Hash tables in C++ are data structures that implement an associative array, allowing for efficient data retrieval via keys using a hashing function.
Here’s a simple example of a hash table implementation using `std::unordered_map` in C++:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> hashTable;
hashTable["apple"] = 1;
hashTable["banana"] = 2;
// Accessing elements
std::cout << "apple: " << hashTable["apple"] << std::endl;
std::cout << "banana: " << hashTable["banana"] << std::endl;
return 0;
}
Understanding the Basics of Hash Tables
Definition and Concept
A hash table is a data structure that implements an associative array abstract data type, a structure that can map keys to values. In other words, it allows for the storage and retrieval of values based on their associated keys.
At the core of a hash table is the hash function, which computes an index into an array of buckets or slots, from which the desired value can be found. The keys are processed through the hash function to generate a unique index for efficient access.
Advantages of Using Hash Tables
The unique design of hash tables provides several advantages:
- Fast Lookups and Insertions: Hash tables achieve average time complexity of O(1) for lookups, inserts, and deletions, making them highly efficient for storing large datasets.
- Efficient Memory Management: By using arrays and linked lists, hash tables can optimize memory usage effectively, allowing dynamic scalability without wasting space.
- Comparison with Other Data Structures: Compared to arrays and linked lists, hash tables excel in search speed. While arrays offer O(n) search time, and linked lists provide O(n) time for searching and insertion, hash tables remain agile.
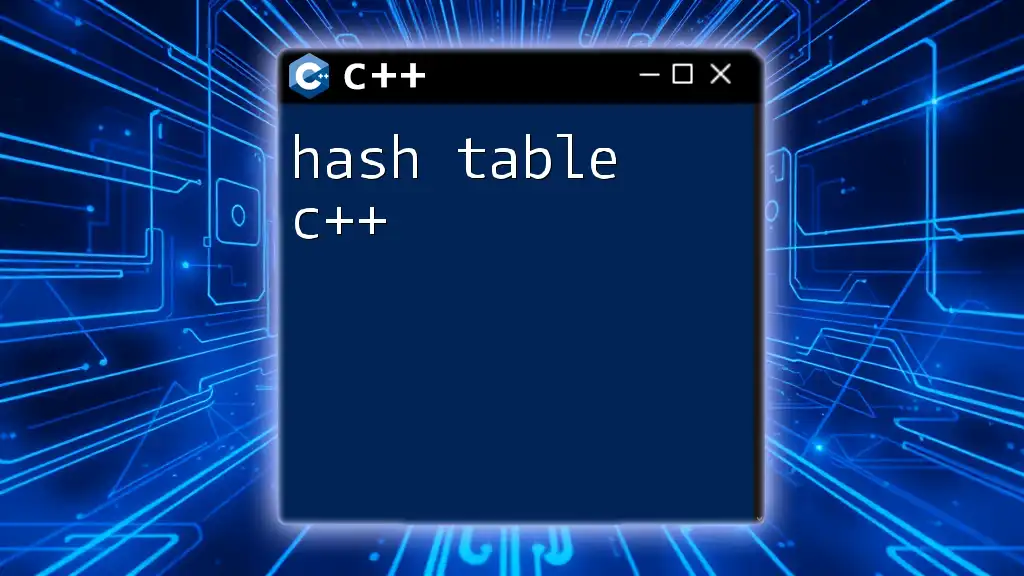
Implementing Hash Tables in C++
The Basic Structure of a Hash Table
To implement a hash table in C++, it is essential to understand its main components: an array to store the data, keys, and values; and a hash function for mapping keys to their respective indices.
Writing a Basic Hash Table Class
Class Definition
In C++, you can start by defining a hash table class. This class will include essential data members like key, value, size, and capacity. Below is a simple outline for your hash table class:
class HashTable {
public:
HashTable(int size);
~HashTable();
void insert(int key, int value);
int search(int key);
void remove(int key);
private:
int capacity;
std::pair<int, int>* table; // Pair of key and value
};
Implementing the Constructor
The constructor initializes the data members and allocates memory for the hash table:
HashTable::HashTable(int size) : capacity(size) {
table = new std::pair<int, int>[capacity];
for (int i = 0; i < capacity; i++) {
table[i] = std::make_pair(-1, -1); // Default values indicating empty slots
}
}
Creating Hash Function
A good hash function is pivotal for the performance of a hash table. It should distribute keys uniformly across the available indices to minimize collisions.
Example of a Simple Hash Function
You can use a simple modulo operation to define a hash function:
int hashFunction(int key) {
return key % capacity; // Simple modulo for index
}
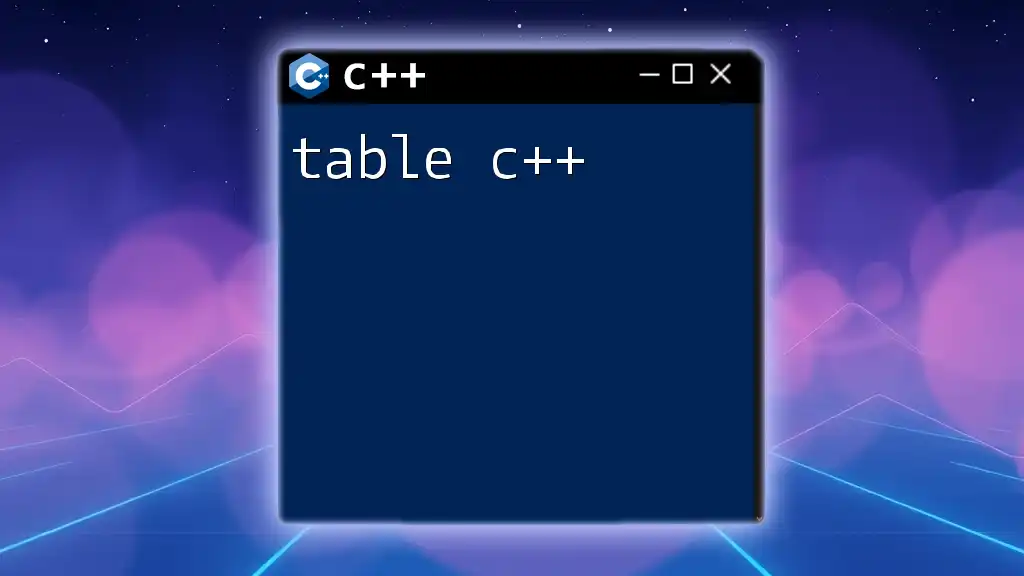
Managing Collisions in Hash Tables
Understanding Collisions
A collision occurs when two keys hash to the same index in the hash table. If two different keys generate the same hash value, it’s essential to have a robust strategy to manage this situation effectively.
Collision Resolution Techniques
Separate Chaining
This technique involves using a linked list (or similar structure) at each index to hold all keys that hash to the same index. When inserting a new key, if a collision is detected, the key-value pair is simply appended to the linked list.
void HashTable::insert(int key, int value) {
int index = hashFunction(key);
// Check if the slot is empty
if (table[index].first == -1) {
table[index] = std::make_pair(key, value); // Insert new key-value pair
} else {
// Handle collision by chaining
// This could involve further extending the definition to linked lists
}
}
Open Addressing
In this method, if a collision occurs, you find the next available slot using probing techniques. Some common probing techniques are:
- Linear Probing: Simply checks the next consecutive slot until an empty one is found.
- Quadratic Probing: Uses a quadratic function to determine the next slot.
- Double Hashing: Uses a secondary hash function to find the next probing index.
int HashTable::search(int key) {
int index = hashFunction(key);
while (table[index].first != -1) {
if (table[index].first == key) {
return table[index].second; // Return associated value
}
index = (index + 1) % capacity; // Linear probing
}
return -1; // Key not found
}
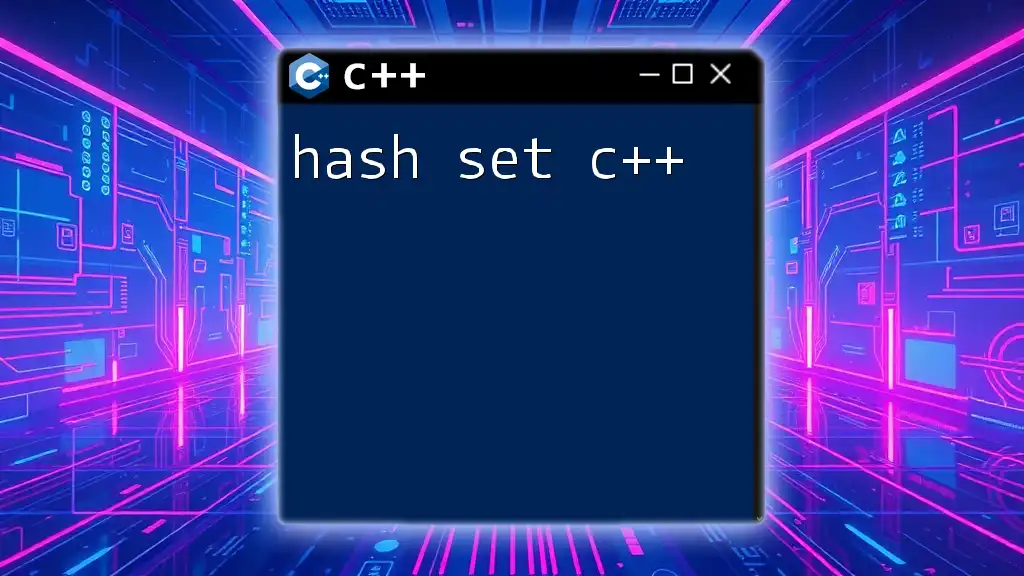
Advanced Hash Table Features
Dynamic Resizing
As a hash table gains more entries, it may become necessary to resize it to maintain performance. Resizing typically involves creating a new, larger hash table and rehashing all existing entries into this new table.
void HashTable::resize() {
int oldCapacity = capacity;
capacity *= 2; // Double the size
std::pair<int, int>* oldTable = table;
table = new std::pair<int, int>[capacity];
for (int i = 0; i < capacity; i++) {
table[i] = std::make_pair(-1, -1); // Reset new table
}
for (int i = 0; i < oldCapacity; i++) {
// Re-insert elements from old table
if (oldTable[i].first != -1) {
insert(oldTable[i].first, oldTable[i].second);
}
}
delete[] oldTable; // Clean up old table
}
Making Hash Tables More Robust
When designing your hash table, consider extending its functionality:
- Handling Deletions: Implement methods to remove key-value pairs from the hash table, which often requires careful handling to maintain the integrity of the structure.
- Best Practices: Optimize the choice of the initial table size and the hash function. Aim for a load factor that balances memory usage and performance.
- Performance Optimization Tips: Regularly test and benchmark your hash table's performance, and adjust capacity as necessary.
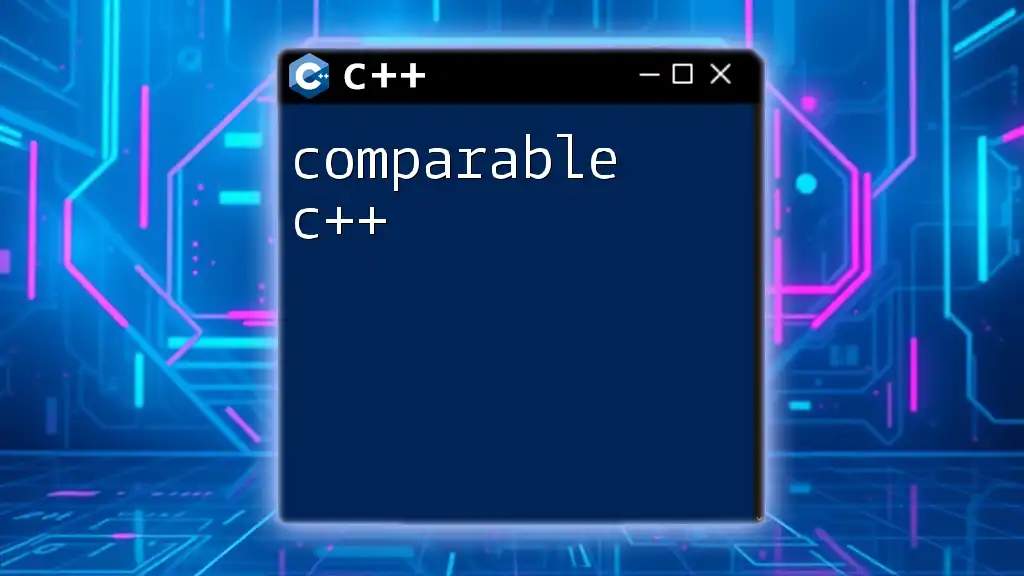
Practical Applications of Hash Tables in C++
Hash tables are widely employed as foundational constructs in various real-world systems.
Use Cases in Real-World Applications
- Caching: Hash tables efficiently store cached data, enabling quick retrieval in applications like web development.
- Database Indexing: They help in indexing and enabling rapid searches in databases.
- Frequency Counting: By mapping items to their counts, hash tables can be used for applications like frequency analyzers.
Example Scenario: Implementing a Simple Phonebook
Imagine creating a phonebook application where names act as keys and phone numbers as values. Using a hash table, users can quickly find a phone number by searching for a name, demonstrating the utility of hash tables in real life.
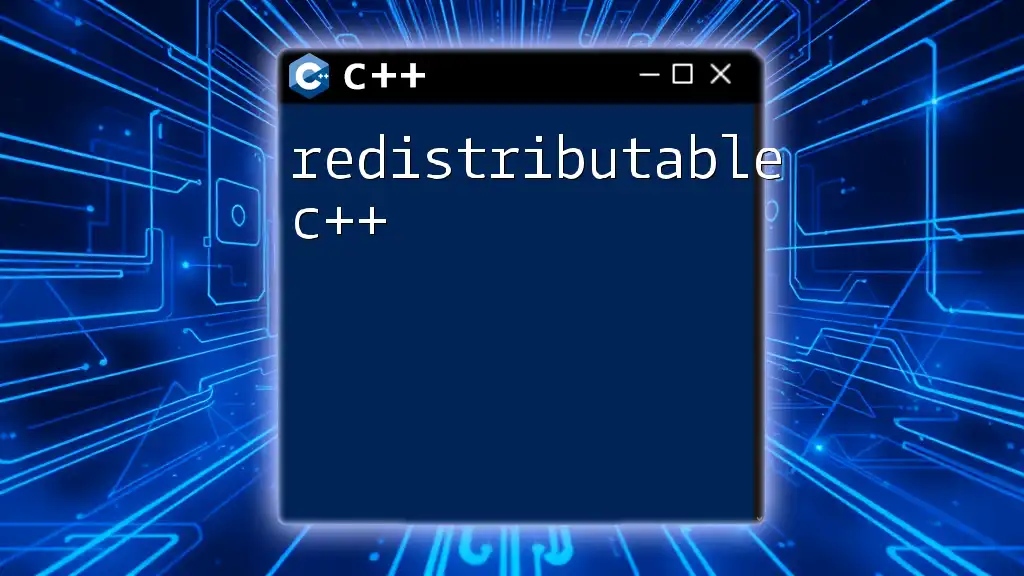
Conclusion
In summary, hash tables in C++ provide a powerful and efficient way to store and manage data. By understanding their structure, implementation, collision management, and practical applications, you can leverage hash tables effectively in your programming projects. As you continue to explore the depths of C++, consider building your own implementations and experimenting with advanced features to deepen your understanding.
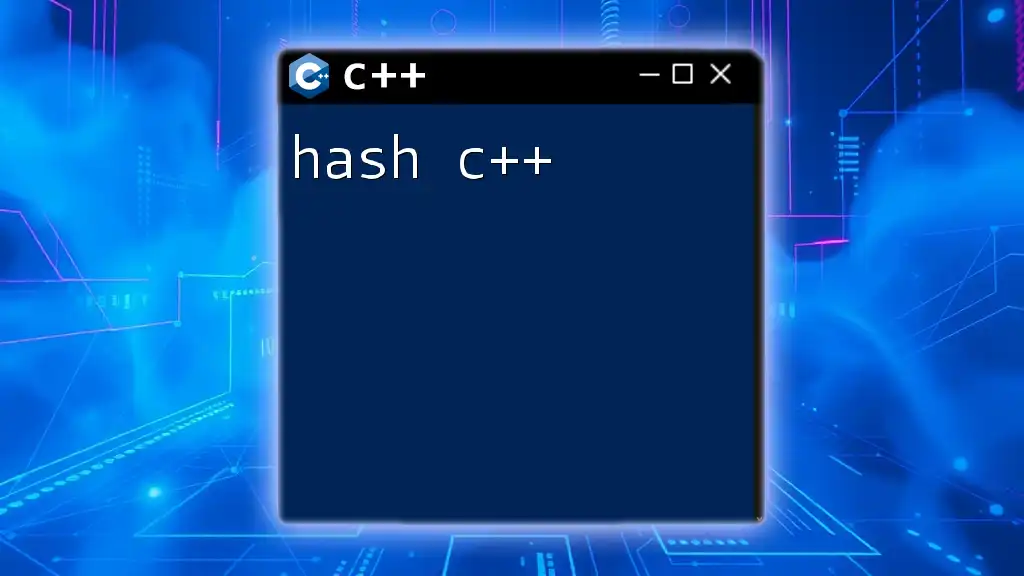
References
For further reading on hash tables, consider exploring various programming textbooks and online tutorials dedicated to data structures and algorithms in C++.
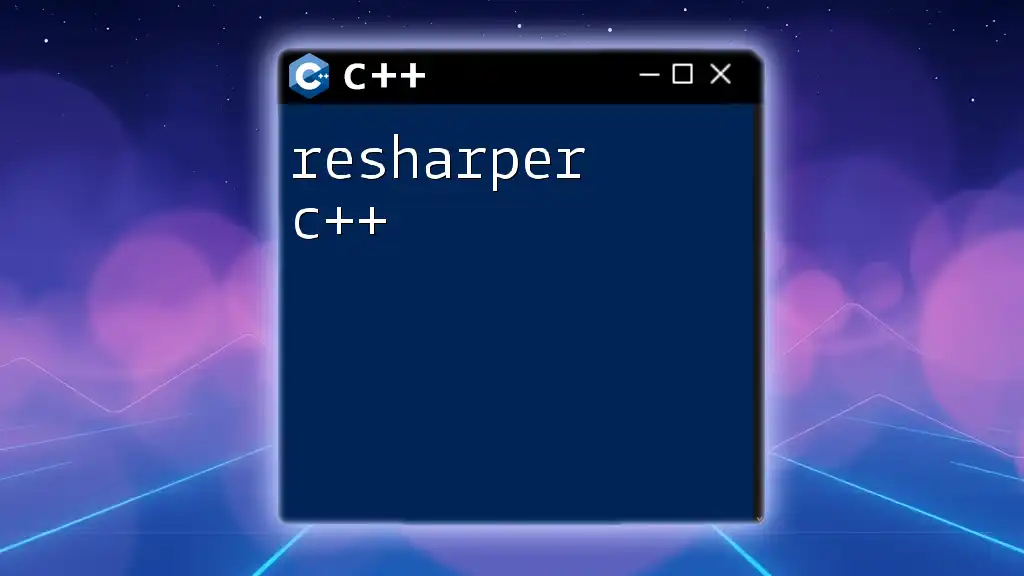
Call to Action
If you’re eager to learn more about C++ and enhance your programming skills, feel free to join our training courses and start your journey toward mastering C++ commands today! Share your experiences and projects using hash tables, and let’s learn together!