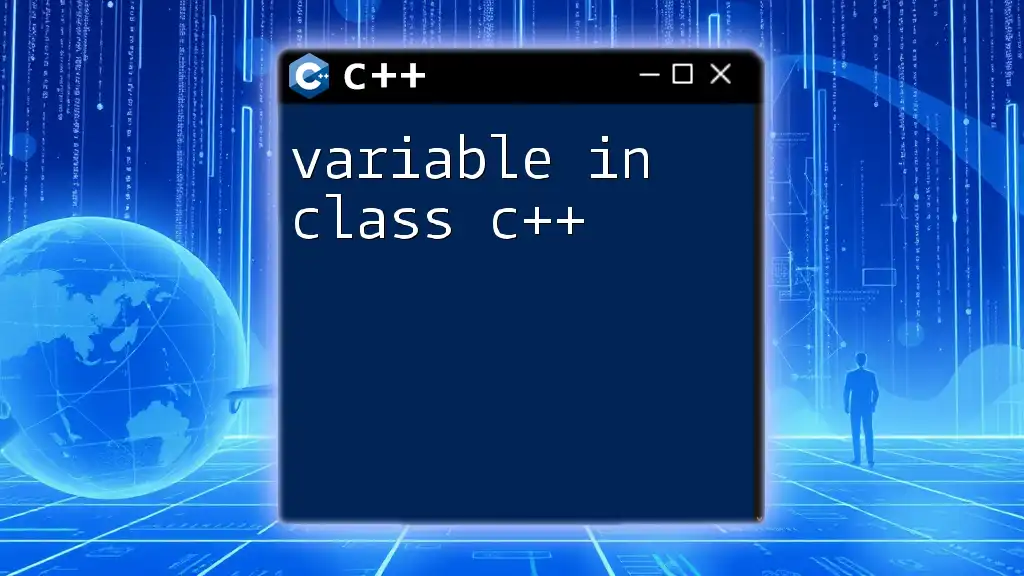
Practical Use Cases for Nested Classes
Complex Data Structures
Nested classes are frequently used in complex data structures, such as trees or graphs. For instance, in a tree data structure, the Node class can be nested inside the Tree class to encapsulate nodes logically related to one another.
Encapsulation and Modular Programming
Nested classes facilitate better encapsulation and modular programming. In GUI frameworks, for example, a Window class may contain Button or Label classes, where these components are only relevant within the context of that specific window, thereby avoiding unnecessary clutter in the global namespace.
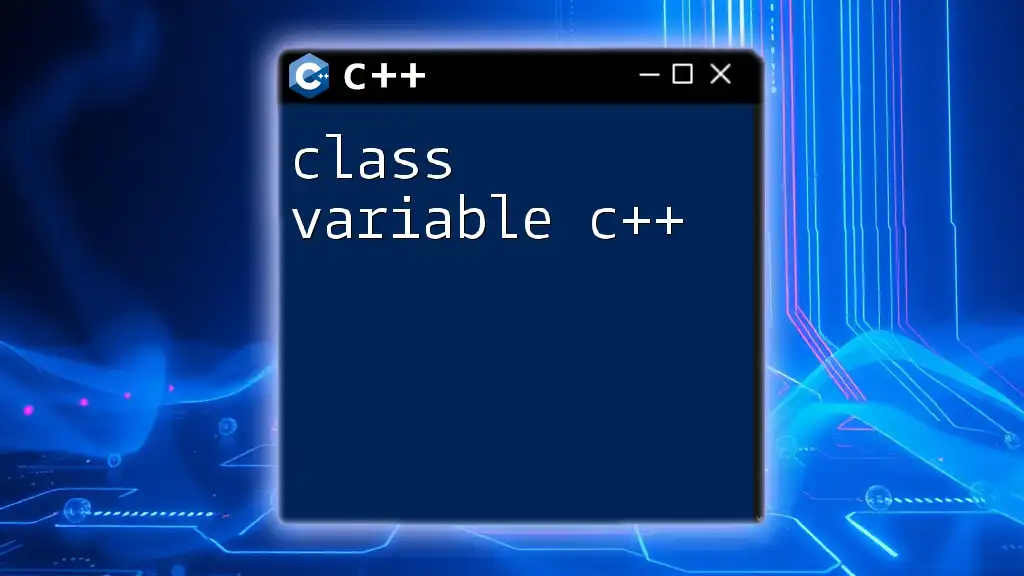
Best Practices for Using Nested Classes
When to Use Nested Classes
Utilize nested classes when:
- You require a class that logically belongs within the context of another.
- You aim to enhance readability or encapsulate closely related functionality.
Avoiding Overuse
While nested classes bring organization, overuse can complicate your codebase. Assess whether the benefits of encapsulation outweigh the added complexity. Prioritize simplicity and clarity when designing your software architecture.
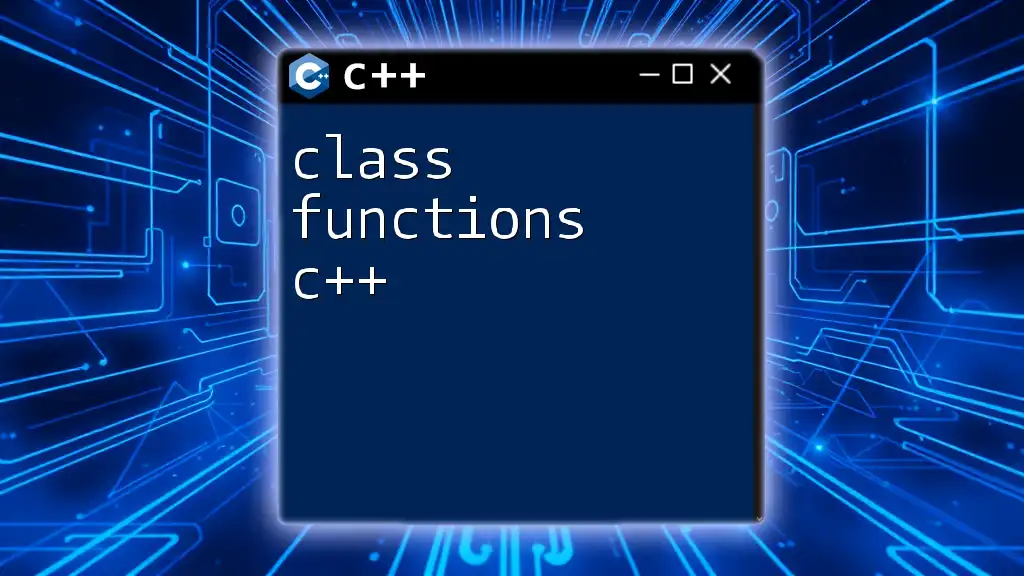
Summary
In this article, we explored the important concept of class in a class C++, also known as nested classes. We discussed the basic structure and syntax, offered illustrative examples, and reviewed practical applications in real-world scenarios. Mastering nested classes can significantly enhance your understanding and effectiveness in C++ programming.
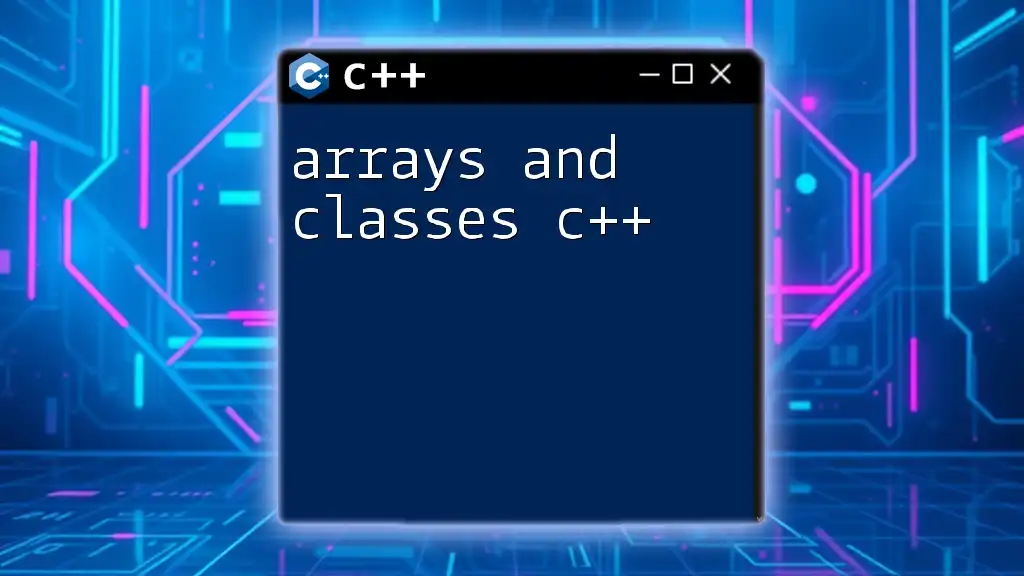
Conclusion
Understanding nested classes deepens your grasp of object-oriented design in C++. They offer a powerful way to structure your code, improve readability, and maintain encapsulation. As you continue your programming journey, experiment with nested classes to see their benefits firsthand.
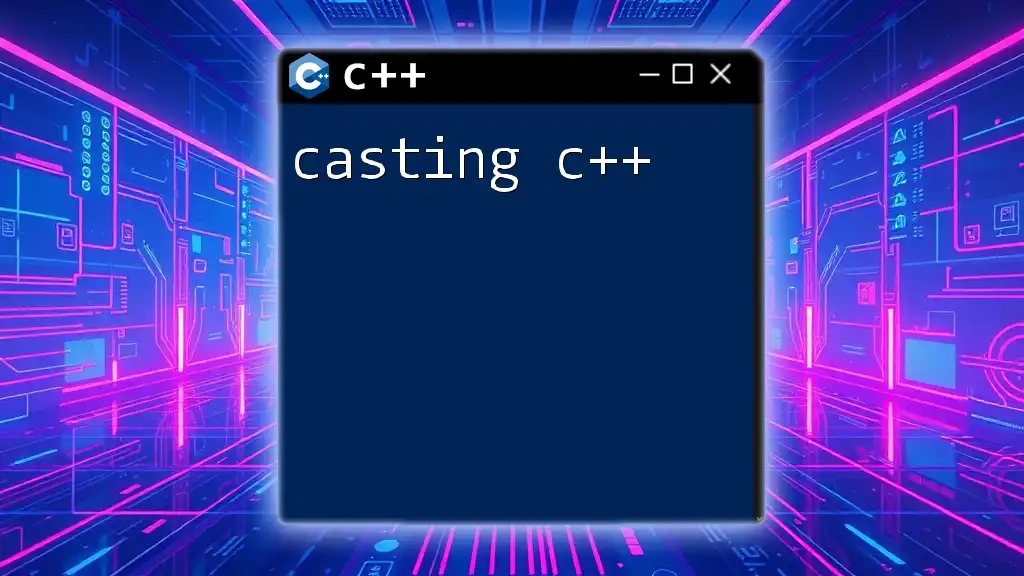
Call-to-Action
Join our C++ community to stay updated on more programming insights and resources!